lambda
使用背景
目前大多实际开发中多用 lambda 表达式,越来越少用 if else ,for循环
1. 函数式接口(使用Lambda表达式前提)
代码样例
// 必须有 @FunctionalInterface 修饰
@FunctionalInterface
interface Test {
// 有且只有一个无方法体方法哦!!! (接口默认缺省属性)
void test();
}
总结:
@FunctionalInterface 修饰
方法体内必须【有且只有一个】缺省属性的方法
函数式接口一般用于方法的增强,直接作为方法的参数,实现【插件式编程】
2. lambda 表达式
1. 基本格式:
(自定义参数临时变量)-> {执行代码块}
lambda表达式只注重 【参数】和【返回值】
2. 细节展示
执行代码块为实现接口内缺省属性的方法
在只有一个参数时,小括号可以省略,在方法只有一步时,可以省略大括号
3. 使用lambda图例展示
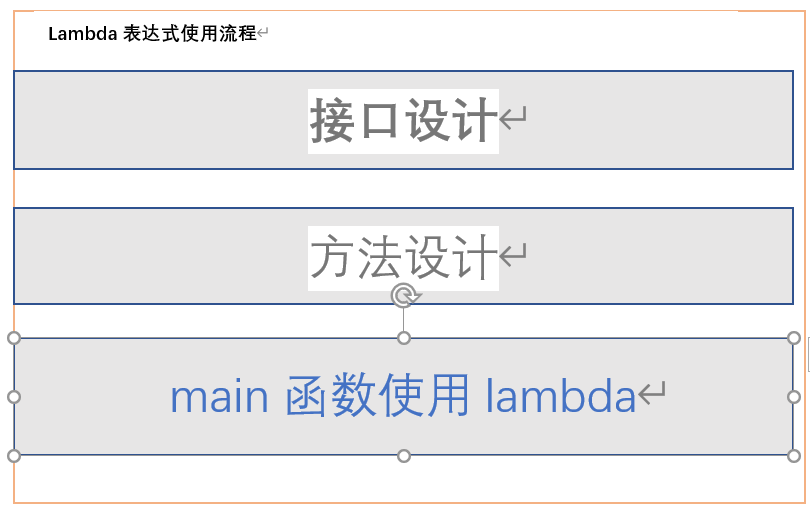
2.1 无参数无返回值(最没用,只起到存在的作用)
代码实现
// 接口设计
@FunctionalInterface
interface A {
// 这里中文方法名只是为了突出他没用 开发中不使用中文
void 方法名真的没有用();
}
public class Demo01{
// main 函数使用lambda
public static void main(String[] args) {
// 基础格式展示
testLambda(() -> {
System.out.println("Lambda 表达式初体验");
});
// 【注意】Lambda 表达式有且只有一行代码,可以省略大括号
testLambda(() -> System.out.println("Lambda 表达式初体验"));
}
// 方法设计
public static void testLambda(A a) {
a.方法名真的没有用();
}
}
2.2有参数无返回值
只拿不给:消费者
接口设计
Consumer 消费者接口,数据最终处理接口
代码实现
// 接口设计
/*
在定义方法遵从该接口时,约束泛型(约束模式)
*/
@FunctionalInterface
public interface Consumer<T> {
// 有参无返回值,典型消费者
void accept(T t);
}
public class Demo02 {
// main 函数使用lambda
public static void main(String[] args) {
// 这里直接使用简单模式
testLambda("lambda表达式需要联想!!!", s ->
System.out.println(Arrays.toString(s.toCharArray()));
);
}
// 方法设计
public static void testLambda(String str, Consumer<String> handle) {
/*
需要方法外部提供针对于当前 String 字符串的处理能力。
处理能力通过 Consumer 接口传入
*/
handle.accept(str);
}
}
2.3 无参数有返回值
不拿只给
生产者接口
代码实现
// 接口设计
@FunctionalInterface
interface Supplier<T> {
T get();
}
public class Demo03 {
// main 函数使用lambda
public static void main(String[] args) {
//基础格式
String s1 = testLambda(() -> {
return "这里也是一个字符串";
});
System.out.println(s1);
// -> 之后只有一代码, return 就可以省略
String s2 = testLambda(() -> "这里也是一个字符串");
System.out.println(s2);
}
// 方法设计
public static String testLambda(Supplier<String> s) {
return s.get();
}
}
2.4 有参数有返回值(比较常用)
2.4.1过滤器接口
代码实现
// 接口实现
// 过滤器接口,判断器接口,条件接口
@FunctionalInterface
interface Predicate<T> {
/**
* 过滤器接口约束的方法,方法参数是用户使用时约束泛型对应具体数据参数
* 返回值类型是 boolean 类型,用于条件判断,数据过来
*
* @param t 用户约束泛型对应的具体数据类型参数
* @return boolean 数据,判断结果反馈
*/
boolean test(T t);
}
public class Demo04 {
// main 函数使用lambda
public static void main(String[] args) {
/*
定义了一个Person类,这里进行实例化,定义5个Person类对象
*/
Person[] array = new Person[5];
// 设计一个循环,随机获得年龄
for (int i = 0; i < array.length; i++) {
