C 数据结构:基于队列的广度优先搜索
一、实现
#include<stdio.h>
#include<stdlib.h>
#include<unistd.h>
#include<string.h>
#define MAZE_ROW 6
#define MAZE_COL 8
typedef struct point
{
int y_;
int x_;
}Point;
typedef struct node
{
Point data_;
struct node* next_;
}Node;
typedef struct queue
{
Node* front_;
Node* rear_;
}Queue;
int maze[MAZE_ROW][MAZE_COL]={
1,1,1,1,1,1,1,1,
1,0,0,1,0,0,0,0,
1,1,0,1,0,1,1,1,
1,1,0,1,0,0,0,1,
0,0,0,0,0,1,1,1,
1,1,1,1,1,1,1,1};
Point path[MAZE_ROW][MAZE_COL];
void initQueue(Queue * queue);
int isEmpty(Queue *queue);
void enQueue(Queue *queue,Point ch);
Point deQueue(Queue *queue);
void destroyQueue(Queue *queue);
void disMaze();
void walkRoad(int y,int x,Point tp,Queue *queue);
int main()
{
Point startPoint={4,0},endPoint={1,7};
memset(path,0xff,sizeof(Point)*MAZE_ROW*MAZE_COL);
Queue q;
initQueue(&q);
enQueue(&q,startPoint);
int finishFlag=0;
while(!isEmpty(&q))
{
disMaze();
sleep(1);
printf("\033c");
Point tmpPoint=deQueue(&q);
maze[tmpPoint.y_][tmpPoint.x_]=2;
if(maze[tmpPoint.y_-1][tmpPoint.x_]>=0&&maze[tmpPoint.y_-1][tmpPoint.x_]==0)
walkRoad(tmpPoint.y_-1,tmpPoint.x_,tmpPoint,&q);
if(maze[tmpPoint.y_+1][tmpPoint.x_]<MAZE_ROW&&maze[tmpPoint.y_+1][tmpPoint.x_]==0)
walkRoad(tmpPoint.y_+1,tmpPoint.x_,tmpPoint,&q);
if(maze[tmpPoint.y_][tmpPoint.x_-1]>=0&&maze[tmpPoint.y_][tmpPoint.x_-1]==0)
walkRoad(tmpPoint.y_,tmpPoint.x_-1,tmpPoint,&q);
if(maze[tmpPoint.y_][tmpPoint.x_+1]<MAZE_COL&&maze[tmpPoint.y_][tmpPoint.x_+1]==0)
walkRoad(tmpPoint.y_,tmpPoint.x_+1,tmpPoint,&q);
if(endPoint.y_==tmpPoint.y_&&endPoint.x_==tmpPoint.x_)
{
finishFlag=1;
break;
}
}
if(1==finishFlag)
{
printf("find!\n");
Point pp=endPoint;
while(-1!=pp.y_)
{
printf("(%2d,%2d)",pp.y_,pp.x_);
pp=path[pp.y_][pp.x_];
}
putchar(10);
}
if(0==finishFlag) printf("find none!\n");
disMaze();
destroyQueue(&q);
return 0;
}
void initQueue(Queue * queue)
{
queue->front_=queue->rear_=(Node*)malloc(sizeof(Node));
if(NULL==queue->front_) exit(-1);
queue->front_->next_=NULL;
}
int isEmpty(Queue *queue){ return queue->front_==queue->rear_; }
void enQueue(Queue *queue, Point ch)
{
Node* ptr=(Node*)malloc(sizeof(Node));
if(NULL==ptr) exit(-1);
ptr->data_=ch;
ptr->next_=NULL;
queue->rear_->next_=ptr;
queue->rear_=ptr;
}
Point deQueue(Queue *queue)
{
Point tmp=queue->front_->next_->data_;
if(queue->front_->next_==queue->rear_)
{
queue->front_=queue->rear_;
free(queue->front_->next_);
queue->front_->next_=NULL;
}
else
{
Node* tp=queue->front_->next_;
queue->front_->next_=tp->next_;
free(tp);
}
return tmp;
}
void destroyQueue(Queue *queue)
{
Node *tp=queue->front_->next_;
queue->front_->next_=NULL;
queue->rear_=queue->front_;
Node* t;
while(tp)
{
t=tp->next_;
free(tp);
tp=t;
}
free(queue->front_);
}
void disMaze()
{
for(int j=0;j<MAZE_ROW;++j)
{
for(int i=0;i<MAZE_COL;++i)
{
if(1==maze[j][i]) printf("# ");
else if(0==maze[j][i]) printf(" ");
else printf("o ");
}
puts("");
}
}
void walkRoad(int y,int x,Point tp,Queue *queue)
{
Point p={y,x};
enQueue(queue,p);
path[y][x]=tp;
}
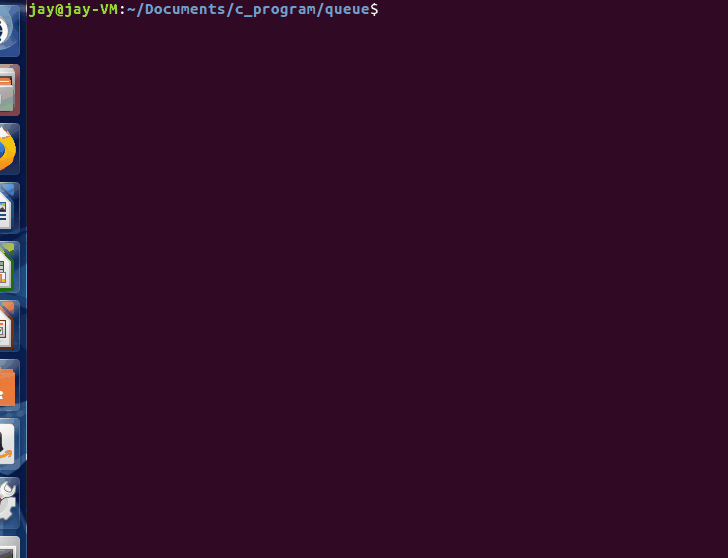