项目中用过代码:
/**
* 添加一张图片,完成分享
*/
public void shareImg(OnekeyShare oks) {
//查找图片,如果有直接分享,如果没有,加到的本地在分享
String SDPATH = Environment.getExternalStorageDirectory() + "/一创资源文件夹/图片资源/logo.png";
String fileDir = Environment.getExternalStorageDirectory() + "/一创资源文件夹/图片资源";
File imgFile = new File(SDPATH);
if (!imgFile.exists()) {
try {
//创建目录
File dir = new File(fileDir);
if (!dir.exists())
dir.mkdir();
//创建
imgFile.createNewFile();
InputStream is = getActivity().getAssets().open("logo.png");
FileOutputStream fos = new FileOutputStream(imgFile);
int len = 0;
byte[] buffer = new byte[1024 * 4];
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.flush();
fos.close();
is.close();
oks.setImagePath(SDPATH);
} catch (IOException e) {
e.printStackTrace();
}
} else {
//直接存储
oks.setImagePath(SDPATH);
}
}
转载:http://www.open-open.com/lib/view/open1358904594286.html
先上一张图片

布局就是两个按钮,id分别为downloadTxt、downloadMp3
Download.java在OnCreate方法里面先添加控件获取,控件上的值获取,事件绑定
1
2
3
4
5
6
7
|
downloadTxtButton = (Button) findViewById(R.id.downloadTxt);
downloadTxtButton.setText(R.string.downloadTxt);
downloadTxtButton.setOnClickListener(
new
DownloadTxtListener());
downloadMp3Button = (Button) findViewById(R.id.downloadMp3);
downloadMp3Button.setText(R.string.downloadMp3);
downloadMp3Button.setOnClickListener(
new
DownloadMp3Listener());
|
再添加下面这一段,新版本中要是没有下面这一段会报
An exception occurred: android.os.NetworkOnMainThreadException
1
2
3
4
5
6
7
|
StrictMode.setThreadPolicy(
new
StrictMode.ThreadPolicy.Builder()
.detectDiskReads().detectDiskWrites().detectNetwork()
// 这里或者用 .detectAll() 方法
.penaltyLog().build());
StrictMode.setVmPolicy(
new
StrictMode.VmPolicy.Builder()
.detectLeakedSqlLiteObjects().detectLeakedClosableObjects()
//探测SQLite数据库操作
.penaltyLog()
//打印logcat
.penaltyDeath().build());
|
下面添加两个类,其中HttpDownloader是自己定义的下载工具类。另外为了方便获取文件,用的是本地的tomcat,http://192.168.68.100:8080/test/aa.lrc",在tomcat根目录下的webapps下的test文件夹下放置两个文件aa.lrc、1.mp3。然后启动tomcat下的bin\startup.bat,这点做过web项目的都懂的。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
class
DownloadTxtListener
implements
OnClickListener{
@Override
public
void
onClick(View v) {
HttpDownloader httpDownloader =
new
HttpDownloader();
String lrc = httpDownloader.download(
"http://192.168.68.100:8080/test/aa.lrc"
);
System.out.println(lrc);
}
}
class
DownloadMp3Listener
implements
OnClickListener{
@Override
public
void
onClick(View v) {
HttpDownloader httpDownloader =
new
HttpDownloader();
int
result = httpDownloader.downFile(
"http://192.168.68.100:8080/test/1.mp3"
,
"voa/"
,
"123.mp3"
);
System.out.println(result);
}
}
|
下面新建一个放置工具类的包utils,包下面是两个文件FileUtils.java、HttpDownloader.java
FileUtils.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
|
public
class
FileUtils {
private
String SDPATH;
public
String getSDPATH(){
return
SDPATH;
}
//构造方法
public
FileUtils(){
//得到当前外部存储设备的目录 /SDCARD/...
