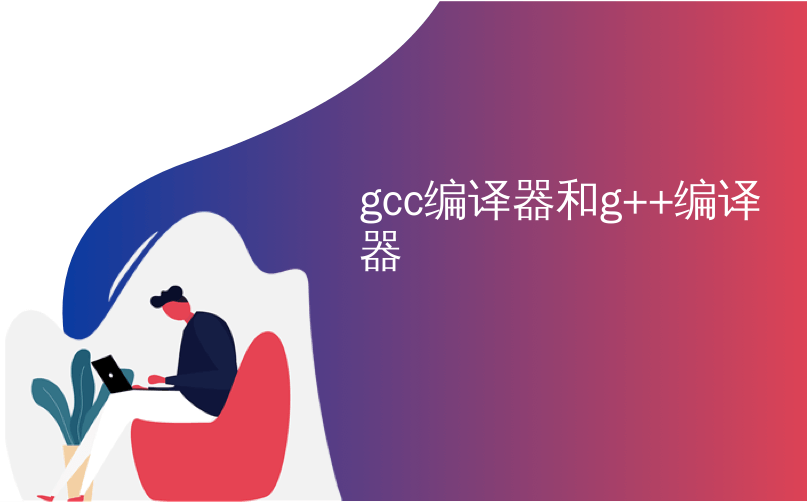
gcc编译器和g++编译器
GCC is de facto compiler UNIX and LINUX Operating Systems. GCC is the acronym of the GNU Compiler Collection. As the name Collection suggest GCC supports C, C++, Java, Ada, Go, etc. In this post, we will look at how to compile C and C++ applications.
GCC实际上是UNIX和LINUX操作系统的编译器。 GCC是GNU编译器集合的首字母缩写。 顾名思义,GCC支持C,C ++,Java,Ada,Go等。在本文中,我们将研究如何编译C和C ++应用程序。
正在安装 (Installing)
By default compiler, related tools are not installed. We can install them from repositories easily like below.
默认情况下,编译器未安装相关工具。 我们可以轻松地从存储库中安装它们,如下所示。
Ubuntu,Debian,Mint,Kali: (Ubuntu, Debian, Mint, Kali:)
$ sudo apt-get install gcc -y
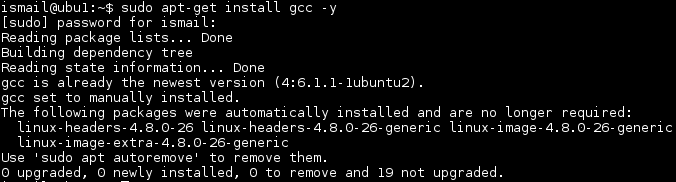
As we see GCC is already installed.
我们看到已经安装了GCC。
CentOS,Fedora,Red Hat: (CentOS, Fedora, Red Hat:)
$ yum install gcc -y
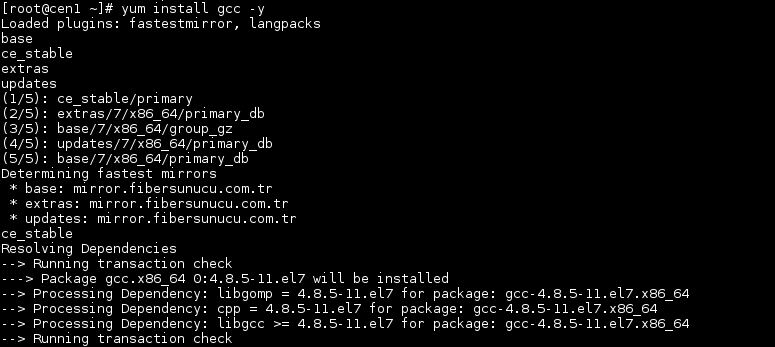
什么正在编译源代码或应用程序(What Is Compiling A Source Code or Application)
Compiling is the process of creating executable files from source code. There are some intermediate states but we do not dive into them. For example to print some messages to the standard output a program is written which is consists of source codes. Then the program is compiled with GCC to create a new executable file that can be run in the Linux. Here is our source code:
编译是从源代码创建可执行文件的过程。 有一些中间状态,但我们不会深入其中。 例如,为了将一些消息打印到标准输出,将编写一个包含源代码的程序。 然后,使用GCC编译程序,以创建可以在Linux中运行的新可执行文件。 这是我们的源代码:
#include <stdio.h>
void main(){
printf("Hi poftut");
}
用GCC编译C源代码 (Compile C Source Code with GCC)
We have the following source code to compile which just prints some text to the standard output.
我们有以下源代码可以编译,仅将一些文本打印到标准输出中。
$ gcc main.c

