这里的smart dialogs 一般都是指的非模态对话框,就是用户想要实时的看到自己的操作对主窗口的影响,当然在某些模态对话框中,添加预览功能可以实现上面的需求,但是这些在非模态对话框中是很容易做到的。
我们在前面模态对话框的字体选择程序基础上加以修改。
先写一个类,ModelessDialog.py,如下:
#!/usr/bin/env python
#coding=utf-8
from PyQt4.QtCore import *
from PyQt4.QtGui import *
class FontPropertiesDlg(QDialog):
def __init__(self,format,parent=None):
super(FontPropertiesDlg, self).__init__(parent)
self.format=format
FontStyleLabel = QLabel(u"中文字体:")
self.FontstyleComboBox = QComboBox()
self.FontstyleComboBox.addItems([u"宋体", u"黑体", u"仿宋",
u"隶书", u"楷体"])
self.FontEffectCheckBox =QCheckBox(u"使用特效")
FontSizeLabel = QLabel(u"字体大小")
self.FontSizeSpinBox = QSpinBox()
self.FontSizeSpinBox.setRange(1, 90)
applyButton = QPushButton(u"应用")
cancelButton = QPushButton(u"取消")
buttonLayout = QHBoxLayout()
buttonLayout.addStretch()
buttonLayout.addWidget(applyButton)
buttonLayout.addWidget(cancelButton)
layout = QGridLayout()
layout.addWidget(FontStyleLabel, 0, 0)
layout.addWidget(self.FontstyleComboBox, 0, 1)
layout.addWidget(FontSizeLabel, 1, 0)
layout.addWidget(self.FontSizeSpinBox, 1, 1)
layout.addWidget(self.FontEffectCheckBox,1,2)
layout.addLayout(buttonLayout, 2, 0)
self.setLayout(layout)
self.connect(applyButton,SIGNAL("clicked()"),self.apply) self.connect(cancelButton,SIGNAL("clicked()"),self,SLOT("reject()"))
self.setWindowTitle(u"字体")
def apply(self):
self.format["fontstyle"] = unicode(self.FontstyleComboBox.currentText())
self.format["fontsize"] = self.FontSizeSpinBox.value()
self.format["fonteffect"] = self.FontEffectCheckBox.isChecked()
self.emit(SIGNAL("changed"))
然后我们在上一个模态对话框程序中添加:
Import ModelessDialog
然后添加函数
def FontModalessDialog(self):
dialog = ModelessDialog.FontPropertiesDlg(self.format,self)
self.connect(dialog,SIGNAL("changed"),self.updataData)
dialog.show()
即可。
结果如下:
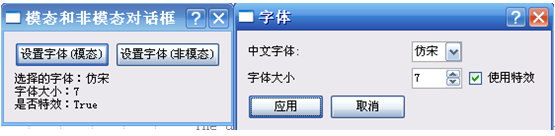
只要我们点击应用,左边主对话框立刻更新用户选择。我们也可以看到程序中两个最重要的地方:
1:self.emit(SIGNAL("changed"))
2:self.connect(dialog,SIGNAL("changed"),self.updataData)
可以看到只要用户点击“应用”,后程序发现填出对话框内容发生改变,则立刻产生消息”changed”,主程序消息循环得到这个消息,立刻运行updataData,所以主窗口更新了内容。
下面我们再看一种比上面非模态对话框更方便快速的方法,就是没有按钮,只要我们改变了对话框的内容,结果就立马显示。我们称这种对话框为”live dialog”。
首先还是先写一个类,单独一个py文件:LiveDialog.py
#!/usr/bin/env python
#coding=utf-8
import sys
from PyQt4.QtCore import *
from PyQt4.QtGui import *
class FontPropertiesDlg(QDialog):
def __init__(self,format,callback,parent=None):
super(FontPropertiesDlg, self).__init__(parent)\
self.format = format
self.callback = callback
FontStyleLabel = QLabel(u"中文字体:")
self.FontstyleComboBox = QComboBox()
self.FontstyleComboBox.addItems([u"宋体", u"黑体", u"仿宋",
u"隶书", u"楷体"])
self.FontEffectCheckBox =QCheckBox(u"使用特效")
FontSizeLabel = QLabel(u"字体大小")
self.FontSizeSpinBox = QSpinBox()
self.FontSizeSpinBox.setRange(1, 90)
layout = QGridLayout()
layout.addWidget(FontStyleLabel, 0, 0)
layout.addWidget(self.FontstyleComboBox, 0, 1)
layout.addWidget(FontSizeLabel, 1, 0)
layout.addWidget(self.FontSizeSpinBox, 1, 1)
layout.addWidget(self.FontEffectCheckBox,1,2)
self.setLayout(layout)
self.connect(self.FontstyleComboBox,SIGNAL("itemSelected"),self.apply)
self.connect(self.FontEffectCheckBox,SIGNAL("toggled(bool)"), self.apply)
self.connect(self.FontSizeSpinBox,SIGNAL("valueChanged(int)"), self.apply)
self.setWindowTitle(u"字体")
def apply(self):
self.format["fontstyle"] = unicode(self.FontstyleComboBox.currentText())
self.format["fontsize"] = self.FontSizeSpinBox.value()
self.format["fonteffect"] = self.FontEffectCheckBox.isChecked()
self.callback()
然后在font.py中添加:
import LiveDialog
在类的init中添加:
self.FontPropertiesDlg=None
然后添加方法:
def FontLiveDialog(self):
if self.FontPropertiesDlg is None:
self.FontPropertiesDlg = LiveDialog.FontPropertiesDlg(self.format,self.updataData,self)
self.FontPropertiesDlg.show()
self.FontPropertiesDlg.raise_()
self.FontPropertiesDlg.activateWindow()
运行就可以实时改变用户的选择了。
http://blog.sina.com.cn/s/blog_4b5039210100gxw8.html