介绍
概念:
Bridge模式又叫做桥接模式,是构造型的设计模式之- -。Bridge模式基于类的最小设计
原则,通过使用封装,聚合以及继承等行为来让不同的类承担不同的责任。它的主要特点是
把抽象(abstraction 与行为实现(implementation)分离开来,从而可以保持各部分的独
立性以及应对它们的功能扩展。
- Abstractione
抽象类接口(接口或抽象类)维护对行为实现(Implementor)的引用。 - Refined Abstractione
Abstraction子类。 - Implementors
行为实现类接口(Abstraction 接口定义了基于Implementor接口的更高层次的操作) - Concretelmplementors
Implementor子类。
优点:将抽象部分与实现部分分离(解耦合),使它们都可以独立的变化。
UML类图
简单代码:
#ifndef SIMPLE_BRIDGE_H
#define SIMPLE_BRIDGE_H
#include <iostream>
using namespace std;
class Implementor
{
public:
virtual void operation() = 0;
};
class ConcreteImplementorA : public Implementor
{
void operation() override
{
cout<<"具体实现A的方法执行"<<endl;
}
};
class ConcreteImplementorB : public Implementor
{
void operation() override
{
cout<<"具体实现B的方法执行"<<endl;
}
};
class Abstraction
{
public:
void setImplementor(Implementor *imp)
{
m_imp = imp;
}
void operation()
{
m_imp->operation();
}
protected:
Implementor *m_imp;
};
class RefinedAbstraction : public Abstraction
{
public:
void operation()
{
m_imp->operation();
}
};
#endif // SIMPLE_BRIDGE_H
调用:
void simpleBridge()
{
Abstraction *ab = new RefinedAbstraction;
ab->setImplementor( new ConcreteImplementorA);
ab->operation();
ab->setImplementor( new ConcreteImplementorB);
ab->operation();
}
手机软件和品牌例子:(大话设计模式第22章)
UML图
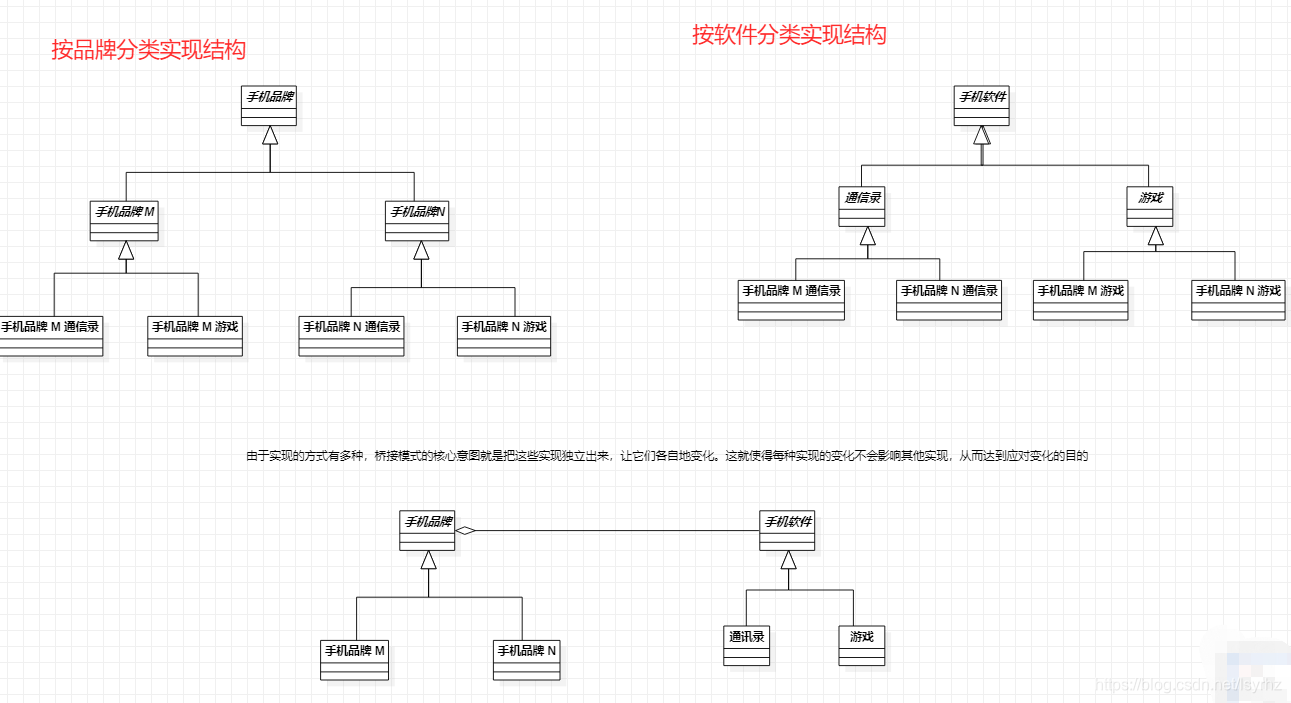
代码:
#ifndef MOBILEPHONE_MODE_H
#define MOBILEPHONE_MODE_H
#include <iostream>
using namespace std;
/**
* @brief The HandsetSoft class
* 手机软件
*/
class HandsetSoft
{
public:
virtual void run() = 0;
};
/**
* @brief The HandsetGame class
* 手机游戏
*/
class HandsetGame : public HandsetSoft
{
public:
void run() override
{
cout<<" 运行手机游戏 "<<endl;
}
};
/**
* @brief The HandsetAddressList class
* 手机通信录
*/
class HandsetAddressList : public HandsetSoft
{
public:
void run() override
{
cout<<" 运行手机通信录 "<<endl;
}
};
/**
* @brief The HandsetMedia class
* +新加多媒体
*/
class HandsetMedia : public HandsetSoft
{
public:
void run() override
{
cout<<" 运行手机多媒体 "<<endl;
}
};
/**
* @brief The HandsetBrand class
* 手机品牌
*/
class HandsetBrand
{
public:
//设置手机软件
void setHandsetSoft(HandsetSoft *soft)
{
m_soft = soft;
}
virtual void run() = 0;
protected:
HandsetSoft *m_soft;
};
/**
* @brief The HandsetBrandN class
* 手机品牌N
*/
class HandsetBrandN : public HandsetBrand
{
public:
void run() override
{
cout<<"手机品牌 N:"<<endl;
m_soft->run();
}
};
/**
* @brief The HandsetBrandM class
* 手机品牌M
*/
class HandsetBrandM : public HandsetBrand
{
public:
void run() override
{
cout<<"手机品牌 M:"<<endl;
m_soft->run();
}
};
/**
* @brief The HandsetBrandS class
* +新增手机品牌
*/
class HandsetBrandS : public HandsetBrand
{
public:
void run() override
{
cout<<"手机品牌 S:"<<endl;
m_soft->run();
}
};
#endif // MOBILEPHONE_MODE_H
调用:
void mobilephoneBidge()
{
HandsetBrand *ab = nullptr;
ab = new HandsetBrandN();
ab->setHandsetSoft( new HandsetGame() );
ab->run();
ab->setHandsetSoft(new HandsetAddressList() );
ab->run();
ab = new HandsetBrandM();
ab->setHandsetSoft( new HandsetGame() );
ab->run();
ab->setHandsetSoft(new HandsetAddressList() );
ab->run();
delete ab;
ab = nullptr;
}