Ref:
https://blog.youkuaiyun.com/w_j_f_/article/details/82717894
https://isocpp.github.io/CppCoreGuidelines/CppCoreGuidelines
https://www.cnblogs.com/qionglouyuyu/p/4620714.html(以智能指针为例解释深浅拷贝,string类深浅拷贝比较,shared_ptr简单实现)
重点知识点例题:https://blog.youkuaiyun.com/caoshangpa/article/details/51530482(string类实现!拷贝构造深拷贝实现)
智能指针:(https://blog.youkuaiyun.com/caoshangpa/article/details/79221544)
因此,我们使用智能指针的原因至少有以下三点:
1)智能指针能够帮助我们处理资源泄露问题;
2)它也能够帮我们处理空悬指针的问题;
3)它还能够帮我们处理比较隐晦的由异常造成的资源泄露。
深浅拷贝区别:
浅拷贝(shallow copy)与深拷贝(deep copy)对于值拷贝的处理相同,都是创建新对象,但对于引用拷贝的处理不同,深拷贝将会重新创建新对象,返回新对象的引用字。浅拷贝不会创建新引用类型:
浅拷贝只拷贝指针,但拷贝后两个指针指向同一个内存空间;
深拷贝不但对指针进行拷贝,而且对指针指向的内容进行拷贝,
调用拷贝构造函数后,浅拷贝还有联系,深拷贝的两个对象完全独立。
浅拷贝类似于文件创建快捷方式,而深拷贝好比文件复制。
编译器默认提供的默认拷贝构造函数是浅拷贝,深拷贝的构造函数需自己实现。
判断:
根据类的实现
1。如果它有一个用原生指针指针实现的对象引用,或是用boost::shared_ptr等引用分享所有权的智能指针实现的对象引用,则这个拷贝是浅拷贝
2。如果是用copy_ptr这种实现了深拷贝的智能指针实现的对象引用,就是深拷贝了。copy_ptr在内部保留一个指针,当它自己解析时,它同时也销毁它在内部保存的这个指针。
最能体现深层拷贝与浅层拷贝的,就是‘=’的重载。以此为例。
浅拷贝:
class string
{
char *m_str; //对象之中含有指针数据类型
public:
string(char *s)
{
m_str=s;
}
string(){};
string&operator=(const string s)
{
m_str=s.m_str; //s1,s2指向同一个内存
return *this}
};
int main()
{
string s1("abc"),s2;
s2=s1;
cout<<s2.m_str;
}
};
深拷贝:
string&operator=(const string&s)
{
if(strlen(m_str)!=strlen(s.m_str))
m_str=new char[strlen(s.m_str)+1]; //为被赋值对象申请了一个新的内存
if(*this!=s)
strcmp(m_str,s.m_str);
return *this;
}
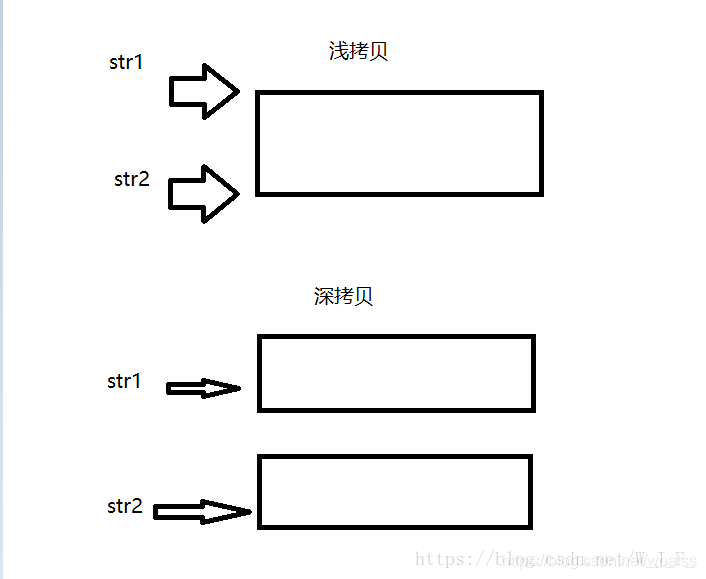
总结:
1.在对含有指针成员的对象进行拷贝时,必须要自己定义拷贝构造函数,使拷贝后的对象指针成员有自己的内存空间,即进行深拷贝,这样就避免了内存泄漏发生。(分析:https://blog.youkuaiyun.com/caoshangpa/article/details/79226270)
2.当函数的参数为对象时,实参传递给形参的实际上是实参的一个拷贝对象,系统自动通过拷贝构造函数实现;
3.当函数的返回值为一个对象时,该对象实际上是函数内对象的一个拷贝,用于返回函数调用处。
4.浅拷贝带来问题的本质在于析构函数释放多次堆内存,使用std::shared_ptr(也是浅拷贝),可以完美解决这个问题。(关于std::shared_ptr的原理和实现可参考:C++笔试题之smart pointer的实现)
注意:浅拷贝多个对象共用一个资源,当一个对象销毁时,资源就会释放。如果对另一个对在进行销毁。会因为资源重复释放造成程序崩溃!
