jQuery应用举例
一.隔行换色
- 页面:
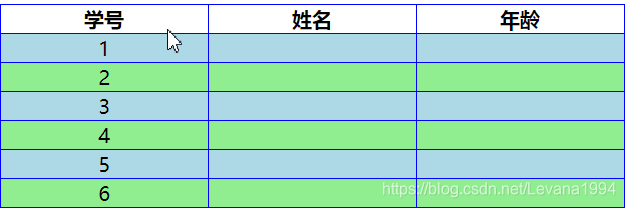
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
table{
width: 500px;
margin: 0 auto;
border-collapse: collapse;
}
td,th{
border: 1px solid blue;
text-align: center;
}
.evenTr{
background-color: lightblue;
}
.oddTr{
background-color: lightgreen;
}
.activeTr{
background-color: #ccc;
}
</style>
</head>
<body>
<table>
<tr>
<th>学号</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<tr>
<td>1</td>
<td></td>
<td></td>
</tr>
<tr>
<td>2</td>
<td></td>
<td></td>
</tr>
<tr>
<td>3</td>
<td></td>
<td></td>
</tr>
<tr>
<td>4</td>
<td></td>
<td></td>
</tr>
<tr>
<td>5</td>
<td></td>
<td></td>
</tr>
<tr>
<td>6</td>
<td></td>
<td></td>
</tr>
</table>
<script src="../js/jquery-3.3.1.min.js"></script>
<script>
$("tr:gt(0):odd").addClass("oddTr");
$("tr:gt(0):even").addClass("evenTr");
$("tr:gt(0)").mouseover(function () {
$(this).toggleClass("activeTr");
}).mouseout(function () {
$(this).toggleClass("activeTr");
})
</script>
</body>
</html>
二.全选全消
- 页面:
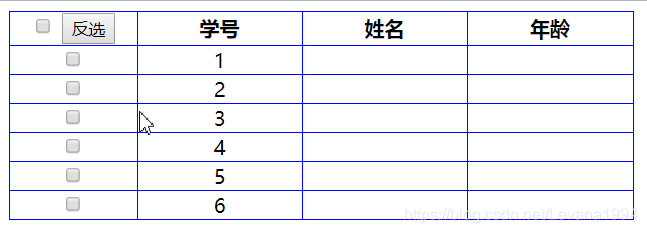
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
table{
width: 500px;
margin: 0 auto;
border-collapse: collapse;
}
td,th{
border: 1px solid blue;
text-align: center;
}
</style>
</head>
<body>
<table>
<tr>
<th style="width:100px;">
<input type="checkbox" id="checkall" title="全选/全消"/>
<input type="button" value="反选" id="reverse">
</th>
<th>学号</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>1</td>
<td></td>
<td></td>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>2</td>
<td></td>
<td></td>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>3</td>
<td></td>
<td></td>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>4</td>
<td></td>
<td></td>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>5</td>
<td></td>
<td></td>
</tr>
<tr>
<td>
<input type="checkbox" class="item" />
</td>
<td>6</td>
<td></td>
<td></td>
</tr>
</table>
<script src="../js/jquery-3.3.1.min.js"></script>
<script>
$("#checkall").click(function () {
$(".item").prop("checked", this.checked);
});
$("#reverse").click(function () {
$(".item").click();
});
$(".item").click(function () {
var v = $(".item").length === $(".item:checked").length;
$("#checkall").prop("checked", v);
});
</script>
</body>
</html>
