1. 内置对象
之前我们使用一个对象,必须通过类名new出来,之后才能使用!
User user=new User();
user.属性名/user.方法
但是我们刚才看到了一些列的request.getXXX()
疑问?request对象何时被创建?
在jsp中,不需要我们手动的实例化,直接使用的对象====》内置对象
内置对象 | 作用 | 对应的Java类 |
Page | 当前页面 | This |
pageContext | 当前页面上下文对象 | PageContext |
request | 请求对象 | HttpServletRequest |
response | 响应对象 | HttpServletResponse |
session | 会话对象 | HttpSession |
application | 应用程序对象 | ServletContext |
out | 输出对象 | JspWriter |
config | 配置对象 | ServletConfig |
exception | 异常对象 | Throwable |
JSP执行流程
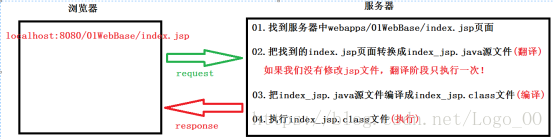
声明成员变量和局部变量
<%
//声明局部变量 会在service方法中出现
int num1=5;
int num2=10;
%>
<%! //声明成员变量 会在 index_jsp.java类中出现
int num1=50;
int num2=100;
//定义方法
public int getSum(){
System.out.print(num1+num2);
return num1+num2;
}
%>
<%!
//成员变量
int initNum=0;
int serviceNum=0;
int destroyNum=0;
public void jspInit(){
System.out.println("jspInit====>初始化执行了"+(++initNum)+"次");
//只有在用户第一次访问页面的时候执行
}
public void jspDestroy(){
System.out.println("jspDestroy====>销毁执行了"+(++destroyNum)+"次");
//只有在关闭服务器的时候执行
}
%>
<% // 只要是写在这个 <%中的数据 务必在service方法中执行
System.out.println("jspInit====>初始化执行了"+(initNum)+"次");
System.out.println("jspDestroy====>销毁执行了"+(destroyNum)+"次");
System.out.println("jspService====>service执行了"+(++serviceNum)+"次");
System.out.println("==========================================================");
// 用户每访问一次 执行一次
%>
二:JSP的数据交互
2.1:Request请求对象
1.登录 login.jsp页面
<body>
<h1>登录页面</h1>
<form action="doLogin.jsp" method="get">
<input type="text" name="userName" placeholder="请输入用户名"> <br/>
<input type="password" name="pwd" placeholder="请输入密码"><br/>
爱好:<br/>
<input type="checkbox" name="love" value="java">java<br/>
<input type="checkbox" name="love" value="sql">sql<br/>
<input type="checkbox" name="love" value="javaScript">javaScript<br/>
<input type="submit" value="提交信息">
</form>
</body>
2. 处理页面 doLogin.jsp
解决get请求方式乱码治本的办法
<body>
<h1>这是doLogin处理页面</h1>
<%
//解决post请求方式乱码治标的办法 后续使用 过滤器Filter 根本上解决post请求乱码的问题
request.setCharacterEncoding("utf-8");
//使用request请求对象 获取用户在login.jsp页面输入和选择的内容
//通过form表单中的name属性值获取对应的value值
String userName= request.getParameter("userName");
String pwd= request.getParameter("pwd");
out.println("用户名==》"+userName+"<br/>");
out.println("密码==》"+pwd+"<br/>");
String [] loves= request.getParameterValues("love"); //获取用户选择项
if(loves!=null&&loves.length>0){
for(String love:loves){
out.println(love+"<br/>");
}
}
%>
</body>
3. request的常用方法
getContextPath | 获取web项目的相对路径 |
getSchme | 获取的协议名称 |
getServerName | 获取服务器名称 |
getServlerPort | 获取服务器端口号 |
getParameter | 获取指定的请求参数值 |
GetParameterValues | 获取指定的请求参数集合 |
GetSession | 获取当前请求所在的session对象 |
GetCookies | 获取所有的cookie数组 |
SetAttribute | 向request作用域中保存属性和值 |
GetAttribute | 从request作用域中获取指定属性的值 |
SetCharacterEncoding | 设置请求的编码格式 |
GetMethod | 获取请求类型 |
GetRequestDispatcher | 获取转发器 |
2.2:Response响应对象
SetCharacterEncoding | 设置响应的编码格式 |
addCookie | 添加cookie |
SetHeader | 设置消息头信息 |
SetStatus | 设置状态码 |
SendRedirect | 重定向 |
SetContentType | 设置浏览器的数据类型 |
GetOutputStream | 获取输出到浏览器的字节流对象 |
GetWriter | 获取输出到浏览器的字符流对象 |
2.3:转发
特点:
01.转发是服务器的行为
02.URL地址不会变化
03.数据不会丢失
04.延长一次请求作用域
Aaa.jsp
<body>
<form action="Bbb.jsp" method="get">
<table>
<tr>
<td>用户名</td>
<td><input type="userName" name="userName"></td>
</tr>
<tr>
<td>密码</td>
<td><input type="password" name="password"></td>
</tr>
<tr>
<td colspan="2">
<input type="submit" value="登录">
</td>
</tr>
</table>
</form>
</body>
Bbb.jsp
<body>
<%
String userName = request.getParameter("userName");
String password = request.getParameter("password");
if (userName.equals("meng") && password.equals("3588105")) {
//
request.getRequestDispatcher("Ccc.jsp").forward(request, response);
}else{
out.print("账号或者密码不正确");
}
%>
</body>
Ccc.jsp
<body>
您好欢迎访问
</body>

2.4:重定向
特点:
01.重定向是客户端的行为
02.URL地址会变化,是最后一次请求的路径
03.数据会丢失
04.至少两次访问服务器
<body>
<form action="Bbb.jsp" method="get">
<table>
<tr>
<td>用户名</td>
<td><input type="userName" name="userName"></td>
</tr>
<tr>
<td>密码</td>
<td><input type="password" name="password"></td>
</tr>
<tr>
<td colspan="2">
<input type="submit" value="登录">
</td>
</tr>
</table>
</form>
</body>
这个是:Bbb.jsp<body>
<%
String userName = request.getParameter("userName");
String password = request.getParameter("password");
if (userName.equals("meng") && password.equals("3588105")) {
request.getRequestDispatcher("Ccc.jsp").forward(request, response);
}else{
out.print("账号或者密码不正确");
}
%>
</body>
<body>
<%
String userName = request.getParameter("userName");
String password = request.getParameter("password");
if (userName.equals("meng") && password.equals("3588105")) {
request.getRequestDispatcher("Ccc.jsp").forward(request, response);
}else{
out.print("账号或者密码不正确");
}
%>
</body>
<body>
<%
String userName = request.getParameter("userName");
String password = request.getParameter("password");
if (userName.equals("meng") && password.equals("3588105")) {
//1.通过响应告诉浏览器该抢救这样的一个地址
//2.浏览器访问指定的URL==== Ccc.jsp
response.sendRedirect("Ccc.jsp");
}else{
out.print("账号或者密码不正确");
}
%>
</body>
<body>
您好欢迎访问 Ccc.jsp
</body>
总结:
选择进入
<body>
<a href="result.jsp?color=黄色">黄色</a>
<a href="result.jsp?color=绿色">绿色</a>
<a href="result.jsp?color=紫色">紫色</a>
<a href="result.jsp?color=黑色">黑色</a>
<a href="result.jsp?color=粉色">粉色</a>
</body>
<body>
您所选择的是<%= request.getParameter("color") %>
</body>