代码:
#include <stdio.h>
#include<sys/stat.h>
#include<sys/types.h>
#include<fcntl.h>
#include<pthread.h>
#include<unistd.h>
//定义读取数据的变量
char buf[2]="";
//创建条件变量
int flag=0; //控制先运行哪个线程
pthread_cond_t cond=PTHREAD_COND_INITIALIZER;
pthread_mutex_t mutex=PTHREAD_MUTEX_INITIALIZER;
//读取回调函数
void* callback_read(void* arg)
{
int fd=*(int*)arg;
int i=1;
while(1)
{
//上锁
pthread_mutex_lock(&mutex);
if(flag!=0)
{
pthread_cond_wait(&cond,&mutex);
}
i=read(fd,buf,1);
if(0==i)
{
break;
}
flag=1;
//唤醒线程
pthread_cond_signal(&cond);
//解锁
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
//写到终端回调函数
void* callback_printf(void* arv)
{
int a=*(int*)arv;
for(int i=0;i<a;i++)
{
//上锁
pthread_mutex_lock(&mutex);
if(flag!=1)
{
pthread_cond_wait(&cond,&mutex);
}
fprintf(stdout,"%s",buf);
flag=0;
//唤醒线程
pthread_cond_signal(&cond);
//解锁
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
//打开读取的文件
int fd_r;
fd_r=open("./pthread_printf.c",O_RDONLY);
if(-1==fd_r)
{
perror("open");
return -1;
}
//获取文件大小
int a=lseek(fd_r,0,SEEK_END);
//修改文件偏移量到开头
lseek(fd_r,0,SEEK_SET);
//创建线程的tid号
pthread_t read;
pthread_t write;
//创建读取线程
pthread_create(&read,NULL,callback_read,&fd_r);
//创建打印线程
pthread_create(&write,NULL,callback_printf,&a);
//主线程阻塞等待分支线程退出
pthread_join(read,NULL);
pthread_join(write,NULL);
//销毁互斥锁
pthread_mutex_destroy(&mutex);
//销毁条件变量
pthread_cond_destroy(&cond);
return 0;
}
效果:
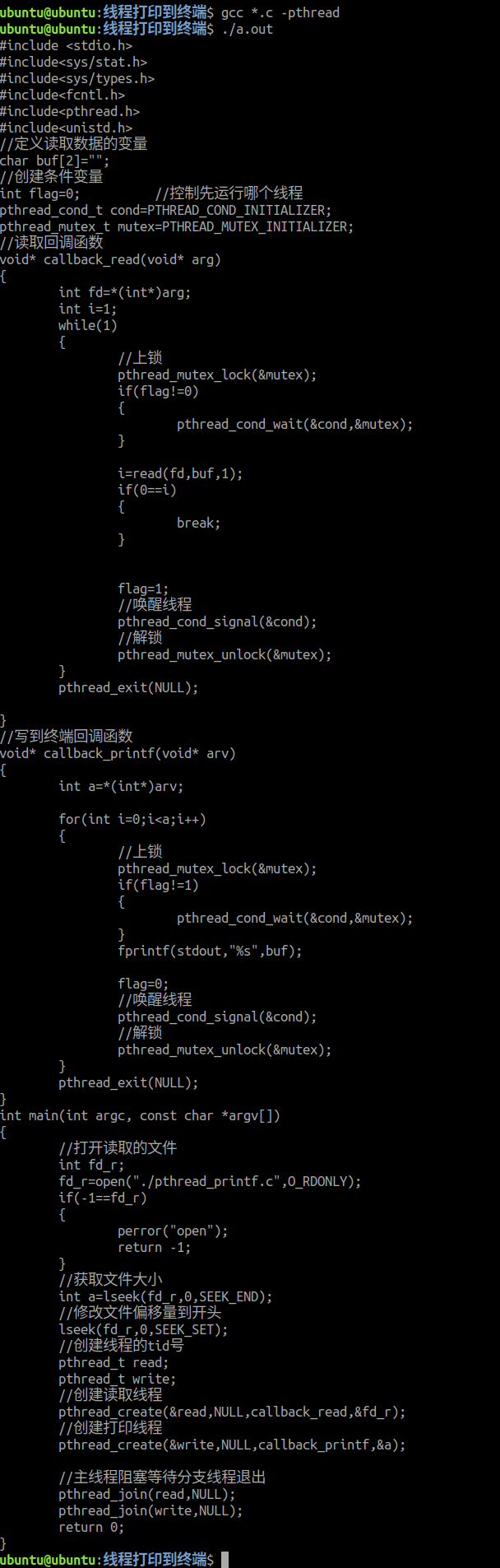