前期准备:
在企业微信群聊里面添加机器人,获取Webhook地址
脚本工作目录下准备花名册文件,如果有入离职记得更新
设置windows定时任务(可参考:https://jingyan.baidu.com/article/9080802200cc15fd91c80fcf.html)
测试截图:
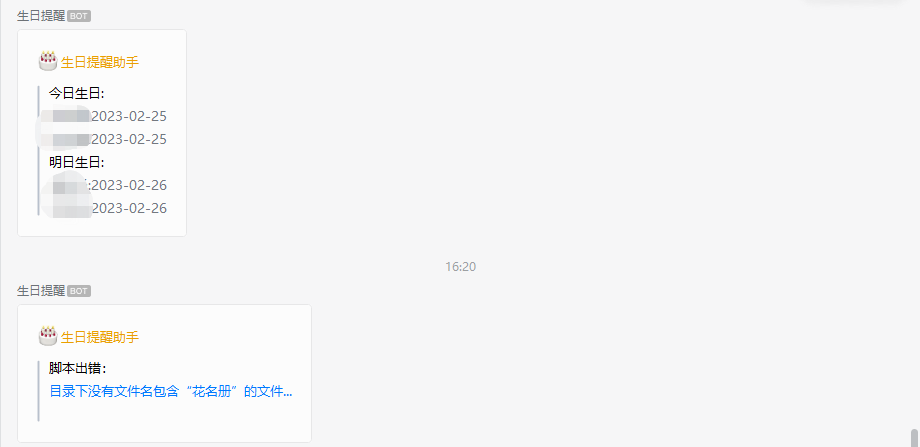
脚本代码:
# -*- coding: utf-8 -*-
import os
import re
import sys
import xlrd
import time
import datetime
import requests
import json
from plyer import notification
Webhook = "" #Webhook地址
def window_send(text,second):
notification.notify(
title="🎂生日提醒助手",
message=text,
app_icon="",
timeout=second
)
def right_send(birth_today,birth_nextday):
oWX_URL = Webhook
sent_msg = {
"msgtype": "markdown",
"markdown": {
"content": f'''<font color="warning">🎂生日提醒助手</font>\n> 今日生日:\n<font color="comment">{birth_today}</font>\n> 明日生日:\n<font color="comment">{birth_nextday}</font>'''
}
}
print(sent_msg)
headers = {'Content-Type': 'application/json'}
try:
r = requests.post(url=oWX_URL, data=json.dumps(sent_msg), headers=headers, timeout=5)
except Exception as e:
print(e.args[0])
def wrong_send(text):
oWX_URL = Webhook
sent_msg = {
"msgtype": "markdown",
"markdown": {
"content": f'''<font color="warning">🎂生日提醒助手</font>\n> 注意:\n<font color="Blue">{text}</font>\n>'''
}
}
print(sent_msg)
headers = {'Content-Type': 'application/json'}
try:
r = requests.post(url=oWX_URL, data=json.dumps(sent_msg), headers=headers, timeout=5)
except Exception as e:
print(e.args[0])
def main():
if not os.path.isdir("history"):
os.mkdir("history")
open(f"./history/{str(datetime.datetime.today()).split(' ')[0]}","w+")
print("注意事项:\n文件夹下有文件名包含“花名册”的表格,且存在“在职员工”sheet\n标题行必须包含“姓名”,“证件号”或者“身份证”\n如果今明两日有人过生日才会发送消息提醒...")
num = 0
#print(os.path.dirname(os.path.realpath(sys.executable)))
print(os.path.dirname(os.path.realpath(sys.argv[0])))
files = os.listdir(os.path.dirname(os.path.realpath(sys.argv[0])))
print(files)
print(re.findall('花名册',str(files)))
print(str(re.findall('花名册',str(files))))
if re.findall('花名册',str(files)) == []:
wrong_send("目录下没有文件名包含“花名册”的文件...")
time.sleep(15)
try:
for file in files:
if '花名册' in file:
workbook = xlrd.open_workbook(str(os.path.dirname(os.path.realpath(sys.argv[0])))+ "/" + file)
print("目录下有此花名册:" + str(file))
sheet = workbook.sheet_by_name('在职员工')
row_1 = sheet.row_values(0)
print(row_1)
if '证件号' in row_1:
a = row_1.index('证件号')
elif '身份证' in row_1:
a = row_1.index('身份证')
b = row_1.index('姓名')
birth_today = ""
birth_nextday = ""
for i in range(1, sheet.nrows):
row_i = sheet.row_values(i)
name = row_i[b]
id_num = str(datetime.datetime.today().year) + row_i[a][10:14]
if len(id_num) == 8:
birth_date = datetime.datetime.strptime(id_num, '%Y%m%d').date()
#今日生日
if birth_date == datetime.date.today():
birth_today = birth_today + row_i[b] + ':' + str(birth_date) + '\n'
num += 1
#明日生日
elif birth_date == datetime.date.today() + datetime.timedelta(days=1):
birth_nextday =birth_nextday + row_i[b] + ':' + str(birth_date) + '\n'
num += 1
print(num)
if num != 0:
birth_today = birth_today.strip("\n")
birth_nextday = birth_nextday.strip("\n")
right_send(birth_today,birth_nextday)
try:
xinxi = f"今日生日:\n{birth_today}\n明日生日:\n{birth_nextday}"
window_send(xinxi,600)
except Exception as e:
print(e.args[0])
else:
print("没人过生日~")
wrong_send("最近没人过生日(推送是为了检测脚本是否每天定时运行~)")
except Exception as e:
print(str(e))
wrong_send(str(e))
time.sleep(15)
if __name__ == '__main__':
main()