翻转二叉树
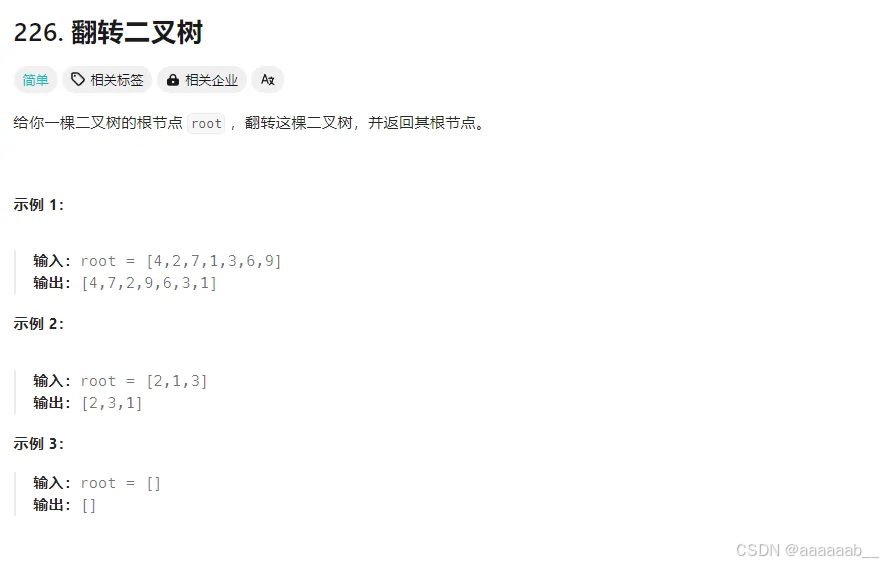
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if(root == NULL) return root;
swap(root->left, root->right);
invertTree(root->left);
invertTree(root->right);
}
};
对称二叉树
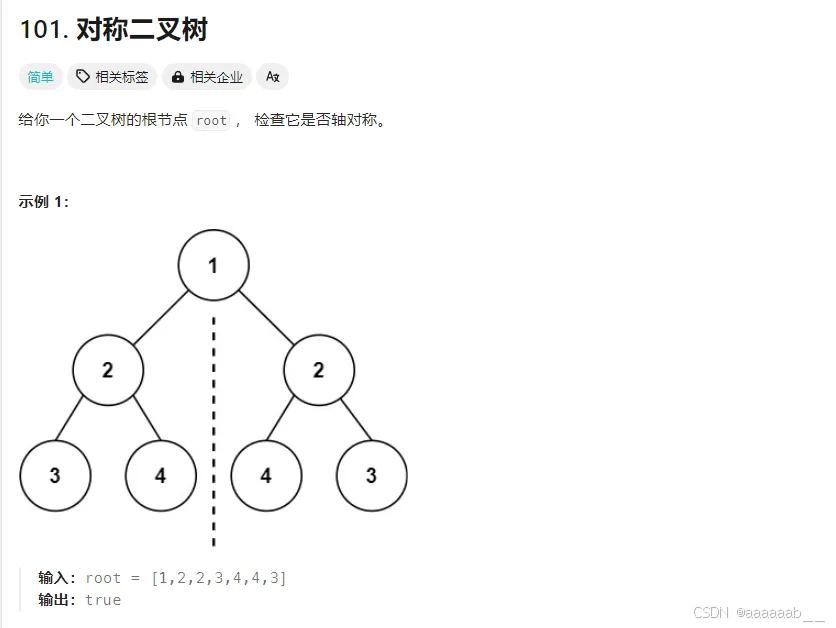
class Solution {
public:
bool compare(TreeNode* left, TreeNode* right)
{
if(left == NULL && right != NULL) return false;
else if(left != NULL && right == NULL) return false;
else if(left == NULL && right == NULL) return true;
else if(left->val != right->val) return false;
bool outside = compare(left->left, right->right);
bool inside = compare(left->right, right->left);
bool bSame = outside&&inside;
return bSame;
}
bool isSymmetric(TreeNode* root) {
if (root == NULL) return false;
return compare(root->left, root->right);
}
};
