1. 比mock等测试框架更加强大:可以模拟私有类,静态类等..
2. 遇到异常信息可以抓取:
3. powerMock的用法: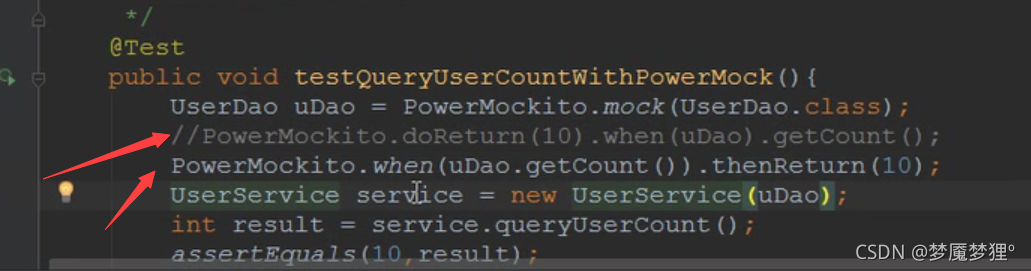
由于powermock不支持注解形式,这时候用mock反而更好
4. 执行无返回值的方法时,可以让其什么都不做:
5. 判断是否被调用: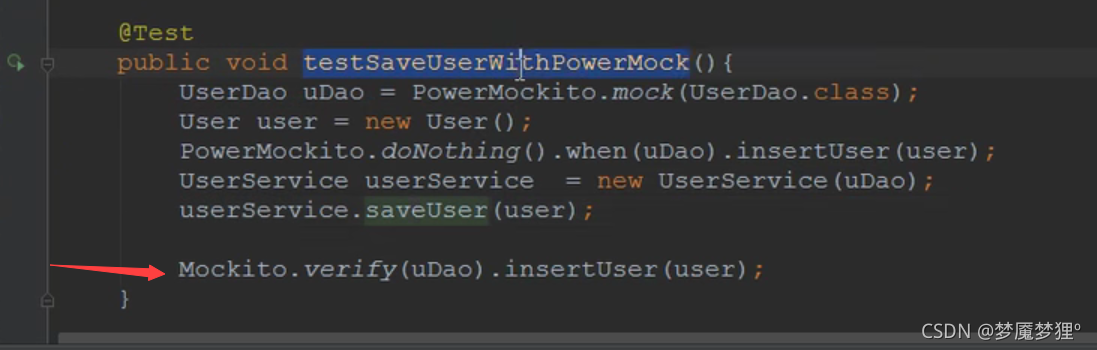
6. 当new无参构造时返回mock的对象:
7.mock静态类:
8. mock抛异常:
@Test
public void testDelUser() throws Exception {
int toDelete = 1;
PowerMockito.when(userService.delUser(toDelete)).thenThrow(new Exception("mock exception"));
boolean result = uc.delUser(toDelete);
Assert.assertEquals(result, false);
}
9. mock静态方法
@Test
public void mockFileHelper() {
PowerMockito.mockStatic(FileHelper.class);
PowerMockito.when(FileHelper.getName("lucy")).thenReturn("lily");
Assert.assertEquals(FileHelper.getName("lucy"), "lily");
}
注意:需要在prepare...注解中注入对应类
10. mock 返回值为 void 的方法
@Test
public void testSaveUser() throws Exception {
UserDto userDto = new UserDto();
// way one:
PowerMockito.doNothing().when(userService, "saveUser", userDto);
// way two:
PowerMockito.doNothing().when(userService).saveUser(userDto);
uc.saveUser(userDto);
}
11. mock含有Autowried注解的方法(存在bug)
需要加:
MockitoAnnotations.initMocks(this);
因为注解注入需要spring容器启动后才会生效,所以需要特殊操作,并且一般不推荐注解注入的方式
12. 模拟静态方法:
@Before
public void before(){
PowerMockito.mockStatic(ApplicationContextHelper.class);
PowerMockito.mockStatic(ApplicationContext.class);
//模拟方法
ApplicationContext applicationContext = PowerMockito.mock(ApplicationContext.class);
Mockito.when(ApplicationContextHelper.getContext()).thenReturn(applicationContext);
Mockito.when(applicationContext.getBean(DjiContractService.class)).thenReturn(djiContractService);
}
13.模拟返回体报文
ContractVO contractVO = new ContractVO();
ResponseEntity<String> responseEntity = new ResponseEntity(JSON.toJSON(contractVO),HttpStatus.OK);
when(ocBaseFeignClient.queryByUuid(any(),any())).thenReturn(responseEntity);
这句很重要:
ResponseUtils.setObjectMapper(new ObjectMapper());
14. mock私有方法(直接不执行私有方法)
PowerMockito.doReturn("test").when(mockPrivateClass,"privateFunc");
//如果是带参数的方法,将参数加在后面
PowerMockito.doReturn("test").when(mockPrivateClass,"privateFunc","paramA",,"paramB");
具体方法:
@RunWith(PowerMockRunner.class)
@PrepareForTest({MockPrivateClass.class})
public class MockPrivateClassTest {
private MockPrivateClass mockPrivateClass;
@Test
public void mockPrivateFunc() throws Exception {
mockPrivateClass = PowerMockito.spy(new MockPrivateClass());
PowerMockito.doReturn("test").when(mockPrivateClass,"privateFunc");
String realResult = mockPrivateClass.mockPrivateFunc();
Assert.assertEquals("test", realResult);
PowerMockito.verifyPrivate(mockPrivateClass, Mockito.times(1)).invoke("privateFunc");
}
}
15. 本类方法调用本类方法,不执行方法内部,直接mock
@InjectMocks
private Demo demo;
public void test(){
Demo demo1 = Mockito.spy(demo);
Mockito.doReturn(返回值).when(demo1).methodB(Mockito.any());
demo1.mainMethod();
}
...参照收藏