We have compiled our source code and a binary named a.out is created. Then we executed the new binary and it prints out the message “Hello poftut” .
我们已经编译了源代码,并创建了一个名为a.out的二进制文件。 然后,我们执行了新的二进制文件,并打印出消息“ Hello poftut” 。
设置输出文件名 (Set Output Filename)
By default after compile operation, the created executable file name will be a.out
as we have seen in the previous example. We can specify the executable file name after compilation like below.
在编译操作后,默认情况下,创建的可执行文件名将为a.out
,如上例所示。 我们可以在编译后指定可执行文件的名称,如下所示。
$ gcc main.c -o mybinary

使用GCC可执行调试(Debug Executable with GCC)
If our program has some problems with performance or errors we need to debug it. To debug an application it must be compiled with debug options to add some debug data into the binary files. Keep in mind debug information will be made binary slower and bigger in size.
如果我们的程序在性能方面存在问题或错误,则需要对其进行调试。 要调试应用程序,必须使用调试选项对其进行编译,以将一些调试数据添加到二进制文件中。 请记住,调试信息将使二进制文件变慢并且变大。
$ gcc -g main.c -o mybinary

使用GCC优化代码(Optimize Code with GCC)
In the previous example, we have seen that debug information made the executable slower and higher in size. For the production environment, we need to make the executable more optimized. We can make code more optimized in performance and size with -O
parameters. But keep in mind that in rare situations optimization can make things worst.
在前面的示例中,我们已经看到调试信息使可执行文件的速度变慢且变大。 对于生产环境,我们需要使可执行文件更加优化。 我们可以使用-O
使代码在性能和大小上更加优化 参数。 但是请记住,在极少数情况下,优化会使情况更糟。
$ gcc -O main.c -o mybinary
在使用GCC进行编译时包括库 (Include Libraries During Compilation with GCC)
We have looked at simple source code but in a real-world project, there are a lot of code files and external libraries. We should specify the library we have used in the related code file. We can provide external libraries with -l
parameters.
我们已经看过简单的源代码,但是在实际项目中,有很多代码文件和外部库。 我们应该指定在相关代码文件中使用的库。 我们可以为外部库提供-l
参数。
$ gcc -O main.c -lm -o mybinary
Here -lm
will provide the C standard math library to be used in this application.
在这里-lm
将提供用于该应用程序的C标准数学库。
使用GCC检查代码质量 (Check Code Quality with GCC)
GCC has a good feature which will give suggestions about the code quality. This option will check written code in a more strict manner. But the code should be correct syntactically and compiled correctly. We will use -Wall
option to use this feature.
GCC具有良好的功能,可以提供有关代码质量的建议。 此选项将以更严格的方式检查书面代码。 但是代码在语法上应该正确并正确编译。 我们将使用-Wall
选项来使用此功能。
$ gcc -Wall main.c

显示GCC版本(Show GCC Version)
Version is an important aspect of compile operation. Because GCC gains, dismiss separate features in each version, and modifying related configuration is important. The version of GCC can get with -v
option. This will not provide only the version also give information on the configuration of GCC.
版本是编译操作的重要方面。 由于获得了GCC,因此请在每个版本中取消单独的功能,并且修改相关配置非常重要。 GCC的版本可以通过-v
选项获得。 这不仅会提供版本,还会提供有关GCC配置的信息。
$ gcc -v
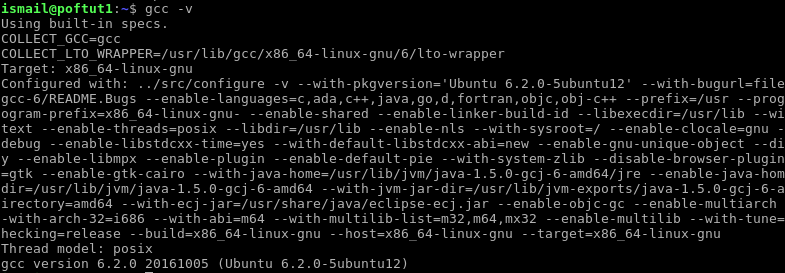
gcc编译器和g++编译器