1.依赖
<!-- lookup parent from repository -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.1.6.RELEASE</version>
<relativePath/>
</parent>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.10</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-ws</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- json -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.8</version>
</dependency>
</dependencies>
<!-- 打包 -->
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<start-class>com.springboot.Application</start-class>
<java.version>1.7</java.version>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
2.启动类
package com.springboot;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan
@EnableAutoConfiguration
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class,args);
}
}
3.接口
package com.springboot.resource;
import org.springframework.stereotype.Component;
import org.springframework.web.bind.annotation.*;
import java.util.HashMap;
import java.util.Map;
@Component("com.springboot.resource.Admin")
@RequestMapping("/admin/**")
public class Admin {
/**
* curl -X POST -H "Content-Type: application/json" -i "http://localhost:8080/admin/query" -d '{"username":"wzy1", "password":"wzy111"}'
*/
@RequestMapping(value = "/query2",method = RequestMethod.POST,consumes = "application/json",produces="application/json")
@ResponseBody
public Object query(@RequestBody User user){
Map<String,Object> map = new HashMap<String,Object>();
map.put("timestamp",String.valueOf(System.currentTimeMillis()));
map.put("user",user);
return map;
}
}
3.实体类(lombok插件可以使用注解代替setter,getter)
package com.springboot.resource;
import lombok.Data;
@Data
public class User {
private String username;
private String password;
}
4.接口测试

5.打包(前提是maven环境配置好)
mvn install
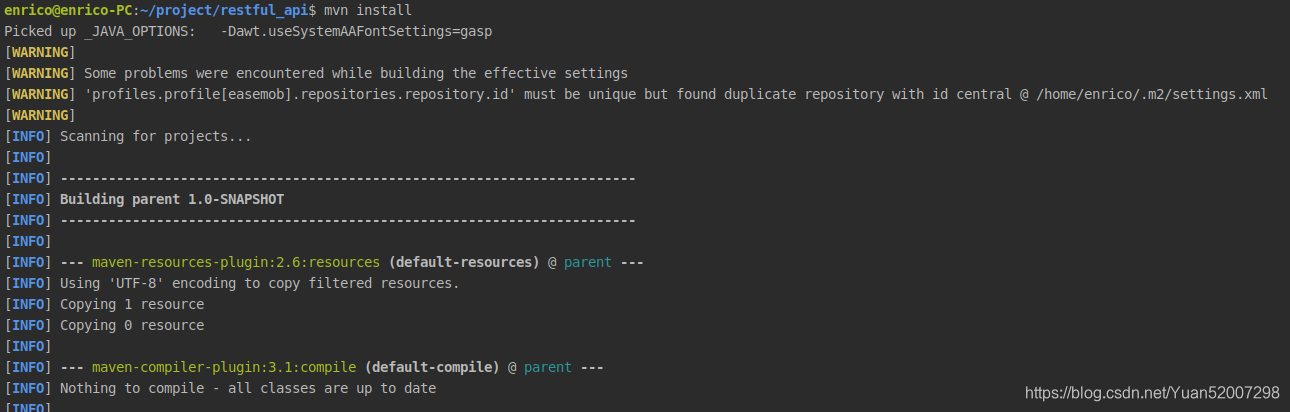
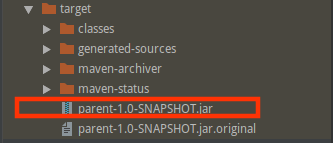
6.运行jar测试
java -jar parent-1.0-SNAPSHOT.jar
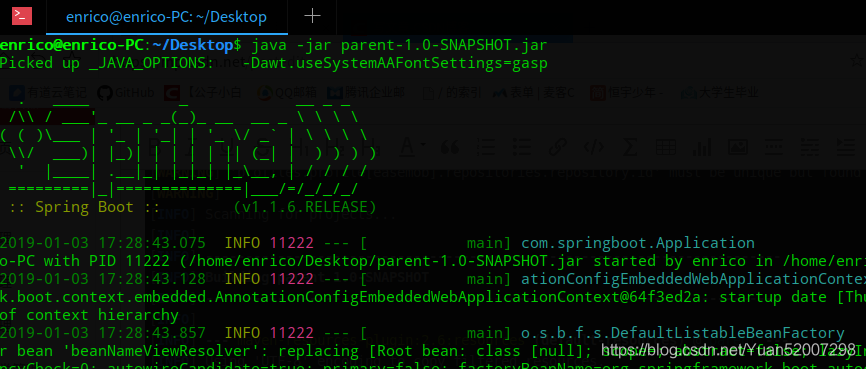
