HTTPS
- HTTPS : Hyper Text Transfer Protocol over Secure Socket Layer,是以安全为目标的HTTP通道,简单讲是HTTP的安全版.即HTTP下加入SSL层,HTTPS的安全基础是SSL.
- SSL : Secure Sockets Layer,表示安全套接层.
- TLS : Transport Layer Security,是SSL的继任者,表示传输层安全.
- SSL与TLS是为网络通信提供安全及数据完整性的一种安全协议。TLS与SSL在传输层对网络连接进行加密.
HTTPS和HTTP区别
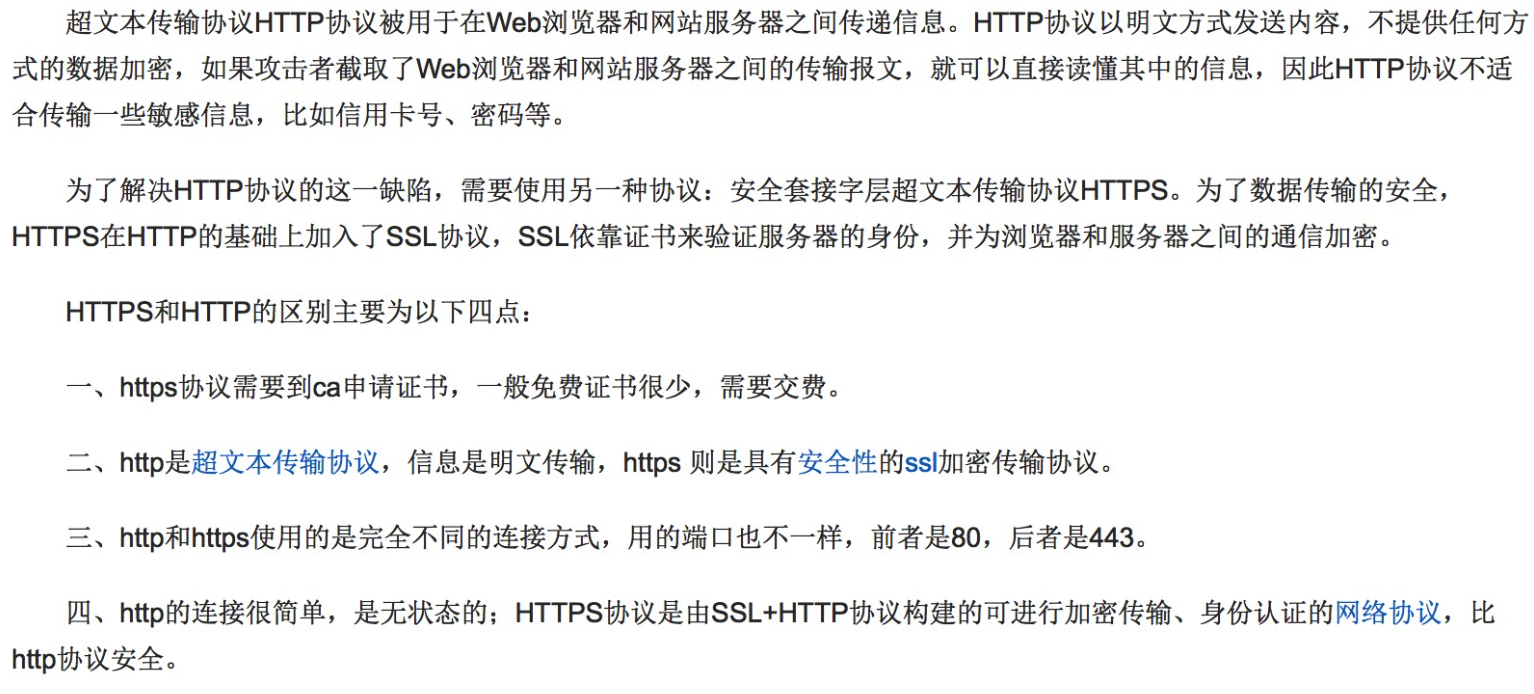
HTTPS访问图解
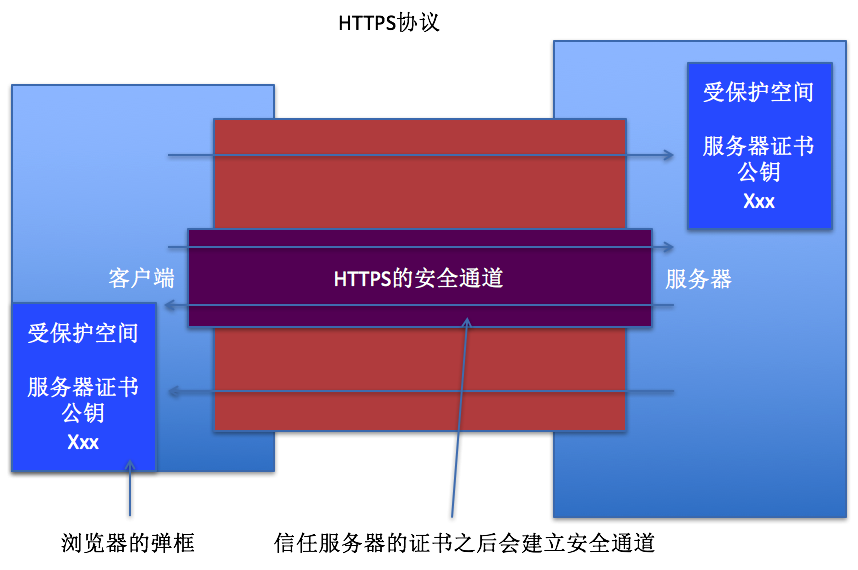
NSURLSession 实现 HTTPS 访问
#import "ViewController.h"
@interface ViewController () <NSURLSessionDataDelegate>
@property (nonatomic, strong) NSURLSession *session;
@end
@implementation ViewController
- (NSURLSession *)session {
if (_session == nil) {
NSURLSessionConfiguration *config = [NSURLSessionConfiguration defaultSessionConfiguration];
_session = [NSURLSession sessionWithConfiguration:config delegate:self delegateQueue:nil];
}
return _session;
}
- (void)viewDidLoad {
[super viewDidLoad];
}
- (void)viewWillDisappear:(BOOL)animated {
[super viewWillDisappear:animated];
[self.session finishTasksAndInvalidate];
}
- (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event {
[self HTTPSDemo];
}
- (void)HTTPSDemo {
NSURL *URL = [NSURL URLWithString:@"https://mail.itcast.cn"];
NSURLSessionDataTask *dataTask = [self.session dataTaskWithURL:URL completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) {
if (error == nil && data != nil) {
NSString *html = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
NSLog(@"%@",html);
}else {
NSLog(@"%@",error);
}
}];
[dataTask resume];
}
#pragma NSURLSessionDataDelegate
- (void)URLSession:(NSURLSession *)session task:(NSURLSessionTask *)task
didReceiveChallenge:(NSURLAuthenticationChallenge *)challenge
completionHandler:(void (^)(NSURLSessionAuthChallengeDisposition disposition, NSURLCredential * __nullable credential))completionHandler {
if ([challenge.protectionSpace.authenticationMethod isEqualToString:NSURLAuthenticationMethodServerTrust]) {
NSURLCredential *credential = [NSURLCredential credentialForTrust:challenge.protectionSpace.serverTrust];
completionHandler(NSURLSessionAuthChallengeUseCredential,credential);
}
}
@end
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
AFNetworking之HTTPS请求
- (void)loadData {
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager];
manager.securityPolicy.validatesDomainName = NO;
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/json", @"text/json", @"text/javascript", @"text/html" ,nil];
manager.responseSerializer = [AFHTTPResponseSerializer serializer];
NSString *URLStr = @"https://mail.itcast.cn";
[manager GET:URLStr parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
NSString *html = [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding];
NSLog(@"%@",html);
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"出错 %@",error);
}];
}