乐在其中设计模式(C#) - 中介者模式(Mediator Pattern)
作者: webabcd
介绍
用一个中介对象来封装一系列的对象交互。中介者使各对象不需要显式地相互引用,从而使其耦合松散,而且可以独立地改变它们之间的交互。
示例
有一个Message实体类,某个对象对它的操作有Send()和Insert()方法,现在用一个中介对象来封装这一系列的对象交互。
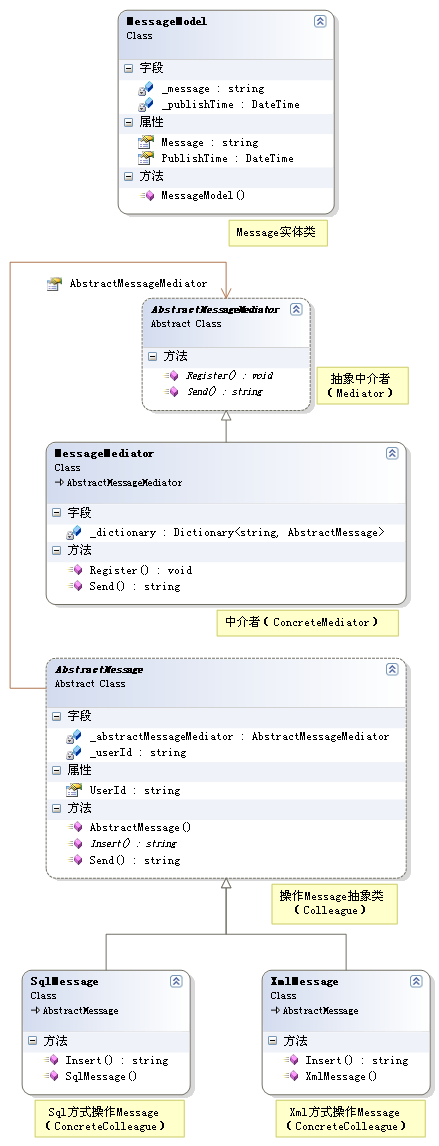
MessageModel












































