1.useSearchParams
参考链接:https://reactrouter.com/en/6.6.2/hooks/use-search-params
emmm,先看下有哪些方法
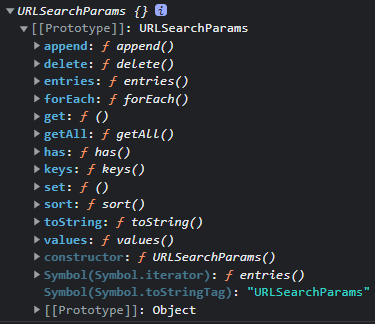
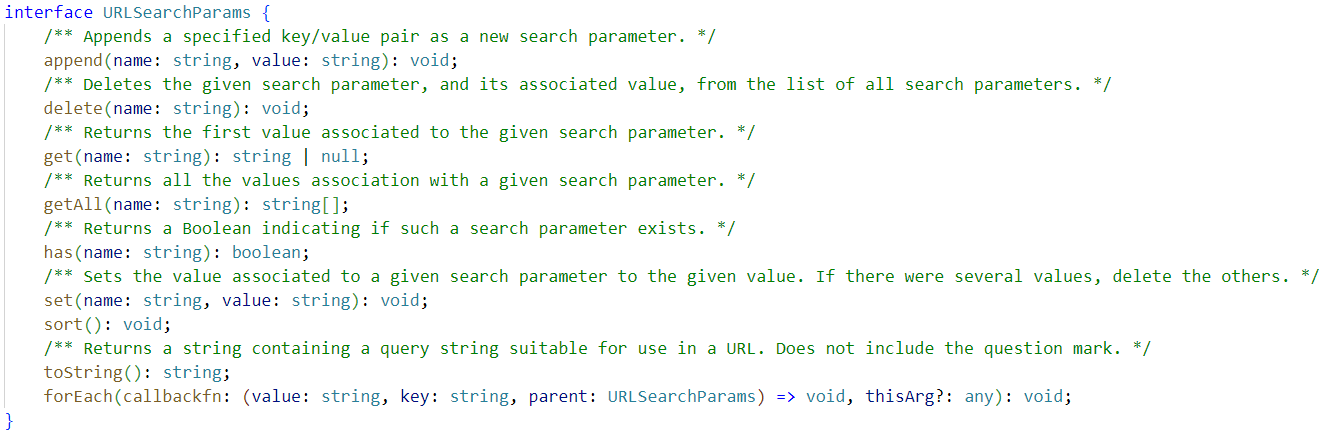
get,has,append,delete,getAll
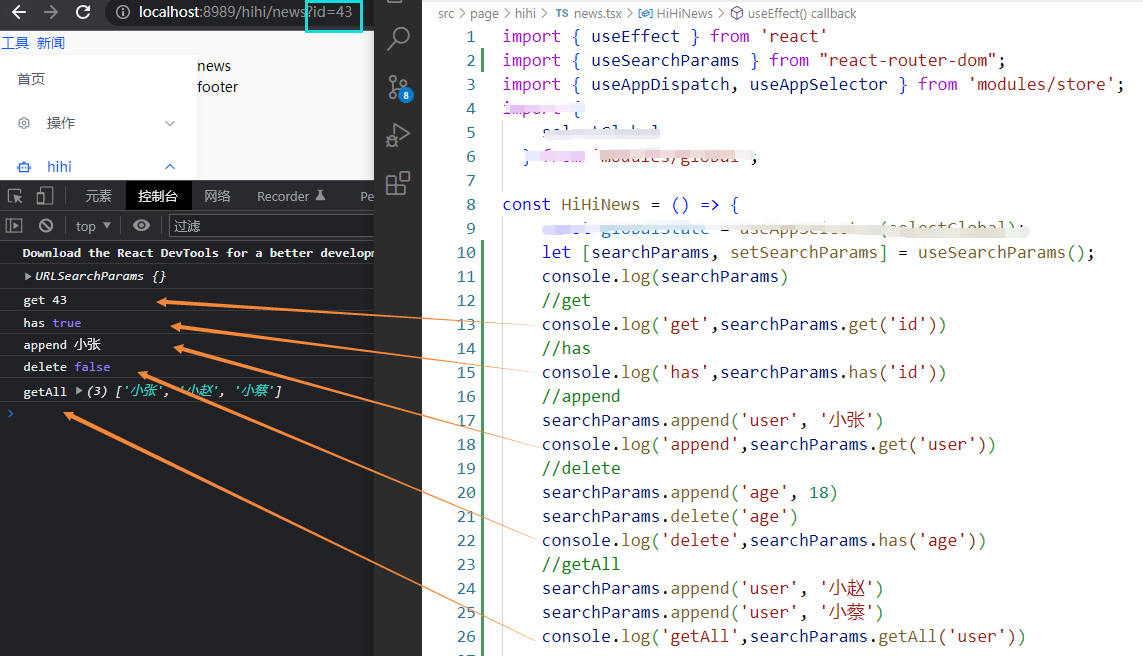
set,keys,sort,forEach,toString
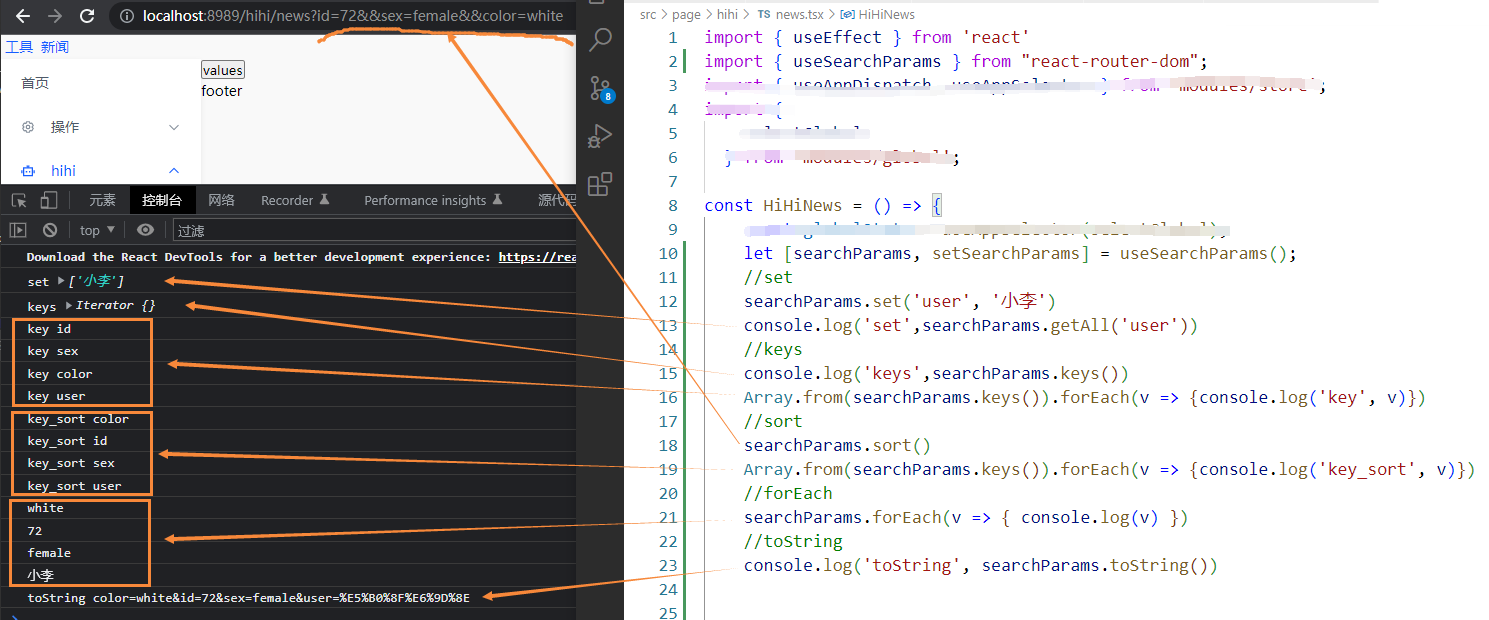
values,entries
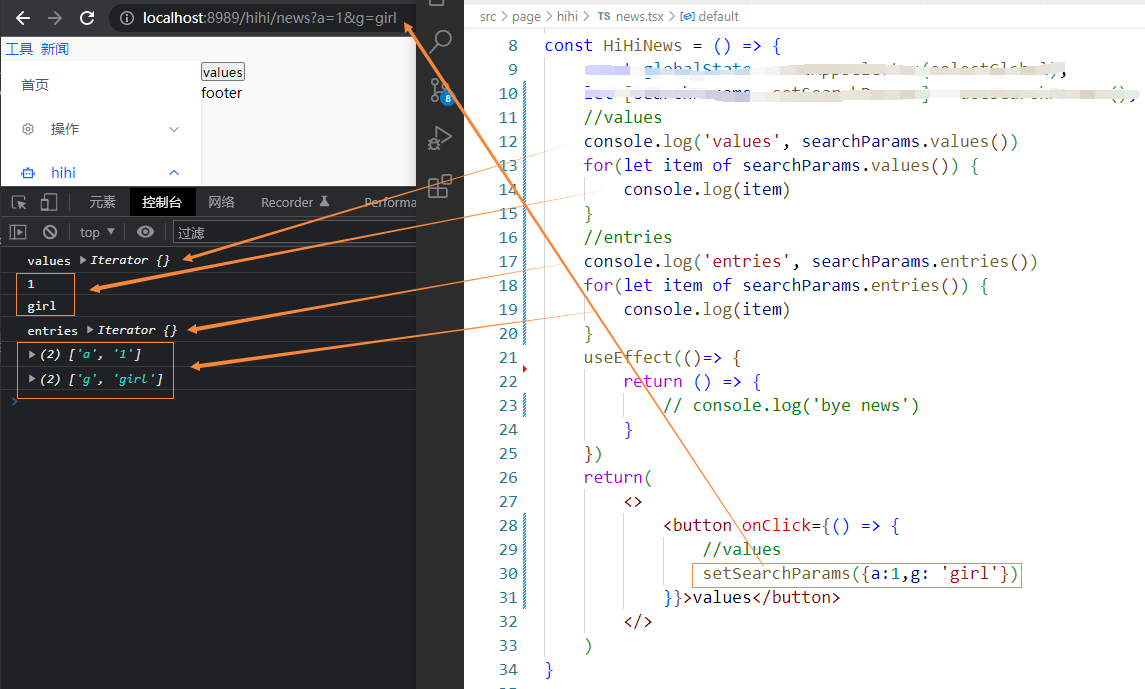
2.useParams
获取URL路由参数,首先设置路由news为news/:id
import { lazy, ReactNode, Suspense } from 'react';
import { IRouter } from '../routes'
import { IconRobot } from '@arco-design/web-react/icon';
const News = lazy(() => import('page/hihi/news'))
const Tools = lazy(() => import('page/hihi/tools'))
const lazyLoad = (children: ReactNode): ReactNode =>{
return <Suspense fallback={<h1>Loading...</h1>}>
{children}
</Suspense>
}
const HiHiRoutes:IRouter[] = [
{
path: '/hihi',
children: [
{
path: 'news/:id/:page',
