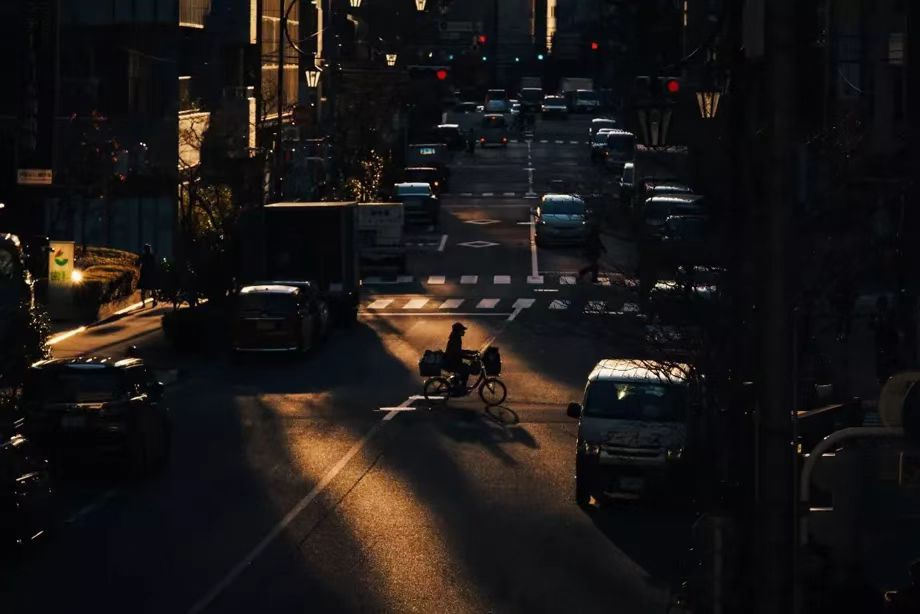
工作过程中,客户将input数据存放在FTP服务器的情况,通过查阅相关资料了解可从远程FTP下载文件到本地的功能需求,特此记录以下内容方便日后查阅与学习
问题:客户每周周一会上传最新的LTPerformance_FOC_xxxxxxxxxxxxxx到FTP服务器上,现需要将指定目录下的文件从FTP服务器中进行下载并加载到本地目录
LTPerformance_FOC_20220606151214数字含义
2022代表年份2022年
0606代表月份日期6月6号
15代表小时数 12代表分钟数 14代表秒数
数据(txt文本形式)存储在Pure Data文件夹中
实现上述功能代码如下所示:
from ftplib import FTP
import datetime
def get_current_monday():
'''
作用:获取当前周的周一
参数:无
返回值:年月日格式的字符串类型数值
'''
monday = datetime.date.today()
one_day = datetime.timedelta(days=1)
while monday.weekday() != 0:
monday -= one_day
return datetime.datetime.strftime(monday, "%Y%m%d")
def conn_ftp():
'''
作用:连接ftp服务器
参数:无
返回:ftp服务器连接的对象
'''
# ftp连接信息(可修改)
ftp_ip = "xx.xxx.xx.xx"
# 默认端口21
ftp_port = 21
# 连接ftp的用户名(可修改)
ftp_user = "xxx"
# 连接ftp的密码(可修改)
ftp_password = "xxx"
ftp = FTP()
# 连接ftp
ftp.connect(ftp_ip, ftp_port)
# ftp登录
ftp.login(ftp_user, ftp_password)
# 查看欢迎信息
print(ftp.getwelcome())
return ftp
def download_file(ftp, key, path, local_path):
'''
作用: 根据关键词下载文件
参数1:ftp连接对象
参数2:下载的关键词
参数3:要展示的目录
参数4:本地存放路径
返回:无
'''
# 进入指定目录
ftp.cwd(path)
for file_name in ftp.nlst():
if(key in file_name):
try:
print(file_name)
local_filename = local_path + "/" + file_name
f = open(local_filename, "wb")
# 下载ftp文件
ftp.retrbinary('RETR ' + file_name, f.write)
print('ftp文件成功下载到本地')
f.close()
except Exception as e:
print(e)
Monday = get_current_monday()
ftp = conn_ftp()
# 设置编码,解决上传的文件包含中文的问题
ftp.encoding = 'GBK'
# key下载的关键词(可修改)
key = "LTPerformance_FOC_"+ Monday
path = "/Pure Data/"
local_path = "D:/Pure_data/LTPerformance"
download_file(ftp, key, path, local_path)
导入本地电脑地址:"D:/Pure_data/LTPerformance"
对上述FTP有关函数进行优化,编写在ftp_func.py脚本中
# ftp_func.py
from ftplib import FTP
def ftp_connect(ftp_ip,ftp_port,ftp_user,ftp_password):
'''
作用: 连接ftp服务器
参数
1.ftp_ip:ftp连接信息(str格式)
2.ftp_port:端口(默认21)
3.ftp_user:连接ftp的用户名(str格式)
4.ftp_password:连接ftp的密码(str格式)
返回结果: ftp服务器连接的对象
示例: ftp = ftp_connect("xx.xxx.xx.xx",21,"xxx","xxx")
'''
ftp = FTP()
# 连接ftp
ftp.connect(ftp_ip, ftp_port)
# ftp登录
ftp.login(ftp_user, ftp_password)
# 查看欢迎信息
print(ftp.getwelcome())
return ftp
def download_file(ftp, key, path, local_path):
'''
作用: 根据关键词下载文件
参数:
1.ftp连接对象
2.下载的关键词(str格式)
3.读取存放ftp的目录(str格式)
4.本地存放路径(str格式)
返回结果: 读取成功并下载到本地则返回"ftp文件成功下载到本地"
示例:
ftp = ftp_connect("xx.xxx.xx.xx",21,"xxx","xxx")
key = "LTPerformance_FOC_20221017"
path = "/Pure Data/"
local_path = "D:/Pure_data/LTPerformance/LTPerformance_FOC"
download_file(ftp, key, path, local_path)
'''
# 进入指定目录
ftp.cwd(path)
for file_name in ftp.nlst():
if(key in file_name):
try:
print(file_name)
local_filename = local_path + "/" + file_name
f = open(local_filename, "wb")
# 下载ftp文件
ftp.retrbinary('RETR ' + file_name, f.write)
print('ftp文件成功下载到本地')
f.close()
except Exception as e:
print(e)
导入下述代码,即可使用ftp_connect以及download_file函数
from ftp_func import ftp_connect,download_file