三.QQ表情选择
- 页面
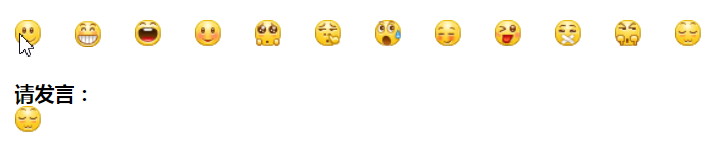
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>QQ表情选择</title>
<style type="text/css">
*{margin: 0;padding: 0;list-style: none;}
.emoji{margin:50px;}
ul{overflow: hidden;}
li{float: left;width: 48px;height: 48px;cursor: pointer;}
.emoji img{ cursor: pointer; }
</style>
</head>
<body>
<div class="emoji">
<ul>
<li><img src="img/01.gif" height="22" width="22" alt="" /></li>
<li><img src="img/02.gif" height="22" width="22" alt="" /></li>
<li><img src="img/03.gif" height="22" width="22" alt="" /></li>
<li><img src="img/04.gif" height="22" width="22" alt="" /></li>
<li><img src="img/05.gif" height="22" width="22" alt="" /></li>
<li><img src="img/06.gif" height="22" width="22" alt="" /></li>
<li><img src="img/07.gif" height="22" width="22" alt="" /></li>
<li><img src="img/08.gif" height="22" width="22" alt="" /></li>
<li><img src="img/09.gif" height="22" width="22" alt="" /></li>
<li><img src="img/10.gif" height="22" width="22" alt="" /></li>
<li><img src="img/11.gif" height="22" width="22" alt="" /></li>
<li><img src="img/12.gif" height="22" width="22" alt="" /></li>
</ul>
<strong>请发言:</strong>
<p class="word" contenteditable="true">
<img src="img/12.gif" height="22" width="22" alt="" />
</p>
</div>
<script src="../js/jquery-3.3.1.min.js"></script>
<script>
$("ul img").click(function () {
$(this).clone().appendTo(".word");
});
</script>
</body>
</html>
四.分组菜单
- 页面
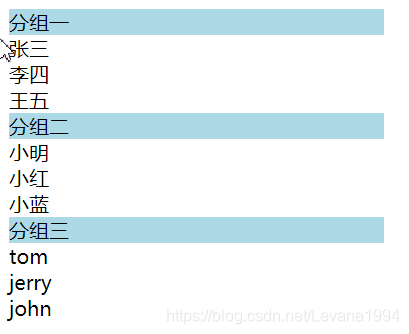
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
div{
width:300px;
}
span, a{
display: block;
width:300px;
}
span{
background-color: lightblue;
}
</style>
</head>
<body>
<div>
<span>分组一</span>
<a>张三</a>
<a>李四</a>
<a>王五</a>
</div>
<div>
<span>分组二</span>
<a>小明</a>
<a>小红</a>
<a>小蓝</a>
</div>
<div>
<span>分组三</span>
<a>tom</a>
<a>jerry</a>
<a>john</a>
</div>
<script type="text/javascript" src="./jquery-3.3.1.js" ></script>
<script type="text/javascript">
$("a").hide();
$("span").click(function(){
$(this).siblings("a").show(200);
$(this).parent().siblings("div").children("a").hide(200);
});
</script>
</body>
</html>
五.省市联动
- 页面

<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<select id="province">
<option value="">--选择省--</option>
<option value="0">河南省</option>
