import cv2
img = cv2.imread(“./images/8.jpg”,cv2.IMREAD_GRAYSCALE)
cv2.imshow(“res”,img)
dst1 = cv2.equalizeHist(img)
cv2.imshow(“dst1”,dst1)
clahe = cv2.createCLAHE(clipLimit=2.0,tileGridSize=(8,8))
dst2 = clahe.apply(img)
cv2.imshow(“dst2”,dst2)
cv2.waitKey(0)
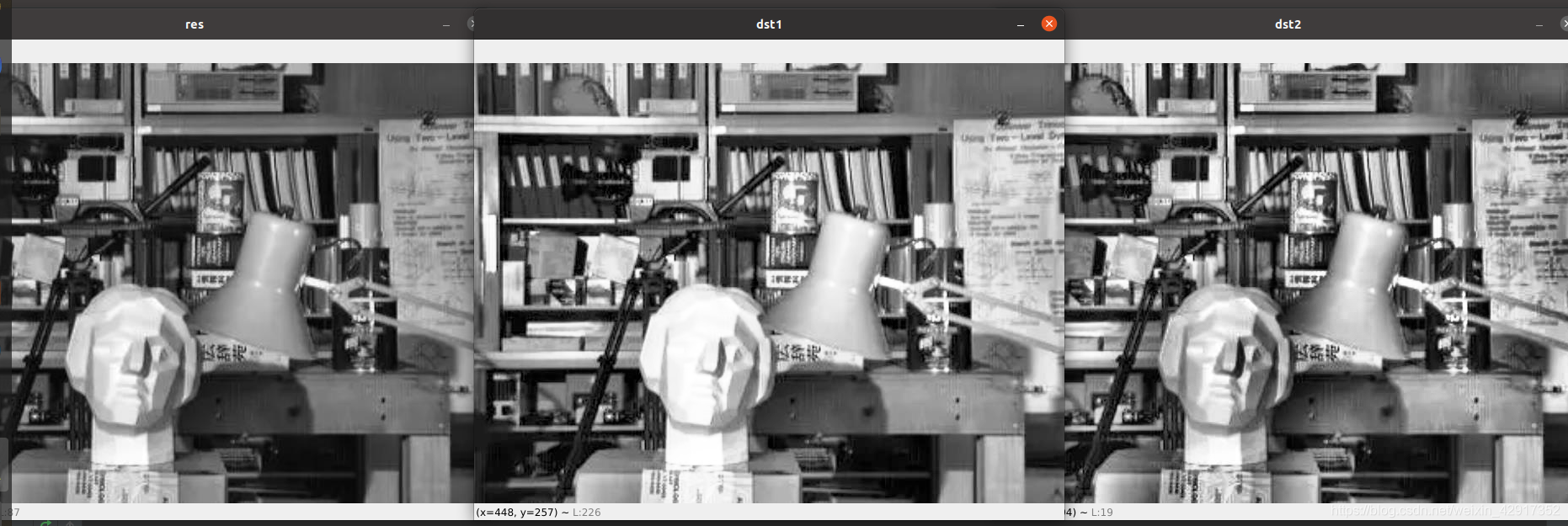
### 直方图均值化
* 去雾好用
import cv2
import matplotlib.pyplot as plt
img = cv2.imread(‘7.jpg’, 0)
cv2.imshow(“src”, img)
cv2.waitKey(0)
his = cv2.calcHist([img], [0], None, [255], [0, 255])
plt.plot(his, label=‘his’, color=‘r’)
plt.show()
dst = cv2.equalizeHist(img)
cv2.imshow(“dst”, dst)
cv2.waitKey(0)
cv2.imwrite(“15.jpg”, dst)
his = cv2.calcHist([dst], [0], None, [255], [0, 255])
plt.plot(his, label=‘his’, color=‘b’)
plt.show()
* 均值化前
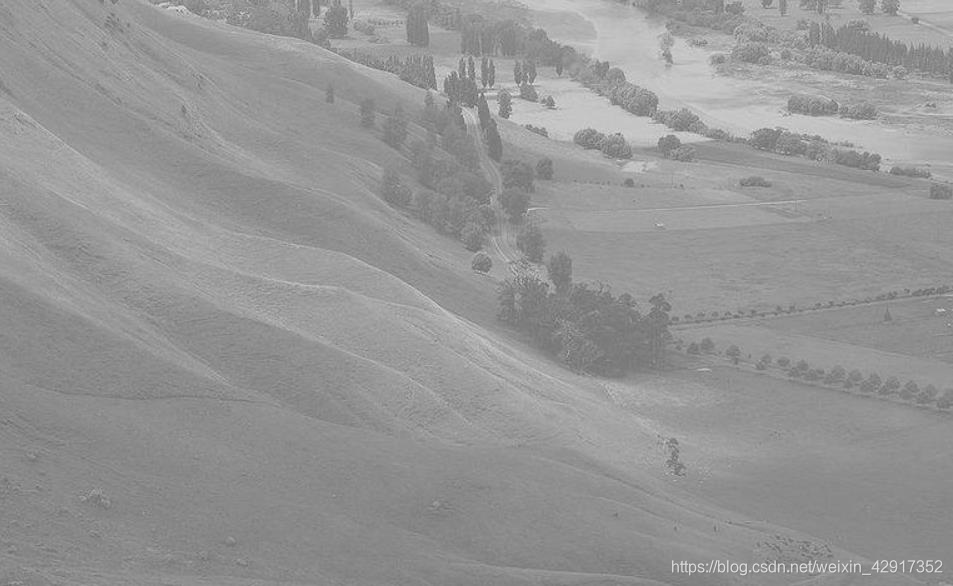
* 均值化后图
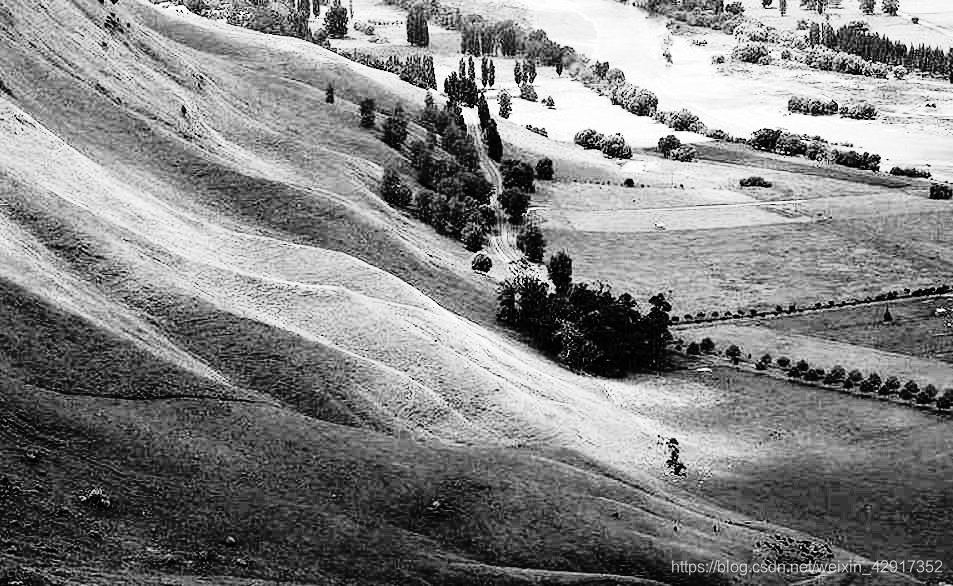
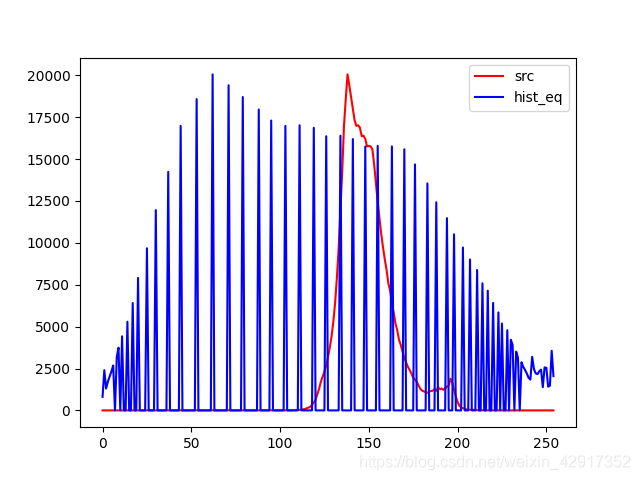
### 直方图计算
-*- coding: utf-8 -*-
“”"
@Time : 2021/7/23 上午10:17
@Auth : 陈伟峰
@File :hist.py
@phone: 15882085601
@IDE :PyCharm
@Motto:ABC(Always Be Coding)
“”"
import cv2
import matplotlib.pyplot as plt
img = cv2.imread(‘./images/1.jpg’)