BUG经验:
1.使用拷贝构造函数(浅拷贝)时,用的是默认的,但自定义了构造函数,构造函数中给指针赋值,但默认拷贝构造函数是无参的。当调用构造及拷贝后,再析构会析构两次指向相同地址的指针,导致crash!
#include <iostream>
using namespace std;
class Student
{
private:
int num;
char *name;
public:
Student();
~Student();
};
Student::Student()
{
name = new char(20);
cout << "Student" << endl;
}
Student::~Student()
{
cout << "~Student " << static_cast<const void *>(name) << endl;
//cout << "~Student " << (void *)(name) << endl;
delete name;
name = NULL;
}
int main()
{
{// 花括号让s1和s2变成局部对象,方括号后便析构,方便测试
Student s1;
Student s2(s1);// 复制对象
}
system("pause");
return 0;
}
解决:1.使用std::shared_ptr(依然为浅拷贝)
#include <iostream>
#include <cstring>
#include <memory>
using namespace std;
class Student
{
public:
shared_ptr<char> name;
int num;
Student();
Student(const Student& s);
~Student();
public:
Student& operator=(const Student& s)
{
*name = *s.name;
cout << "assign Student" << endl;
return *this;
}
};
Student::Student(const Student &s):name{s.name}
{
*name = *s.name;
cout << "copy Student from:" << s.num<< endl;
}
Student::Student()
{
allocator<char> aloc;
name = allocate_shared<char>(aloc); //add count
cout << "Student" << endl;
}
Student::~Student()
{
cout << "~Studnet count " <<"addr"<<(void *)name.get()<<" cout:"<<name.use_count()<< endl; //call destructor how much add count!
}
int main()
{
{// 花括号让s1和s2变成局部对象,方括号后便析构,方便测试
Student s1;
cout << "s1 count " <<s1.name.use_count()<< endl;
Student s2(s1);// 复制对象
//Student s2 = s1; //call copy when new a object!!!!even use "="
s2.num = 2;
//s3 is deep copy!first alloc , then just give value!
Student s3;
s3 = s1; //here change value,so call assign function!
cout << "s1 count " <<s1.name.use_count()<< endl;
cout << "s2 count " <<s2.name.use_count()<< endl; //share with s1
cout << "s3 count " <<s3.name.use_count()<< endl; //not share,its new one
}
return 0;
}
结果:
Student
s1 count 1
copy Student from:1
Student
assign Student
s1 count 2
s2 count 2
s3 count 1
~Studnet count addr0x180cc60 cout:1
~Studnet count addr0x180bc30 cout:2
~Studnet count addr0x180bc30 cout:1
2.修改为深拷贝,这样就避免了内存泄漏发生(不过此时,拷贝的指针和原指针不再指向同一内存,也就是改变一个另一个不变)
Student::Student(const Student &s)
{
name = new char(20);
memcpy(name, s.name, strlen(s.name));
cout << "copy Student" << endl;
}
3.使用vector来定义动态数组,尽量不使用new