例一:LeetCode225
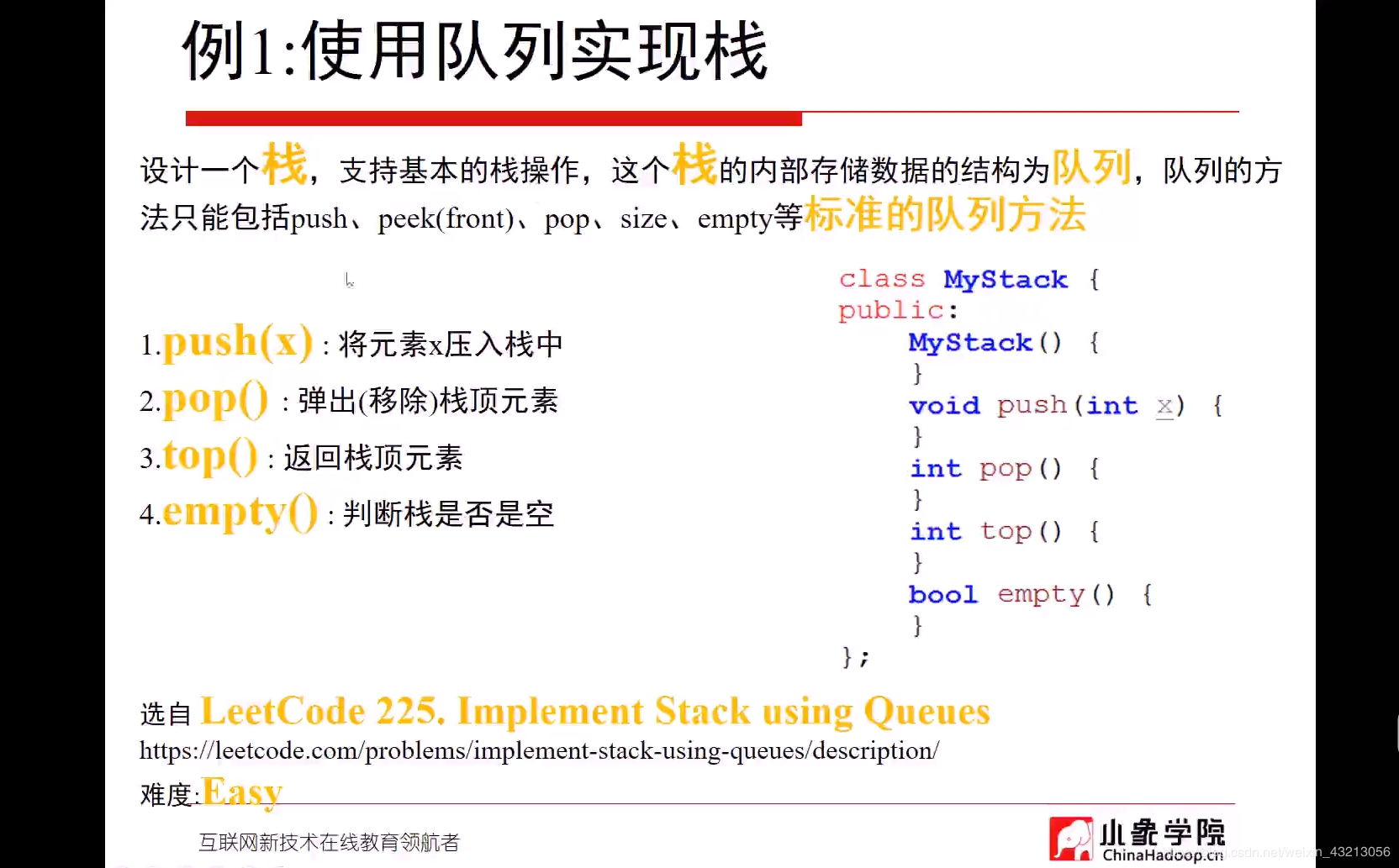
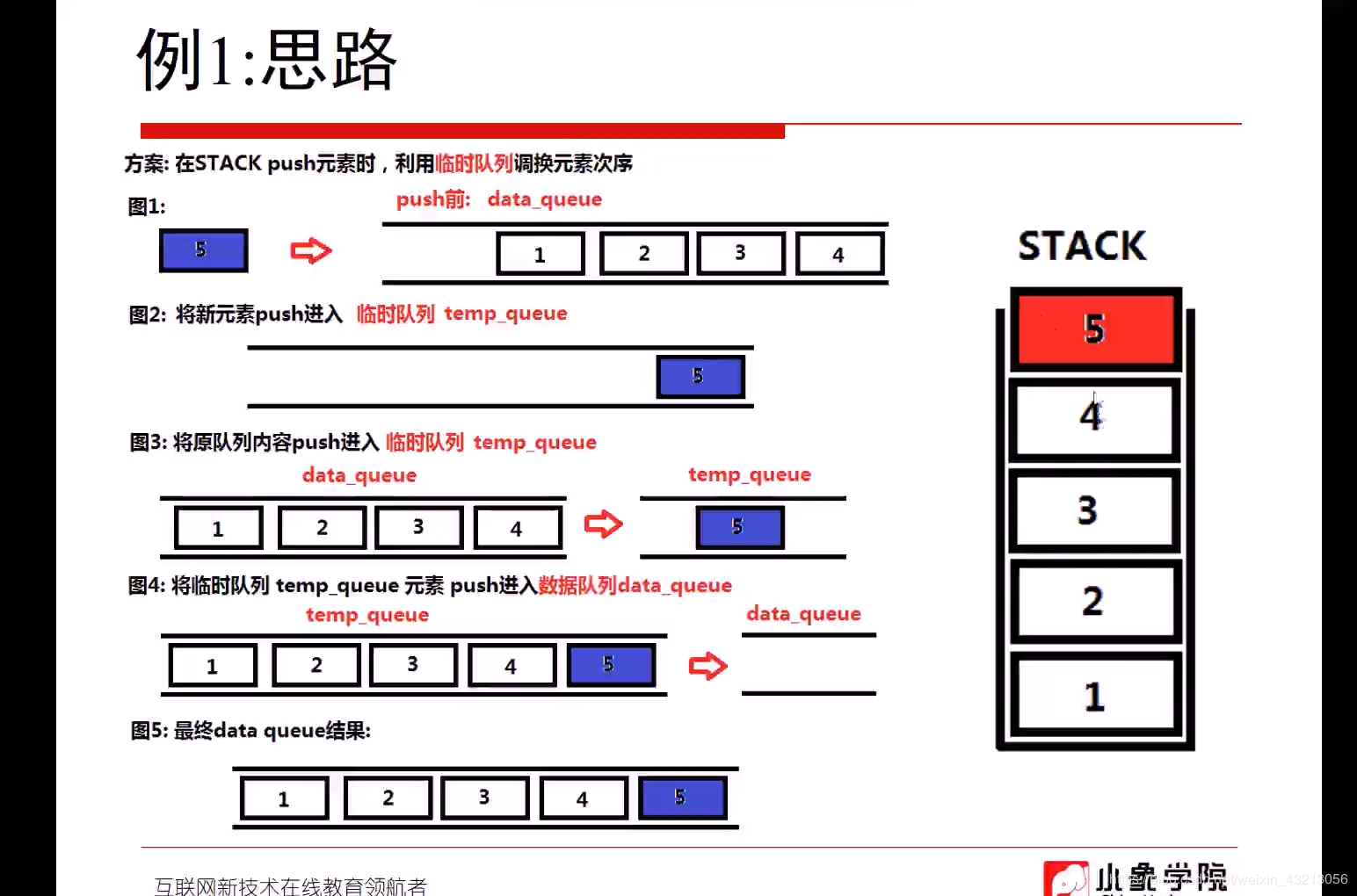
#include <iostream>
#include <queue>
using namespace std;
class Mystack {
public:
Mystack() {
}
void push(int x) {
queue<int>temp_queue;
temp_queue.push(x);//将新元素push进临时队列
while (!_data.empty()) {//将原队列push进临时队列
temp_queue.push(_data.front());
_data.pop();
}
while (!temp_queue.empty()) {//将临时队列push进data队列
_data.push(temp_queue.front());
temp_queue.pop();
}
}
int pop() {
int x = _data.front();
_data.pop();
return x;
}
int top() {
return _data.front();
}
bool empty() {
return _data.empty();
}
private:
queue<int>_data;
};
int main() {
Mystack S;
S.push(1);
S.push(2);
S.push(3);
S.push(4);
printf("%d\n", S.top());
S.pop();
printf("%d\n", S.top());
S.pop();
printf("%d\n", S.top());
return 0;
}
例二:LeetCode232
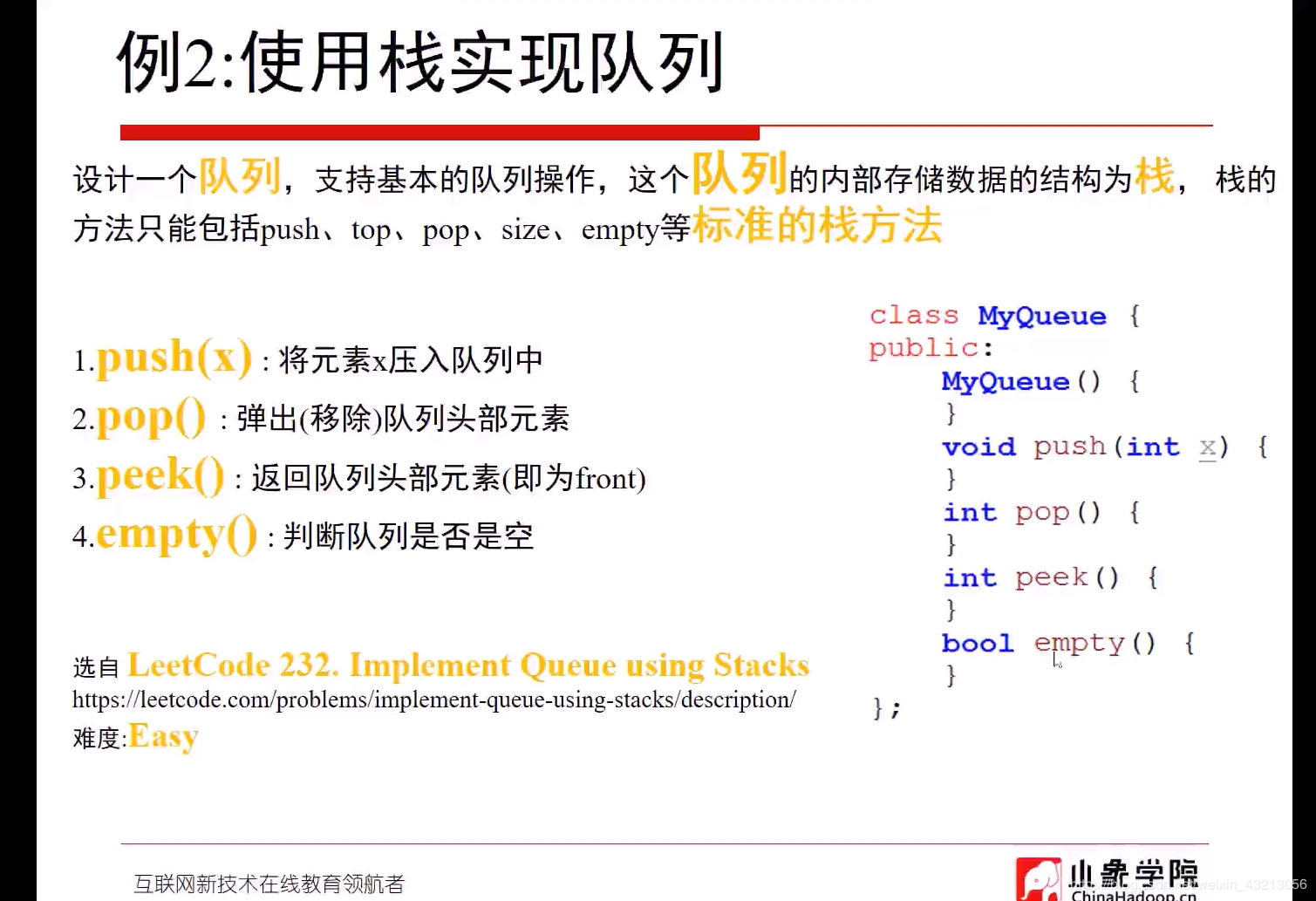
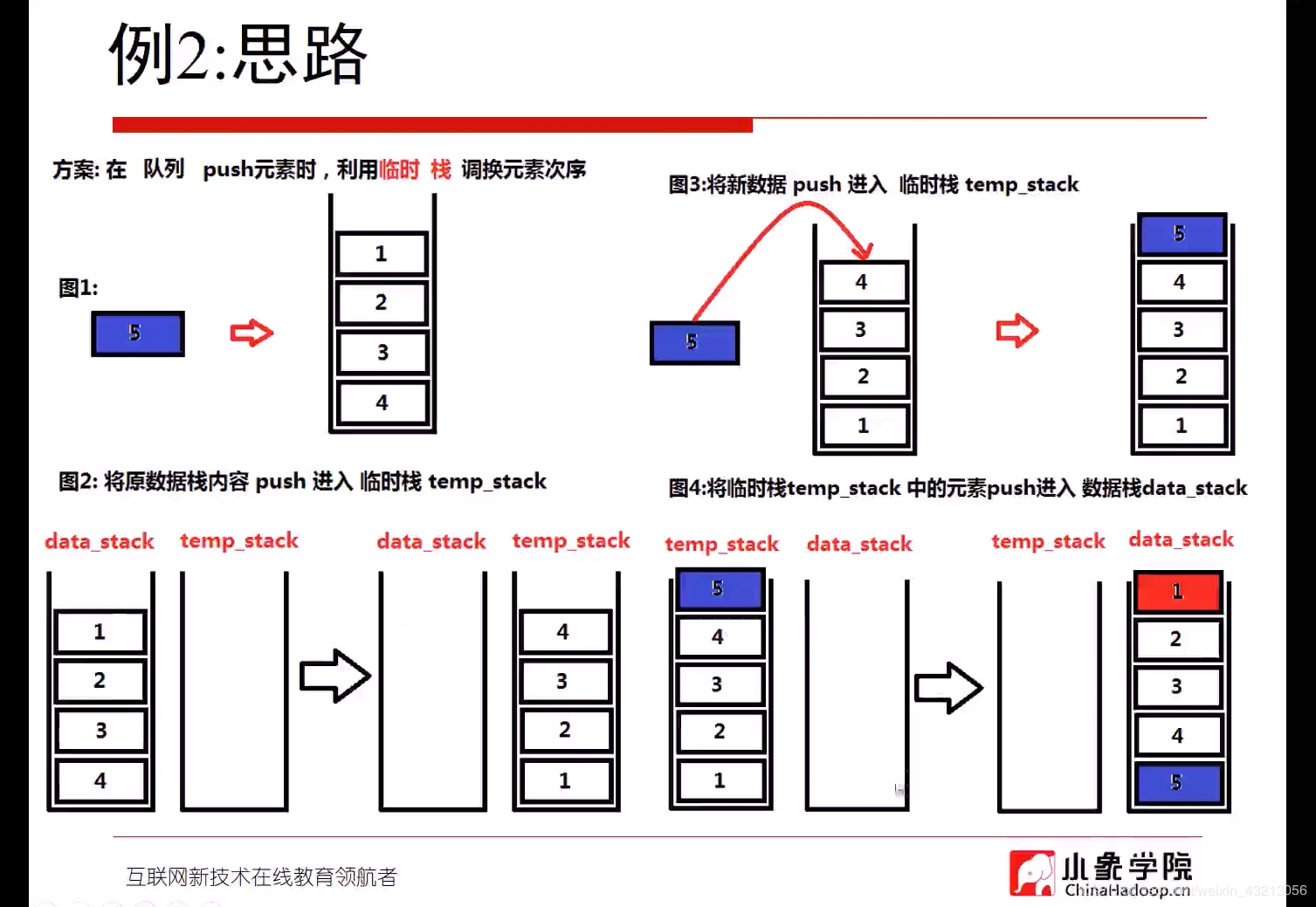
#include <iostream>
#include <stack>
