今天C语言的复习告一段落,虽然不是特别完美,但还是要记录一下单链表的基本操作,上代码:
void head_insert(STU **head) {//需要改变指针的值,所以传**类型
//1.申请节点空间
STU *new_node = (STU*)malloc(sizeof(STU));
if (new_node == NULL) {//申请失败判断
perror("malloc");
}
memset(new_node, 0, sizeof(STU));//清空结构体
//2.给节点赋值
scanf("%d %s %f",&new_node->num,new_node->name,&new_node->score);
//3.对头(节点)进行判断
STU *pf=*head;
*head = new_node;//指针赋值
pf == NULL?(new_node->next = NULL):(new_node->next = pf);
}
void tail_insert(STU **head) {
STU *new_node = (STU*)malloc(sizeof(STU));
if (new_node == NULL) {
perror("malloc");
}
memset(new_node, 0, sizeof(STU));
scanf("%d %s %f", &new_node->num, new_node->name, &new_node->score);
STU *pf = *head;
if(pf==NULL)
*head = new_node;
else {
while (pf->next != NULL) {//因为要给尾节点的next赋值,所以要用上一个节点来判断
pf = pf->next;
}
pf->next= new_node;
}
}
void sort_insert(STU **head) {
STU *new_node = (STU*)calloc(1,sizeof(STU)),*temp=NULL;
if (new_node == NULL) {
perror("calloc");
}
scanf("%d %s %f", &new_node->num, new_node->name, &new_node->score);
STU *pf = *head;
if (pf == NULL)
*head = new_node;
else {
while (pf != NULL) {
//按成绩的多少插入,大的在前
if (pf->score >= new_node->score ) {
temp = pf;//定义上一个节点的指针
pf = pf->next;
}
else {
break;
}
}
if (pf == *head ) {//在头部插入
*head = new_node;
new_node->next = pf;
}
else if (pf !=NULL) {//在中间插入
new_node->next = temp->next;
temp->next = new_node;
}
else {//在尾部插入
temp->next = new_node;
}
}
}
void search_link(STU **head) {
int temp = 0;
char temp1[32] = { 0 };
printf("请输入你想查询学生的姓名:");
scanf("%s", temp1);
STU* pf = *head;
if (pf == NULL) {
printf("link not find!\n");
}
else {
while (pf != NULL) {
if (strcmp(pf->name, temp1) == 0){
printf("学号=%d 姓名=%s 成绩=%.1f\n", pf->num, pf->name, pf->score);
temp = 1;
}
pf = pf->next;
}
if (temp == 0) {
printf("没有找到姓名为%s相关的节点信息\n", temp1);
}
}
}
void delete_link(STU **head) {
int temp1=0,temp=0;
printf("请输入你想删除学生的学号:");
scanf("%d", &temp1);
STU* pf = *head, *temp2=NULL, *temp3 = NULL;
if (pf == NULL) {
printf("link not find!\n");
}
else {
while (pf != NULL) {
if (pf->num == temp1) {
if(pf==*head){
*head = pf->next;
temp2 = pf;
}
else if (pf ->next!= NULL) {
temp2 = pf;
temp3->next = pf->next;
}
else {
temp2 = pf;
temp3->next = NULL;
temp = 1;
break;
}
temp = 1;
}
temp3 = pf;
pf = pf->next;
}
if (temp == 0)printf("没有找到学号%d相关的节点信息\n", temp1);
else printf("删除学号%d相关的节点信息成功!!!\n",temp1);
if (temp2 != NULL) {
free(temp2);
temp2 = NULL;
}
}
}
void free_link(STU **head) {
STU* pf = *head;
if (pf == NULL) {
printf("link not find!\n");
}
else {
while (pf != NULL) {
*head = pf;
pf = pf->next;
if (*head != NULL) {
free(*head);
*head = NULL;
}//释放时必须传入的头结点操作
}
printf("link释放成功\n");
}
}
void print_link(STU **head) {
STU *pf = *head;
if (pf == NULL) {
printf("link no find!\n");
}
while(pf != NULL){
printf("学号=%d 姓名=%s 成绩=%.1f\n", pf->num, pf->name, pf->score);
pf = pf->next;
}
}
运行结果:
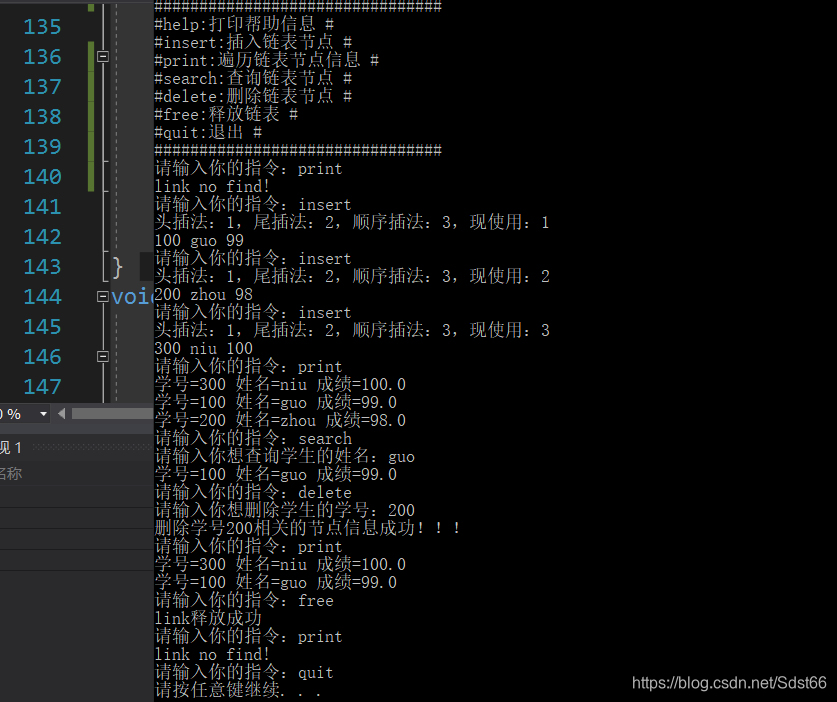