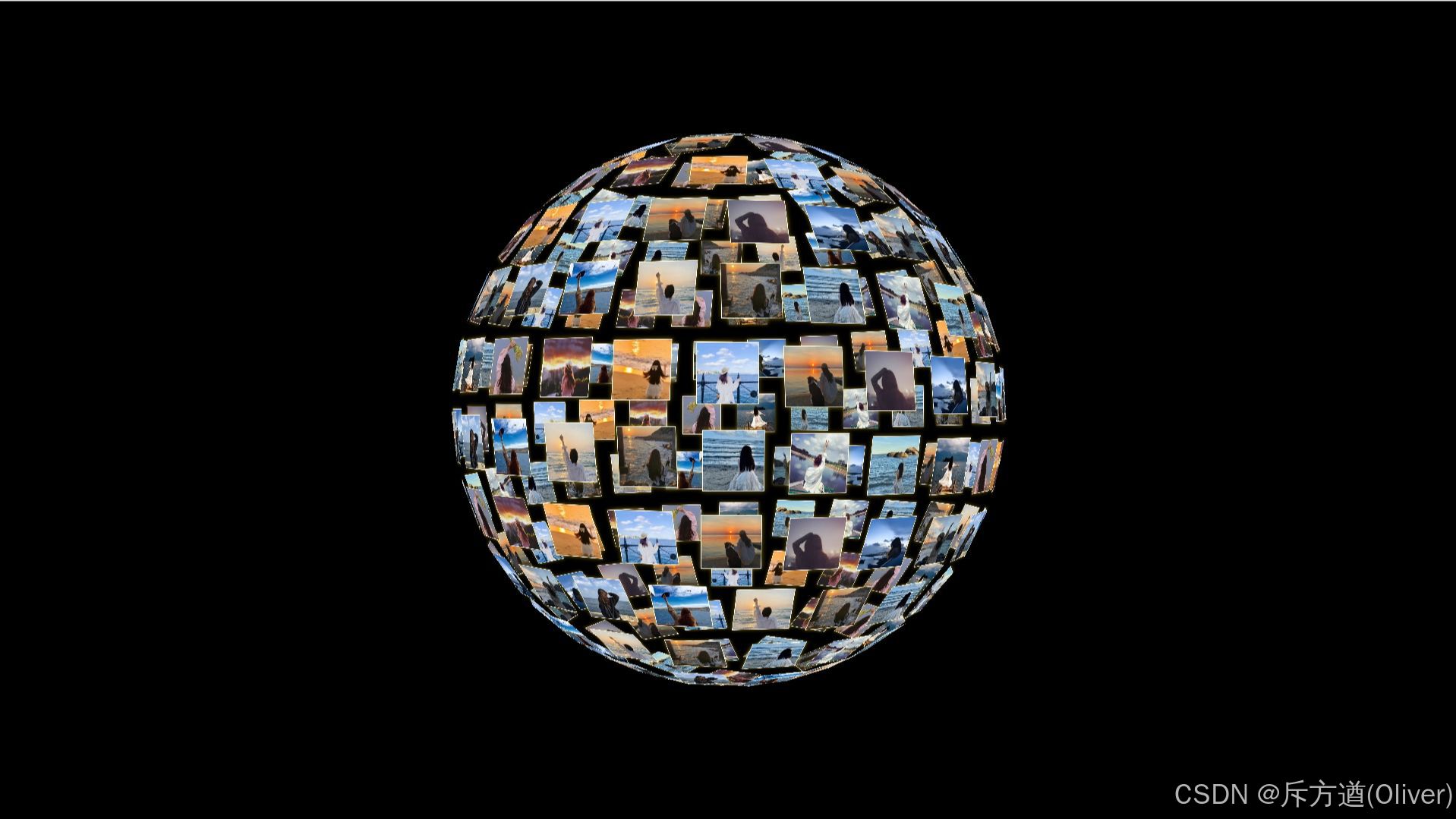
<div
ref="threeContainer"
style="width: 100%; height: 100%; display: flex; justify-content: center; align-items: flex-start"
></div>
// 头像
var circleHead = new THREE.CircleGeometry(40, 62);
var meshStandard = new THREE.Mesh(circleHead);
var newGeometry = meshStandard.geometry.clone();
var newMaterial = meshStandard.material.clone();
for (var i = 0; i < this.table.length; i++) {
// 创建圆形头像
var mesh = new THREE.Mesh(newGeometry, newMaterial);
mesh.position.x = Math.random() * 4000 - 2000;
mesh.position.y = Math.random() * 4000 - 2000;
mesh.position.z = Math.random() * 4000 - 2000;
mesh.name = 'Avatar';
let url = this.getRandomAvatar();
const texture = new THREE.TextureLoader().load(url);
var material = new THREE.MeshBasicMaterial({
map: texture,
});
material.side = THREE.DoubleSide; //单面贴就删除
mesh.material = material;
that.objects.push(mesh);
var _object2 = new THREE.Object3D();
_object2.position.z = -30000;
this.targets.table.push(_object2);
// 背面
if(this.animationType == 2 || this.animationType == 3){
var newGeometry = mesh.geometry.clone();
var newMaterial = mesh.material.clone();
var mesh2 = new THREE.Mesh(newGeometry, newMaterial);
mesh2.position.z = -2;
that.objects[i].add(mesh2);
}
}
// 头像框
var circleHead2 = new THREE.CircleGeometry(50, 32);
var meshStandard2 = new THREE.Mesh(circleHead2);
let options = {
transparent: true, //允许透明
};
if (this.avatarBorderDecoration) {
let url = this.avatarBorderDecoration ;
const texture = new THREE.TextureLoader().load(url);
options.map = texture;
} else {
options.color = this.themeColor;
}
var material = new THREE.MeshBasicMaterial(options);
material.side = THREE.DoubleSide; //单面贴就删除
meshStandard2.material = material;
var newGeometry = meshStandard2.geometry.clone();
var newMaterial = meshStandard2.material.clone();
for (var i = 0; i < this.table.length; i++) {
// 创建圆形头像
var mesh = new THREE.Mesh(newGeometry, newMaterial);
mesh.position.z = -1;
that.objects[i].add(mesh);
}
for (let index = 0; index < that.objects.length; index++) {
