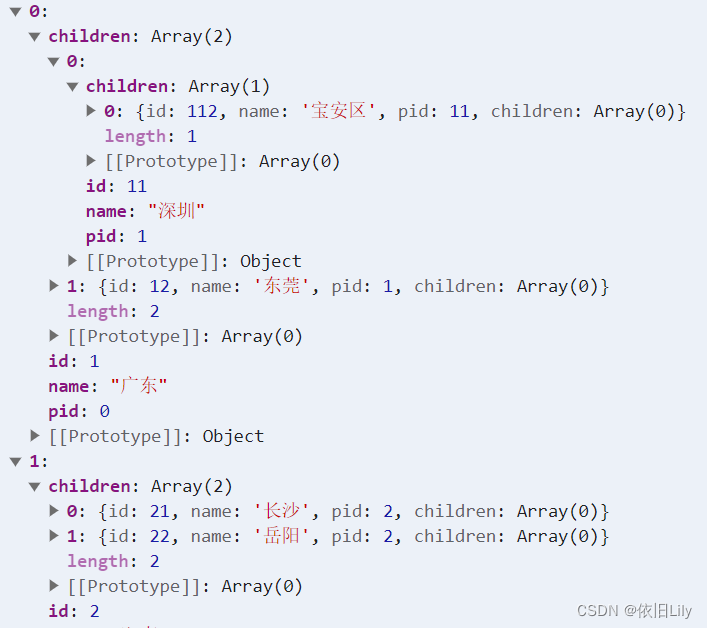
方法一:利用递归,类似于数据结构中的树形结构
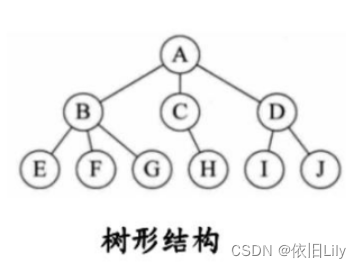
核心思想:从根节点开始找子节点,一条路径找完,再返回上一级,遍历完所有数组即结束。
学习建议:递归本身我觉得有点难以理解,所以我建议学习递归的时候,在代码中打个断点,观察每一步是怎么走的,每一步的数据是什么。
// 递归有很多种解决办法
// 该问题我总结了两种递归代码
// 第一种递归代码:
const arrayToTree = (arr, pid) => {
let result = []
// debugger
handleArr(arr, result, pid)
return result
}
const handleArr = (arr, result, pid) => {
arr.forEach(item => {
if(item.pid === pid) {
const newObj = {...item, children: []}
result.push(newObj)
// console.log(result)
// debugger
handleArr(arr, newObj.children, item.id)
}
})
}
console.log(arrayToTree(arr, 0))
// 第二种递归代码:用forEach
const arrayToTree = (arr, pid) => {
// 装节点的数组
const result = []
// debugger
// 遍历数组找到这一级的节点
arr.forEach(item => {
if (item.pid === pid) {
result.push(item)
// 找下一级,就是再调用这个函数并传入当前id作为pid
item.children = arrayToTree(arr, item.id)
}
})
return result
}
console.log(arrayToTree(arr, 0))
// 第三种递归:用filter,代码最短
function array2Tree(arr, pid) {
return arr.filter(v => {
if (v.pid === pid) {
const children = array2Tree(arr, v.id)
v.children = children
return true
}
})
}
方法二:利用循环,这个循环是有几层循环才能找到几级。
// 该数组有三级,所以我写了三层循环
// 方法一:用forEach方法
const result = []
// 进行遍历
arr.forEach( item1 => {
// 找到1级
if (item1.pid === 0) {
result.push(item1)
// 每个1级还要继续找他的2级(找children)
const children1 = []
arr.forEach( item2 => {
if (item2.pid === item1.id) {
children1.push(item2)
// 把找到的二级数组放到1级这个对象children属性里
item1.children = children1
// 每个2级还要继续找他的3级
const children2 = []
arr.forEach(item3 => {
if(item3.pid === item2.id) {
children2.push(item3)
// 把找到的三级数组放到2级这个对象children属性里
item2.children = children2
}
})
}
})
}
})
console.log(result)
// 方法二:用filter方法
const result = arr.filter(v => {
if (v.pid === '') {
// 找到一级
// 在一级里面找二级
const children = arr.filter(v2 => {
// 找二级
return v2.pid === v.id
})
v.children = children
return true
}
})
console.log(result)
如果有错误,欢迎指正。