https://open.dingtalk.com/document/robots/custom-robot-access
添加Webhook机器人,我选择的安全方式是关键词==“消息”==
使用Newtonsoft
Imports System.Net.Http
Imports System.Text
Imports Newtonsoft.Json
Public Class Form1
Async Function SendMarkdownMessage(ByVal webhookUrl As String, ByVal title As String, ByVal markdownContent As String) As Task
Using client As New HttpClient()
Dim payload = New With {
.msgtype = "markdown",
.markdown = New With {
.title = title,
.text = markdownContent
}
}
Dim jsonPayload As String = JsonConvert.SerializeObject(payload)
Dim content As New StringContent(jsonPayload, Encoding.UTF8, "application/json")
Dim response As HttpResponseMessage = Await client.PostAsync(webhookUrl, content)
If response.IsSuccessStatusCode Then
Console.WriteLine("Markdown message sent successfully!")
Else
Console.WriteLine("Failed to send Markdown message. Status code: " & response.StatusCode)
End If
End Using
End Function
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim webhookUrl As String = "https://oapi.dingtalk.com/robot/send?access_token=你自己的token"
Dim markdownContent As String = "# 消息Hello, Markdown!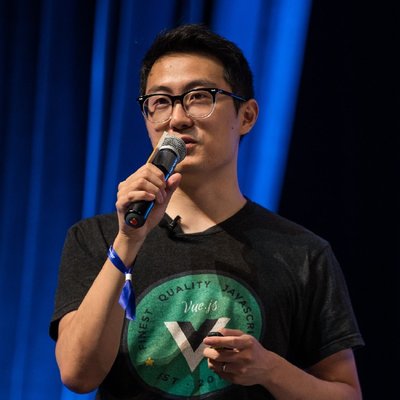"
Dim title As String = "Markdown Message"
SendMarkdownMessage(webhookUrl, title, markdownContent).Wait()
End Sub
End Class
最简单的机器人,效果如下,但是不能发送本地文件,需要换个方式
不用Newtonsoft
```vbnet
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
Dim webhookUrl As String = "https://oapi.dingtalk.com/robot/send?access_token=96eb8d5ef7a660d2f360f81d8d79143dfa4f70a895bc383cc4e4e4a311f65dfc"
Dim message As String = "# 消息Without NewtonSoft!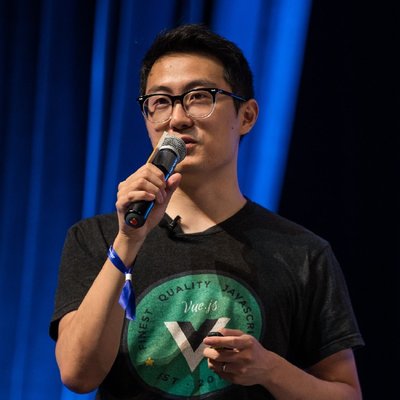"
Dim postData As String = "{""msgtype"": ""markdown"", ""markdown"": {""title"": ""DingTalk Markdown Message"", ""text"": """ & message & """}}"
Dim request As WebRequest = WebRequest.Create(webhookUrl)
request.Method = "POST"
request.ContentType = "application/json"
Dim data As Byte() = Encoding.UTF8.GetBytes(postData)
request.ContentLength = data.Length
Using stream As Stream = request.GetRequestStream()
stream.Write(data, 0, data.Length)
End Using
Dim response As WebResponse = request.GetResponse()
Using streamReader As New StreamReader(response.GetResponseStream())
Dim result As String = streamReader.ReadToEnd()
Debug.Print(result)
End Using
Console.ReadLine()
End Sub
改用加签发方式
Imports System.Net.Http
Imports System.Security.Cryptography
Imports System.Text
Public Class Form1
Async Function SendMarkdownMessage(webhookUrl As String, accessToken As String, secret As String, title As String, markdownContent As String) As Task
Dim timestamp As Long = DateTimeOffset.Now.ToUnixTimeMilliseconds()
Dim sign As String = ComputeSignature(secret, timestamp)
Dim url As String = $"{webhookUrl}?access_token={accessToken}×tamp={timestamp}&sign={sign}"
Using client As New HttpClient()
Dim payload = New With {
.msgtype = "markdown",
.markdown = New With {
.title = title,
.text = markdownContent
}
}
Dim jsonPayload As String = Newtonsoft.Json.JsonConvert.SerializeObject(payload)
Dim content As New StringContent(jsonPayload, Encoding.UTF8, "application/json")
Dim response As HttpResponseMessage = Await client.PostAsync(url, content)
If response.IsSuccessStatusCode Then
Console.WriteLine("Markdown message sent successfully!")
Else
Console.WriteLine("Failed to send Markdown message. Status code: " & response.StatusCode)
End If
End Using
End Function
Function ComputeSignature(secret As String, timestamp As Long) As String
Dim stringToSign As String = $"{timestamp}{vbLf}{secret}"
Dim secretBytes As Byte() = Encoding.UTF8.GetBytes(secret)
Dim stringToSignBytes As Byte() = Encoding.UTF8.GetBytes(stringToSign)
Using hmac As New HMACSHA256(secretBytes)
Dim hashBytes As Byte() = hmac.ComputeHash(stringToSignBytes)
Return Convert.ToBase64String(hashBytes)
End Using
End Function
Private Async Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim webhookUrl As String = "https://oapi.dingtalk.com/robot/send"
Dim accessToken As String = "你的token"
Dim secret As String = "你设置加签后的密钥"
Dim markdownContent As String = "# VB.NET11dian使用加签无拘无束!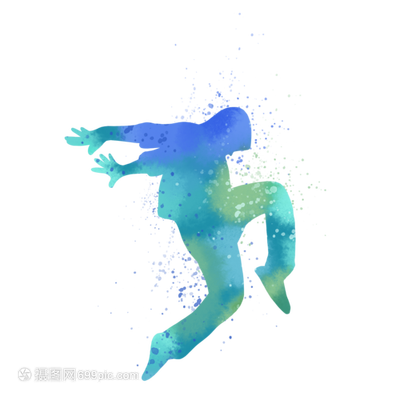"
Dim title As String = "Markdown Message"
Await SendMarkdownMessage(webhookUrl, accessToken, secret, title, markdownContent)
End Sub
End Class