流的
基本
概念
- 输入流:程序从外部介质(键盘、磁盘、网络)上获得数据时,创建的输入流对象。
- 输出流:程序内存中的数据输出到外部介质(控制台显示器、磁盘、网络)时,创建的输出流对象。
Java 语言是通过 java.io 包中的类和接口提供输入输出与文件操作的。在整个 Java.io 包
中最重要的就是 5 个类和 1 个接口。5 个类指的是 File、InputStream、OutputStream、Reader、
Writer,1 个接口指的是 Serializable。
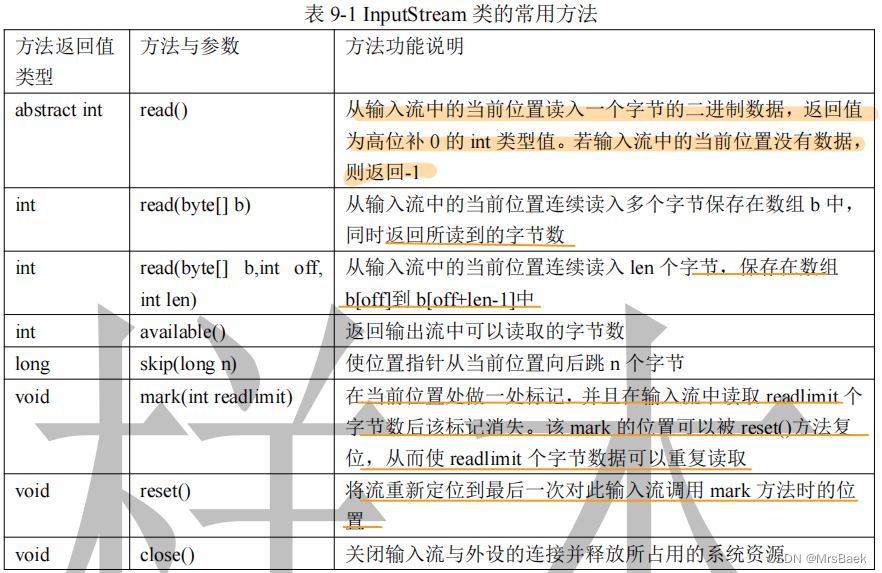
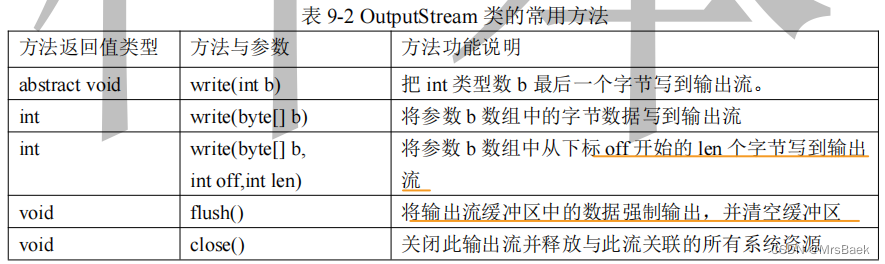
文件输入流
FileInputStream
类
| |
1)public FileInputStream(String filename) throws FileNotFoundException
|
根据磁盘文件的文件名创建 FileInputStream 类型的文件输入流对象,通过该对
象的 read()方法,以字节为单位读取文件中的数据。filename 是磁盘文件完整名称,包括盘
符、路径和文件名。
|
2)public FileInputStream(File file) throws FileNotFoundException
|
该构造方法参数中用到了 File 类。File 类ᨀ 供了对文件操作与获得文件基本信息的
方法,通过这些方法,可以得到文件的存储路径、文件名、大小、日期、文件长度、读写属
性等。File 类的构造方法如下:
public File(String filename)
参数 filename 是一个包括路径与文件名的完整的字符串。
public File(String pathname, String filename)
在这个构造方法中,将路径名与文件名字符串作为两个参数
|
文件输出流
FileOutputStream
类
| |
1)public FileOutputStream(String filename) throws FileNotFoundException
|
根据磁盘文件的文件名创建 FileInputStream 类的文件输出流对象。filename 是磁盘文
件名称,包括盘符、路径和文件名。
|
2)public FileOutputStream(File file) throws FileNotFoundException
| 通过文件对象 file 创建 FileOutputStream 类型的文件输出流对象。 |
3)public FileOutputStream(String filename, boolean append) throws
FileNotFoundException
|
通过文件名 filename 创建 FileOutputStream 类型的文件输出流对象,根据 append 参数
确定是覆盖原内容还是添加到原内容尾部。
|
4)public FileOutputStream(File file,boolean append) throws FileNotFoundException |
通过文件对象 file 创建 FileOutputStream 类型的文件输出流对象,根据 append 参数确
定是覆盖原内容还是添加到原内容尾部。
|
import java.io.*;public class Example13 {public static void main(String[] args) throws IOException{char c='A';boolean b=false;int i=3721;long x= 18912342033L;float f=3.14f;double d=3.1415926535;String str="hello world";DataOutputStream output;output=new DataOutputStream(new FileOutputStream(".\\datastream.dat"));output.writeChar(c);output.writeBoolean(b);output.writeInt(i);output.writeLong(x);output.writeFloat(f);output.writeDouble(d);output.writeUTF(str);output.close();DataInputStream input=new DataInputStream(new FileInputStream(".\\datastream.dat"));char cc=input.readChar();boolean bb=input.readBoolean();int ii=input.readInt();long xx=input.readLong();float ff=input.readFloat();double dd=input.readDouble();String sstr=input.readUTF();input.close();System.out.println(cc+"\n"+bb+"\n"+ii+"\n"+xx+"\n"+ff+"\n"+dd+"\n"+sstr);}}
