FreeMarker 模板静态化
使用freemarker将页面生成html文件,本节测试html文件生成的方法:
1、使用模板文件静态化 定义模板文件,使用freemarker静态化程序生成html文件。
2、使用模板字符串静态化 定义模板字符串,使用freemarker静态化程序生成html文件。
@SpringBootTest(classes = FreemarkerTest.class)
@RunWith(SpringRunner.class)
public class FreemarkerTest {
// 静态化 基于ftl模板文件生成HTML文件
@Test
public void testGenerateHtml() throws Exception {
// 得到配置类
Configuration configuration = new Configuration(Configuration.getVersion());
// 获得classPath路径
String classPath = this.getClass().getResource("/").getPath();
// 设置模板加载路径
configuration.setDirectoryForTemplateLoading(new File(classPath + "/templates/"));
// 获取模板
Template template = configuration.getTemplate("test1.ftl");
// 模板数据
Map<String, Object> map = getMap();
// 静态化
String content = FreeMarkerTemplateUtils.processTemplateIntoString(template, map);
InputStream inputStream = IOUtils.toInputStream(content);
FileOutputStream fileOutputStream = new FileOutputStream(new File("E:/test1.html"));
// 输出文件
IOUtils.copy(inputStream, fileOutputStream);
inputStream.close();
fileOutputStream.close();
}
// 静态化 基于模板文件内容(String)生成HTML文件
@Test
public void testGenerateHtmlByString() throws Exception {
// 得到配置类
Configuration configuration = new Configuration(Configuration.getVersion());
// 模板内容
String templateString = "<html>\n" +
" <head></head>\n" +
" <body>\n" +
" 名称:${name}\n" +
" </body>\n" +
"</html>";
// 使用一个模板加载器变成模板
StringTemplateLoader stringTemplateLoader = new StringTemplateLoader();
// 设置模板内容及名称
stringTemplateLoader.putTemplate("template", templateString);
// 设置模板加载器
configuration.setTemplateLoader(stringTemplateLoader);
// 获取template内容
Template template = configuration.getTemplate("template", "utf-8");
// 模板数据
Map<String, Object> map = getMap();
// 静态化
String content = FreeMarkerTemplateUtils.processTemplateIntoString(template, map);
InputStream inputStream = IOUtils.toInputStream(content);
FileOutputStream fileOutputStream = new FileOutputStream(new File("E:/test2.html"));
// 输出文件
IOUtils.copy(inputStream, fileOutputStream);
inputStream.close();
fileOutputStream.close();
}
// 設置模板数据
public static Map<String, Object> getMap() {
Map<String, Object> map = new HashMap<>();
map.put("name", "11");
Student stu1 = new Student();
stu1.setName("小明");
stu1.setAge(18);
stu1.setMoney(1000.86f);
stu1.setBirthday(new Date());
Student stu2 = new Student();
stu2.setName("小红");
stu2.setMoney(200.1f);
stu2.setAge(19); //
stu2.setBirthday(new Date());
List<Student> friends = new ArrayList<>();
friends.add(stu1);
stu2.setFriends(friends);
stu2.setBestFriend(stu1);
List<Student> stus = new ArrayList<>();
stus.add(stu1);
stus.add(stu2); //向数据模型放数据
map.put("stus", stus); //准备map数据
HashMap<String, Student> stuMap = new HashMap<>();
stuMap.put("stu1", stu1);
stuMap.put("stu2", stu2); //向数据模型放数据
map.put("stu1", stu1); //向数据模型放数据
map.put("stuMap", stuMap); //返回模板文件名称
return map;
}
}
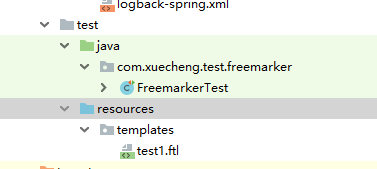
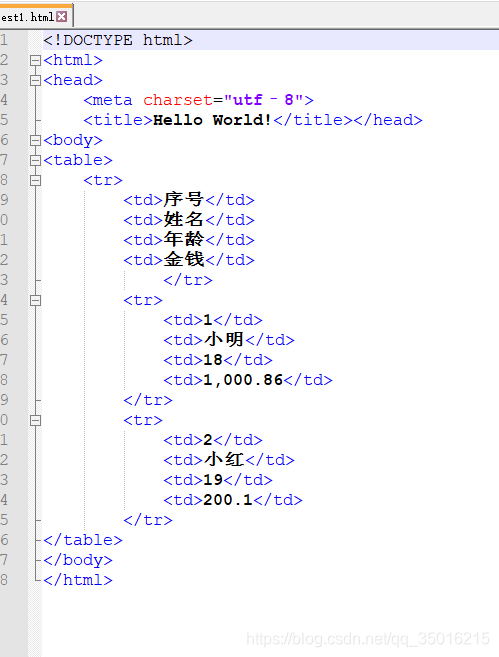