一.所有文件一览
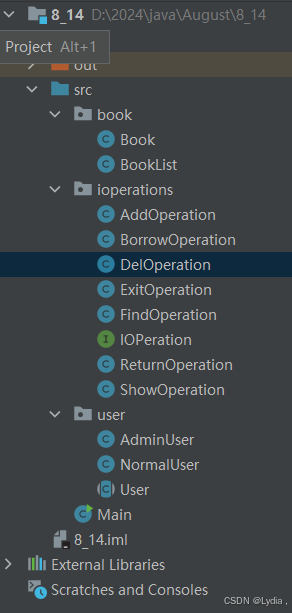
二.代码实现
1.book
1.1 Book.java
1.1.1快捷键
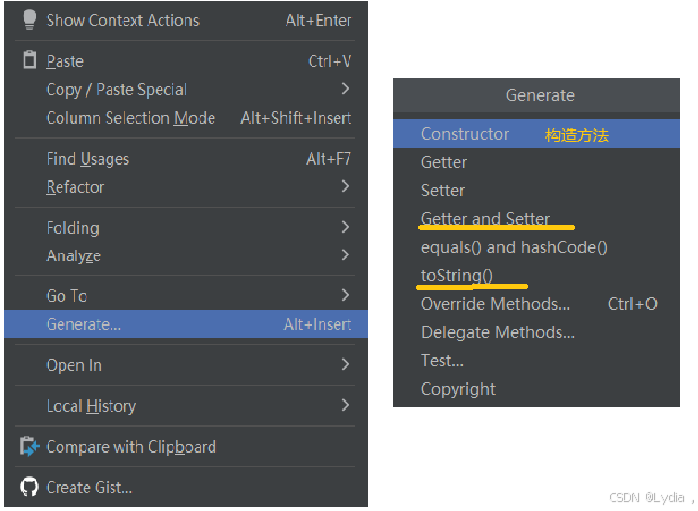
package book;
public class Book {
//全部使用private进行封装
private String name;
private String author;
private int price;
private String type;
private boolean isBorrowed; //默认是false,不用设置构造方法
//创建构造方法
public Book(String name, String author, int price, String type) {
this.name = name;
this.author = author;
this.price = price;
this.type = type;
//isBorrowed默认是false,不用设置构造方法
}
//使用get,set方法读写成员变量
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public void setBorrowed(boolean borrowed) {
isBorrowed = borrowed;
}
public boolean isBorrowed() {
return isBorrowed;
}
//重写toString,输出
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
", type='" + type + '\'' +"," +
((isBorrowed == true) ? "已借出" : "未借出") +
//", isBorrowed=" + isBorrowed +
'}';
}
}
1.1.2代码
1.2 BookList.java
package book;
public class BookList {
private Book[] books = new Book[10]; //书架容量
private int usedSize; //有效的数据个数(实际放书的个数)
public BookList() {
books[0] = new Book("三国演义","罗贯中", 10, "小说");
books[1] = new Book("西游记","吴承恩", 17, "小说");
books[2] = new Book("红楼梦","罗贯中", 13, "小说");
this.usedSize = 3;
}
public int getUsedSize() {
return usedSize;
}
public void setUsedSize(int usedSize) {
this.usedSize = usedSize;
}
public Book getBooks(int pos) {
return books[pos];
}
public void setBooks(int pos,Book book) {
this.books[pos] = book;
}
public Book[] getBooks() {
return books;
}
}
2.ioperations
2.1AddOperation.java
package ioperations;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class AddOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("新增图书:");
//判满
int currentSize = bookList.getUsedSize();
if(currentSize == bookList.getBooks().length) {
System.out.println("书架满了, 不能放了...");
return;
}
//输入
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书名");
String name = scanner.nextLine();
System.out.println("请输入作者");
String author = scanner.nextLine();
System.out.println("请输入书的类型");
String type = scanner.nextLine();
System.out.println("请输入价格");
int price = scanner.nextInt();
Book newbook = new Book(name, author, price, type);
//判断是否重复
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBooks(i);
if (book.getName().equals(name)) {
System.out.println("有这本书!不能插入!");
System.out.println(book);
return;
}
}
//插入这本书
bookList.setBooks(currentSize,newbook);
bookList.setUsedSize(currentSize+1);
System.out.println("新增图书成功");
}
}
2.2BorrowOperation.java
package ioperations;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class BorrowOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("借阅图书:");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书名");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBooks(i);