<option value="1">河北省</option>
<option value="2">山东省</option>
<option value="3">山西省</option>
</select>
<select id="city">
<option value="">--选择市--</option>
</select>
<script type="text/javascript" src="./jquery-3.3.1.js" ></script>
<script type="text/javascript">
var cities = [
["郑州市","洛阳市","开封市"],
["石家庄市","保定市","唐山市"],
["济南市","烟台市","青岛市"],
["太原市","晋中市","临汾市"]
];
$("#province").change(function(){
$("#city").html("<option value=''>--选择市--</option>");
var myCities = cities[this.value];
$(myCities).each(function(){
$("<option></option>").html(this).appendTo("#city");
});
});
</script>
</body>
</html>
六.左右选择
- 页面
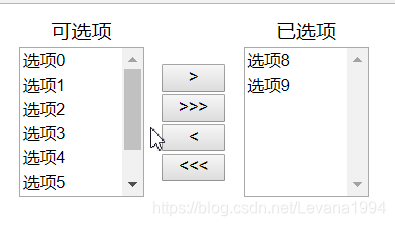
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
table{
width: 300px;
margin: 0 auto;
}
select{
width: 100px;
height: 120px;
}
td{
text-align: center;
}
input[type="button"]{
width: 50px;
}
</style>
</head>
<body>
<table>
<tr>
<td>可选项</td>
<td></td>
<td>已选项</td>
</tr>
<tr>
<td>
<select id="left" multiple="multiple">
<option>选项0</option>
<option>选项1</option>
<option>选项2</option>
<option>选项3</option>
<option>选项4</option>
<option>选项5</option>
<option>选项6</option>
<option>选项7</option>
</select>
</td>
<td>
<input type="button" value=">" id="toRight"/><br />
<input type="button" value=">>>" id="allToRight"/><br />
<input type="button" value="<" /><br />
<input type="button" value="<<<" /><br />
</td>
<td>
<select id="right" multiple="multiple">
<option>选项8</option>
<option>选项9</option>
</select>
</td>
</tr>
</table>
<script type="text/javascript" src="./jquery-3.3.1.js" ></script>
<script type="text/javascript">
$("#toRight").click(function(){
$("#left>option:selected").appendTo("#right");
});
$("#allToRight").click(function(){
$("#left>option").appendTo("#right");
});
</script>
</body>
</html>
七.定时广告
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>定时广告</title>
</head>
<body>
<img id="ad" src="img/ad.jpg" alt="" style="width:100%;display: none">
<script src="js/jquery-3.3.1.min.js"></script>
<script>
setTimeout(function () {
$("#ad").fadeIn(500);
setTimeout(function () {
$("#ad").fadeOut(500);
}, 3000);
}, 2000);
</script>
</body>
</html>
八.抽奖程序
- 页面
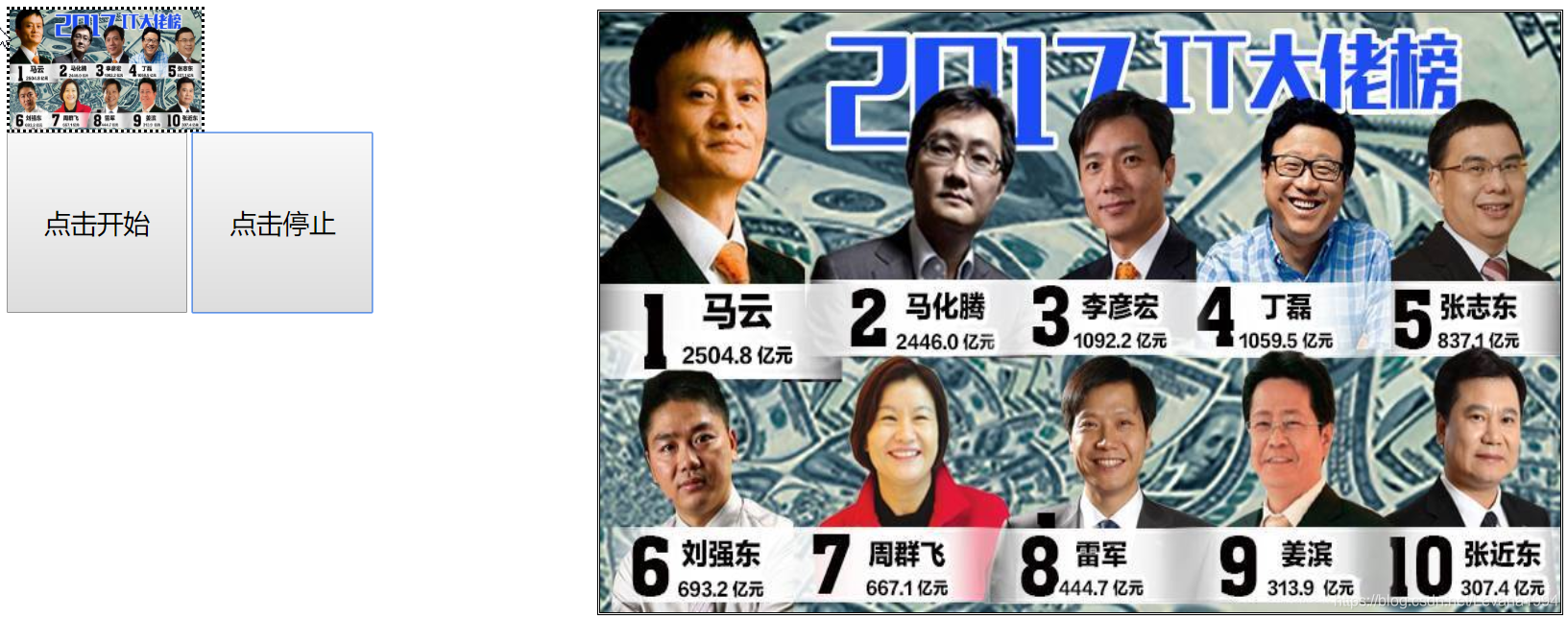
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>jquery案例之抽奖</title>
</head>
<body>