AbstractMessageMediator




























MessageMediator

















































AbstractMessage





























































SqlMessage




































XmlMessage




































Test












































运行结果
Sql方式插入Message(user1发送给user2) - 内容:你好! - 时间:2007-5-19 23:43:19
Sql方式插入Message(user2发送给user1) - 内容:我不好! - 时间:2007-5-19 23:43:19
Sql方式插入Message(user1发送给user2) - 内容:不好就不好吧。 - 时间:2007-5-19 23:43:19
Xml方式插入Message(user3发送给user4) - 内容:吃了吗? - 时间:2007-5-19 23:43:19
Xml方式插入Message(user4发送给user3) - 内容:没吃,你请我? - 时间:2007-5-19 23:43:19
Xml方式插入Message(user3发送给user4) - 内容:不请。 - 时间:2007-5-19 23:43:19
参考
http://www.dofactory.com/Patterns/PatternMediator.aspx
OK
[源码下载]
设计模式22:Mediator Pattern (调解者模式)
英文原文:http://www.dofactory.com/Patterns/PatternMediator.aspx
一、Mediator Pattern (调解者模式)的定义
Define an object that encapsulates how a set of objects interact. Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it lets you vary their interaction independently.
定义一个对象将一系列对象如何相互作用的操作进行封装。调解者通过避免一个对象明确指向其余的对象来促使自由耦合,同时能够让你单独的改变他们之间的相互作用。
二、UML类图
- Mediator (IChatroom)
- 定义一个接口用于与同事级对象进行通信
- ConcreteMediator (Chatroom)
- 通过与同事级对象进行对等来实现相互协作行为
- 知道并且维持本身的同事级对象
- Colleague classes (Participant)
- 每个同事级对象都知道自己的调节者对象
- 每个同事级对象在与另外的同事级对象进行通信时必须要与调节者进行通信。
三、调解者模式实例代码
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- namespace Mediator_Pattern
- {
- /// <summary>
- /// MainApp startup class for Structural
- /// Mediator Design Pattern.
- /// </summary>
- class MainApp
- {
- /// <summary>
- /// Entry point into console application.
- /// </summary>
- static void Main()
- {
- ConcreteMediator m = new ConcreteMediator();
- ConcreteColleague1 c1 = new ConcreteColleague1(m);
- ConcreteColleague2 c2 = new ConcreteColleague2(m);
- m.Colleague1 = c1;
- m.Colleague2 = c2;
- c1.Send("How are you?");
- c2.Send("Fine, thanks");
- // Wait for user
- Console.ReadKey();
- }
- }
- /// <summary>
- /// The 'Mediator' abstract class
- /// </summary>
- abstract class Mediator
- {
- public abstract void Send(string message,
- Colleague colleague);
- }
- /// <summary>
- /// The 'ConcreteMediator' class
- /// </summary>
- class ConcreteMediator : Mediator
- {
- private ConcreteColleague1 _colleague1;
- private ConcreteColleague2 _colleague2;
- public ConcreteColleague1 Colleague1
- {
- set { _colleague1 = value; }
- }
- public ConcreteColleague2 Colleague2
- {
- set { _colleague2 = value; }
- }
- public override void Send(string message,
- Colleague colleague)
- {
- if (colleague == _colleague1)
- {
- _colleague2.Notify(message);
- }
- else
- {
- _colleague1.Notify(message);
- }
- }
- }
- /// <summary>
- /// The 'Colleague' abstract class
- /// </summary>
- abstract class Colleague
- {
- protected Mediator mediator;
- // Constructor
- public Colleague(Mediator mediator)
- {
- this.mediator = mediator;
- }
- }
- /// <summary>
- /// A 'ConcreteColleague' class
- /// </summary>
- class ConcreteColleague1 : Colleague
- {
- // Constructor
- public ConcreteColleague1(Mediator mediator)
- : base(mediator)
- {
- }
- public void Send(string message)
- {
- mediator.Send(message, this);
- }
- public void Notify(string message)
- {
- Console.WriteLine("Colleague1 gets message: "
- + message);
- }
- }
- /// <summary>
- /// A 'ConcreteColleague' class
- /// </summary>
- class ConcreteColleague2 : Colleague
- {
- // Constructor
- public ConcreteColleague2(Mediator mediator)
- : base(mediator)
- {
- }
- public void Send(string message)
- {
- mediator.Send(message, this);
- }
- public void Notify(string message)
- {
- Console.WriteLine("Colleague2 gets message: "
- + message);
- }
- }
- }
四、另外一个使用调解者模式的实例代码
This real-world code demonstrates the Mediator pattern facilitating loosely coupled communication between different Participants registering with a Chatroom. The Chatroom is the central hub through which all communication takes place. At this point only one-to-one communication is implemented in the Chatroom, but would be trivial to change to one-to-many.
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- namespace Mediator_Pattern
- {
- /// <summary>
- /// MainApp startup class for Real-World
- /// Mediator Design Pattern.
- /// </summary>
- class MainApp
- {
- /// <summary>
- /// Entry point into console application.
- /// </summary>
- static void Main()
- {
- // Create chatroom
- Chatroom chatroom = new Chatroom();
- // Create participants and register them
- Participant George = new Beatle("George");
- Participant Paul = new Beatle("Paul");
- Participant Ringo = new Beatle("Ringo");
- Participant John = new Beatle("John");
- Participant Yoko = new NonBeatle("Yoko");
- chatroom.Register(George);
- chatroom.Register(Paul);
- chatroom.Register(Ringo);
- chatroom.Register(John);
- chatroom.Register(Yoko);
- // Chatting participants
- Yoko.Send("John", "Hi John!");
- Paul.Send("Ringo", "All you need is love");
- Ringo.Send("George", "My sweet Lord");
- Paul.Send("John", "Can't buy me love");
- John.Send("Yoko", "My sweet love");
- // Wait for user
- Console.ReadKey();
- }
- }
- /// <summary>
- /// The 'Mediator' abstract class
- /// </summary>
- abstract class AbstractChatroom
- {
- public abstract void Register(Participant participant);
