组件:多图片上传(el-upload)
<template>
<div>
<el-upload
ref="elUpload"
:action="uploadPath"
:headers="headers"
list-type="picture-card"
:on-preview="handlePictureCardPreview"
:before-remove="beforeRemove"
:on-remove="handleRemove"
:on-success="handleUploadSuccess"
:on-exceed="handleUploadExceed"
:file-list="imageList"
accept=".jpeg,JPEG,.jpg,.JPG,.png,.PNG,gif,.GIF"
:before-upload="beforeAvatarUpload"
:limit="limit"
>
<i class="el-icon-plus" />
</el-upload>
<el-dialog :visible.sync="dialogVisible" top="2vh" append-to-body>
<img width="100%" :src="dialogImageUrl" alt="">
</el-dialog>
</div>
</template>
<script>
import { getToken } from '@/utils/auth'
export default {
props: {
value: {
default: '',
type: String
},
limit: {
type: Number,
default() {
return 3
}
}
},
data() {
return {
dialogImageUrl: '',
dialogVisible: false,
resourcesUrl: '',
uploadPath: process.env.VUE_APP_BASE_API + '/api/file/test/upload',
headers: {
'Authorization': getToken()
}
}
},
computed: {
imageList() {
const res = []
if (this.value) {
const imageArray = this.value.split(',')
for (let i = 0; i < imageArray.length; i++) {
res.push({ url: this.resourcesUrl + imageArray[i], response: imageArray[i] })
}
}
this.$emit('input', this.value)
return res
}
},
methods: {
handleUploadSuccess(response, file, fileList) {
console.log(file)
console.log(fileList)
const pics = fileList.map(file => {
if ((typeof file.response) === 'object') {
return file.response.fileUrl
} else {
return file.response
}
}).join(',')
console.log(pics)
this.$emit('input', pics)
},
handleUploadExceed(files, fileList) {
this.loading = false
this.$message.warning(`对不起,最多上传个` + fileList.length + `图片`)
},
beforeAvatarUpload(file) {
const isPic =
file.type === 'image/jpeg' ||
file.type === 'image/png' ||
file.type === 'image/gif' ||
file.type === 'image/jpg'
const isLt8M = file.size / 1024 / 1024 < 8
if (!isPic) {
this.$message.error('上传图片只能是 JPG、JPEG、PNG、GIF 格式!')
return false
}
if (!isLt8M) {
this.$message.error('上传图片大小不能超过 8MB!')
}
return isPic && isLt8M
},
beforeRemove(file, fileList) {
return this.$confirm(`确定移除该图片?`)
},
handleRemove(file, fileList) {
const pics = fileList.map(file => {
return file.response
}).join(',')
this.$emit('input', pics)
},
handlePictureCardPreview(file) {
this.dialogImageUrl = file.url
this.dialogVisible = true
}
}
}
</script>
<style lang="scss">
</style>
使用
- 在需要使用文件上传页面的.vue文件中引用
组件路径如下图:
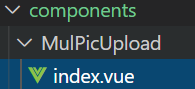
import mulPicUpload from '@/components/MulPicUpload'
- 注入
components: { mulPicUpload }
- 使用(不写limit时,默认文件限制数为3;文件大小限制为8M;多个文件时,picUrl的值为“英文逗号”隔开的字符串)
<mul-pic-upload v-model="form.picUrl" :limit="1"/>