element: lazyLoad(<News />),
meta: {
title: '新闻'
}
},
{
path: 'tools',
element: lazyLoad(<Tools />),
meta: {
title: '工具'
}
}
],
meta: {
title: 'hihi',
Icon: <IconRobot />
}
}
]
export default HiHiRoutes;
import { useParams } from "react-router-dom";
//...当中省略了
const { id, page } = useParams()
console.log('id',id)
console.log('page',page)
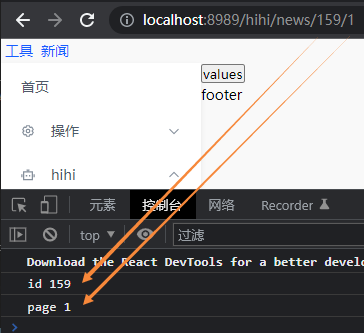
3.useMatch
匹配当前路由URL
import { useMatch } from "react-router-dom";
//...省略
const newsUseMatch = useMatch('/hihi/news/:id/:page')
console.log(newsUseMatch)
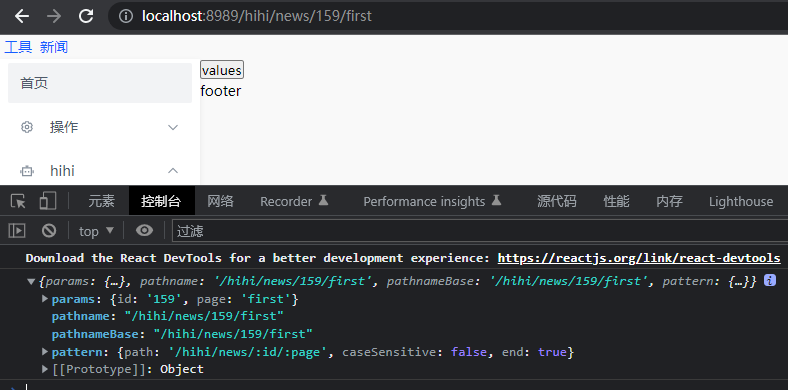
可以看一下类型 O(∩_∩)O
//源码类型
export declare function useMatch<ParamKey extends ParamParseKey<Path>, Path extends string>(pattern: PathPattern<Path> | Path): PathMatch<ParamKey> | null;
//PathMatch返回
/**
* A PathMatch contains info about how a PathPattern matched on a URL pathname.
*/