int age = (int) (Math.random() * 50);
array[i] = new Person(i + 1, "张三", age, false);
}
// 最简模式,省略() 和 {} 还有return
/*
这里执行完 lambda 方法默认返回 boolean 类型
*/
Person[] temp = filterPersonArrayUsingPredicate(array, p -> p.getAge() > 10);
// 结果展示
for (Person person : temp) {
System.out.println(person);
}
}
// 方法设计
public static Person[] filterPersonArrayUsingPredicate(Person[] array, Predicate<Person> filter) {
Person[] temp = new Person[array.length];
int count = 0;
for (int i = 0; i < array.length; i++) {
/*
Predicate 接口提供的方法是 boolean test(T t);
目前泛型约束之后是 boolean test(Person t);
判断当前 Person 对象是否满足要求,如果满足,存储到 temp 数组中。
*/
if (filter.test(array[i])) {
temp[count++] = array[i];
}
}
return temp;
}
}
2.4.2 类型转换器接口
代码实现
//接口设计
// 类型转换器接口
@FunctionalInterface
interface Function<T, R> {
R apply(T t);
}
public class Demo05 {
// main 函数使用lamda
public static void main(String[] args) {
// 最简lambda 格式 ,return 返回的是 int 类型
int i = testLambda(str, s -> s.length());
System.out.println(i);
}
// 方法设计
public static int testLambda(String str, Function<String, Integer> fun) {
return fun.apply(str);
}
}
2.4.3 比较器接口
代码实现
// 接口设计
@FunctionalInterface
interface Comparator<T> {
/**
* 比较器接口要求的方法,参数是泛型参数,用户指定类型
*
* @param o1 用户在使用接口时约束的泛型对应具体数据类型参数
* @param o2 用户在使用接口时约束的泛型对应具体数据类型参数
* @return 返回值为 int 类型,0 表示两个元素一致。
*/
int compare(T o1, T o2);
}
public class Demo06 {
public static void main(String[] args) {
Person[] array = new Person[5];
for (int i = 0; i < array.length; i++) {
int age = (int) (Math.random() * 50);
array[i] = new Person(i + 1, "张三", age, false);
}
// 基础格式,这里为两个参数,所以带(),但方法体只有一行,省略 {}和 return
sortPersonArrayUsingComparator(array, (o1, o2) -> o1.getId() - o2.getId());
// 结果展示
for (Person person : array) {
System.out.println(person);
}
}
// 方法设计
public static void sortPersonArrayUsingComparator(Person[] array, Comparator<Person> condition) {
for (int i = 0; i < array.length - 1; i++) {
int index = i;
for (int j = i + 1; j < array.length; j++) {
/*
condition 是自定义 Comparator 排序接口方法,目前泛型约束为 Person 类型
int compare(T o1, T o2); ==> int compare(Person o1, Person o2);
当前数组存储的数据类型就是 Person 对象,可以作为 compare 方法参数,同时
利用 compare 方法返回 int 类型数据,作为排序算法规则的限制。
*/
if (condition.compare(array[index], array[j]) > 0) {
index = j;
}
}
if (index != i) {
Person temp = array[index];
array[index] = array[i];
array[i] = temp;
}
}
}
}