119. Pascal’s Triangle II*
https://leetcode.com/problems/pascals-triangle-ii/
题目描述
Given a non-negative index k
where k ≤ 33
, return the kth
index row of the Pascal’s triangle.
Note that the row index starts from 0
.
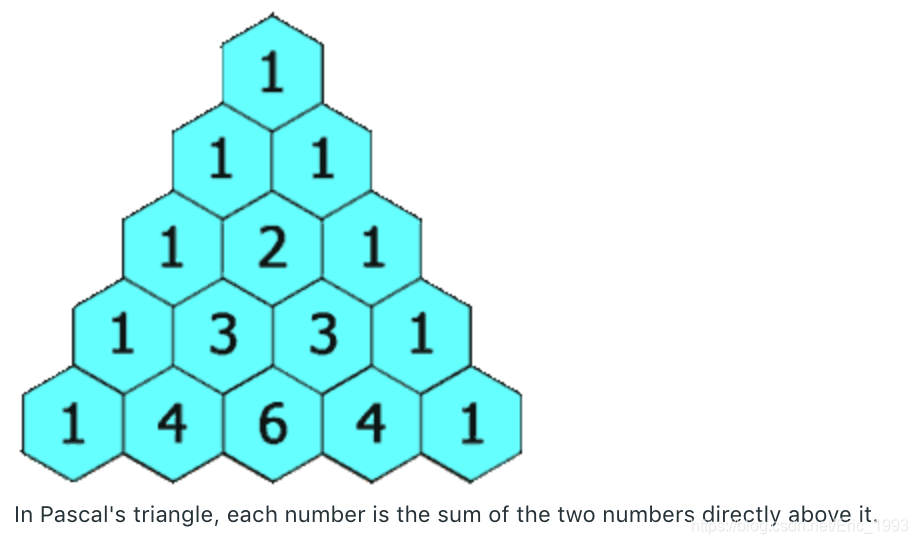
解题思路
杨辉三角形.
C++ 实现 0
20210324 更新: 使用递归更简洁:
class Solution {
public:
vector<int> getRow(int rowIndex) {
if (rowIndex == 0) return {1};
auto prev = getRow(rowIndex - 1);
vector<int> res(prev.size() + 1, 1);
for (int i = 1; i < res.size() - 1; ++ i) {
res[i] = prev[i - 1] + prev[i];
}
return res;
}
};
C++ 实现 1
来自 LeetCode 的 Submission, 我的实现在 C++ 实现 2
中.
class Solution {
public:
vector<int> getRow(int rowIndex) {
vector<int> res(rowIndex + 1, 1);
int prev, cur;
for(int i=2; i<=rowIndex; i++)
{
prev = res[0];
for(int j=1; j<i; j++)
{
cur = res[j];
res[j] = prev + cur;
prev = cur;
}
}
return res;
}
};
C++ 实现 2
class Solution {
private:
void generate_triangle(vector<int> &res, int time) {
if (time >= res.size()) return;
int n = res.size();
int prev = res[0];
for (int i = 1; i < time; ++i) {
int cur = res[i];
res[i] = prev + cur;
prev = cur;
}
generate_triangle(res, time + 1);
}
public:
vector<int> getRow(int rowIndex) {
if (rowIndex < 0) return {};
vector<int> res(rowIndex + 1, 1);
generate_triangle(res, 1);
return res;
}
};
C++ 实现 3
从后向前计算更为简洁.
class Solution {
public:
vector<int> getRow(int rowIndex) {
vector<int> result(rowIndex+1, 0);
result[0] = 1;
for(int i = 1; i <= rowIndex; i++) {
for(int j = i; j >= 1; j--) {
result[j] = result[j] + result[j-1];
}
}
return result;
}
};