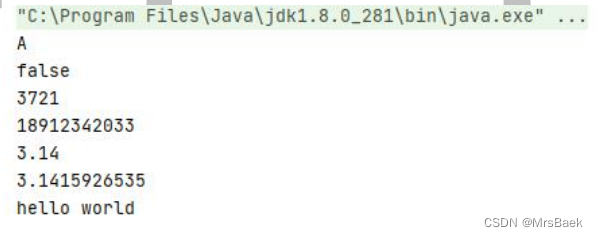
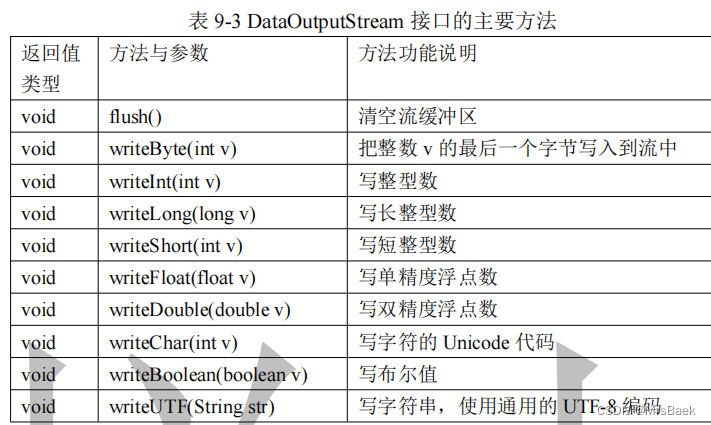
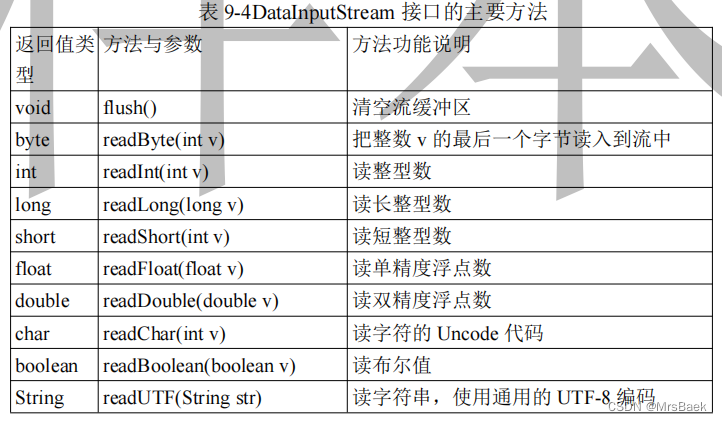
FileWriter 类(必考)
import java.io.FileWriter;import java.io.IOException;public class Example16 {public static void main(String[] args) throws IOException {FileWriter fw;fw=new FileWriter(".\\filestream.txt"); //打开文件输出流char array[]={'文','本','输','入','输','出','实','例','。'};for(int i=0;i<array.length ;i++)fw.write(array[i]); //写数据到文件输出流,也就是写入文件fw.write('\n');fw.write(array); //字符数组可以整体写出fw.write('\n');fw.write("你好,欢迎使用 Java 编程");fw.close(); //关闭文件输出流,即关闭文件}}
import java.io.FileReader;import java.io.IOException;public class Example17{public static void main(String[] args)throws IOException {FileReader fr;fr=new FileReader(".\\filestream.txt"); //打开文件输入流int value;while((value=fr.read())!=-1) //从文件输入流读数据System.out.print((char)value);fr.close(); //关闭文件输入流,即关闭文件}}
PrintWriter
输出字符到文件
import java.io.*;public class Example18{public static void main(String[] args) throws Exception {File file = new File(".\\scores.txt");if (file.exists()) {System.out.println("文件已经存在");System.exit(0);}PrintWriter output = new PrintWriter(file);// 把数据以文本字符格式写入文件output.print("数学成绩 ");output.println(90);output.print("物理成绩 ");output.println(85);output.close();}}
Scanner
import java.io.*;import java.util.Scanner;public class Example19 {public static void main(String args[]) throws FileNotFoundException {File file = new File(".\\scores.txt");String course;int grade;if (!file.exists()) {System.out.println("文件不存在");System.exit(0);}Scanner input=new Scanner(file);while (input.hasNext()) {course = input.next();grade = input.nextInt();System.out.println("Course: " + course + " grade " + grade);}input.close();}}
文件的综合应用
