activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<!--layout_weight: 每个控件占控比例, 设置它后layout_width影响变小-->
<!-- 线性布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context=".MainActivity">
<EditText
android:id="@+id/edit_text"
android:text="消息"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/button_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
-->
<!--相对布局 RelativeLayout -->
<!--layout_alignParentLeft 靠布局的左边显示 -->
<!--layout_alignParentTop 靠布局的顶部显示 -->
<!--layout_centerInParent 靠布局的中间显示 -->
<!--layout_toRightOf 在某个控件右边显示 -->
<!--layout_above 在某个控件的上面显示 -->
<!--
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context=".MainActivity">
<Button
android:id="@+id/button_1"
android:text="button1"
android:layout_above="@+id/button_5"
android:layout_toRightOf="@+id/button_5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/button_2"
android:text="button2"
android:layout_above="@+id/button_5"
android:layout_toLeftOf="@id/button_5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/button_5"
android:text="button5"
android:layout_centerInParent="true"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</RelativeLayout>
-->
<!--约束布局 ConstraintLayout -->
<!--layout_alignParentLeft 靠布局的左边显示 -->
<!--layout_constraintBottom_toBottomOf="parent" 本控件的底部位置和parent父控件的底部位置一致,且不能超过 -->
<!--layout_constraintStart_toStartOf="parent" 本控件的底部位置和parent父控件的左边位置一致,且不能超过 -->
<!--layout_constraintHorizontal_bias="0.0" 本控件的水平偏移量,百分比 -->
<!--layout_constraintVertical_bias="0.436" 本控件的垂直便宜量,百分比 -->
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context=".MainActivity">
<include layout="@layout/title"/>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.464"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.486" />
<TextView
android:id="@+id/text_view1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="我是textview"
app:layout_constraintBottom_toTopOf="@+id/button"
app:layout_constraintEnd_toEndOf="@+id/button"
app:layout_constraintHorizontal_bias="0.949"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.92" />
</androidx.constraintlayout.widget.ConstraintLayout>
title.xml
<?xml version="1.0" encoding="utf-8"?>
<!--在res\values\themes.xml设置风格: parent="Theme.MaterialComponents.DayNight.DarkActionBar.Bridge-->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:background="@drawable/title_img">
<Button
android:id="@+id/back_button"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_margin="5dp"
android:background="@drawable/back_img"
android:gravity="center"
android:text="Back"
android:textColor="#fff" />
<TextView
android:id="@+id/text_view1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:gravity="center"
android:layout_weight="1"
android:text="Title text"
android:textColor="#fff"
android:textSize="24sp"/>
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:gravity="center"
android:background="@drawable/edit_img"
android:layout_margin="5dp"
android:text="Back"
android:textColor="#fff"
/>
</LinearLayout>
MainActivity.java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActionBar actionBar = getSupportActionBar();
if(actionBar != null){
actionBar.hide(); //隐藏原有的标题栏-title
}
}
}
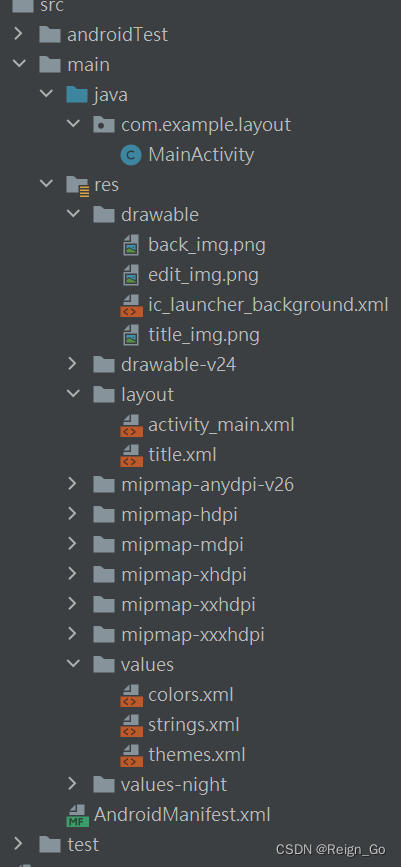