Description
A tournament can be represented by a complete graph in which each vertex denotes a player and a directed edge is from vertex x to vertex y if player x beats player y. For a player x in a tournament T, the score of x is the number of players beaten by x. The score sequence of T, denoted by S(T) = (s1, s2, . . . , sn), is a non-decreasing list of the scores of all the players in T. It can be proved that S(T) = (s1, s2, . . . , sn) is a score sequence of T if and only if
for k = 1, 2, . . . , n and equality holds when k = n. A player x in a tournament is a strong king if and only if x beats all of the players whose scores are greater than the score of x. For a score sequence S, we say that a tournament T realizes S if S(T) = S. In particular, T is a heavy tournament realizing S if T has the maximum number of strong kings among all tournaments realizing S. For example, see T2 in Figure 1. Player a is a strong king since the score of player a is the largest score in the tournament. Player b is also a strong king since player b beats player a who is the only player having a score larger than player b. However, players c, d and e are not strong kings since they do not beat all of the players having larger scores.
The purpose of this problem is to find the maximum number of strong kings in a heavy tournament after a score sequence is given. For example,Figure 1 depicts two possible tournaments on five players with the same score sequence (1, 2, 2, 2, 3). We can see that there are at most two strong kings in any tournament with the score sequence (1, 2, 2, 2, 3) since the player with score 3 can be beaten by only one other player. We can also see that T2 contains two strong kings a and b. Thus, T2 is one of heavy tournaments. However, T1 is not a heavy tournament since there is only one strong king in T1. Therefore, the answer of this example is 2.
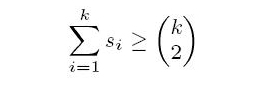
for k = 1, 2, . . . , n and equality holds when k = n. A player x in a tournament is a strong king if and only if x beats all of the players whose scores are greater than the score of x. For a score sequence S, we say that a tournament T realizes S if S(T) = S. In particular, T is a heavy tournament realizing S if T has the maximum number of strong kings among all tournaments realizing S. For example, see T2 in Figure 1. Player a is a strong king since the score of player a is the largest score in the tournament. Player b is also a strong king since player b beats player a who is the only player having a score larger than player b. However, players c, d and e are not strong kings since they do not beat all of the players having larger scores.
The purpose of this problem is to find the maximum number of strong kings in a heavy tournament after a score sequence is given. For example,Figure 1 depicts two possible tournaments on five players with the same score sequence (1, 2, 2, 2, 3). We can see that there are at most two strong kings in any tournament with the score sequence (1, 2, 2, 2, 3) since the player with score 3 can be beaten by only one other player. We can also see that T2 contains two strong kings a and b. Thus, T2 is one of heavy tournaments. However, T1 is not a heavy tournament since there is only one strong king in T1. Therefore, the answer of this example is 2.
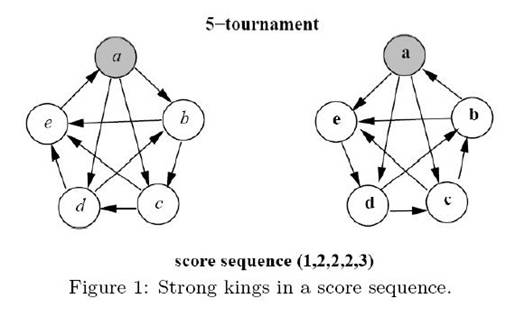
Input
The first line of the input file contains an integer m, m <= 10, which represents the number of test cases. The following m lines contain m score sequences in which each line contains a score sequence. Note that each score sequence contains at most ten scores.
Output
The maximum number of strong kings for each test case line by line.
Sample Input
5 1 2 2 2 3 1 1 3 4 4 4 4 3 3 4 4 4 4 5 6 6 6 0 3 4 4 4 5 5 5 6 0 3 3 3 3 3
Sample Output
2 4 5 3 5
Source
略神的想法,竟然是将边视为点做.