if (book.isBorrowed()) {
System.out.println("这本书已经被借出了");
return;
}
if (book.getName().equals(name)) {
book.setBorrowed(true);
System.out.println("借阅成功");
return;
}
}
}
}
2.3DelOperation.java
package ioperations;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class DelOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("删除图书:");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要删除的书名");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
int pos = -1;
int i = 0;
for (; i < currentSize; i++) {
Book book = bookList.getBooks(i);
if (book.getName().equals(name)) {
pos = i;
break;
}
}
if(i == currentSize) {
System.out.println("没有你要删除的书");
return;
}
// 开始删除
for (int j = pos; j < currentSize - 1; j++) { //如果是j < currentSize有可能会越界
Book book = bookList.getBooks(j+1);
bookList.setBooks(j,book);
}
bookList.setBooks(currentSize-1, null);
bookList.setUsedSize(currentSize - 1);
System.out.println("删除成功!");
}
}
2.4ExitOperation.java
package ioperations;
import book.BookList;
public class ExitOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("退出系统:");
System.exit(0);
}
}
2.5FindOperation.java
package ioperations;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class FindOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("查找图书:");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的书名:");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBooks(i);
if (book.getName().equals(name)) {
System.out.println("找到了这本书!");
System.out.println(book);
return;
}
}
System.out.println("没有你要找的这本书");
}
}
2.6IOPeration.java
package ioperations;
import book.BookList;
public interface IOPeration {
public void work(BookList bookList);
}
2.7ReturnOperation.java
package ioperations;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class ReturnOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("归还图书:");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书名");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBooks(i);
if (book.getName().equals(name)) {
if (book.isBorrowed()) {
book.setBorrowed(false);
System.out.println("归还成功!");
return;
}
}
}
System.out.println("没有你要归还的书...");
}
}
2.8ShowOperation.java
package ioperations;
import book.Book;
import book.BookList;
public class ShowOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("显示图书:");
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBooks(i);
System.out.println(book);
}
}
}
3.user
3.1AdminUser.java
import ioperations.*;
import java.util.Scanner;
public class AdminUser extends User {