export interface PathMatch<ParamKey extends string = string> {
/**
* The names and values of dynamic parameters in the URL.
*/
params: Params<ParamKey>;
/**
* The portion of the URL pathname that was matched.
*/
pathname: string;
/**
* The portion of the URL pathname that was matched before child routes.
*/
pathnameBase: string;
/**
* The pattern that was used to match.
*/
pattern: PathPattern;
}
//PathPattern
export interface PathPattern<Path extends string = string> {
/**
* A string to match against a URL pathname. May contain `:id`-style segments
* to indicate placeholders for dynamic parameters. May also end with `/*` to
* indicate matching the rest of the URL pathname.
*/
path: Path;
/**
* Should be `true` if the static portions of the `path` should be matched in
* the same case.
*/
caseSensitive?: boolean;
/**
* Should be `true` if this pattern should match the entire URL pathname.
*/
end?: boolean;
}
/**
* A data route object, which is just a RouteObject with a required unique ID
*/
export declare type AgnosticDataRouteObject = AgnosticDataIndexRouteObject | AgnosticDataNonIndexRouteObject;
declare type _PathParam<Path extends string> = Path extends `${infer L}/${infer R}` ? _PathParam<L> | _PathParam<R> : Path extends `:${infer Param}` ? Param : never;
/**
* Examples:
* "/a/b/*" -> "*"
* ":a" -> "a"
* "/a/:b" -> "b"
* "/a/blahblahblah:b" -> "b"
* "/:a/:b" -> "a" | "b"
* "/:a/b/:c/*" -> "a" | "c" | "*"
*/
declare type PathParam<Path extends string> = Path extends "*" ? "*" : Path extends `${infer Rest}/*` ? "*" | _PathParam<Rest> : _PathParam<Path>;
export declare type ParamParseKey<Segment extends string> = [
PathParam<Segment>
] extends [never] ? string : PathParam<Segment>;
/**
* The parameters that were parsed from the URL path.