<div style="border-style:dotted;width:160px;height:100px">
<img id="img1ID" src="img/man00.jpg" style="width:160px;height:100px"/>
</div>
<div style="border-style:double;width:800px;height:500px;position:absolute;left:500px;top:10px">
<img id="img2ID" src="img/man00.jpg" width="800px" height="500px"/>
</div>
<input
id="startID"
type="button"
value="点击开始"
style="width:150px;height:150px;font-size:22px"
onclick="imgStart()">
<input
id="stopID"
type="button"
value="点击停止"
style="width:150px;height:150px;font-size:22px"
onclick="imgStop()">
<script type="text/javascript" src="js/jquery-3.3.1.min.js"></script>
<script language='javascript' type='text/javascript'>
var imgs = [
"img/man00.jpg",
"img/man01.jpg",
"img/man02.jpg",
"img/man03.jpg",
"img/man04.jpg",
"img/man05.jpg",
"img/man06.jpg"
];
var timer;
function imgStart() {
timer = setInterval(function () {
var index = Math.floor(Math.random() * 7) ;
var imageSrc = imgs[index];
$("#img1ID").attr("src", imageSrc);
}, 20);
$("#startID").attr("disabled", true);
}
function imgStop() {
clearInterval(timer);
var imageSrc = $("#img1ID").attr("src");
$("#img2ID").attr("src", imageSrc);
$("#startID").attr("disabled", false);
}
</script>
</body>
</html>
九.表单校验
- 页面
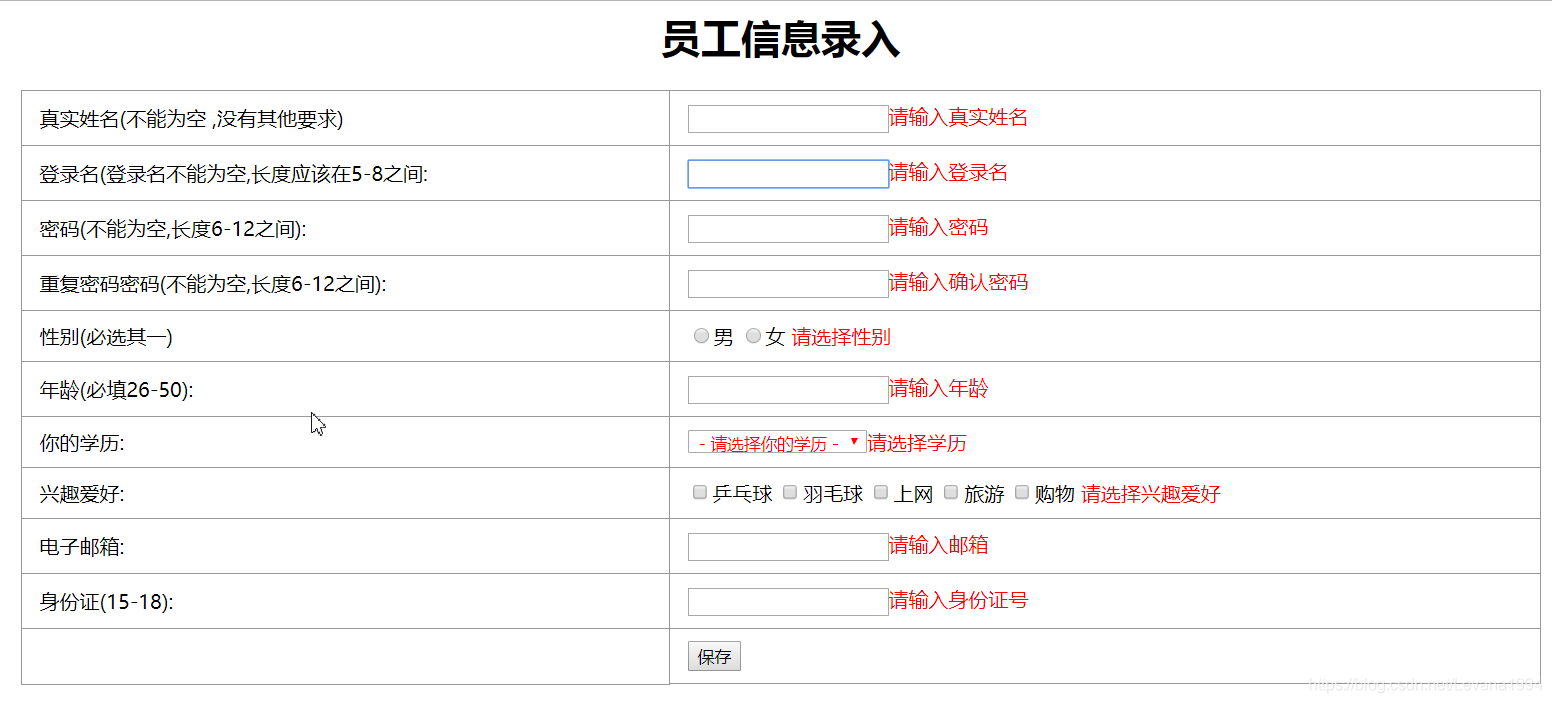
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>我的jquery表单校验页面</title>
<style type="text/css">
h1 {
text-align: center;
}
table {
width: 80%;
margin: 0 auto;
border-collapse: collapse;
}
td {
padding: 10px 14px;
border: 1px solid #999;
}
.error {
color: red;
}
</style>
</head>
<body>
<h1>员工信息录入</h1>
<form name="empForm" id="empForm" method="get" action="#">
<table>
<tr>
<td>真实姓名(不能为空 ,没有其他要求)</td>
<td><input type="text" id="realname" name="realname" />
</td>
</tr>
<tr>
<td>登录名(登录名不能为空,长度应该在5-8之间:</td>
<td><input type="text" id="username" name="username" /></td>
</tr>
<tr>
<td>密码(不能为空,长度6-12之间):</td>
<td><input type="password" id="pwd" name="pwd" /></td>
</tr>
<tr>
<td>重复密码密码(不能为空,长度6-12之间):</td>
<td><input type="password" id="pwd2" name="pwd2" /></td>
</tr>
<tr>
<td>性别(必选其一)</td>
<td>
<input type="radio" id="male" value="m" name="sex" />男
<input type="radio" id="female" value="f" name="sex" />女
<label class="error" for="sex"></label>
</td>
</tr>
<tr>
<td>年龄(必填26-50):</td>
<td><input type="text" id="age" name="age" /></td>