public AdminUser(String name) {
super(name);
this.ioPerations = new IOPeration[]{
new ExitOperation(), //0下标
new FindOperation(),
new AddOperation(),
new DelOperation(),
new ShowOperation(),
};
}
public int menu() {
System.out.println("欢迎" + this.name + "进入图书系统");
System.out.println("********管理员菜单*********");
System.out.println("1.查找图书");
System.out.println("2.新增图书");
System.out.println("3.删除图书");
System.out.println("4.显示图书");
System.out.println("0.退出系统");
System.out.println("***************************");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的操作:");
int choice = scanner.nextInt();
return choice;
}
}
3.2NormalUser.java
package user;
import ioperations.*;
import java.util.Scanner;
public class NormalUser extends User {
public NormalUser(String name) {
super(name);
this.ioPerations = new IOPeration[]{
new ExitOperation(), //0下标
new FindOperation(),
new BorrowOperation(),
new ReturnOperation(),
};
}
public int menu() {
System.out.println("欢迎" + this.name + "进入图书系统");
System.out.println("********普通用户菜单*********");
System.out.println("1.查找图书");
System.out.println("2.借阅图书");
System.out.println("3.归还图书");
System.out.println("0.退出系统");
System.out.println("***************************");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的操作:");
int choice = scanner.nextInt();
return choice;
}
}
3.3User.java
package user;
import book.BookList;
import ioperations.IOPeration;
public abstract class User { //写成抽象类,专门用于继承
protected String name;
//数组没有初始化
protected IOPeration[] ioPerations;
public User(String name) {
this.name = name;
}
public abstract int menu();
public void doIoperation(int choice, BookList bookList) {
ioPerations[choice].work(bookList);
}
}
4.Main.java
import book.BookList;
import user.AdminUser;
import user.NormalUser;
import user.User;
import java.util.Scanner;
public class Main {
public static User login() { //使用向上转型
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的姓名:");
String name = scanner.nextLine();
System.out.println("请输入你的身份:1.管理员 2.普通用户");
int choice = scanner.nextInt();
if(choice == 1) {
return new AdminUser(name);
}else {
return new NormalUser(name);
}
}
// 面向对象就是对象与对象之间协作完成某件事情
public static void main(String[] args) {
BookList bookList = new BookList();
User user = login();
while(true) {
int choice = user.menu();
user.doIoperation(choice, bookList);
}
}
}
三.运行结果
1.管理员
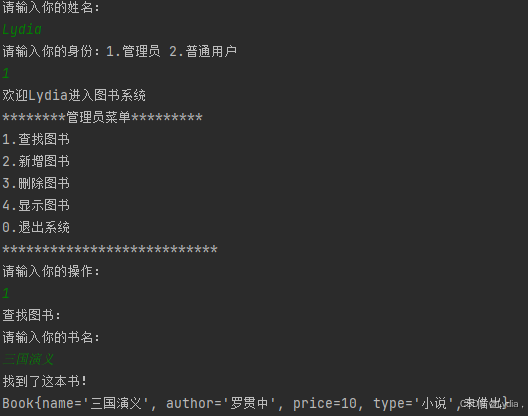
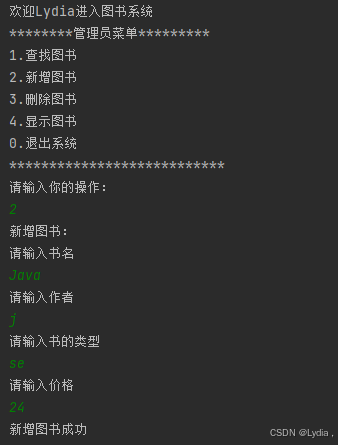
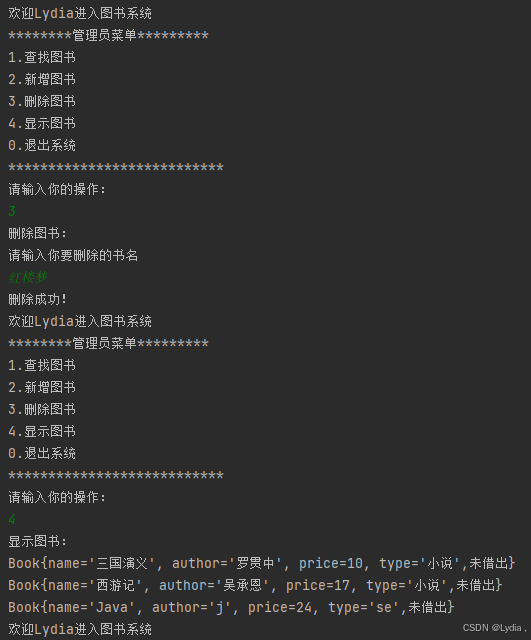
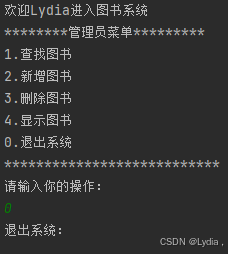
2.普通用户
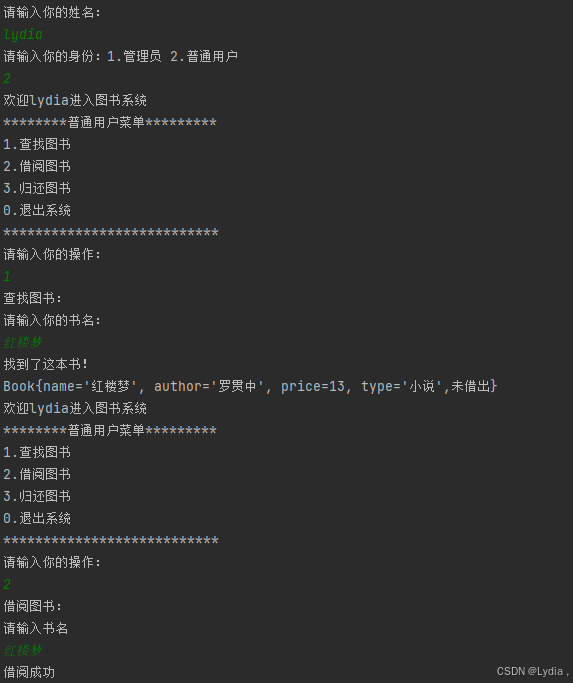
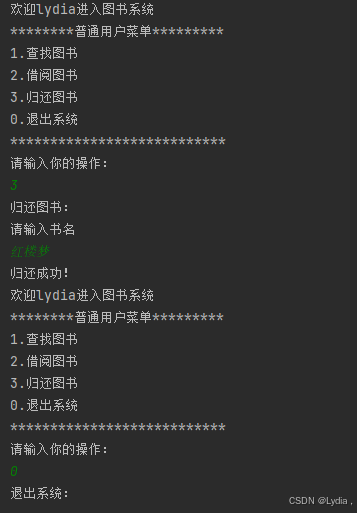