*/
export declare type Params<Key extends string = string> = {
readonly [key in Key]: string | undefined;
};
4.useLocation
import React from 'react';
import { Link, Space } from '@arco-design/web-react';
import { NavLink } from 'react-router-dom';
const layOutHeader = React.memo(() => {
return (
<>
<Link href='hihi/tools'> 工具 </Link>
<NavLink
to = {`hihi/news?id=${Math.ceil(Math.random()*100)}&sex=female&color=white`}
state = {{
username: '小刘',
age: 23,
like: 'food'
}}
>新闻</NavLink>
</>
)
})
export default React.memo(layOutHeader)
import { useLocation } from "react-router-dom";
//...省略
console.log(useLocation())
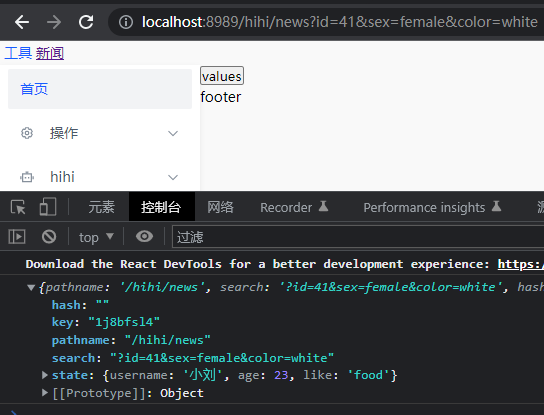
查看类型
export declare function useLocation(): Location;
/**
* The pathname, search, and hash values of a URL.
*/
export interface Path {
/**
* A URL pathname, beginning with a /.
*/
pathname: string;
/**
* A URL search string, beginning with a ?.
*/
search: string;
/**
* A URL fragment identifier, beginning with a #.
*/
hash: string;
}
/**
* An entry in a history stack. A location contains information about the
* URL path, as well as possibly some arbitrary state and a key.
*/
export interface Location extends Path {
/**
* A value of arbitrary data associated with this location.
*/
state: any;
/**
* A unique string associated with this location. May be used to safely store
* and retrieve data in some other storage API, like `localStorage`.
*
* Note: This value is always "default" on the initial location.
*/
key: string;
}
5.useNavigate
路由跳转,页面刷新后,state数据还是保留的
replace用于控制跳转模式(false为push,true为replace),会修改回退路径
import React from 'react';
import { Link, Space } from '@arco-design/web-react';
import { NavLink, useNavigate } from 'react-router-dom';
import { Button } from '@arco-design/web-react';
const layOutHeader = React.memo(() => {
const navigate = useNavigate();
return (
<>
<Link href='hihi/tools'> 工具 </Link>
<Button type='primary' onClick={() => {
navigate("hihi/news", {
replace: false,
state: {
id: 1,
name: '安娜',
dep: '技术部',
}
})
}}>新闻</Button>
</>
)
})
export default React.memo(layOutHeader)
import { useEffect } from 'react'
import { useLocation } from "react-router-dom";
import {
selectGlobal
} from 'modules/global';
const HiHiNews = () => {
const { state: { id, name, dep} } = useLocation()
return(
<>
<div>{ id }</div>
<div>{ name }</div>
<div>{ dep }</div>
</>
)
}
export default HiHiNews
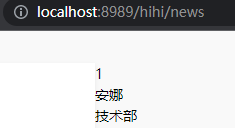
查看类型
/**
* The interface for the navigate() function returned from useNavigate().
*/
export interface NavigateFunction {
(to: To, options?: NavigateOptions): void;
(delta: number): void;
}
/**
* Returns an imperative method for changing the location. Used by <Link>s, but
* may also be used by other elements to change the location.
*
* @see https://reactrouter.com/hooks/use-navigate
*/
export declare function useNavigate(): NavigateFunction;
export declare type RelativeRoutingType = "route" | "path";
//NavigateOptions
export interface NavigateOptions {
replace?: boolean;
state?: any;
preventScrollReset?: boolean;
relative?: RelativeRoutingType;
}
我会继续更新...