that.parent.add(that.objects[index]); //加入到场景
}
// 球 sphere
var vector = new THREE.Vector3();
for (var i = 0, l = this.objects.length; i < l; i++) {
var phi, theta;
if (i == 0) {
phi = Math.acos(-1 + (2 * i) / l) * 1.025;
theta = Math.sqrt(l * Math.PI) * phi * 1.01;
} else {
phi = Math.acos(-1 + (2 * i) / l);
theta = Math.sqrt(l * Math.PI) * phi;
}
var _object4 = new THREE.Object3D();
_object4.position.x = 750 * Math.cos(theta) * Math.sin(phi);
_object4.position.y = 750 * Math.sin(theta) * Math.sin(phi);
_object4.position.z = 750 * Math.cos(phi);
vector.copy(_object4.position).multiplyScalar(2);
_object4.lookAt(vector);
// this.renderer.render(that.scene, that.camera);
this.targets.sphere.push(_object4);
}
function play() {
// 放大进入动画
// that.scene.scale.set(.1,.1,.1)
var enterBlowUp = new TWEEN.Tween({
z: 0.01,
})
.to(
{
z: 3,
},
2e3,
)
.onUpdate(function (t) {
that.parent.scale.set(t, t, t);
});
// 停止缩小动画
var stopShrink = new TWEEN.Tween(that.parent.scale)
.to(
{
x: 0.01,
y: 0.01,
z: 0.01,
},
0.6e3,
)
.onComplete(function (t) {
destroyed();
});
// 放大动画
var runBlowUp = new TWEEN.Tween(that.parent.scale)
.to(
{
x: 1.8,
y: 1.8,
z: 1.8,
},
2e3,
)
.delay(3e3);
// 缩小动画
var runShrink = new TWEEN.Tween(that.parent.scale)
.to(
{
x: 1,
y: 1,
z: 1,
},
2e3,
)
.delay(3e3);
// 循环旋转动画
var rotateLoop = new TWEEN.Tween(that.parent.rotation)
.to(
{
y: 2 * Math.PI,
},
12e3,
)
.repeat(1 / 0)
.onComplete(function () {
that.parent.rotation.y = 0;
})
.onUpdate(function () {
that.lotteryPlayState == 'stop' &&
(runShrink.stop(), runBlowUp.stop(), rotateLoop.stop(), stopShrink.start());
});
rotateLoop.start();
runShrink.chain(runBlowUp);
runBlowUp.chain(runShrink);
// enterBlowUp.onComplete(function(e){
runBlowUp.start();
// });
// enterBlowUp.start();
that.lotteryTweenArr.push(enterBlowUp);
that.lotteryTweenArr.push(stopShrink);
that.lotteryTweenArr.push(rotateLoop);
that.lotteryTweenArr.push(runBlowUp);
that.lotteryTweenArr.push(runShrink);
}
function stop() {
that.lotteryPlayState = 'stop';
}
return {
play: play,
stop: stop,
playing: false,
};