代码:
#include <stdio.h>
#include<sys/stat.h>
#include<sys/types.h>
#include<unistd.h>
#include<string.h>
#include<fcntl.h>
int main(int argc, const char *argv[])
{
//打开文件
int fp=open("./1.jpg",O_RDONLY);
int fd=open("./2.jpg",O_WRONLY|O_CREAT,0664);
//创建父子进程
pid_t pid=fork();
char buf[2]="";
//将子进程的文件偏移量偏移到文件中间
int size;
size_t a=0;
size=lseek(fp,0,SEEK_END);
size_t size_child=lseek(fp,size/2,SEEK_SET);
if(0==pid)
{
lseek(fp,size_child,SEEK_SET);
lseek(fd,size_child,SEEK_SET);
while(1)
{
//循环读取一个字符并且输出一个字符
a=read(fp,buf,1);
if(0==a)
{
printf("子进程中的后半部分复制完毕\n");
break;
}
write(fd,buf,1);
bzero(buf,0);
if(-1==a)
{
perror("read");
return -1;
}
}
}
if(pid>0)
{
sleep(2);
lseek(fp,0,SEEK_SET); //将读取的文件偏移量偏移到开头
lseek(fd,0,SEEK_SET); //将写入的文件的偏移量偏移到开头
for(int i=0;i<(size-size_child);i++)
{
//循环读取一个字符并且输出一个字符
size_t b=read(fp,buf,1);
write(fd,buf,1);
bzero(buf,0);
if(-1==b)
{
perror("read");
return -1;
}
}
printf("父进程中的前半部分复制完毕\n");
}
//关闭父子进程的两个文件
close(fp);
close(fd);
return 0;
}
实现效果:
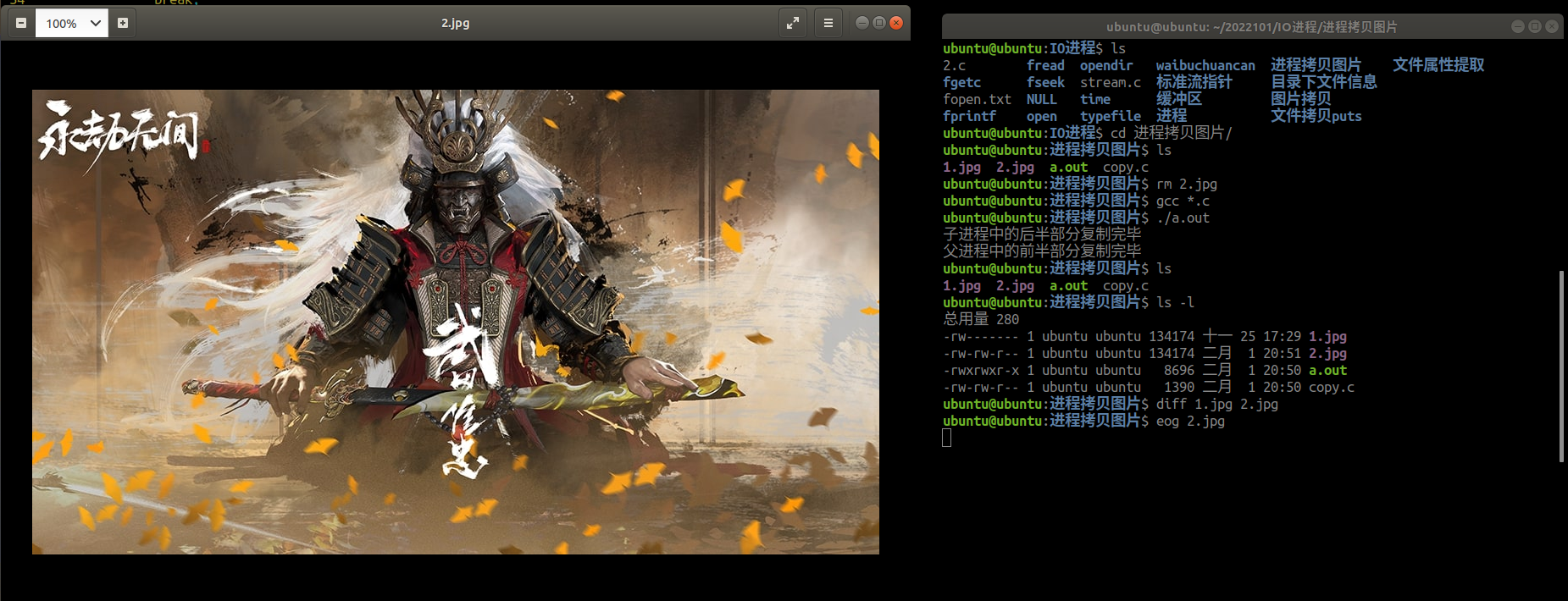