using namespace std;
class MyQueue {
public:
MyQueue() {
}
void push(int x) {
stack <int> temp_stack;
while (!_data.empty()) {
temp_stack.push(_data.top());
_data.pop();
}
temp_stack.push(x);
while (!temp_stack.empty()) {
_data.push(temp_stack.top());
temp_stack.pop();
}
}
int pop() {
int x = _data.top();
_data.pop();
return x;
}
int peek() {
return _data.top();
}
bool empty() {
return _data.empty();
}
private:
stack <int> _data;
};
int main() {
MyQueue Q;
Q.push(1);
Q.push(2);
Q.push(3);
Q.push(4);
printf("%d\n", Q.peek());
Q.pop();
printf("%d\n", Q.peek());
return 0;
}
例三:LeetCode155
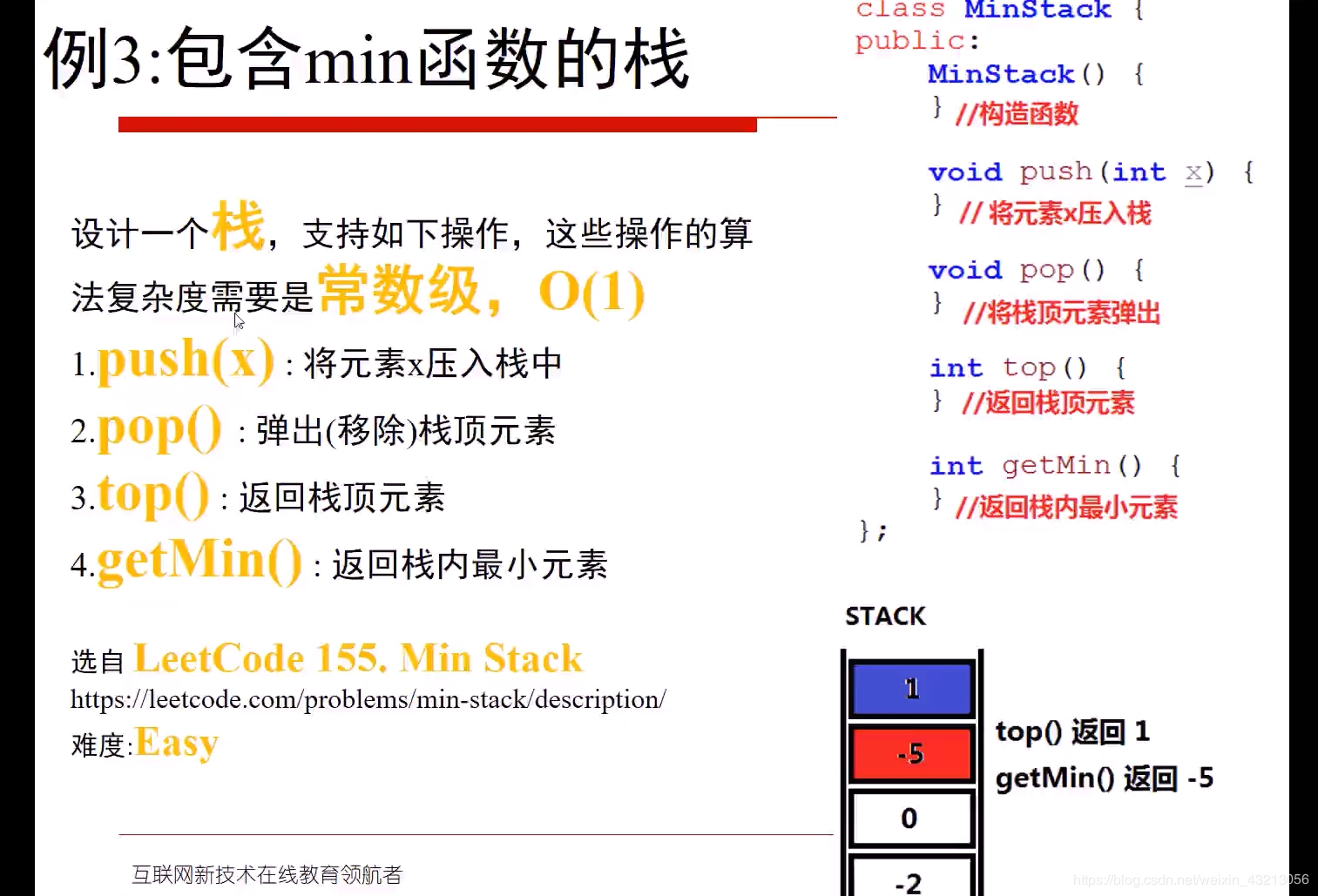
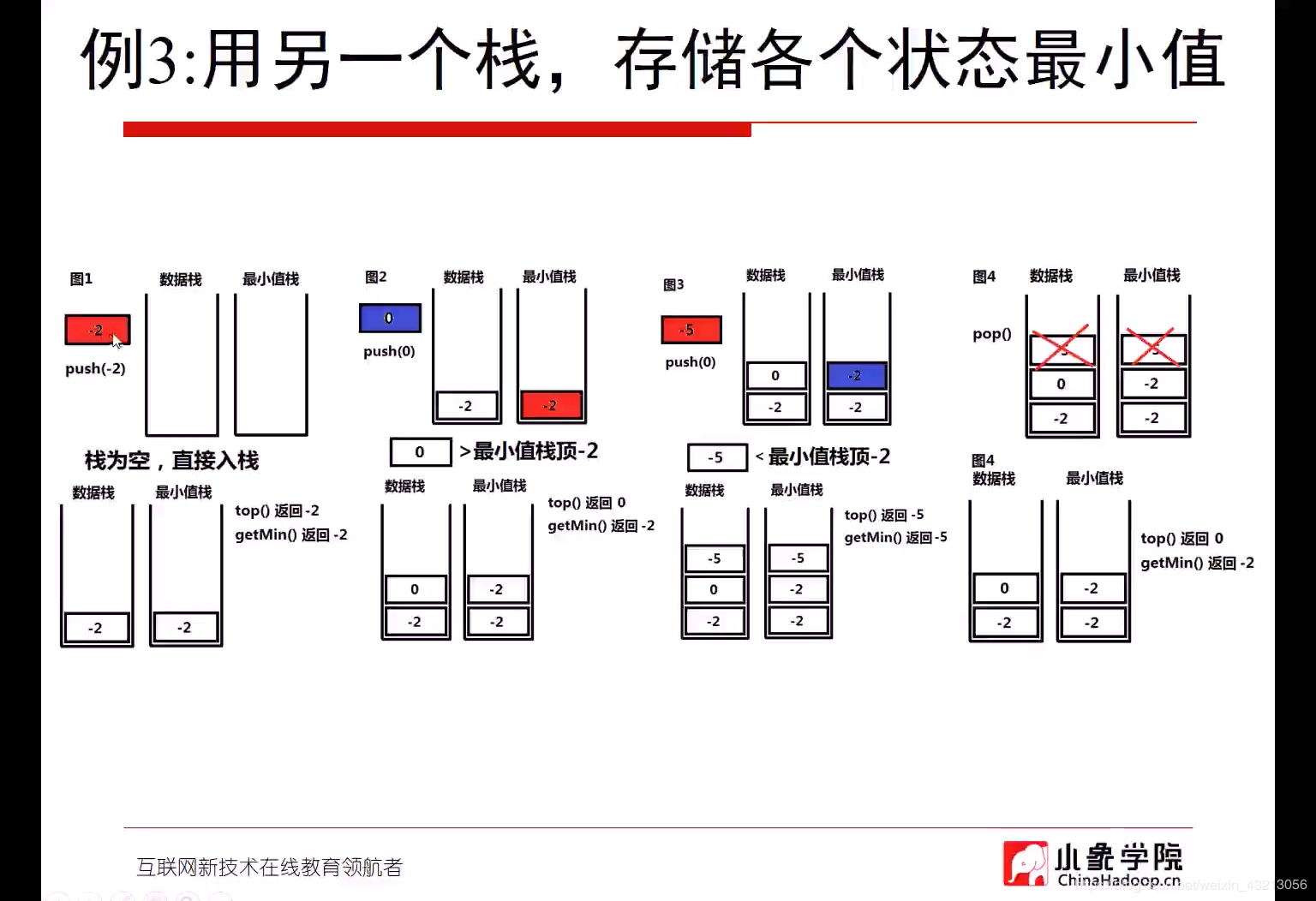
//在栈内找最小值 时间复杂度为O(1)
#include <iostream>
#include <stack>
class MinStack {
public:
void push(int x) {
_data.push(x);
if (_min.empty()) {
_min.push(x);
}
else {
if (x > _min.top()) {
x = _min.top();
}
_min.push(x);
}
}
void pop() {
_data.pop();
_min.pop();
}
int top() {