二叉树的最大深度
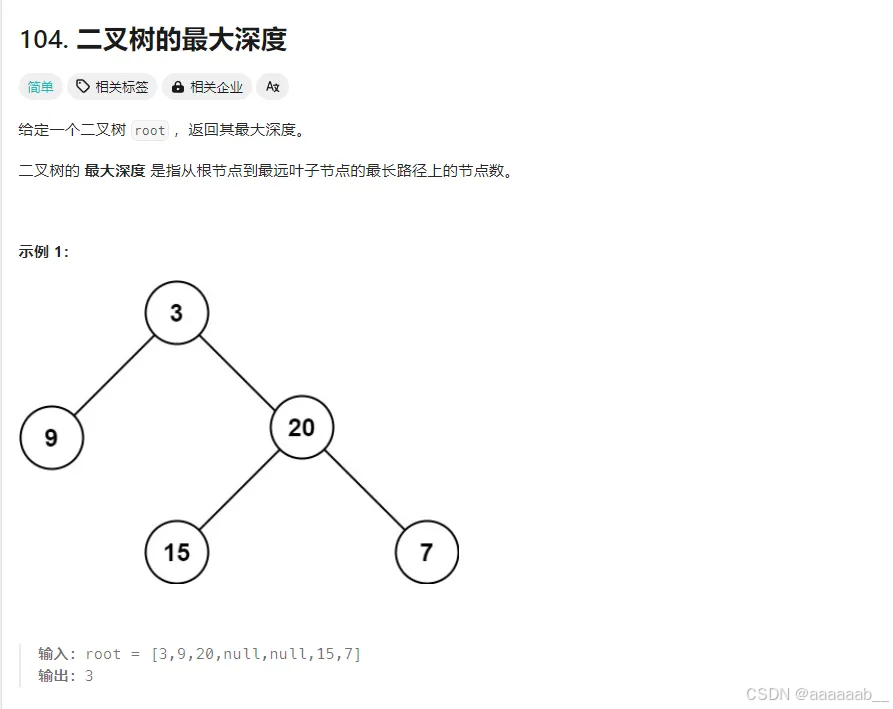
class Solution {
public:
int getDepth(TreeNode *root)
{
if(root == NULL) return 0;
int iLeft = getDepth(root->left);
int iRight = getDepth(root->right);
int depth = 1 + max(iLeft, iRight);
return depth;
}
int maxDepth(TreeNode* root) {
if(root == NULL) return 0;
return getDepth(root);
}
};
二叉树的最小深度
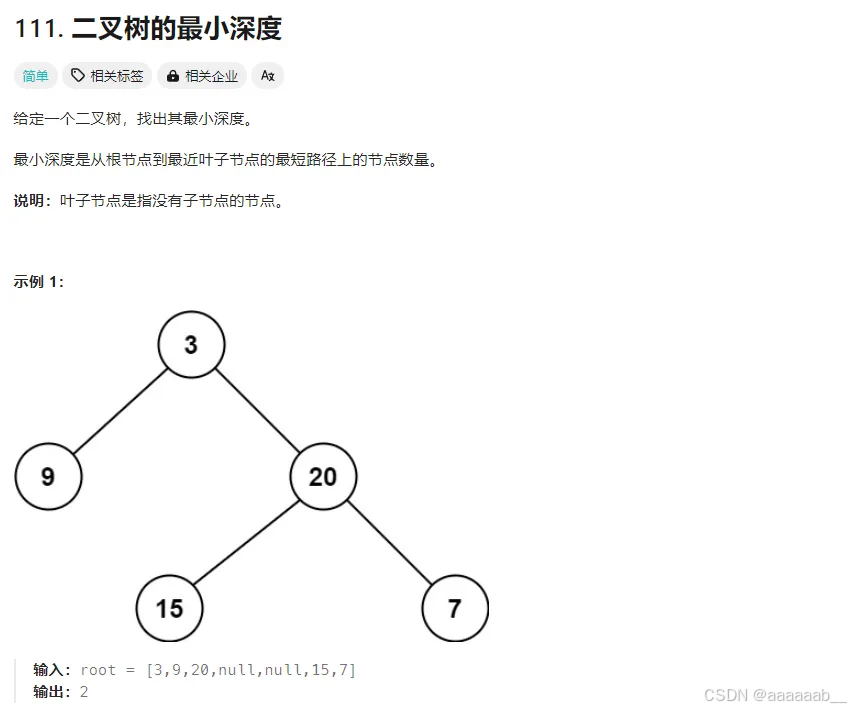
class Solution {
public:
int getMinDepth(TreeNode* root)
{
if(root == NULL) return 0;
int iLeft = getMinDepth(root->left);
int iRight = getMinDepth(root->right);
if(root->left == NULL && root->right != NULL)
return 1+iRight;
if(root->left != NULL && root->right == NULL)
return 1+iLeft;
return 1+min(iLeft,iRight);
}
int minDepth(TreeNode* root) {
if(root == NULL) return 0;
return getMinDepth(root);
}
};