1 /// <reference path="jquery-1.10.2.min.js" />
2 var i = 0;
3 var timer; //设置定时器
4 $(function () {
5 $("#dlunbo").hover(function () {
6 $(".btn").show();
7 }, function () {
8 $(".btn").hide();
9 });
10 $(".ig").eq(0).show().siblings().hide(); //页面打开之后,第一个图片显示,其余图片隐藏
11 StartLunbo();
12 $("#tabs li").hover(function () { //鼠标放上去之后执行一个事件,
13 clearInterval(timer); //鼠标放上去之后,移除定时器,不能轮播
14 i = $(this).index();//获得当前索引
15 ShowPicTab();
16 }, function () { //鼠标离开之后执行一个事件
17 StartLunbo();
18 });
19 $(".btn1").click(function () { //左箭头
20 clearInterval(timer);
21 i--;
22 if (i == -1) {
23 i = 4;
24 }
25 ShowPicTab();
26 StartLunbo();
27 });
28 $(".btn2").click(function () { //右箭头
29 clearInterval(timer);
30 i++;
31 if (i == 5) {
32 i = 0;
33 }
34 ShowPicTab();
35 StartLunbo();
36 });
37 });
38
39
40 //封装相同的部分
41 function ShowPicTab()
42 {
43 $(".ig").eq(i).stop(true,true).fadeIn(300).siblings().stop(true,true).fadeOut(300);//有0.3s的渐变效果,加两个true可以立马将上一个动画结束掉,加一个true则是停止上一个动画
44 $("#tabs li").eq(i).addClass("bg").siblings().removeClass("bg");
45 }
46 function StartLunbo()
47 {
48 timer = setInterval(function () { //间隔4s执行一个轮播事件
49 i++;
50 if (i == 5) {
51 i = 0;
52 }
53 ShowPicTab();
54 }, 4000);
55 }
1 *{
2 padding:0px;
3 margin:0px;
4 font-family:"微软雅黑";
5 list-style-type:none;
6 }
7 #dlunbo{
8 width:520px;
9 height:280px;
10 position:absolute;
11 left:50%;
12 margin-left:-260px;
13 top:50%;
14 margin-top:-140px;
15 }
16 .ig{
17 position:absolute;
18 }
19 #tabs{
20 position:absolute;
21 bottom:10px;
22 left:200px;
23 }
24 #tabs li{
25 width:20px;
26 height:20px;
27 border:solid 1px #fff;
28 float:left;
29 margin-right:5px;
30 border-radius:100%;
31 cursor:pointer;
32 }
33 .btn{
34 position:absolute;
35 width:30px;
36 height:50px;
37 background:rgba(0,0,0,0.5);
38 color:#fff;
39 text-align:center;
40 line-height:50px;
41 font-size:30px;
42 top:50%;
43 margin-top:-25px;
44 cursor:pointer;
45 display:none;
46 }
47 .btn1{
48 left:0px;
49 }
50 .btn2{
51 right:0px;
52 }
53 #tabs .bg{
54 background-color:#ff0000;
55 }
1 <!DOCTYPE html>
2 <html xmlns="http://www.w3.org/1999/xhtml">
3 <head>
4 <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
5 <title></title>
6 <link href="css/demo1.css" rel="stylesheet" />
7 <script src="js/jquery-1.10.2.min.js"></script>
8 <script src="js/demo1.js"></script>
9 </head>
10 <body>
11 <div id="dlunbo">
12 <div id="igs">
13 <div class="ig"><img src="img/1.jpg"/></div>
14 <div class="ig"><img src="img/2.jpg" /></div>
15 <div class="ig"><img src="img/3.jpg" /></div>
16 <div class="ig"><img src="img/4.jpg" /></div>
17 <div class="ig"><img src="img/5.jpg" /></div>
18 </div>
19 <ul id="tabs">
20 <li class="bg"></li>
21 <li></li>
22 <li></li>
23 <li></li>
24 <li></li>
25 </ul>
26 <div class="btn btn1"><</div>
27 <div class="btn btn2">></div>
28 </div>
29 </body>
30 </html>
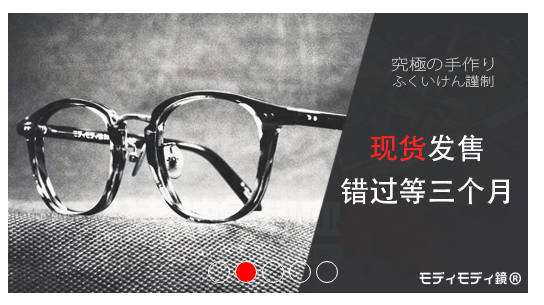