web.xml配置信息:
<context-param>
<param-name>key</param-name>
<param-value>value</param-value>
</context-param>
<servtel>
<servtel-name>demo01</servtel-name>
<servtel-class>com.lanou3g.Demo01</servtel-class>
</servtel>
<init-param>
<param-name>username</param-name>
<param-value>zhangsan</param-value>
</init-param>
<servtel-mapping>
<servtel-name>demo01</servtel-name>
<url-pattern>/demo01</url-pattern>
</servtel-mapping>
<servtel>
<servtel-name>demo02</servtel-name>
<servtel-class>com.lanou3g.Demo02</servtel-class>
</servtel>
<servtel-mapping>
<servtel-name>demo02</servtel-name>
<url-pattern>/demo02</url-pattern>
</servtel-mapping>
Config对象获取Servlet配置信息:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletConfig config = this.getServletConfig();
String value = config.getInitParameter("username");
System.out.println(value);
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
小结:先获取config对象,再通过getInitParameter(username相当于键值对中的键)方法获取对应值。
域对象获取全局配置信息:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletContext application = this.getServletContext();
String value = application.getInitParameter("key");
System.out.println(value);
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
域对象获取服务器文件并读取:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletContext application = this.getServletContext();
String path = application.getRealPath("/WEB-INF/a.properties");
Properties properties = new Properties();
properties.load(new FileInputStream(path));
System.out.println(properties.getProperty("key"));
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
请求转发:
概论:通过浏览器对服务器请求一个Servlet,但该Servlet无法完成,需跳转到另一个Servlet的操作。
注意:此过程中浏览器只发起一次请求。
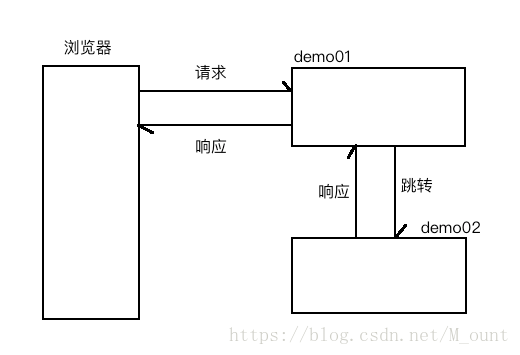
举例:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletContext application = this.getServletContext();
RequestDispatcher dispatcher = application.getRequestDispatcher("/demo02");
dispatcher.forward(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
public class Demo02 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
小结:先获取域对象application,再通过域对象的方法获取请求转发器dispatcher,最后通过dispatcher转发器的forward方法进行转发.
HttpServletResponse(响应对象):
组成:响应行:http/1.1
响应头:告诉浏览器我要做什么。
例如响应给你的文件需要下载,以什么编码格式解析数据
响应体:响应回浏览器的数据
注意:在同一个Servlet不能同时使用response中获取的字符流和字节流。
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setCharacterEncoding("UTF-8");
response.setHeader("Content-type", "text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
out.write("好开心");
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
文件下载:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String path = this.getServletContext().getRealPath("/WEB-INF/classes/团子大家族.png");
int index = path.lastIndexOf("/");
String filename = path.substring(index + 1);
filename = new String(filename.getBytes(), "iso-8859-1");
response.setHeader("content-disposition", "attachment;filename=" + filename);
response.setHeader("content-type", "image/png");
FileInputStream fis = new FileInputStream(path);
ServletOutputStream sos = response.getOutputStream();
int len = 0;
byte[] b = new byte[1024];
while ((len = fis.read(b)) != -1) {
sos.write(b, 0, len);
}
fis.close();
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
请求重定向:
概论:浏览器请求了一个Servlet,该Servlet给浏览器响应了一个带有重定向的访问地址的响应头,浏览器接到响应后,重新发起对访问地址的请求的操作。
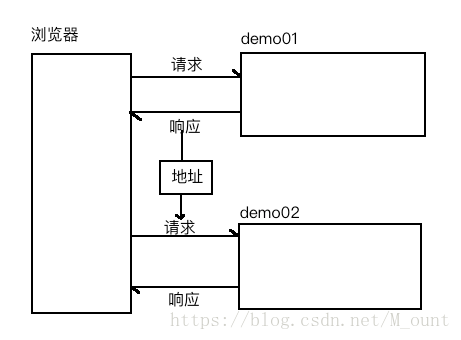
请求重定向和请求转发的区别:
请求重定向:发起两次请求,请求地址发生了变化。
请求转发:只是一次请求。
设置请求重定向的三种方法:
一、添加请求头的方法:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("我要借钱");
System.out.println("我没有,去找demo08");
response.setHeader("location", "/sh-web-servlet02/demo08");
response.setStatus(302);
System.out.println("我去了");
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
二、添加刷新头的方法:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setIntHeader("refresh", 1);
response.getWriter().write(Math.random() + "");
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
三、类似刷新的方法:
public class Demo01 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
response.setHeader("refresh", "3;url=/sh-web-servlet02/demo08");
response.getWriter().write("3秒后跳转");
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
HttpServletRequest(请求对象):
组成:请求行、请求头、请求体。
public class Demo08 extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println(request.getRequestURL());
System.out.println(request.getRequestURI());
System.out.println(request.getMethod());
System.out.println(request.getContextPath());
String username = request.getParameter("username");
String password = request.getParameter("password");
System.out.println(username + " " + password);
String header = request.getHeader("User-Agent");
System.out.println(header);
if (header.toLowerCase().contains("firefox")) {
System.out.println("用的是火狐");
} else if (header.toLowerCase().contains("chrome")) {
System.out.println("用的是谷歌");
} else {
System.out.println("其他浏览器");
}
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}