1. IO流体系
- 按操作数据单位不同分为:字节流(8 bit),字符流(16 bit)
- 按数据流的流向不同分为:输入流,输出流
- 按流的角色的不同分为:节点流,处理流
- 节点流:直接从数据源或目的地读写数据

- 处理流:不直接连接到数据源或目的地,而是“连接”在已存在的流(节点流或处理流)之上,通过对数据的处理为程序提供更为强大的读写功能
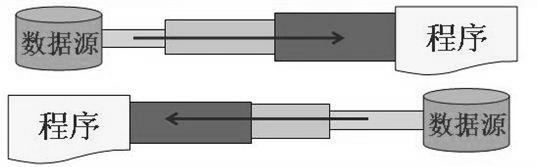
- IO流详细分类图
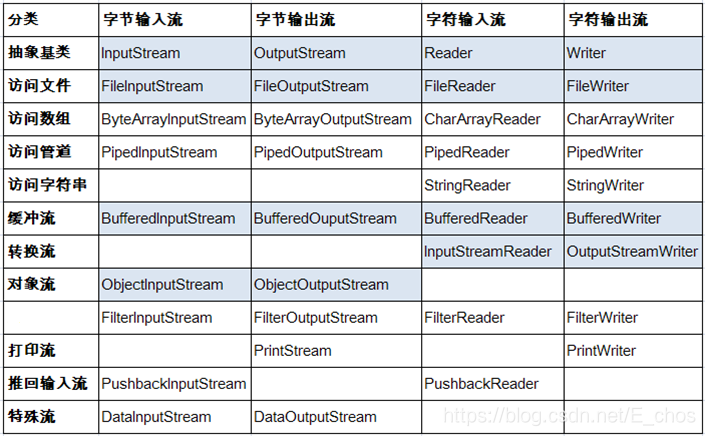
2. 节点流的使用
- 读取操作
@Test
public void test2() {
FileReader fr = null;
try {
File file = new File("src\\echo06\\hi.txt");
fr = new FileReader(file);
char[] cuff = new char[5];
int len;
while ((len = fr.read(cuff)) != -1) {
for (int i = 0; i < len; i++) {
System.out.print(cuff[i]);
}
String str = new String(cuff, 0, len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
- 写出操作
@Test
public void test3() {
FileWriter fw = null;
try {
File file = new File("src\\echo06\\hello.txt");
fw = new FileWriter(file);
fw.write("Hello world!\n");
fw.write("Hello CHINA!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
3. 处理流
- 转换流
@Test
public void test6() {
InputStreamReader isr = null;
try {
FileInputStream fis = new FileInputStream("src\\echo06\\hello.txt");
isr = new InputStreamReader(fis, StandardCharsets.UTF_8);
char[] buff = new char[13];
int len;
while ((len = isr.read(buff)) != -1) {
String str = new String(buff, 0, len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
assert isr != null;
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
- 缓冲流
提高读写速度
public void copyFileBuffered(String srcPath, String destPath) {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File(srcPath);
File destFile = new File(destPath);
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
assert bos != null;
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}