第一题: 数字统计(数学+模拟)
题⽬链接:https://www.nowcoder.com/practice/179d9754eeaf48a1b9a49dc1d438525a?tpId=290&tqId=39941&ru=/exam/oj
题目描述: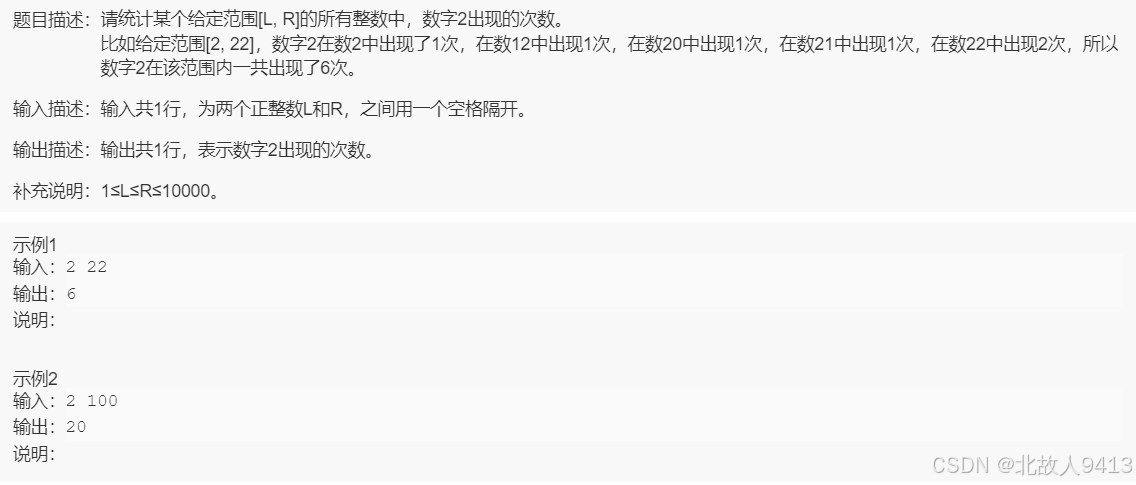
第一次解题
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int n1 = in.nextInt();
int n2 = in.nextInt();
// 遍历
if(n1 % 2 != 0) n1++;
int count = 0;
for(int i = n1; i <= n2; i++) {
count += fun(i);
}
System.out.println(count);
}
private static int fun(int num) {
int ret = 0;
while(num > 0) {
if(num % 10 == 2) {
ret++;
}
num /= 10;
}
return ret;
}
}
第二次解题
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int l = in.nextInt(), r = in.nextInt();
int ret = 0;
for(int i = l; i <= r; i++) {
int tmp = i;
while(tmp > 0) {
if(tmp % 10 == 2) ret++;
tmp /= 10;
}
}
System.out.println(ret);
}
}
总结:
算法思路: 常规操作: 循环提取末尾,然后⼲掉末尾~
第一次解题将判断进行了单独封装,也有点想得复杂了, 就没有第二次解题这么简洁,明了,也没有次二次解题快.
第二题: 两个数组的交集(哈希)
题⽬链接:两个数组的交集_牛客题霸_牛客网
题目描述
第一次解题
public class Solution {
public ArrayList<Integer> intersection1 (ArrayList<Integer> nums1, ArrayList<Integer> nums2) {
// write code here
Set<Integer> set = new HashSet<>();
Set<Integer> count = new HashSet<>();
ArrayList<Integer> ret = new ArrayList<>();
for(int num : nums1) {
set.add(num);
}
for(int num : nums2) {
if(set.contains(num) && !count.contains(num)) {
ret.add(num);
count.add(num);
}
}
return ret;
}
}
第二次解题
import java.util.*;
public class Solution {
public ArrayList<Integer> intersection (ArrayList<Integer> nums1, ArrayList<Integer> nums2) {
boolean[] hash = new boolean[1010];
ArrayList<Integer> ret = new ArrayList<>();
for(Integer n : nums1) {
hash[n] = true; // 存储进hash中
}
for(Integer n : nums2) {
if(hash[n] == true) { // 判断是否在hash中存在
ret.add(n);
hash[n] = false; // 从hash表中删除
}
}
return ret;
}
}
总结:
解题思路
a. 将其中⼀个数组丢进哈希表中;
b. 遍历另⼀个数组的时候,在哈希表中看看就好了。
总的来说,解题时的思路都是差不多, 第一次解题也成功了,但是使用了集合类hash表,但是第二次使用了一个boolean数组来模拟实现hash表,第二次解题耗时更少,代码也更简洁,明了
第三题: 点击消除(栈)
题⽬链接:点击消除_牛客题霸_牛客网
题⽬描述:
题解
目录
// import java.util.*;
import java.util.*;
class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String str = in.nextLine();
StringBuilder stack = new StringBuilder(); // 模拟栈
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (stack.length() != 0 &&
stack.charAt(stack.length() - 1) == ch) { // 判断栈是否为空
stack.deleteCharAt(stack.length() - 1);
} else {
stack.append(ch);
}
}
System.out.println(stack.length() == 0 ? 0 : stack);
}
}
总结:
解题思路: ⽤栈来模拟消除的过程
使用StringBuilder来模拟实现栈.