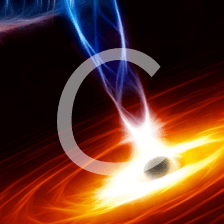
Linked List
文章平均质量分 54
Yingying_code
这个作者很懒,什么都没留下…
展开
-
237. Delete Node in a Linked List*
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node. Supposed the linked list is 1 -> 2 -> 3 -> 4 and you are given the third node with value原创 2017-01-05 18:06:40 · 197 阅读 · 0 评论 -
328. Odd Even Linked List**
Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes. You should try to do it in原创 2017-01-08 17:55:23 · 241 阅读 · 0 评论 -
109. Convert Sorted List to Binary Search Tree**
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST. My code: public TreeNode sortedListToBST(ListNode head) { if(head==null) ret原创 2017-01-08 18:20:32 · 210 阅读 · 0 评论 -
92. Reverse Linked List II**
Reverse a linked list from position m to n. Do it in-place and in one-pass. For example: Given 1->2->3->4->5->NULL, m = 2 and n = 4, return 1->4->3->2->5->NULL. Note: Given m, n satisfy t原创 2017-01-08 18:49:28 · 202 阅读 · 0 评论 -
2. Add Two Numbers*
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return i原创 2017-01-08 19:59:15 · 207 阅读 · 0 评论 -
445. Add Two Numbers II**
You are given two non-empty linked lists representing two non-negative integers. The most significant digit comes first and each of their nodes contain a single digit. Add the two numbers and return i原创 2017-01-08 20:29:29 · 216 阅读 · 0 评论 -
61. Rotate List**
Given a list, rotate the list to the right by k places, where k is non-negative. For example: Given 1->2->3->4->5->NULL and k = 2, return 4->5->1->2->3->NULL. public ListNode rotateRight(L原创 2017-01-08 20:42:30 · 193 阅读 · 0 评论 -
82. Remove Duplicates from Sorted List II**
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list. For example, Given 1->2->3->3->4->4->5, return 1->2->5. Given 1->原创 2017-01-08 21:57:20 · 206 阅读 · 0 评论 -
382. Linked List Random Node**
Given a singly linked list, return a random node's value from the linked list. Each node must have the same probability of being chosen. Follow up: What if the linked list is extremely large and i原创 2017-01-16 23:46:28 · 241 阅读 · 0 评论 -
142. Linked List Cycle II**
Given a linked list, return the node where the cycle begins. If there is no cycle, return null. Note: Do not modify the linked list. Follow up: Can you solve it without using extra space?原创 2017-01-08 16:37:06 · 221 阅读 · 0 评论 -
143. Reorder List**
Given a singly linked list L: L0→L1→…→Ln-1→Ln, reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→… You must do this in-place without altering the nodes' values. For example, Given {1,2,3,4}, reorder it原创 2017-01-08 16:17:06 · 246 阅读 · 0 评论 -
147. Insertion Sort List**
Sort a linked list using insertion sort. public ListNode insertionSortList(ListNode head) { ListNode helper = new ListNode(0); ListNode pre = helper; ListNode cur =head;原创 2017-01-05 22:04:00 · 212 阅读 · 0 评论 -
141. Linked List Cycle*
Given a linked list, determine if it has a cycle in it. Follow up: Can you solve it without using extra space? public boolean hasCycle(ListNode head) { if(head==null) return false;原创 2017-01-05 22:22:15 · 194 阅读 · 0 评论 -
21. Merge Two Sorted Lists*
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists. My code: public ListNode mergeTwoLists(ListNode l1,原创 2017-01-06 23:30:05 · 176 阅读 · 0 评论 -
160. Intersection of Two Linked Lists*
Write a program to find the node at which the intersection of two singly linked lists begins. For example, the following two linked lists: A: a1 → a2 ↘原创 2017-01-06 23:46:40 · 205 阅读 · 0 评论 -
83. Remove Duplicates from Sorted List*
Given a sorted linked list, delete all duplicates such that each element appear only once. For example, Given 1->1->2, return 1->2. Given 1->1->2->3->3, return 1->2->3. My code: public Li原创 2017-01-06 23:55:19 · 199 阅读 · 0 评论 -
203. Remove Linked List Elements**
Remove all elements from a linked list of integers that have value val. Example Given: 1 --> 2 --> 6 --> 3 --> 4 --> 5 --> 6, val = 6 Return: 1 --> 2 --> 3 --> 4 --> 5 My code: public Lis原创 2017-01-07 00:04:59 · 220 阅读 · 0 评论 -
234. Palindrome Linked List*
Given a singly linked list, determine if it is a palindrome. Follow up: Could you do it in O(n) time and O(1) space? public class Solution { public boolean isPalindrome(ListNode head) {原创 2017-01-08 15:09:50 · 234 阅读 · 0 评论 -
24. Swap Nodes in Pairs*
Given a linked list, swap every two adjacent nodes and return its head. For example, Given 1->2->3->4, you should return the list as 2->1->4->3. Your algorithm should use only constant space. Y原创 2017-01-08 15:42:17 · 198 阅读 · 0 评论 -
206. Reverse Linked List*
Reverse a singly linked list. My code: Iteratively: public ListNode reverseList(ListNode head) { if(head==null) return head; ListNode pre = null; ListNode cur = head;原创 2017-01-08 14:53:22 · 237 阅读 · 0 评论