return _data.top();
}
int getMin() {
return _min.top();
}
private:
std::stack<int> _data;
std::stack<int> _min;
};
int main() {
MinStack minStack;
minStack.push(-2);
printf("top = [%d]\n", minStack.top());
printf("min = [%d]\n\n", minStack.getMin());
minStack.push(0);
printf("top = [%d]\n", minStack.top());
printf("min = [%d]\n\n", minStack.getMin());
minStack.push(-5);
printf("top = [%d]\n", minStack.top());
printf("min = [%d]\n\n", minStack.getMin());
minStack.pop();
printf("top = [%d]\n", minStack.top());
printf("min = [%d]\n\n", minStack.getMin());
return 0;
}
例四:POJ1363
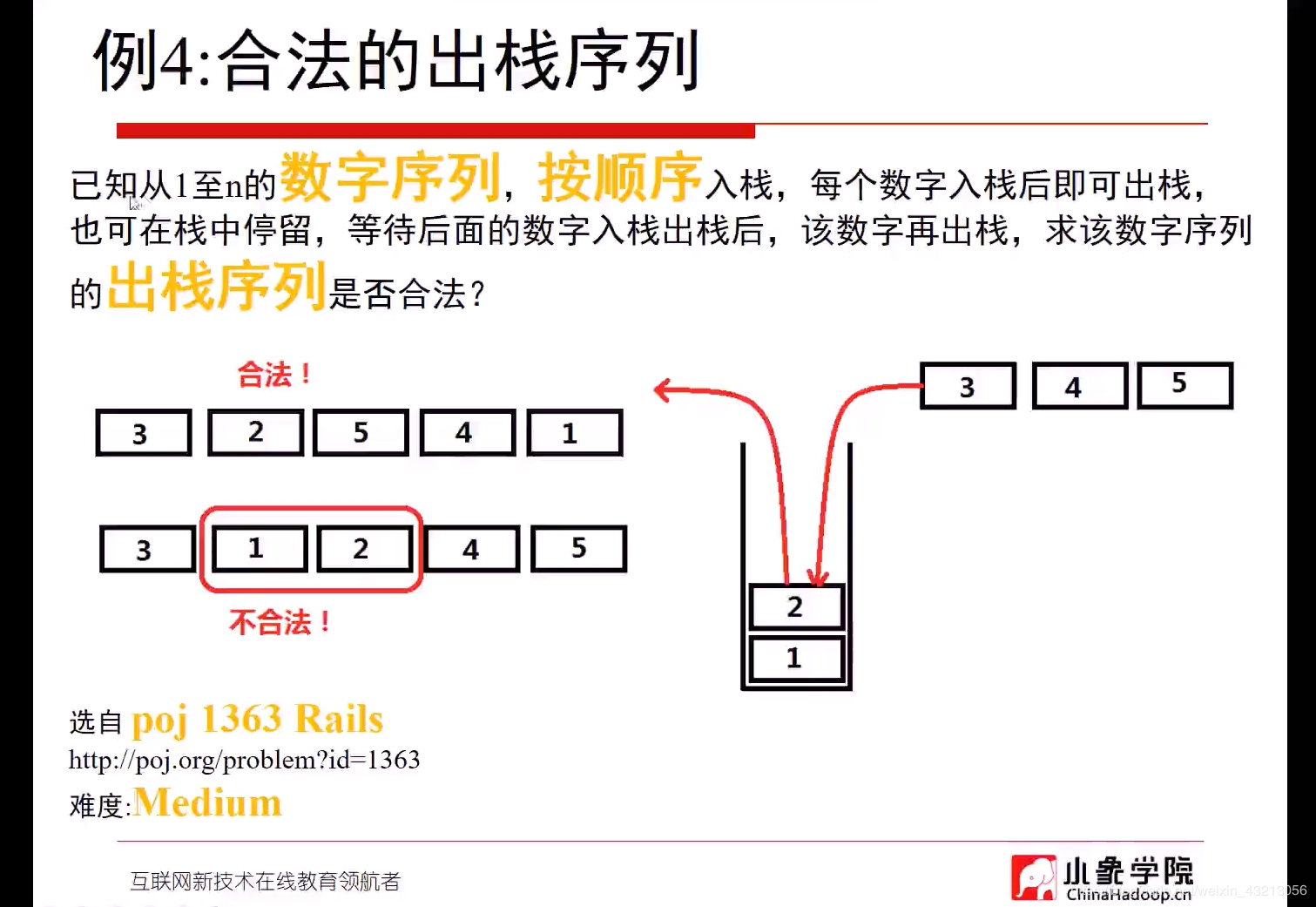
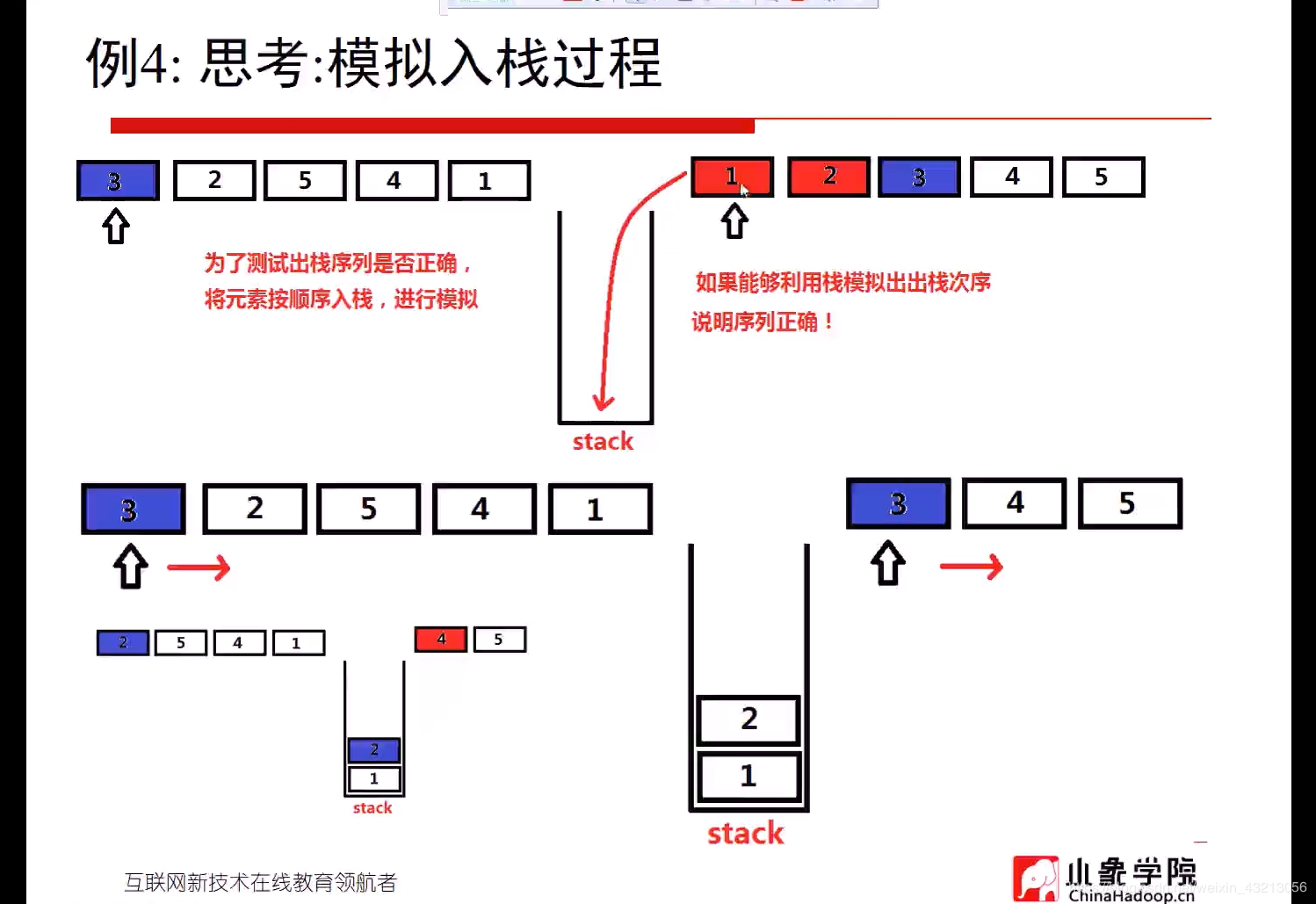
#include <stack>
#include <queue>
#include <stdio.h>
using namespace std;
bool check_is_valid_order(queue<int>& order) {
stack<int> S;
int n = order.size();
for (int i = 1; i <= n; i++) {
S.push(i);
while (!S.empty() && order.front() == S.top()) {
S.pop();
order.pop();
