1:先弄到图片 上传到服务器上
2.下载 unicode 和 表情 映射关系xml
emoji4unicode.xml 在下面的rar 中
3.加入这个一个 转换类
3.调用 servlet
4.写成一个ELfunction 在jsp EL 表达式中 使用
5;编写 tld文件
6:jsp中使用
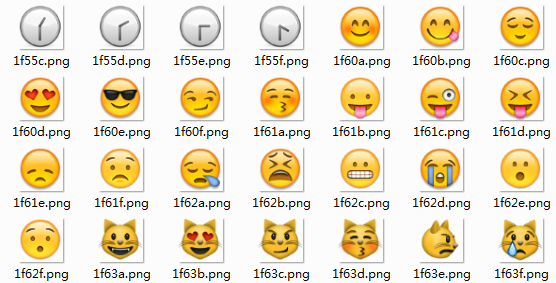
2.下载 unicode 和 表情 映射关系xml
emoji4unicode.xml 在下面的rar 中
3.加入这个一个 转换类
- package com.fanbaobao.util;
- import java.io.InputStream;
- import java.util.ArrayList;
- import java.util.HashMap;
- import java.util.List;
- import java.util.Map;
- import javax.xml.namespace.QName;
- import javax.xml.stream.XMLEventReader;
- import javax.xml.stream.XMLInputFactory;
- import javax.xml.stream.XMLStreamException;
- import javax.xml.stream.events.Attribute;
- import javax.xml.stream.events.StartElement;
- import javax.xml.stream.events.XMLEvent;
- public class EmojiConverter {
- private EmojiConverter() {
- }
- enum Type {
- UNICODE, SOFTBANK
- };
- private Map<List<Integer>, String> convertMap;
- public static class Builder {
- private Type from;
- private Type to;
- public Builder from(Type type) {
- this.from = type;
- return this;
- }
- public Builder to(Type type) {
- this.to = type;
- return this;
- }
- public EmojiConverter build() {
- EmojiConverter converter = new EmojiConverter();
- readMap(converter);
- return converter;
- }
- private static final String TRIM_PATTERN = "[^0-9A-F]*";
- public void readMap(EmojiConverter converter) {
- Map<List<Integer>, String> result = new HashMap<List<Integer>, String>();
- converter.convertMap = result;
- XMLEventReader reader = null;
- try {
- XMLInputFactory factory = XMLInputFactory.newInstance();
- InputStream stream = EmojiConverter.class.getClassLoader()
- .getResourceAsStream("emoji4unicode.xml");
- reader = factory.createXMLEventReader(stream);
- while (reader.hasNext()) {
- XMLEvent event = reader.nextEvent();
- if (event.isStartElement()) {
- StartElement element = (StartElement) event;
- if (element.getName().getLocalPart().equals("e")) {
- Attribute fromAttr = element
- .getAttributeByName(new QName(from.toString().toLowerCase()));
- Attribute toAttr = element
- .getAttributeByName(new QName(to.toString()
- .toLowerCase()));
- if (fromAttr == null) {
- continue;
- }
- List<Integer> fromCodePoints = new ArrayList<Integer>();
- String from = fromAttr.getValue();
- if (from.length() > 6) {
- String[] froms = from.split("\\+");
- for (String part : froms) {
- fromCodePoints.add(Integer.parseInt(
- part.replaceAll(TRIM_PATTERN, ""),
- 16));
- }
- } else {
- fromCodePoints.add(Integer.parseInt(
- from.replaceAll(TRIM_PATTERN, ""), 16));
- }
- if (toAttr == null) {
- result.put(fromCodePoints, null);
- } else {
- String to = toAttr.getValue();
- StringBuilder toBuilder = new StringBuilder();
- if (to.length() > 6) {
- String[] tos = to.split("\\+");
- for (String part : tos) {
- toBuilder.append(Character
- .toChars(Integer.parseInt(part
- .replaceAll(
- TRIM_PATTERN,
- ""), 16)));
- }
- } else {
- toBuilder.append(Character.toChars(Integer
- .parseInt(to.replaceAll(
- TRIM_PATTERN, ""), 16)));
- }
- result.put(fromCodePoints, toBuilder.toString());
- }
- }
- }
- }
- reader.close();
- } catch (Exception e) {
- e.printStackTrace();
- } finally {
- if (reader != null) {
- try {
- reader.close();
- } catch (XMLStreamException e) {
- }
- }
- }
- }
- }
- public String convert(int width,int height,String imgpath,String input) {
- StringBuilder result = new StringBuilder();
- int[]codePoints = toCodePointArray(input);
- for(int i = 0; i < codePoints.length; i++){
- List<Integer> key2 = null;
- if(i + 1 < codePoints.length){
- key2 = new ArrayList<Integer>();
- key2.add(codePoints[i]);
- key2.add(codePoints[i + 1]);
- if(convertMap.containsKey(key2) || 65039==codePoints[i + 1]){ //处理 iphone5 xxxx-fe0f.png
- String aa=Integer.toHexString(codePoints[i])+"-"+Integer.toHexString(codePoints[i+1]);
- String value = convertMap.get(key2);
- if(value != null || 65039==codePoints[i + 1]){
- result.append("<img width=\""+width+"px\" height=\""+height+"px\" style=\"vertical-align: bottom;\" src=\""+imgpath+"");