img[…,0]=0
img[…,1]=0
cv2.imshow(“…”,img)
img_B = cv2.calcHist([img], [0], None, [256], [0, 256])
plt.plot(img_B, label=‘B’, color=‘b’)
img_G = cv2.calcHist([img], [1], None, [256], [0, 256])
plt.plot(img_G, label=‘G’, color=‘g’)
img_R = cv2.calcHist([img], [2], None, [256], [0, 256])
plt.plot(img_R, label=‘R’, color=‘r’)
plt.show()
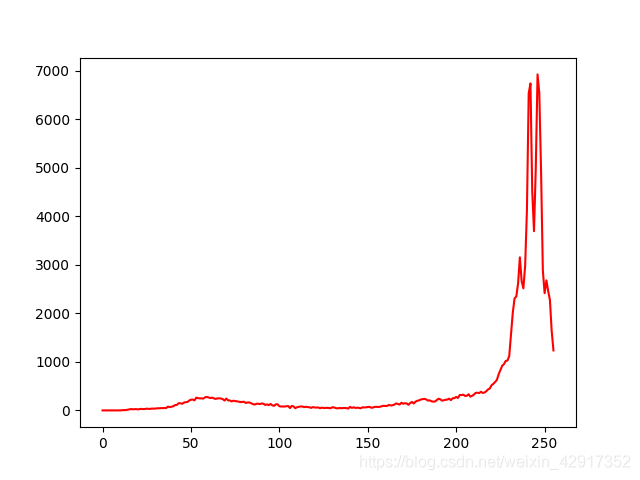
### 直方图反投影
import cv2
import numpy as np
roi = cv2.imread(‘10.jpg’)
hsv = cv2.cvtColor(roi, cv2.COLOR_BGR2HSV)
target = cv2.imread(‘9.jpg’)
hsvt = cv2.cvtColor(target, cv2.COLOR_BGR2HSV)
roihist = cv2.calcHist([hsv], [0, 1], None, [180, 256], [0, 180, 0, 256])
cv2.normalize(roihist, roihist, 0, 255, cv2.NORM_MINMAX)
dst = cv2.calcBackProject([hsvt], [0, 1], roihist, [0, 180, 0, 256], 1)
disc = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (5, 5))
dst = cv2.filter2D(dst, -1, disc)
ret, thresh = cv2.threshold(dst, 50, 255, 0)
thresh = cv2.merge((thresh, thresh, thresh))
res = cv2.bitwise_and(target, thresh)
res = np.hstack((target, thresh, res))
cv2.imshow(‘img’, res)
cv2.waitKey(0)