文件
深入理解计算机中文件的概念_BigXMeng的博客-优快云博客
什么是IO流?
所谓的IO流 就是输入输出流
IO的“I”对应单词Input IO的“O”对应单词Output
那么这里的Input和output的数据的来源和去向分别是哪里?
- Input数据的来源是外部存储设备(硬盘、光盘等外部设备) 去向是内存
- Output数据的来源是内存 去向是外部设备(硬盘、光盘等外部设备)
接下来的描述中,我将直接使用“I”和“O”来表示Input和Output
流的分类
(1)由于我们在进行I和O的时候,数据的传送单位不同(数据传送的单位可能是字节或者是字符),我们将流分为字节流和字符流(一个字符 >= 1个字节)
(2)由于我们在进行I和O的时候,数据的流向方向不同(数据传送的方向可能是 从其他存储设备流向内存 或者 从内存流向其他存储设备),我们将流分为输入流和输出流
(3)由于我们在进行I和O的时候,流当前所扮演的角色不同(流扮演的角色可能是字节流或者是处理流 处理流也成为包装流),我们将流分为字节流和处理流
当前上述的(1)(2)(3)当前只熟悉概念即可
流的具体分类(常用)
编程实践
字节流编程实践
FileInputStream 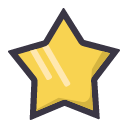
/**
@author Liu Xianmeng
@createTime 2022/12/1 9:07
@instruction 演示文件字节输入流的使用
*/
@SuppressWarnings({"all"})
public class JinFileInputStream {
public static void main(String[] args) throws Exception {
/**
* 字节读取模式
*/
String filePath = "E:\\abc.txt";
int readData = 0;
FileInputStream fileInputStream = null;
fileInputStream = new FileInputStream(filePath);
while ((readData = fileInputStream.read()) != -1) {
System.out.print((char)readData); }
fileInputStream.close();
System.out.println("\n******************");
/**
* 字节数组读取模式
*/
fileInputStream = new FileInputStream(filePath);
byte[] buf = new byte[8]; // 八个字节一读
int readLen = 0;
while ((readLen = fileInputStream.read(buf)) != -1) {
/** to display the reading string */
System.out.print(new String(buf, 0, readLen));
}
}
}
控制台结果:
FileInputStream 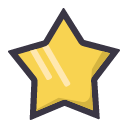
/**
@author Liu Xianmeng
@createTime 2022/12/1 9:17
@instruction 演示FileOutputStream的使用
*/
@SuppressWarnings({"all"})
public class JinFileOutputStream {
public static void main(String[] args) throws Exception {
String filePath = "E:\\abc.txt";
FileOutputStream fileOutputStream = null;
fileOutputStream = new FileOutputStream(filePath, true); /** true -> 是否 追加写 */
fileOutputStream.write(' ');
fileOutputStream.write('M');
fileOutputStream.write(' '); /** M */
String str = "jin, world!";
fileOutputStream.write(str.getBytes());
fileOutputStream.write(str.getBytes(), 0, 3);// write(byte[] b, int off 开始位置, int len 追加长度)
fileOutputStream.close();
}
}
(后续更新...)
字符流编程实践
InputStreamReader && BufferedReader && Stytem.in
/**
@author Liu Xianmeng
@createTime 2022/11/29 8:37
@instruction 用BufferedReader对象读取键盘输入的行信息 并打印输出
*/
@SuppressWarnings({"all"})
public class IOTEST {
public static void main(String[] args) throws Exception {
// 创建一个BufferedReader包装输入流
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
while(true){ // 持续按行读取并打印此行
String str = br.readLine();
System.out.println(str);
}
}
}
(后续更新...)
谢谢读取 >.<