反射
- 反射在Java框架中应用的很多
(1)反射的价值在于处理编译时未知的类型,从而编写更具有通用性的代码。
(2)反射机制是java实现动态语言的关键,在不修改源码的情况下,进行功能的扩展。
User user = new User();
System.out.println(user.name);
- 传统的直接操作成员的方式很清晰,但是有些场景中需要动态的去操作一些成员。比如:在运行时根据配置文件中的类名和方法名、或者是基于字符串变量来动态实例化对象或调用方法时,这种传统的方法就不适用了。而反射确提供了这种能力。反射关键在于Class对象,称为类对象
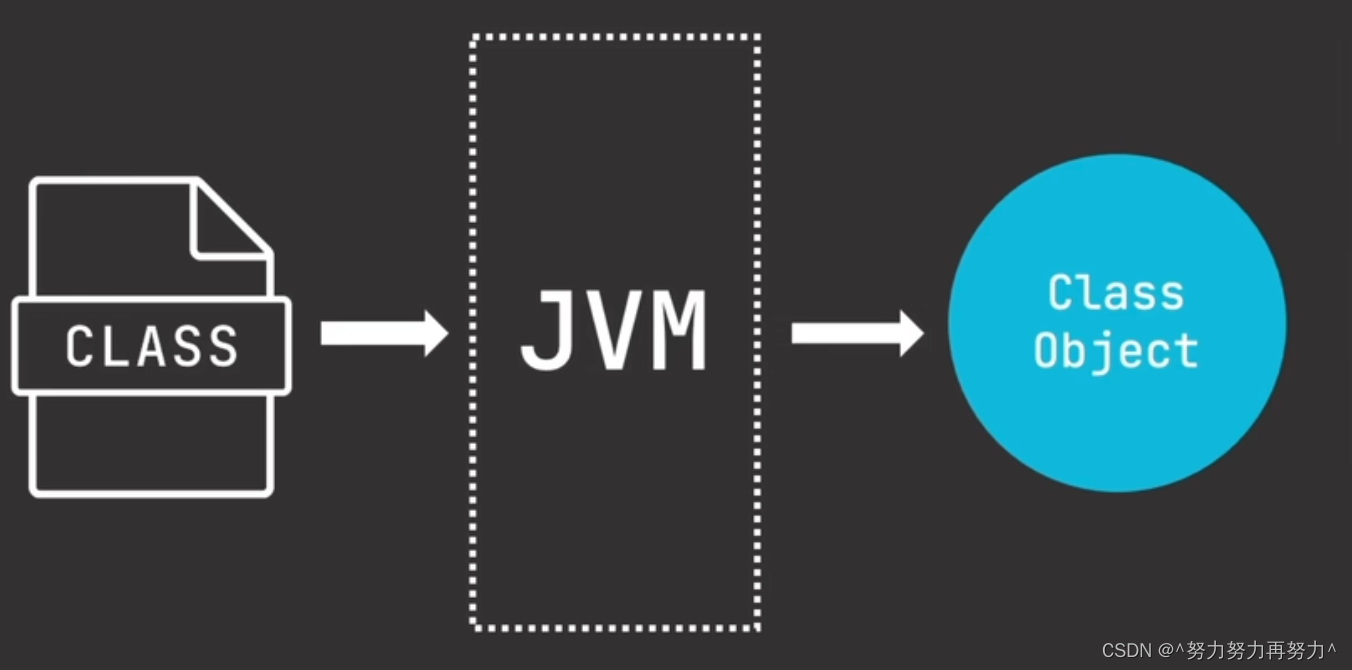
- Class对象是由虚拟机自动创建的,用于存储类的信息,通过拿到Class对象,就可以访问类的结构,以及类本身和它的实例进行操作,
- 虚拟机创建类对象的创建过程如下
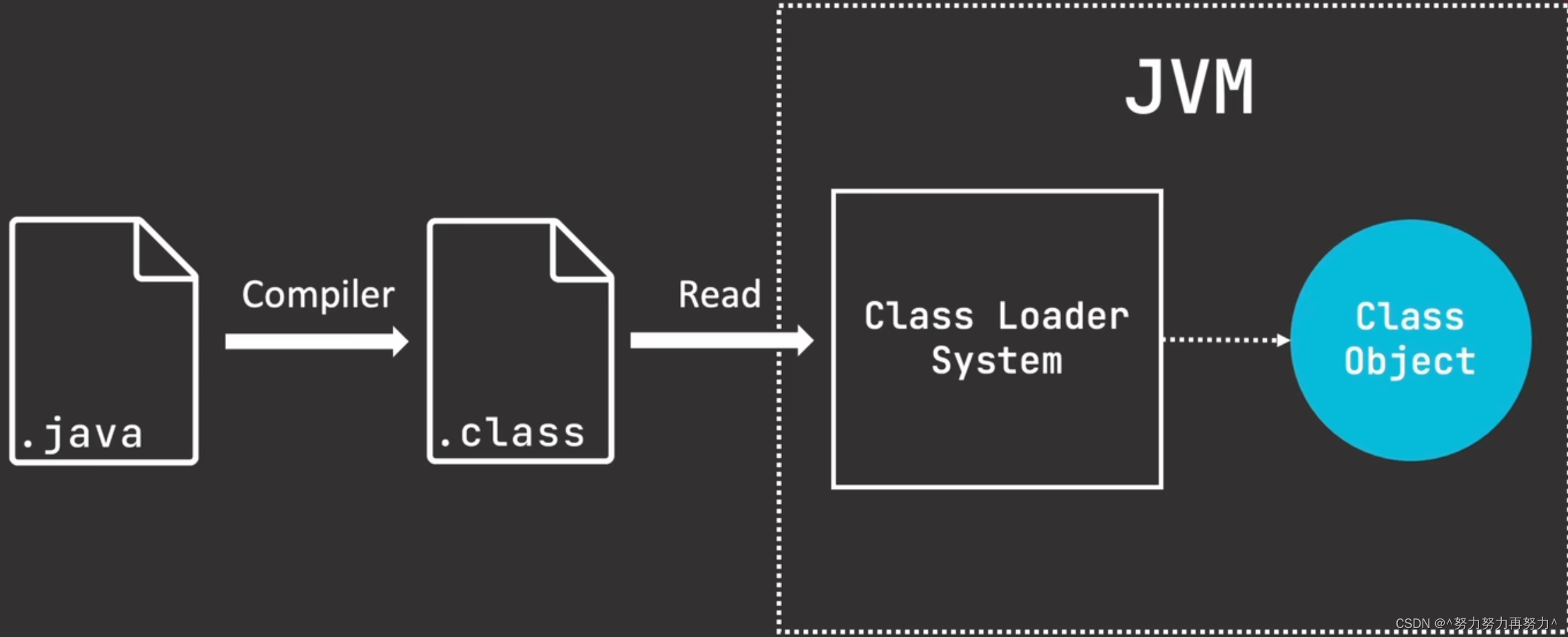
- 我们编写完一个类(Cat)时,并完成编译以后,编译器会将其转换为字节码文件(存储在.class文件中)
- 虚拟机JVM 用 ClassLoader 读取.class文件,将里面的字节码加载到内存的堆中,并基于这些信息创建Class对象,而且类只加载一次,所以在内存的堆中,每个类都唯一的对应着一个Class对象
- 创建类对象有三种方式
- 类是明确的------> User.class-------> 在编译时就确定了具体的类,属于静态引用
- 如果有了某个类的实例对象------> Class<?> clazz = user.getClass() ----> 这里的泛型为什么是通配符---->Class对象是在运行时从user实例获取的,而User实例的具体类型,只能在运行时创建和确定,在编译阶段,我们无法准确的判断Class对象的确切类型,所以说,如果填写一个确切的泛型,就会报错。
- 使用Class的forName的静态方法,用于在运行时动态加载指定的类,并返回该类的Class对象实例------> 通常用于类名在编译时不可知的场景中。 Class<?> clazz = Class.forName(“org.example.Cat”);----> 它也是在运行时才能确定类型,泛型为 ? --------> 通过这种方法得到Class对象,会立即触发类的初始化、类的静态代码块,即static块
- Class对象有很多方法
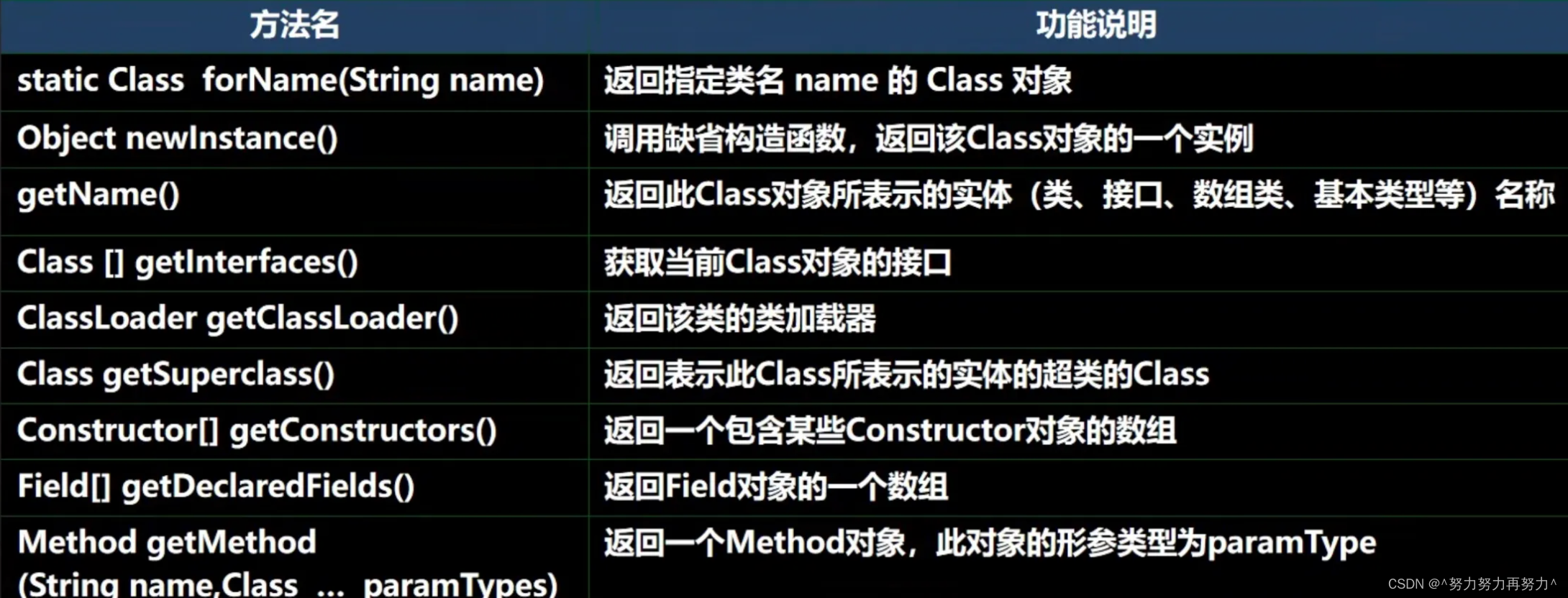
- 通过反射获取字段的过程,是在运行时动态执行的,所以无法在编译阶段进行错误的检测或捕获,错误只能在程序执行时 被发现和处理-------> 所以在使用反射时,要有完善的错误处理机制。
Class<?> cls = Class.forName("org.reflection_.Person");