- result.append(aa);
- result.append(".png\"/>");
- }
- i++;
- continue;
- }
- }
- List<Integer> key1 = new ArrayList<Integer>();
- key1.add(codePoints[i]);
- if(convertMap.containsKey(key1)){
- String aa=Integer.toHexString(codePoints[i]);
- String value = convertMap.get(key1);
- if(value != null){
- result.append("<img width=\""+width+"px\" height=\""+height+"px\" style=\"vertical-align: bottom;\" src=\""+imgpath+"");
- result.append(aa);
- result.append(".png\"/>");
- //System.out.println("key:"+key1);
- //System.out.println("Map:"+value);
- }
- continue;
- }
- if(128529==codePoints[i]){ //处理空格
- result.append(" ");
- continue;
- }
- result.append(Character.toChars(codePoints[i]));
- }
- return result.toString();
- }
- int[] toCodePointArray(String str) {
- char[] ach = str.toCharArray();
- int len = ach.length;
- int[] acp = new int[Character.codePointCount(ach, 0, len)];
- int j = 0;
- for (int i = 0, cp; i < len; i += Character.charCount(cp)) {
- cp = Character.codePointAt(ach, i);
- acp[j++] = cp;
- }
- return acp;
- }
- }
3.调用 servlet
- public class ShareServlet extends HttpServlet {
- private static EmojiConverter converter;
- private static String imgpath="http://xx.xxxx.com/opt/siteimg/mika/emoji/unicode/";
- @Override
- public void init() throws ServletException {
- converter = new EmojiConverter.Builder()
- .from(Type.UNICODE)
- .to(Type.SOFTBANK)
- .build();
- }
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- FbbServiceClient service=null;
- try {
- String userid=request.getParameter("userid");
- String bid=request.getParameter("bid");
- if(userid!=null && bid!=null && userid.length()>0 && bid.length()>0){
- service=new FbbServiceClient();
- SnapService.Client client=service.open();
- SnapItem item=client.getItemByIdS(Long.valueOf(userid),Long.valueOf(bid));
- //进行转换
- if(item.getUserName()!=null && item.getUserName().length()>0)
- item.setUserName(converter.convert(20,20,imgpath,item.getUserName()));
- if(item.getItemName()!=null && item.getItemName().length()>0)
- item.setItemName(converter.convert(20,20,imgpath,item.getItemName()));
- request.setAttribute("SnapItem", item);
- request.getRequestDispatcher("/wxshare.jsp").forward(request, response);
- }
- } catch (Exception e) {
- e.printStackTrace();
- logger.error("errorcode ::: " + e.getMessage(), e);
- }finally{
- if(service!=null)
- service.close();
- }
- }
- }
4.写成一个ELfunction 在jsp EL 表达式中 使用
- package com.fanbaobao.util;
- import com.fanbaobao.util.EmojiConverter.Type;
- public class EmojiFunction {
- private static EmojiConverter converter;
- private static String imgpath="http://xx.xxxx.com/opt/siteimg/mika/emoji/unicode/";
- static{
- converter = new EmojiConverter.Builder()
- .from(Type.UNICODE)
- .to(Type.SOFTBANK)
- .build();
- }
- public static String emoji(String arg){
- return emojiFun(null,null,arg);
- }
- public static String emojiFun(Integer width,Integer height,String arg){
- if(width==null){
- width=20;
- }
- if(height==null){
- height=20;
- }
- return converter.convert(width.intValue(),height.intValue(),imgpath,arg);
- }
- }
5;编写 tld文件
- <?xml version="1.0" encoding="UTF-8"?>
- <taglib version="2.0" xmlns="http://java.sun.com/xml/ns/j2ee"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee web-jsptaglibrary_2_0.xsd">
- <tlib-version>1.0</tlib-version>
- <short-name>mk</short-name>
- <uri>/mikadata-tags</uri>
- <function>
- <name>emoji</name>
- <function-class>com.fanbaobao.util.EmojiFunction</function-class>
- <function-signature>
- java.lang.String emoji(java.lang.String)
- </function-signature>
- </function>
- <function>
- <name>emojiFun</name>
- <function-class>com.fanbaobao.util.EmojiFunction</function-class>
- <function-signature>
- java.lang.String emojiFun(java.lang.Integer,java.lang.Integer,java.lang.String)
- </function-signature>
- </function>
- </taglib>
6:jsp中使用
- <%@ taglib uri="/mikadata-tags" prefix="mk"%>
- <td>${mk:emoji(dataObject.comment)}</td>