题目
输入两个链表,找出它们的第一个公共节点。
分析
代码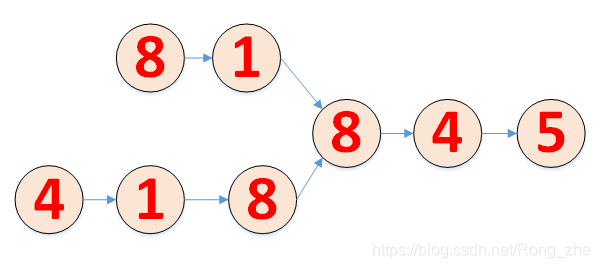
class ListNode{
public int data;
public ListNode next;
public ListNode(int data) {
this.data = data;
}
@Override
public String toString() {
return "ListNode{" +
"data=" + data +
'}';
}
}
import java.util.Stack;
public class Solution {
public static void main(String[] args) {
//链表1
ListNode head = new ListNode(8);
ListNode node1 = new ListNode(1);
ListNode node2 = new ListNode(8);
ListNode node3 = new ListNode(4);
ListNode node4 = new ListNode(5);
head.next = node1;
node1.next = node2;
node2.next = node3;
node3.next = node4;
//链表2
ListNode head2 = new ListNode(4);
ListNode node6 = new ListNode(1);
ListNode node7 = new ListNode(8);
head2.next = node6;
node6.next = node7;
node7.next = node2;
System.out.println(getIntersectionNode(head,head2));
}
/**
* 寻找两个链表的第一个公共节点
* @param headA 链表
* @param headB 链表
* @return 第一个公共节点
*/
public static ListNode getIntersectionNode(ListNode headA, ListNode headB) {
Stack<ListNode> stackA = ListNode2Stack(headA);
Stack<ListNode> stackB = ListNode2Stack(headB);
ListNode temp = null;
while (!stackA.isEmpty() && !stackB.isEmpty()){
if(stackA.peek() == stackB.peek()) {
stackA.pop();
temp = stackB.pop();
}
else {
break;
}
}
return temp;
}
/**
* 遍历单向链表 将每个节点压入栈中
* @param head
*/
public static Stack<ListNode> ListNode2Stack(ListNode head){
Stack<ListNode> stack = new Stack<ListNode>();//创建栈
while (head != null){
stack.add(head);//将每个节点添加到栈中
head = head.next;
}
return stack;
}
}
分析
代码
public class Solution {
public static void main(String[] args) {
//链表1
ListNode head = new ListNode(8);
ListNode node1 = new ListNode(1);
ListNode node2 = new ListNode(8);
ListNode node3 = new ListNode(4);
ListNode node4 = new ListNode(5);
head.next = node1;
node1.next = node2;
node2.next = node3;
node3.next = node4;
//链表2
ListNode head2 = new ListNode(4);
ListNode node6 = new ListNode(1);
ListNode node7 = new ListNode(8);
head2.next = node6;
node6.next = node7;
node7.next = node2;
System.out.println(getIntersectionNode(head,head2));
}
/**
* 寻找两个链表的第一个公共节点
* @param headA 链表
* @param headB 链表
* @return 第一个公共节点
*/
public static ListNode getIntersectionNode(ListNode headA, ListNode headB) {
int lenA = getListLength(headA);//链表长度
int lenB = getListLength(headB);
//链表1长度大于链表2
if (lenA > lenB){
//先将链表1向前走 lenA-lenB
for (int i = 0; i < lenA-lenB; i++){
headA = headA.next;
}
}else {
for (int i = 0; i < lenB-lenA; i++){
headB = headB.next;
}
}
for (int i = 0; i < Math.min(lenA,lenB); i++){
if (headA == headB){
break;
}
headA = headA.next;
headB = headB.next;
}
return headA;
}
/**
* 获取链表长度
* @param head 头节点
* @return 链表长度
*/
public static int getListLength(ListNode head){
int count = 0;
while (head != null){
count++;
head = head.next;
}
return count;
}
分析
代码
public class Solution {
public static void main(String[] args) {
//链表1
ListNode head = new ListNode(8);
ListNode node1 = new ListNode(1);
ListNode node2 = new ListNode(8);
ListNode node3 = new ListNode(4);
ListNode node4 = new ListNode(5);
head.next = node1;
node1.next = node2;
node2.next = node3;
node3.next = node4;
//链表2
ListNode head2 = new ListNode(4);
ListNode node6 = new ListNode(1);
ListNode node7 = new ListNode(8);
head2.next = node6;
node6.next = node7;
node7.next = node2;
System.out.println(getIntersectionNode(head,head2));
}
/**
* 寻找两个链表的第一个公共节点
* @param headA 链表
* @param headB 链表
* @return 第一个公共节点
*/
public static ListNode getIntersectionNode(ListNode headA, ListNode headB) {
ListNode node1 = headA;
ListNode node2 = headB;
//同时遍历两个链表
while (node1 != node2){
node1 = node1 == null? headB : node1.next;
node2 = node2 == null? headA : node2.next;
}
return node1;
}
}