}
}
if (!S.empty()) {
return false;
}
return true;
}
int main() {
int n;
int train;
~scanf("%d", &n);
while (n) {
~scanf("%d", &train);
while (train) {
queue<int>order;
order.push(train);
for (int i = 1; i < n; i++) {
~scanf("%d", &train);
order.push(train);
}
if (check_is_valid_order(order)) {
printf("Yes\n");
}
else {
printf("No\n");
}
~scanf("%d", &train);
}
printf("\n");
~scanf("%d", &n);
}
return 0;
}
例五:LeetCode224
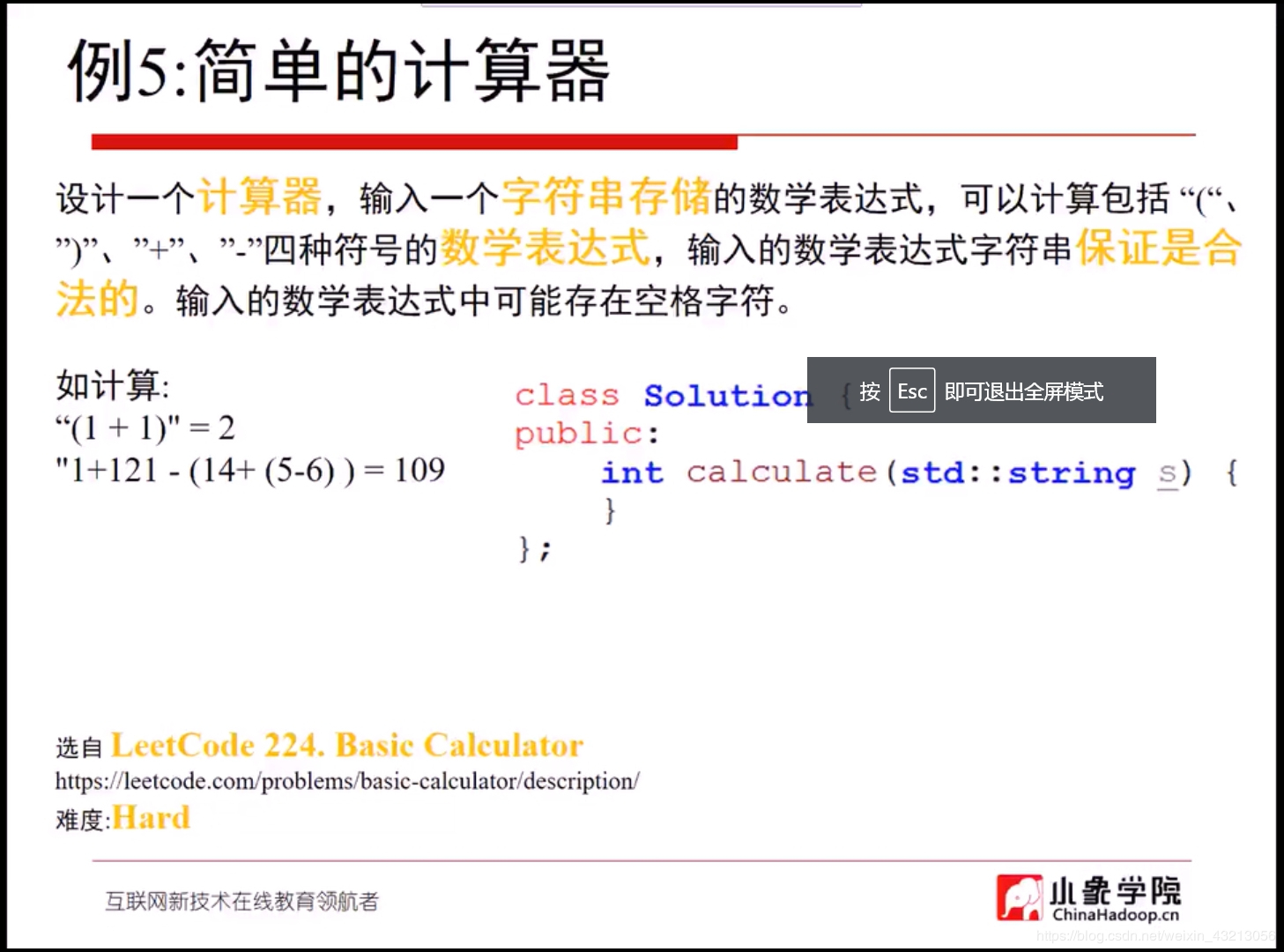
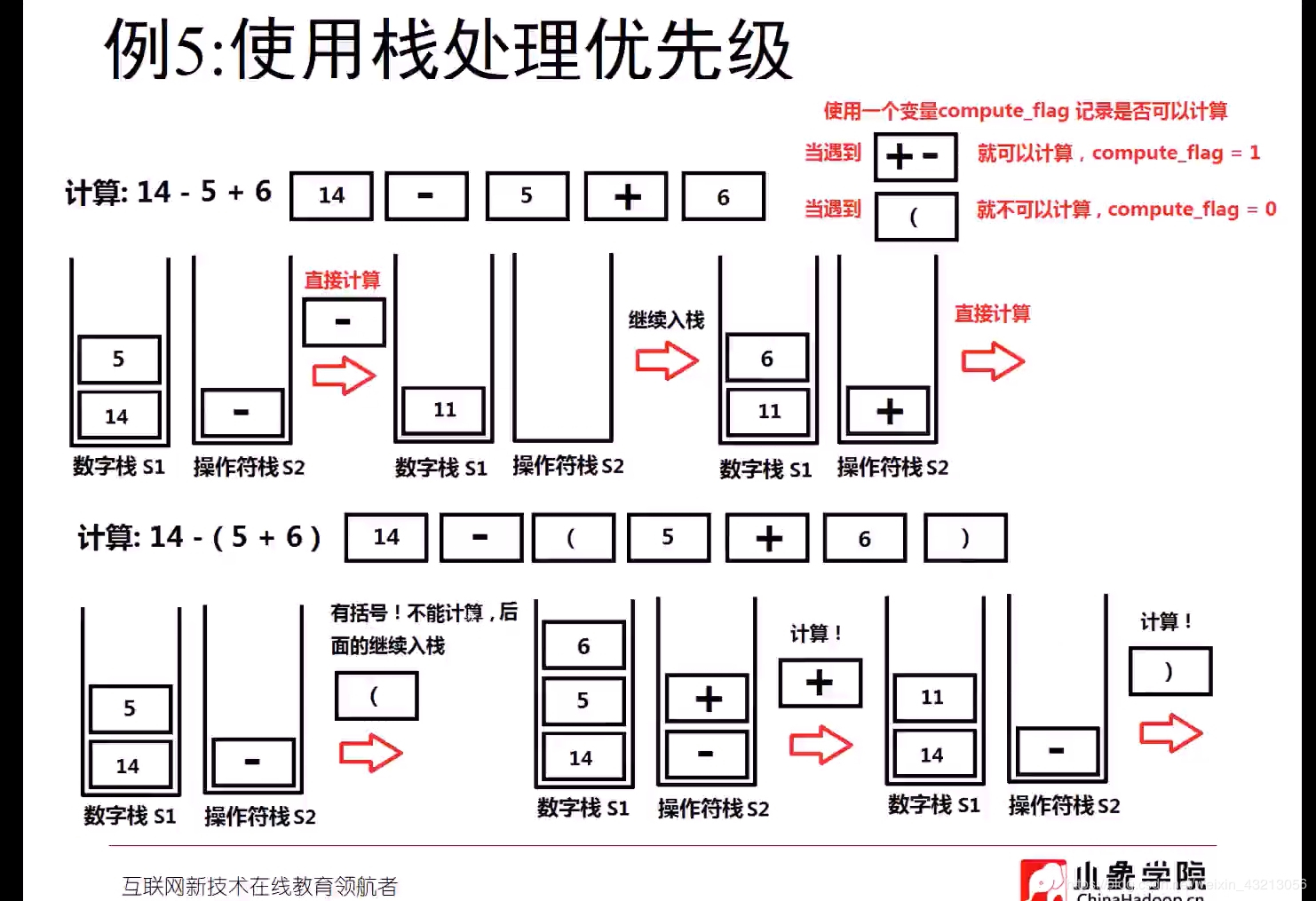
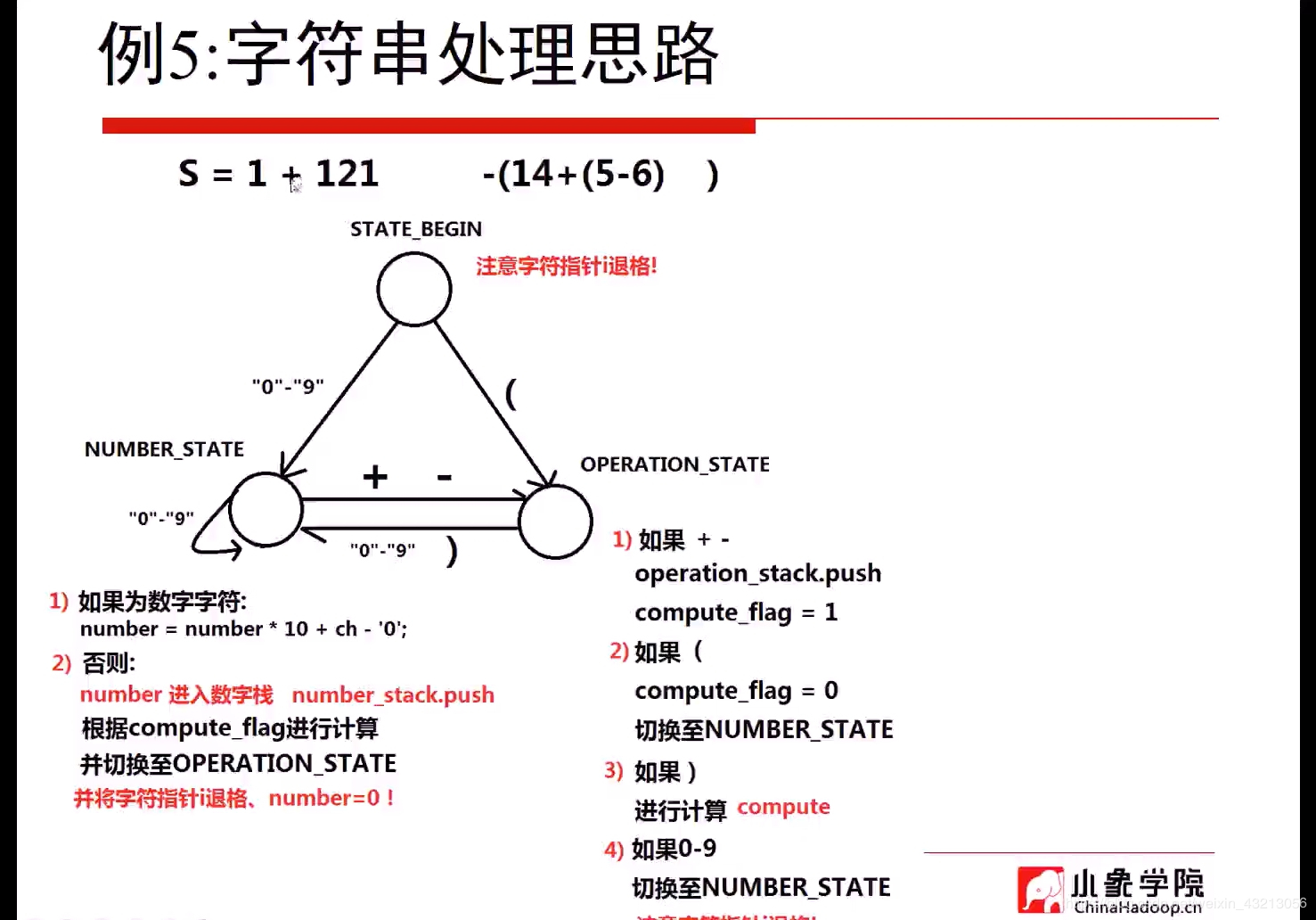
#include <stdio.h>
#include <string>
#include <stack>
class Solution {
public:
int calculate(std::string s) {
static const int STATE_BEGINI = 0;
static const int NUMBER_STATE = 1;
static const int OPERATION_STATE = 2;
std::stack<int> number_stack;
std::stack<char> operation_stack;
long long number = 0; // 用 int 通过不了所有测试数据
int STATE = STATE_BEGINI;
int computer_flag = 0;