import java.io.*;import java.util.Scanner;public class Example20{static int MAX_NUM=1000;//职工人数不超过 1000 人static Staff_Com[] st=new Staff_Com[MAX_NUM];;static int realNum;public Example20(){}public static void main(String arg[])throws Exception{Example20 stfs=new Example20();Scanner input=new Scanner(System.in);stfs.readStaff();//把存放在 salary.dat 工资数据读回int choice=0;while (true){System.out.println("1. 增加新员工");System.out.println("2. 按员工的姓名的升序显示员工信息");System.out.println("3. 显示员工总人数、工资总和");System.out.println("4. 把所有员工信息保存到 salary.dat");System.out.println("5. 修改某个员工的工资");System.out.println("6. 删除某个员工");System.out.println("0. 退出执行");System.out.println("请输入选项 0--8");choice=input.nextInt();switch (choice) {case 1:stfs.addStaff();break;case 2:stfs.nameSort();break;case 3://stfs.totalSalary()break;case 4:stfs.writeStaff();break;case 5://stfs.modifySalary()break;case 6://stfs.deleteStaff();break;case 0:break;}if (choice==0)break;}}void readStaff() throws Exception{FileInputStream fis = null;ObjectInputStream in;try {fis = new FileInputStream(".\\salary.dat");} catch (FileNotFoundException e) {System.out.println("salary 文件还没创建,没有员工薪酬数据\n\n");}if (fis!=null) {in = new ObjectInputStream(fis);realNum=(int) in.readInt();st=(Staff_Com[]) in.readObject(); //整个数组一次读回fis.close();}}void writeStaff() throws Exception{FileOutputStream fos = new FileOutputStream(".\\salary.dat");ObjectOutputStream out = new ObjectOutputStream(fos);out.writeInt(realNum);out.writeObject(st); //写整个数组fos.close();}void nameSort(){for (int i=0;i<realNum;i++)for (int j=0;j<realNum-i-1;j++)if (st[j].compareTo(st[j+1])>0){Staff_Com t;t=st[j];st[j]=st[j+1];st[j+1]=t;}for (int i=0;i<realNum;i++)System.out.println(st[i]);}void addStaff(){Scanner input=new Scanner(System.in);System.out.println("编号 姓名 基本工资");String code,name;double salary;code =input.next();name=input.next();salary=input.nextDouble();st[realNum]=new Staff_Com(code,name,salary);realNum++;}}class Staff_Com implements Serializable,Comparable<Staff_Com>{String id;String name;double salary;Staff_Com(String i, String n, double s){id=i;name=n;salary=s;}public String toString(){return "Staff_ID:" +id+"Name: "+name+"Salary: "+salary;}@Overridepublic int compareTo(Staff_Com t) {return (int)(this.salary-t.salary);}}
程序运行后,首先执行 stfs.readStaff()把存放在 salary.dat 工资数据读回,先读取文
件中的第一个数据,该数据是员工的个数 realNum,其后就是员工的信息,读取并存放在 st[]。
注意这里不管有多少员工信息,用一个 st=(Staff_Com[]) in.readObject()就可以全部读回。
、