首先他的答案具有单调性,我们可以二分有多少个kings,这里直接枚举也行。
然后对于k个kings,我们枚举有哪些是最后的答案,对于所有边u<-->v(score[u]<=score[v]),如果存在u是king,且score[u]<score[v]则说明u一定要赢v,就将这条边的标号向u连一条权值为1的边,否则他们谁赢都行,就将这条边的标号向u与v同时连一条权值为1的边,表示他可以随便取,然后s向每条边连一条权值为1的边,表示一条边只能用一次,每个点向t连一条权值为score[]的边,表示一个点要赢score[]次,跑一边最大流,看流量是否达到N*(N-1)/2。
#include<cstdio>
#include<cstdlib>
#include<iostream>
#include<cstring>
#include<string>
#include<algorithm>
#include<cmath>
#include<ctime>
using namespace std;
int N,a[200000];
const int inf=0x7fffffff;
int tot,nex[200000],fir[200000],en[200000],f[200000];
void ins(int a,int b,int c){
nex[++tot]=fir[a];fir[a]=tot;en[tot]=b;f[tot]=c;
nex[++tot]=fir[b];fir[b]=tot;en[tot]=a;f[tot]=0;
}
int flog=0,s,t;
int num[200000],his[200000],pre[200000],now[200000],d[200000];
void sap(){
flog=0;
for (int i=0;i<=t;i++){
d[i]=num[i]=pre[i]=his[i]=0;
now[i]=fir[i];
}
num[0]=t;
int aug=inf;
int i=s;
bool flag;
while (d[s]<t){
his[i]=aug;
flag=false;
for (int k=now[i];k;k=nex[k])
if (d[i]==d[en[k]]+1&&f[k]>0){
pre[en[k]]=i;
now[i]=k;
aug=min(aug,f[k]);
flag=true;
i=en[k];
if (i==t){
flog+=aug;
while (i!=s){
i=pre[i];
f[now[i]]-=aug;
f[now[i]^1]+=aug;
}
aug=inf;
}
break;
}
if (flag) continue;
int k1=0,minn=t;
for (int k=fir[i];k;k=nex[k])
if (d[en[k]]<minn&&f[k]>0){
minn=d[en[k]];
k1=k;
}
num[d[i]]--;
if (!num[d[i]]) return;
d[i]=minn+1;
num[d[i]]++;
now[i]=k1;
if (i!=s){
i=pre[i];
aug=his[i];
}
}
}
int u[200000],v[200000];
int Sum[200000];
bool check(int x){
if (a[N-x+1]<Sum[N-x+1]) return false;
tot=1;flog=0;
s=N+N*(N-1)/2+1;t=s+1;
for (int i=1;i<=t;i++) fir[i]=0;
for (int i=1;i<=N;i++) ins(s,i,a[i]);
int idx=0;
for (int i=1;i<=N-1;i++)
for (int j=i+1;j<=N;j++){
++idx;
ins(idx+N,t,1);
if (N-x+1<=i&&a[i]<a[j]) ins(i,idx+N,1);
else ins(i,idx+N,1),ins(j,idx+N,1);
}
sap();
if (flog>=N*(N-1)/2) return true;
else return false;
}
int main(){
// freopen("a.in","r",stdin);
// freopen("a.out","w",stdout);
int T;scanf("%d\n",&T);
while (T--){
char str[2000];
gets(str);
N=1;
for(int i=0;i<strlen(str);i++)
if(str[i]>='0'&& str[i]<='9')
a[N++]=str[i]-'0';
N--;
for (int i=1;i<=N-1;i++)
for (int j=i+1;j<=N;j++){
if (a[i]<a[j]) Sum[i]++;
}
int pos=0;
for (pos=N;pos>=1&&check(pos)==false;pos--);
printf("%d\n",pos);
for (int i=1;i<=N;i++) a[i]=Sum[i]=0;
}
return 0;
}