for (int i = 0; i < s.length(); i++) {
if (s[i] == ' ') {
continue;
}
switch (STATE) {
case STATE_BEGINI:
if (s[i] >= '0' && s[i] <= '9') {
STATE = NUMBER_STATE;
}
else {
STATE = OPERATION_STATE;
}
i--;
break;
case NUMBER_STATE:
if (s[i] >= '0' && s[i] <= '9') {
number = number * 10 + s[i] - '0';
}
else {
number_stack.push(number);
if (computer_flag == 1) {
compute(number_stack, operation_stack);
}
number = 0;
i--;
STATE = OPERATION_STATE;
}
break;
case OPERATION_STATE:
if (s[i] == '+' || s[i] == '-') {
operation_stack.push(s[i]);
computer_flag = 1;
}
else if (s[i] == '(') {
STATE = NUMBER_STATE;
computer_flag = 0;
}
else if (s[i] >= '0' && s[i] <= '9') {
STATE = NUMBER_STATE;
i--;
}
else if (s[i] == ')') {
compute(number_stack, operation_stack);
}
break;
}
}
if (number != 0) {
number_stack.push(number);
compute(number_stack, operation_stack);
}
if (number == 0 && number_stack.empty()) {
return 0;
}
return number_stack.top();
}
private:
void compute(std::stack<int>& number_stack, std::stack<char>& operation_stack) {
if (number_stack.size() < 2) {
return;
}
int num2 = number_stack.top();
number_stack.pop();
int num1 = number_stack.top();
number_stack.pop();
if (operation_stack.top() == '+') {
number_stack.push(num1 + num2);
}
else if (operation_stack.top() == '-') {
number_stack.push(num1 - num2);
}
operation_stack.pop();
}
};
int main() {
std::string s = "1+121 - (14+(5-6) )";
Solution solve;
printf("%d\n", solve.calculate(s));
return 0;
}
例六:LeetCode215
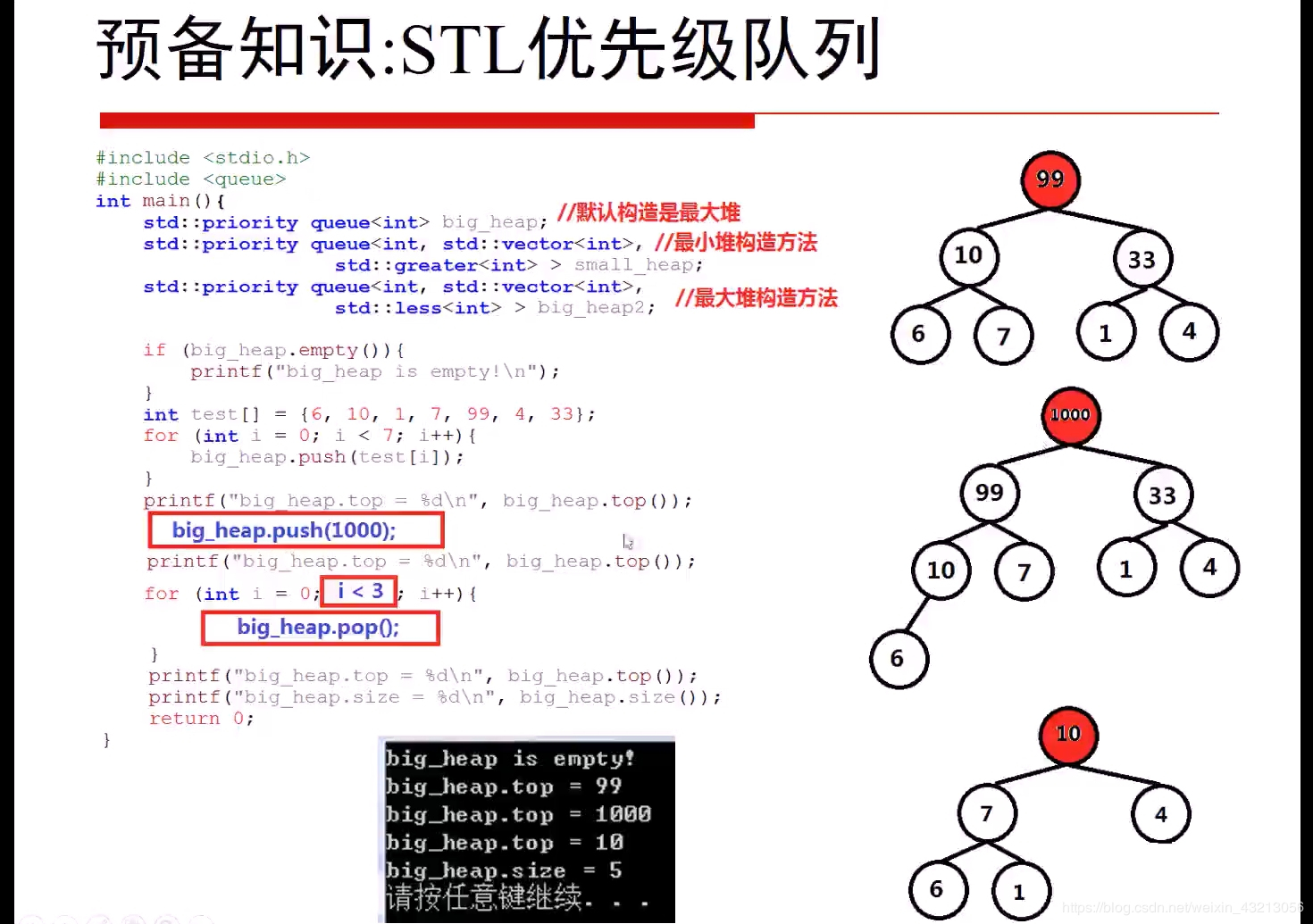
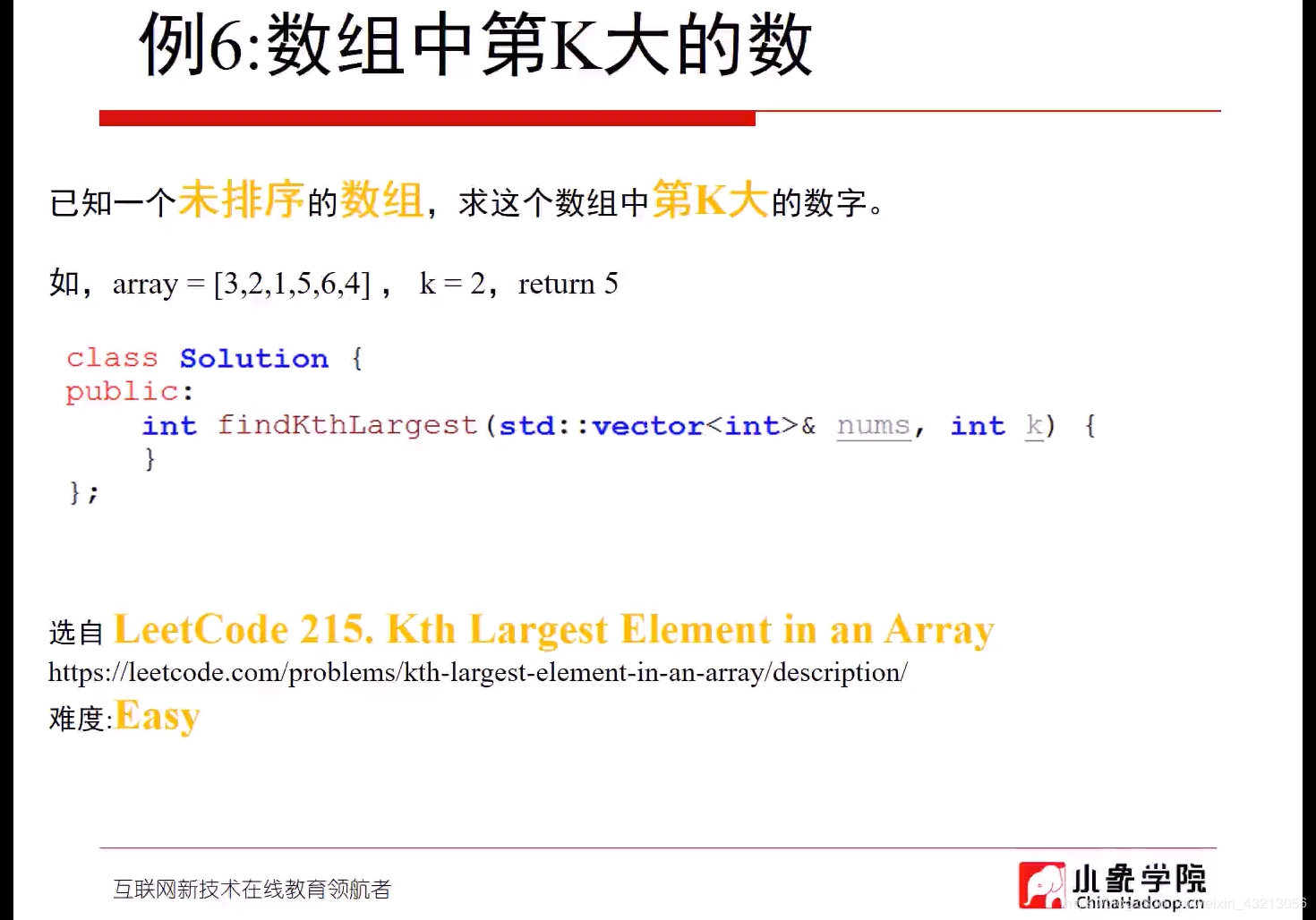
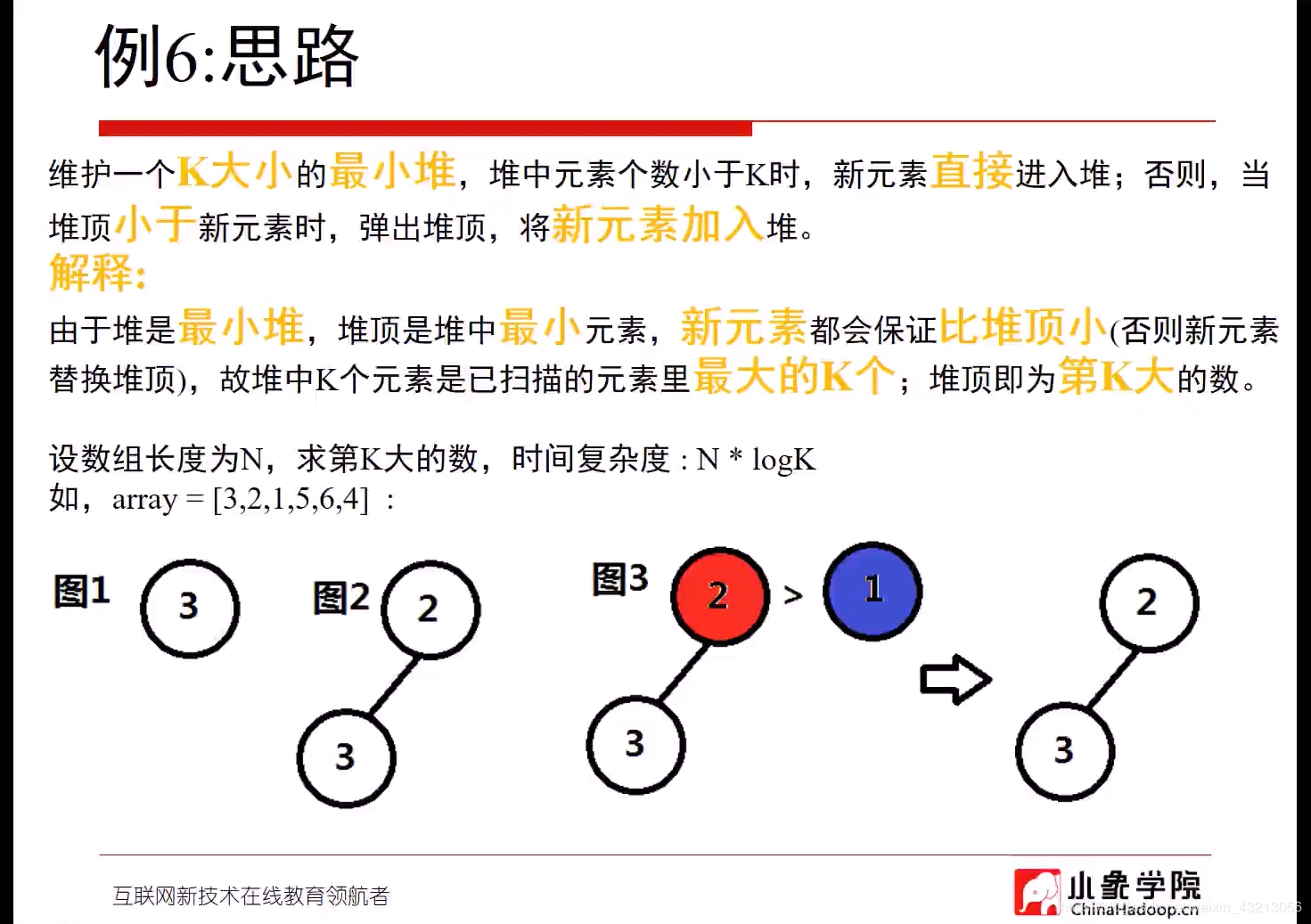
//利用最小堆求第K大的数 n*logK
#include <vector>
#include <queue>
#include <cstdio>
class Solution {
public:
int findKthLargest(std::vector<int>& nums, int k) {
std::priority_queue <int, std::vector<int>, std::greater<int>> Q;
for (int i = 0; i < nums.size(); i++) {
if (Q.size() < k) {