Method hiMethodName = cls.getDeclaredMethod("hi", String.class);
hiMethodName.setAccessible(true);
hiMethodName.invoke(null, "Tom");
System.out.println(o2);
- 为了更好的理解反射的实际应用场景,最后模拟了一下框架功能,创建一些通用方法,通过一些配置,来实现服务的依赖注入。
package org.reflection_;
import org.Bean;
public class Customer {
private String name;
private String email;
public Customer(String name, String email) {
this.name = name;
this.email = email;
}
public String getName() {
return name;
}
@Bean
public String getEmail() {
return email;
}
@Bean
public void printName() {
System.out.println("Customer name" + name);
}
public void printEmail() {
System.out.println("Customer email" + email);
}
}
package org.reflection_;
public class Address {
public String street;
public String postCode;
public Address(String street, String postCode) {
this.street = street;
this.postCode = postCode;
}
public void getStreet() {
System.out.println("Address street: " + street);
}
public void getPostCode() {
System.out.println("Address postcode" + postCode);
}
}
package org.reflection_;
import org.Bean;
public class Config {
@Bean
public Customer customer() {
return new Customer("Alex", "Alex@gemail.com");
}
@Bean
public Address address() {
return new Address("石家庄市", "050000");
}
public Message message(){
return new Message("Hi there");
}
}
package org.reflection_;
import org.Autowired;
