Given a triangle ABC, the Extriangles of ABC are constructed as follows:
On each side of ABC, construct a square (ABDE, BCHJ and ACFG in the figure below).
Connect adjacent square corners to form the three Extriangles (AGD, BEJ and CFH in the figure).
The Exomedians of ABC are the medians of the Extriangles, which pass through vertices of the original triangle,extended into the original triangle (LAO, MBO and NCO in the figure. As the figure indicates, the three Exomedians intersect at a common point called the Exocenter (point O in the figure).
This problem is to write a program to compute the Exocenters of triangles.
On each side of ABC, construct a square (ABDE, BCHJ and ACFG in the figure below).
Connect adjacent square corners to form the three Extriangles (AGD, BEJ and CFH in the figure).
The Exomedians of ABC are the medians of the Extriangles, which pass through vertices of the original triangle,extended into the original triangle (LAO, MBO and NCO in the figure. As the figure indicates, the three Exomedians intersect at a common point called the Exocenter (point O in the figure).
This problem is to write a program to compute the Exocenters of triangles.
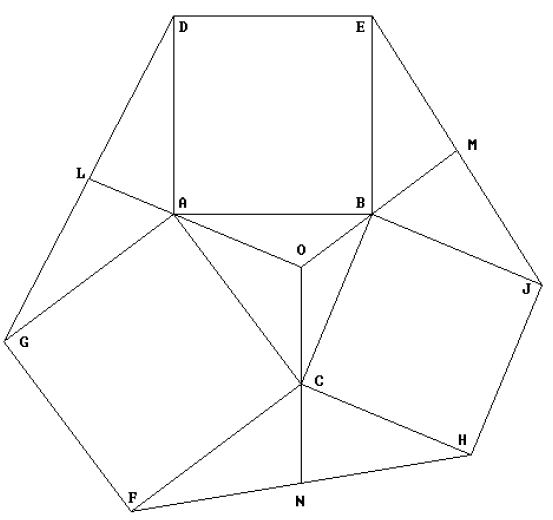
Input
The first line of the input consists of a positive integer n, which is the number of datasets that follow. Each dataset consists of 3 lines; each line contains two floating point values which represent the (two -dimensional) coordinate of one vertex of a triangle. So, there are total of (n*3) + 1 lines of input. Note: All input triangles wi ll be strongly non-degenerate in that no vertex will be within one unit of the line through the other two vertices.
Output
For each dataset you must print out the coordinates of the Exocenter of the input triangle correct to four decimal places.
Sample Input
2 0.0 0.0 9.0 12.0 14.0 0.0 3.0 4.0 13.0 19.0 2.0 -10.0
Sample Output
9.0000 3.7500 -48.0400 23.3600
求的是垂心 直接套公式
但是不知道为什么 大神说答案要加上1e-8才能过 以后几何题都要加1e-8再输出
#include <stdio.h>
int main()
{
int cas;
scanf("%d",&cas);
double x1,y1,x2,y2,x3,y3,x,y;
while(cas--)
{
scanf("%lf %lf %lf %lf %lf %lf",&x1,&y1,&x2,&y2,&x3,&y3);
printf("%.4lf ",1e-8 + -( (x1 *x2* y1) - (x1* x3* y1) - (x1* x2* y2) + (x2* x3* y2) + (y1*y1* y2) - (y1* y2*y2) + (x1* x3* y3) - (x2* x3* y3) - (y1*y1* y3) + (y2*y2* y3) + (y1 *y3*y3) - (y2* y3*y3) )/( (-x2* y1) + (x3 *y1) + (x1 *y2) - (x3* y2) - (x1* y3) + (x2 *y3)) );
printf("%.4lf\n",1e-8 + -( (x1*x1* x2) - (x1* x2*x2) - (x1*x1* x3) + (x2*x2* x3) + (x1* x3*x3) - (x2* x3*x3) + (x1* y1 *y2 )- (x2* y1* y2) - (x1* y1* y3) + (x3* y1* y3) + (x2* y2* y3) - (x3* y2* y3) )/( (x2* y1) - (x3* y1) - (x1* y2) + (x3 *y2) + (x1* y3) - (x2* y3)));
}
return 0;
}