- public abstract void Send(
- string from, string to, string message);
- }
- /// <summary>
- /// The 'ConcreteMediator' class
- /// </summary>
- class Chatroom : AbstractChatroom
- {
- private Dictionary<string, Participant> _participants =
- new Dictionary<string, Participant>();
- public override void Register(Participant participant)
- {
- if (!_participants.ContainsValue(participant))
- {
- _participants[participant.Name] = participant;
- }
- participant.Chatroom = this;
- }
- public override void Send(
- string from, string to, string message)
- {
- Participant participant = _participants[to];
- if (participant != null)
- {
- participant.Receive(from, message);
- }
- }
- }
- /// <summary>
- /// The 'AbstractColleague' class
- /// </summary>
- class Participant
- {
- private Chatroom _chatroom;
- private string _name;
- // Constructor
- public Participant(string name)
- {
- this._name = name;
- }
- // Gets participant name
- public string Name
- {
- get { return _name; }
- }
- // Gets chatroom
- public Chatroom Chatroom
- {
- set { _chatroom = value; }
- get { return _chatroom; }
- }
- // Sends message to given participant
- public void Send(string to, string message)
- {
- _chatroom.Send(_name, to, message);
- }
- // Receives message from given participant
- public virtual void Receive(
- string from, string message)
- {
- Console.WriteLine("{0} to {1}: '{2}'",
- from, Name, message);
- }
- }
- /// <summary>
- /// A 'ConcreteColleague' class
- /// </summary>
- class Beatle : Participant
- {
- // Constructor
- public Beatle(string name)
- : base(name)
- {
- }
- public override void Receive(string from, string message)
- {
- Console.Write("To a Beatle: ");
- base.Receive(from, message);
- }
- }
- /// <summary>
- /// A 'ConcreteColleague' class
- /// </summary>
- class NonBeatle : Participant
- {
- // Constructor
- public NonBeatle(string name)
- : base(name)
- {
- }
- public override void Receive(string from, string message)
- {
- Console.Write("To a non-Beatle: ");
- base.Receive(from, message);
- }
- }
- }
四.中介者模式案例分析(Example)
1、场景
实现一个聊天室功能,聊城室就是一个中介者,参与聊天的人就是同事对象,如下图所示
AbstractChatroom类:抽象聊天室类,做为Participant的交互的中介。
Register()方法:会员注册功能;Send()方法:发送消息功能。
Chatroom类:具体聊天室类,实现抽象聊天室类中的方法。
Participant类:参与者类,主要有发送消息Send()功能和接受消息Receive()功能。
Beatle类,NonBeatle类:参与者类的具体实现,实现父类Paticipant类中的方法。
2、代码
1、抽象聊天室类AbstractChatroom及其具体聊天室Chatroom
/// <summary>
/// The 'Mediator' abstract class
/// </summary>
abstract class AbstractChatroom
{
public abstract void Register(Participant participant);
public abstract void Send(string from, string to, string message);
}
/// <summary>
/// The 'ConcreteMediator' class
/// </summary>
class Chatroom : AbstractChatroom
{
private Dictionary<string, Participant> _participants =new Dictionary<string, Participant>();
public override void Register(Participant participant)
{
if (!_participants.ContainsValue(participant))
{
_participants[participant.Name] = participant;
}
participant.Chatroom = this;
}
public override void Send(string from, string to, string message)
{
Participant participant = _participants[to];
if (participant != null)
{
participant.Receive(from, message);
}
}
}
2、参与者Participant类及其实现Beatle、NonBeatle
/// <summary>
/// The 'AbstractColleague' class
/// </summary>
class Participant
{
private Chatroom _chatroom;
private string _name;
// Constructor
public Participant(string name)
{
this._name = name;
}
// Gets participant name
public string Name
{
get { return _name; }
}
// Gets chatroom
public Chatroom Chatroom
{
set { _chatroom = value; }
get { return _chatroom; }
}
// Sends message to given participant
public void Send(string to, string message)
{
_chatroom.Send(_name, to, message);
}
// Receives message from given participant
public virtual void Receive(string from, string message)
{
Console.WriteLine("{0} to {1}: '{2}'",from, Name, message);
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class Beatle : Participant
{
// Constructor
public Beatle(string name)
: base(name)
{
}
public override void Receive(string from, string message)
{
Console.Write("To a Beatle: ");
base.Receive(from, message);
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class NonBeatle : Participant
{
// Constructor
public NonBeatle(string name)
: base(name)
{
}
public override void Receive(string from, string message)
{
Console.Write("To a non-Beatle: ");
base.Receive(from, message);
}
}
3、客户端代码
static void Main(string[] args)
{
// Create chatroom
Chatroom chatroom = new Chatroom();
// Create participants and register them
Participant George = new Beatle("George");
Participant Paul = new Beatle("Paul");
Participant Ringo = new Beatle("Ringo");
Participant John = new Beatle("John");
Participant Yoko = new NonBeatle("Yoko");
chatroom.Register(George);
chatroom.Register(Paul);
chatroom.Register(Ringo);
chatroom.Register(John);
chatroom.Register(Yoko);
// Chatting participants
Yoko.Send("John", "Hi John!");
Paul.Send("Ringo", "All you need is love");
Ringo.Send("George", "My sweet Lord");
Paul.Send("John", "Can't buy me love");
John.Send("Yoko", "My sweet love");
// Wait for user
Console.ReadKey();
}
3、运行结果
五、总结(Summary)
中介者模式(Mediator Pattern),定义一个中介对象来封装系列对象之间的交互。中介者使各个对象不需要显示地相互引用,从而使其耦合性松散,而且可以独立地改变他们之间的交互。中介者模式一般应用于一组对象以定义良好但是复杂的方法进行通信的场合,以及想定制一个分布在多个类中的行为,而不想生成太多的子类的场合。