</tr>
<tr>
<td>你的学历:</td>
<td>
<select name="edu" id="edu">
<option value="">-请选择你的学历-</option>
<option value="a">专科</option>
<option value="b">本科</option>
<option value="c">研究生</option>
<option value="e">硕士</option>
<option value="d">博士</option>
</select>
</td>
</tr>
<tr>
<td>兴趣爱好:</td>
<td colspan="2">
<input type="checkbox" name="hobby" id="pp" value="0" />乒乓球
<input type="checkbox" name="hobby" id="ym" value="1" />羽毛球
<input type="checkbox" name="hobby" id="sw" value="2" />上网
<input type="checkbox" name="hobby" id="ly" value="3" />旅游
<input type="checkbox" name="hobby" id="gw" value="4" />购物
<label class="error" for="hobby"></label>
</td>
</tr>
<tr>
<td align="left">电子邮箱:</td>
<td><input type="text" id="email" name="email" /></td>
</tr>
<tr>
<td align="left">身份证(15-18):</td>
<td><input type="text" id="idcard" name="idcard" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" id="smtBtn" value="保存"></td>
</tr>
</table>
</form>
<script src="js/jquery-3.3.1.min.js"></script>
<script src="js/jquery.validate.min.js"></script>
<script>
$("#empForm").validate({
rules:{
realname:{
required:true
},
username:{
required:true,
rangelength:[5, 8]
},
pwd:{
required:true,
rangelength:[6, 12]
},
pwd2:{
required:true,
rangelength:[6, 12],
equalTo:"#pwd"
},
sex:{
required:true
},
age:{
required:true,
range:[26, 50],
digits:true
},
edu:{
required:true
},
hobby:{
required:true
},
email:{
required:true,
email:true
},
idcard:{
required:true,
card:true
}
},
messages:{
realname:{
required:"请输入真实姓名"
},
username:{
required:"请输入登录名",
rangelength:"登录名必须是5~8位"
},
pwd:{
required:"请输入密码",
rangelength:"密码必须是6~12位"
},
pwd2:{
required:"请输入确认密码",
rangelength:"确认密码必须是6~12位",
equalTo:"两次密码输入不一致"
},
sex:{
required:"请选择性别"
},
age:{
required:"请输入年龄",
range:"年龄必须是26~50岁之间",
digits:"年龄必须是整数"
},
edu:{
required:"请选择学历"
},
hobby:{
required:"请选择兴趣爱好"
},
email:{
required:"请输入邮箱",
email:"邮箱格式不正确"
},
idcard:{
required:"请输入身份证号",
card:"身份证号格式不正确"
}
}
});
$.validator.addMethod("card",function(value,element,params){
var reg = /^\d{15}(\d{2}[\dX])?$/i;
var result = reg.test(value);
if (!result) {
$(element).css("background-color","green");
}else{
$(element).css("background-color","white");
}
return result;
}, "idcard number error");
</script>
</body>
</html>