SDPATH = Environment.getExternalStorageDirectory() +
"/"
;
}
/**
* 在SD卡上创建文件
*
* @param fileName
* @return
* @throws IOException
*/
public
File createSDFile(String fileName)
throws
IOException{
File file =
new
File(SDPATH + fileName);
file.createNewFile();
return
file;
}
/**
* 在SD卡上创建目录
*
* @param dirName
* @return
*/
public
File createSDDir(String dirName){
File dir =
new
File(SDPATH + dirName);
dir.mkdir();
return
dir;
}
/**
* 判断SD卡上的文件夹是否存在
*
* @param fileName
* @return
*/
public
boolean
isFileExist(String fileName){
File file =
new
File(SDPATH + fileName);
return
file.exists();
}
/**
* 将一个InputStream里面的数据写入到SD卡中
*
* @param path
* @param fileName
* @param input
* @return
*/
public
File writeToSDFromInput(String path,String fileName,InputStream input){
File file =
null
;
OutputStream output =
null
;
try
{
createSDDir(path);
file = createSDFile(path + fileName);
output =
new
FileOutputStream(file);
byte
buffer[] =
new
byte
[
4
*
1024
];
while
((input.read(buffer)) != -
1
){
output.write(buffer);
}
//清缓存,将流中的数据保存到文件中
output.flush();
}
catch
(IOException e) {
e.printStackTrace();
}
finally
{
try
{
output.close();
}
catch
(Exception e) {
e.printStackTrace();
}
}
return
file;
}
}
|
HttpDownload.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
|
public
class
HttpDownloader {
private
URL url =
null
;
private
InputStream inputStream =
null
;
/**
* 根据URL下载文件,前提是这个文件当中的内容是文本,函数的返回值就是文件当中的内容
* 1.创建一个URL对象
* 2.通过URL对象,创建一个HttpURLConnection对象
* 3.得到InputStream
* 4.从InputStream当中读取数据
*
* @param urlStr 要下载的文件地址
* @return
*/
public
String download(String urlStr){
StringBuffer sb =
new
StringBuffer();
String line =
null
;
//BufferedReader有一个readLine()方法,可以每次读取一行数据
BufferedReader buffer =
null
;
try
{
//创建一个URL对象
url =
new
URL(urlStr);
//创建一个Http连接
HttpURLConnection urlConn = (HttpURLConnection) url
.openConnection();
//使用IO流读取数据,InputStreamReader将读进来的字节流转化成字符流
//但是字符流还不是很方便,所以再在外面套一层BufferedReader,
//用BufferedReader的readLine()方法,一行一行读取数据
buffer =
new
BufferedReader(
new
InputStreamReader(urlConn
.getInputStream()));
while
((line = buffer.readLine()) !=
null
){
sb.append(line);
}
}
catch
(Exception e) {
e.printStackTrace();
}
finally
{
try
{
buffer.close();
}
catch
(Exception e) {
e.printStackTrace();
}
}
return
sb.toString();
}
/**
* 该函数返回整型 -1:代表下载文件出错 0:代表下载文件成功 1:代表文件已经存在
*
* @param urlStr 下载文件的网络地址
* @param path 想要把下载过来的文件存放到哪一个SDCARD目录下
* @param fileName 下载的文件的文件名,可以跟原来的名字不同,所以这里加一个fileName
* @return
*/
public
int
downFile(String urlStr, String path, String fileName){
try
{
FileUtils fileUtils =
new
FileUtils();
if
(fileUtils.isFileExist(path + fileName)){
return
1
;
}
else
{
inputStream = getInputStreamFromURL(urlStr);
File resultFile = fileUtils.writeToSDFromInput(path, fileName, inputStream);
if
(resultFile ==
null
){
return
-
1
;
}
}
}
catch
(Exception e){
e.printStackTrace();
return
-
1
;
}
finally
{
try
{
inputStream.close();
}
catch
(Exception e){
e.printStackTrace();
}
}
return
0
;
}
/**
* 根据URL得到输入流
*
* @param urlStr
* @return
* @throws IOException
*/
public
InputStream getInputStreamFromURL(String urlStr){
HttpURLConnection urlConn =
null
;
try
{
url =
new
URL(urlStr);
urlConn = (HttpURLConnection) url.openConnection();
inputStream = urlConn.getInputStream();
}
catch
(MalformedURLException e) {
e.printStackTrace();
}
catch
(IOException e) {
e.printStackTrace();
}
return
inputStream;
}
}
|
strings.xml下添加按钮上显示的值
1
2
|
<string name=
"downloadTxt"
>下载TXT文件</string>
<string name=
"downloadMp3"
>下载Mp3文件</string>
|
另外由于需要访问网络和操作SDCARD,需要在AndroidManifest.xml的 <uses-sdk...同一级添加配置
1
2
|
<
uses-permission
android:name
=
"android.permission.INTERNET"
/>
<
uses-permission
android:name
=
"android.permission.WRITE_EXTERNAL_STORAGE"
/>
|
点击“下载TXT文件”,效果如下图:

点击“下载Mp3文件”,然后cmd进入命令行窗口,输入adb shell,就可以使用Linux命令行了,然后输入ls,查看有哪些文件,看到一个sdcard,然后输入cd sdcard,然后再输入ls,看到有一个voa文件夹,voa就是DownloadMp3Listener 这个类里面的downFile方法调用的第二个参数。再输入cd voa,再输入ls,就会发现有一个123.mp3文件,原来的mp3文件名已经修改,具体见DownloadMp3Listener 这个类里面的downFile方法调用的第三个参数
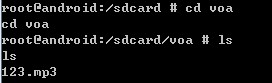