Q.push(nums[i]);
}
else if (Q.top() < nums[i]) {
Q.pop();
Q.push(nums[i]);
}
}
return Q.top();
}
};
int main() {
std::vector<int> nums;
nums.push_back(3);
nums.push_back(2);
nums.push_back(1);
nums.push_back(5);
nums.push_back(6);
nums.push_back(4);
Solution solve;
printf("%d\n", solve.findKthLargest(nums, 2));
return 0;
}
例七:LeetCode295
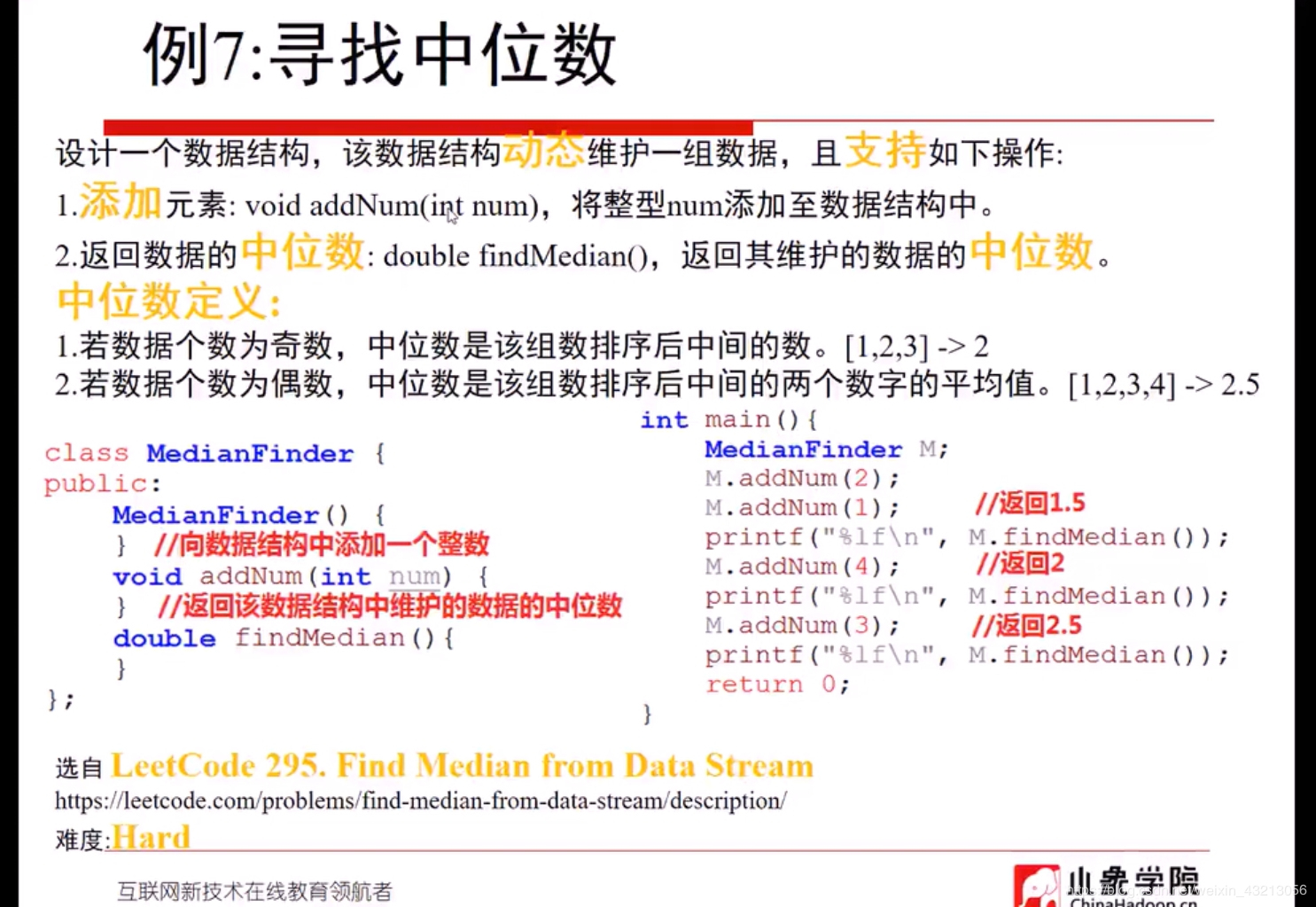
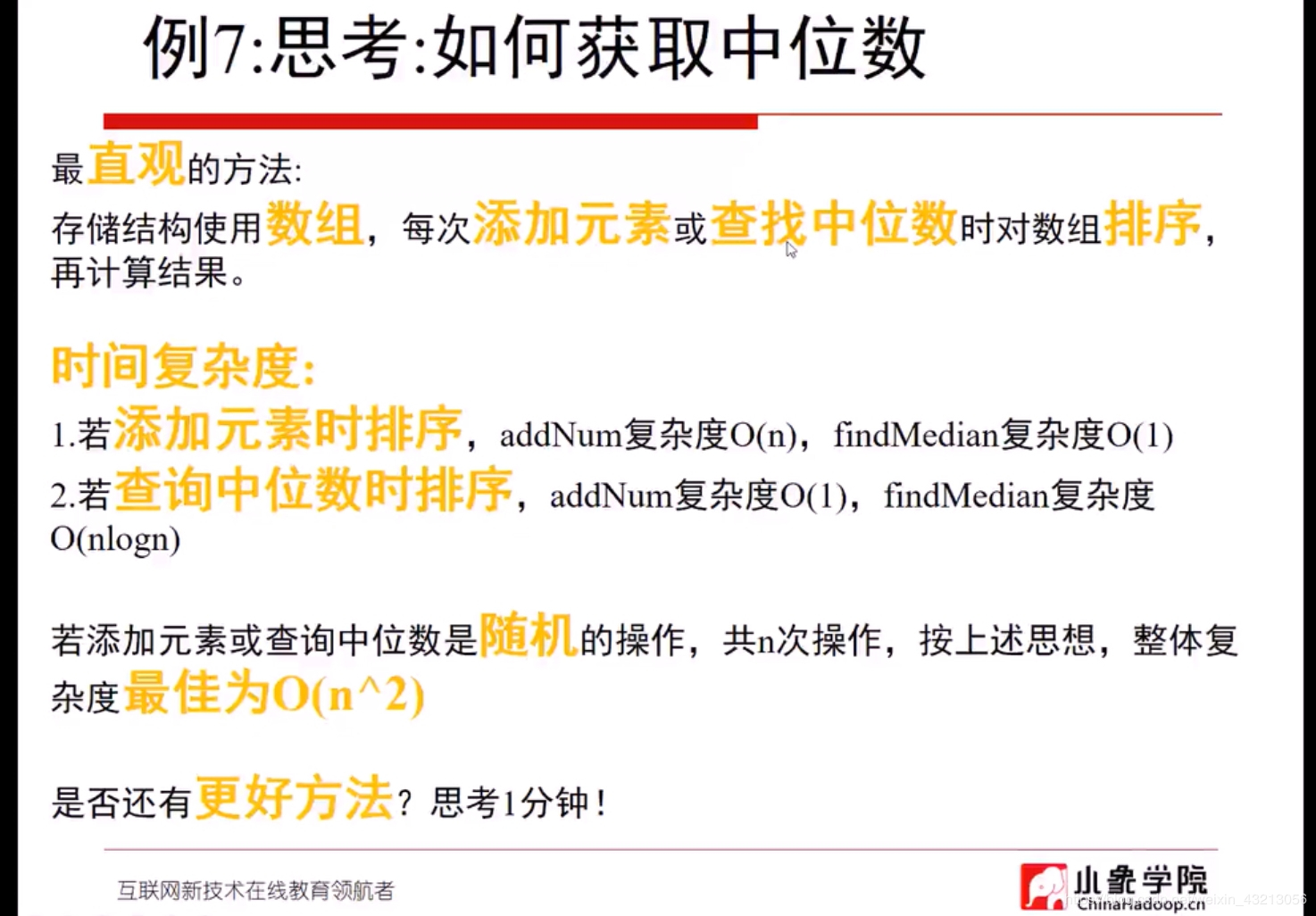
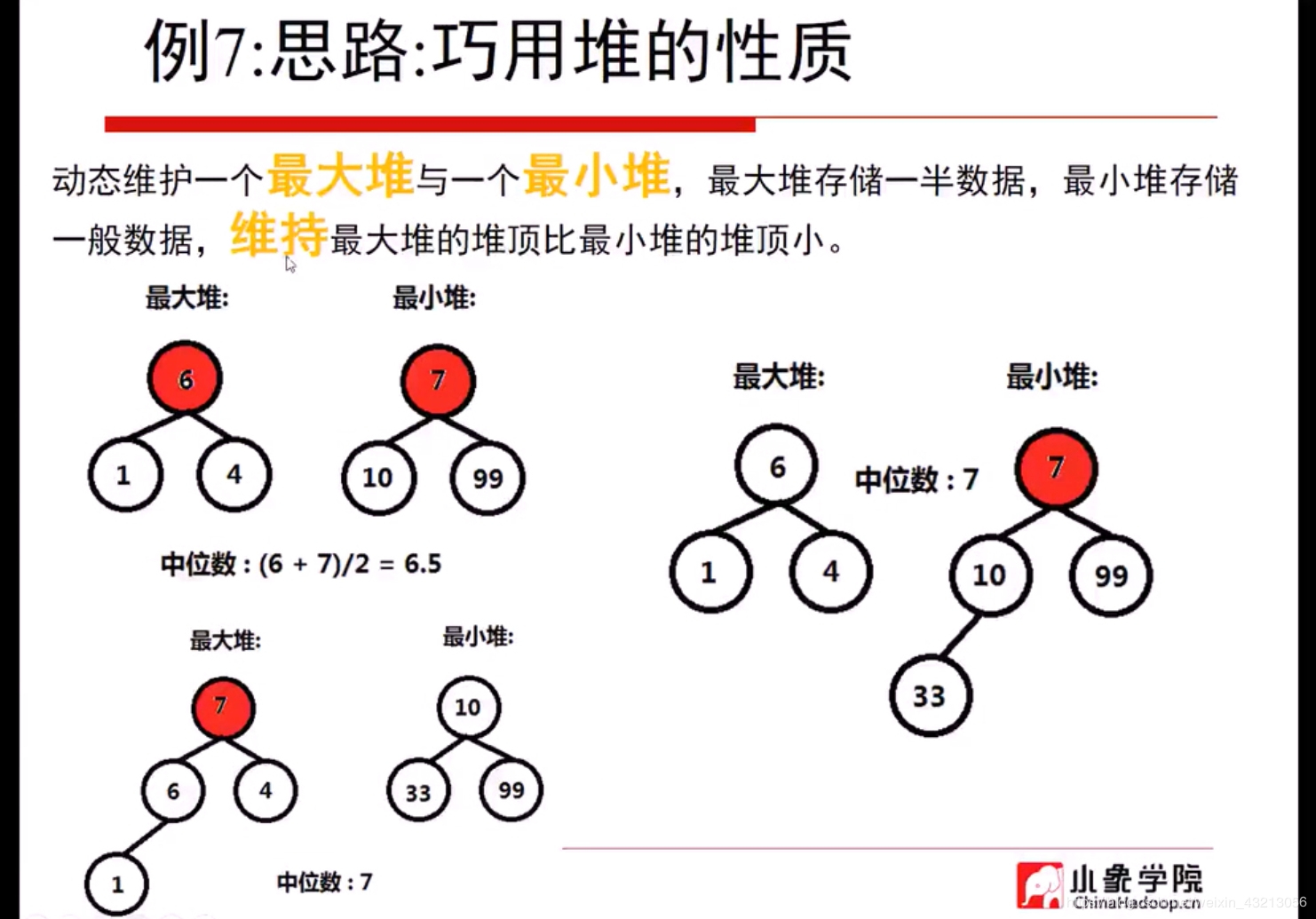
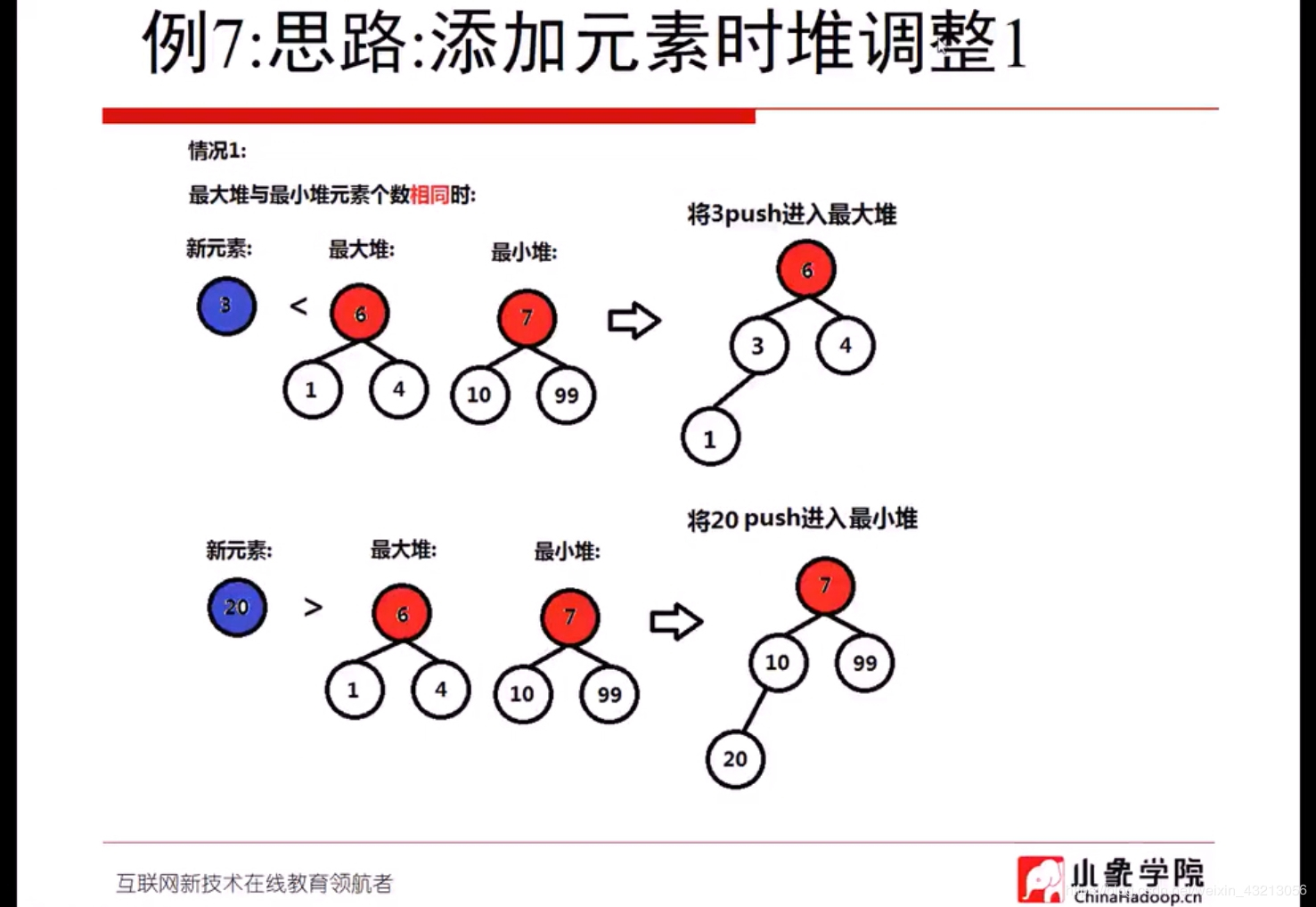
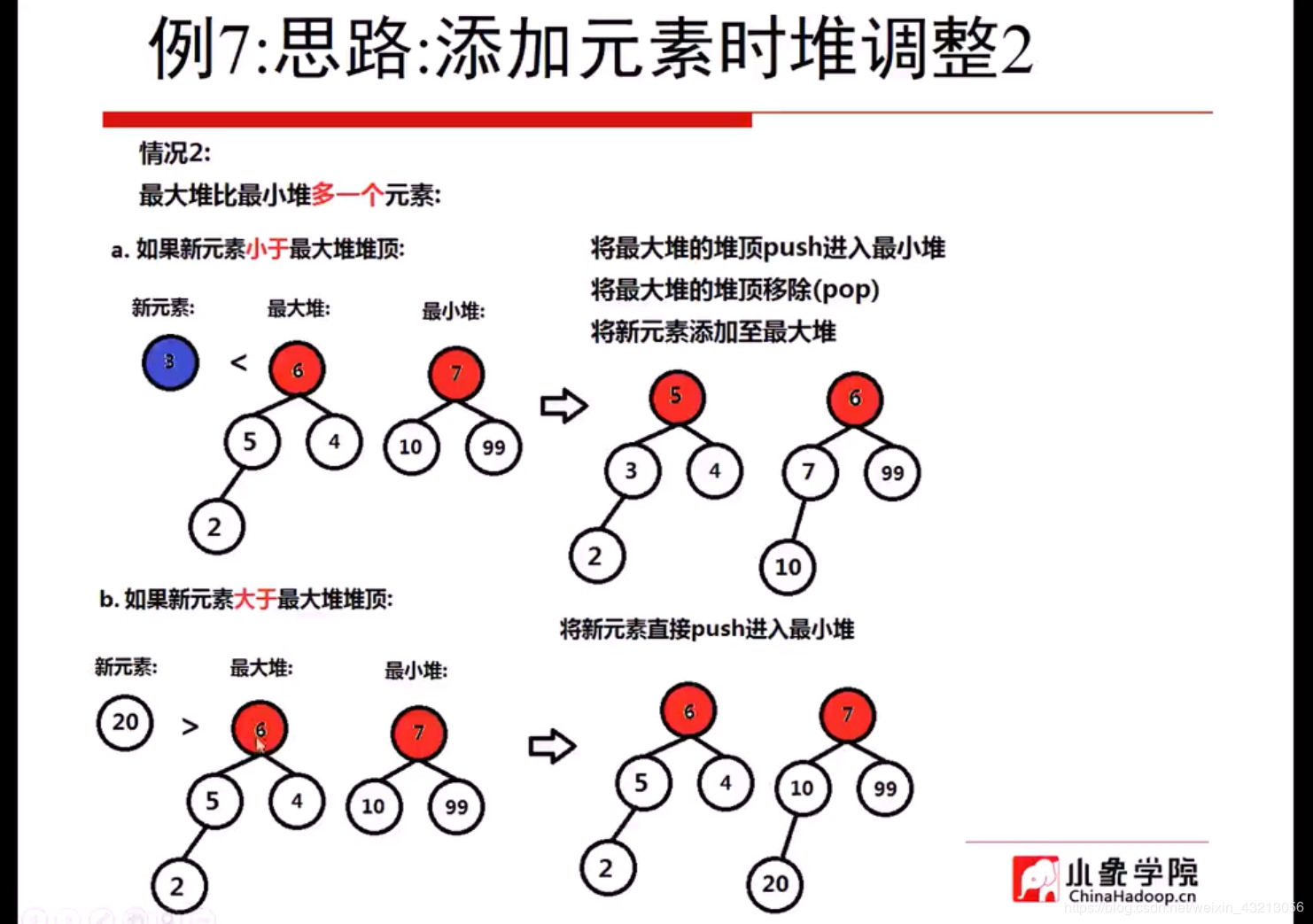
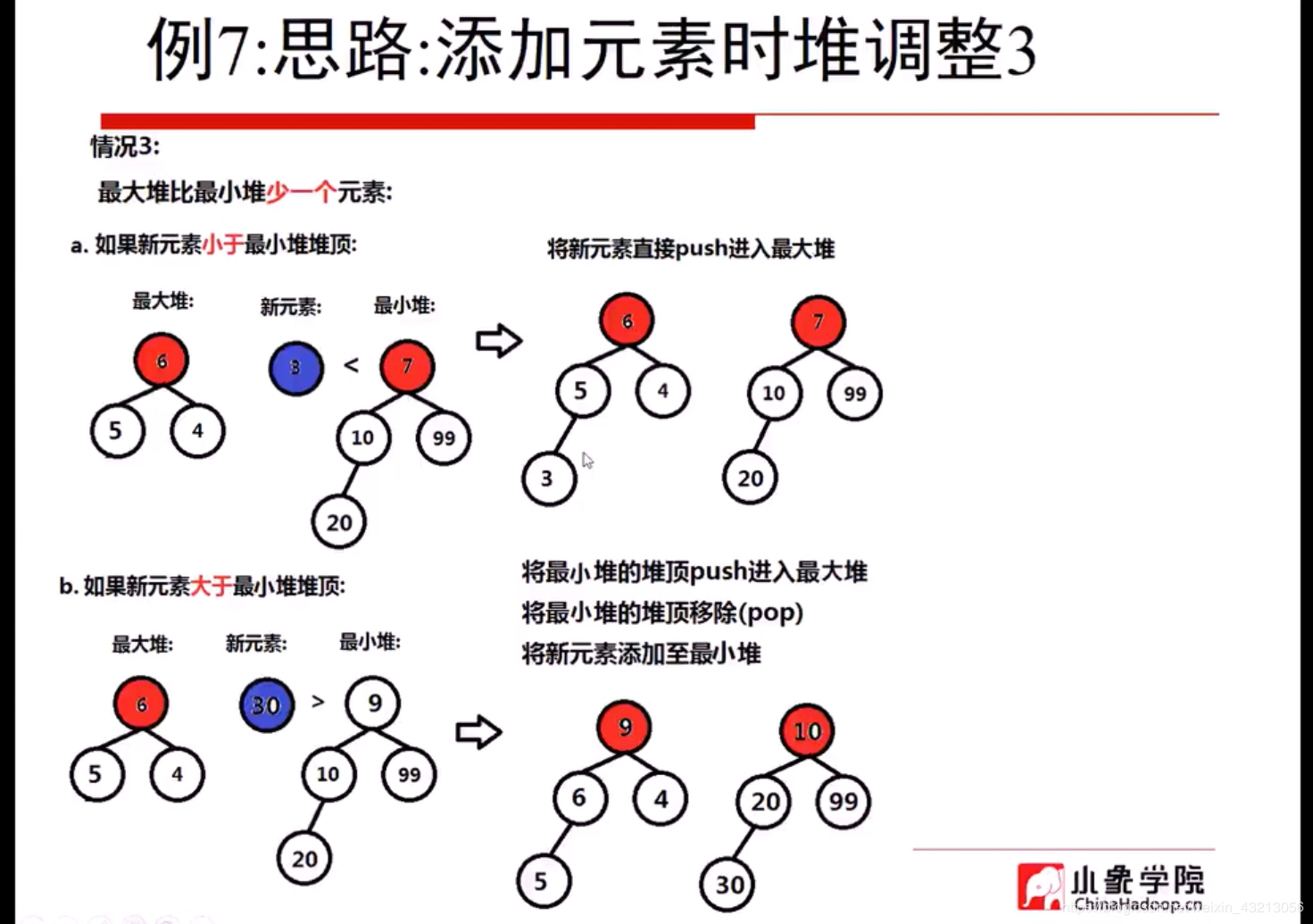
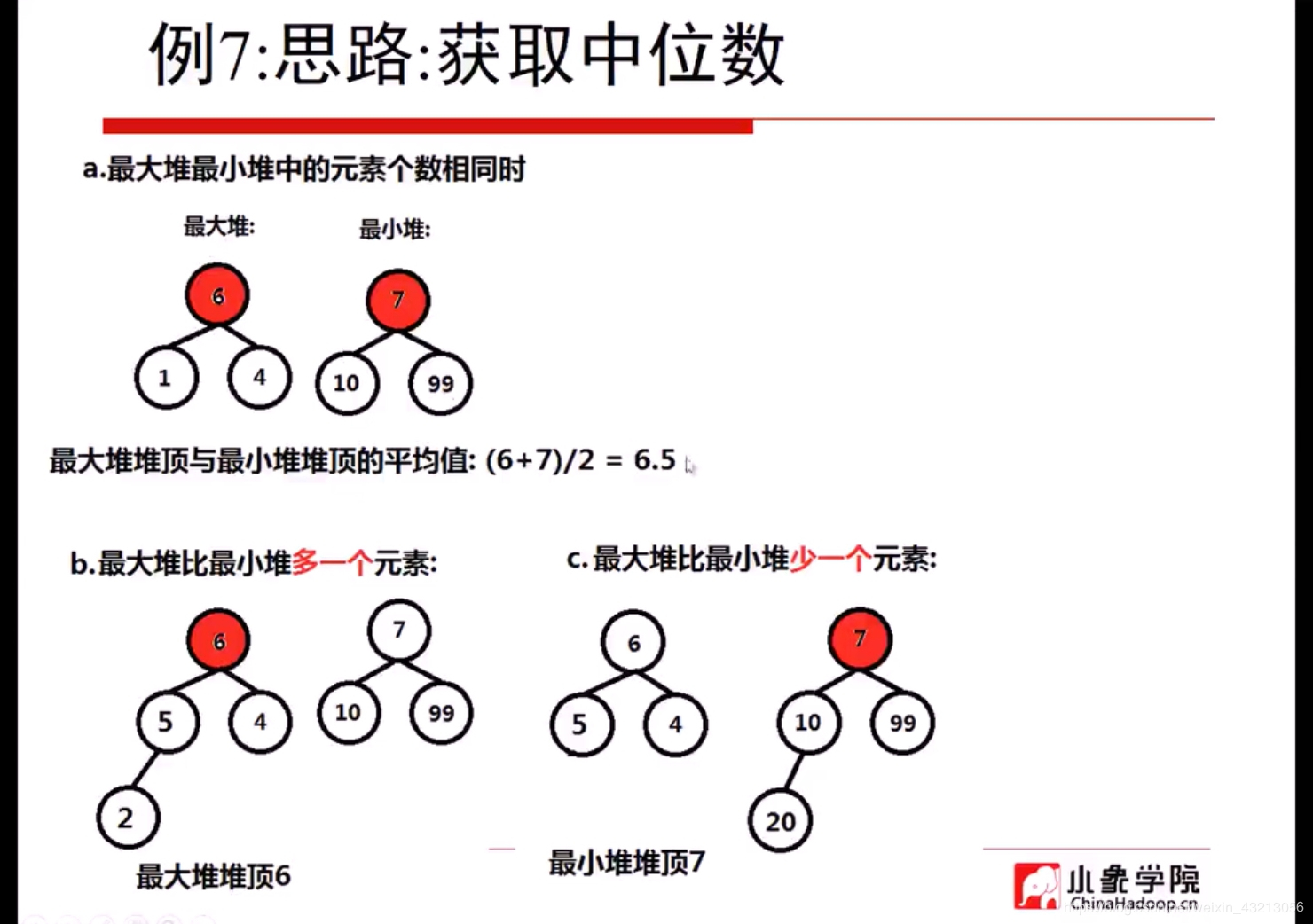
/*设计一个数据结构,动态维护一组数据,且支持如下操作
1、添加元素
2、返回数据的中位数
*/
#include <stdio.h>
#include <queue>
class MedinFinder {
public:
MedinFinder() {}
void addNum(int num) {
if (big_queue.empty()) {
big_queue.push(num);
return;
}
if (big_queue.size() == small_queue.size()) {
if (num < big_queue.top()) {
big_queue.push(num);
}
else {
small_queue.push(num);
}
}
else if (big_queue.size() > small_queue.size()) {
if (num > big_queue.top()) {
small_queue.push(num);
}
else {
small_queue.push(big_queue.top());
big_queue.pop();
big_queue.push(num);
}
}
else if (big_queue.size() < small_queue.size()) {
if (num < small_queue.top()) {
big_queue.push(num);
}
else {
big_queue.push(small_queue.top());
small_queue.pop();
small_queue.push(num);
}
}
}
double findMedian() {
if (big_queue.size() == small_queue.size()) {
return (big_queue.top() + small_queue.top()) / 2;
}
else if (big_queue.size() > small_queue.size()) {
return big_queue.top();
}
return small_queue.top();
}
private:
std::priority_queue <double> big_queue;
std::priority_queue <double, std::vector<double>, std::greater<double>> small_queue;
};
int main() {
MedinFinder M;
int test[] = { 6,10,1,7,99,4,33 };
for (int i = 0; i < 7; i++) {
M.addNum(test[i]);
printf("%lf\n", M.findMedian());
}
return 0;
}