import org.Bean;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
public class Container {
private Map<Class<?>, Method> methods;
private Object config;
private Map<Class<?>, Object> service;
public void init() throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, InstantiationException, IllegalAccessException {
this.methods = new HashMap<>();
this.service = new HashMap<>();
Class<?> clazz = Class.forName("org.reflection_.Config");
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
if (method.getAnnotation(Bean.class) != null) {
this.methods.put(method.getReturnType(), method);
}
}
this.config = clazz.getConstructor().newInstance();
}
public Object getServiceInstanceByClass(Class<?> clazz) throws InvocationTargetException, IllegalAccessException {
if (this.service.containsKey(clazz)) {
return service.get(clazz);
} else {
if (this.methods.containsKey(clazz)) {
Method method = this.methods.get(clazz);
Object obj = method.invoke(this.config);
this.service.put(clazz, obj);
return obj;
}
}
return null;
}
public Object createInstance(Class<?> clazz) throws InvocationTargetException, IllegalAccessException, InstantiationException, NoSuchMethodException {
Constructor<?>[] constructors = clazz.getDeclaredConstructors();
for (Constructor<?> constructor : constructors) {
if (constructor.getAnnotation(Autowired.class) != null) {
Class<?>[] parameterTypes = constructor.getParameterTypes();
Object[] arguments = new Object[parameterTypes.length];
for (int i = 0; i < parameterTypes.length; i++) {
arguments[i] = getServiceInstanceByClass(parameterTypes[i]);
}
return constructor.newInstance(arguments);
}
}
return clazz.getConstructor().newInstance();
}
}
package org.reflection_;
import org.Bean;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class Main {
public static void main(String[] args) throws ClassNotFoundException, InvocationTargetException, NoSuchMethodException, InstantiationException, IllegalAccessException, NoSuchFieldException {
Container container = new Container();
container.init();
String className = "org.reflection_.Order";
String fieldName = "customer";
Class<?> clazz = Class.forName(className);
Object obj = container.createInstance(clazz);
Field filed = clazz.getDeclaredField(fieldName);
filed.setAccessible(true);
Object filedValue = filed.get(obj);
Method[] methods = filedValue.getClass().getDeclaredMethods();
for (Method method : methods) {
if (method.getAnnotation(Bean.class) != null) {
method.invoke(filedValue);
}
}
}
}
- 采用这种设计,对象的实例化和方法的调用不再依赖硬编码的类名和方法名,而是基于字符串和注解Annotation 所提供的配置信息进行, 这使得代码非常的灵活且通用,特别适合于需要高度解耦的框架和应用