一、概述
需求如下:在一个多行文本最后添加一个可点击的文本。
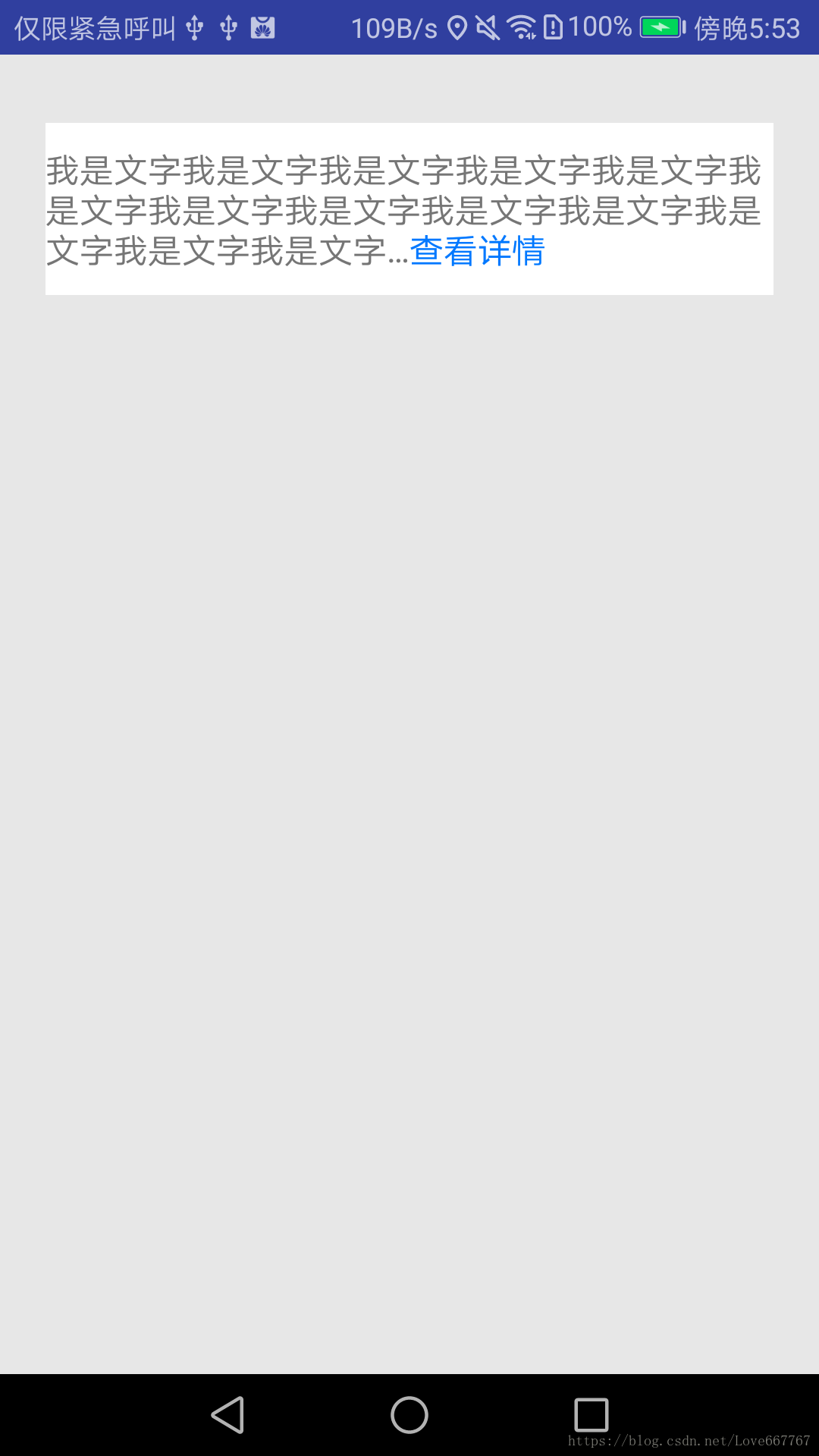
二、代码
布局文件
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#31999999">
<!-- 左右边距是20dp -->
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="30dp"
android:background="#ffffff"
android:paddingBottom="10dp"
android:paddingTop="10dp"
android:textSize="15sp" />
</RelativeLayout>
代码
public class MainActivity extends FragmentActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String content = "我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字我是文字";
String suffix = "查看详情";
TextView titleTv = ((TextView) findViewById(R.id.title));
renderDesc(titleTv, content, suffix);
}
private int dp2px(float dpVal) {
return (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, dpVal,
getResources().getDisplayMetrics());
}
private int sp2px(float dpVal) {
return (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, dpVal,
getResources().getDisplayMetrics());
}
private int getDeviceWidth() {
DisplayMetrics dm = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(dm);
return dm.widthPixels;
}
// 渲染文字
private void renderDesc(final TextView textView, final String content, @Nullable String suffix) {
if (TextUtils.isEmpty(content)) {
return;
}
int deviceWidth = getDeviceWidth();
int leftPadding = dp2px(20);//控件左右边距
int availLength = (int) (2.5 * (deviceWidth - leftPadding * 2));//2.5表示截取2.5行的文字长度
final TextPaint textPaint = textView.getPaint();
// 核心Api
String ellipsizeStr = (String) TextUtils.ellipsize(content, textPaint, availLength, TextUtils.TruncateAt.END);
boolean isSuffixEmpty = TextUtils.isEmpty(suffix);
String totalContent = isSuffixEmpty ? ellipsizeStr : (ellipsizeStr + suffix);
SpannableString spanString = new SpannableString(totalContent);
if (!isSuffixEmpty) {
spanString.setSpan(new ClickableSpan() {
@Override
public void onClick(View widget) {
Toast.makeText(MainActivity.this, "点击查看详情", Toast.LENGTH_SHORT).show();
}
@Override
public void updateDrawState(TextPaint ds) {
super.updateDrawState(ds);
ds.setColor(Color.parseColor("#0078FF"));//可点击文字的颜色
ds.setAntiAlias(true);
ds.setUnderlineText(false);//移除下划线
}
}, ellipsizeStr.length(), totalContent.length(), Spanned.SPAN_EXCLUSIVE_INCLUSIVE);
textView.setMovementMethod(LinkMovementMethod.getInstance());//增加该方法,否则点击不生效
}
textView.setText(spanString);
}
}
小结
核心Api:TextUtils.ellipsize()
可以截取指定行数长度的文本内容,不能识别文本内的换行符。