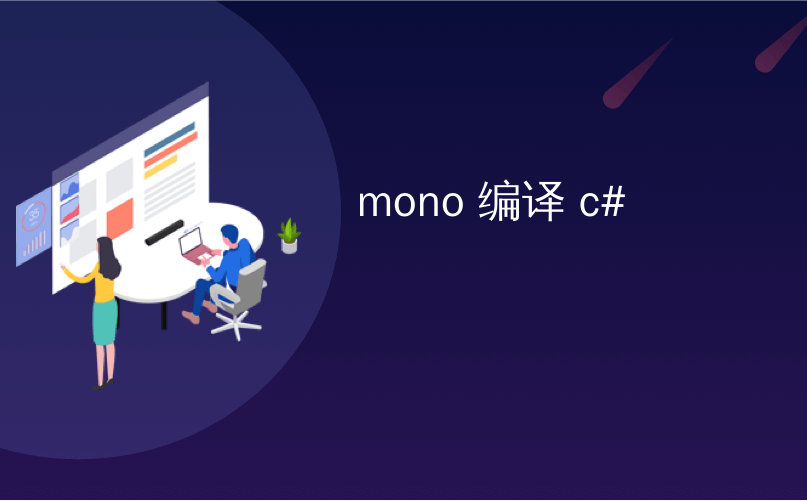
mono 编译 c#
Blazor quietly marches on. In case you haven't heard (I've blogged about Blazor before) it's based on a deceptively simple idea - what if we could run .NET Standard code in the browsers? No, not Silverlight, Blazor requires no plugins and doesn't introduce new UI concepts. What if we took the AOT (Ahead of Time) compilation work pioneered by Mono and Xamarin that can compile C# to Web Assembly (WASM) and added a nice UI that embraced HTML and the DOM?
开拓者悄悄地前进。 如果您没有听说过(我之前曾写过有关Blazor的文章),它是基于一个看似简单的想法-如果我们可以在浏览器中运行.NET Standard代码怎么办? 不,不是Silverlight,Blazor不需要插件,也不会引入新的UI概念。 如果我们接受Mono和Xamarin率先进行的AOT(提前)编译工作,该工作可以将C#编译为Web Assembly (WASM),并添加一个包含HTML和DOM的精美UI,该怎么办?
Sound bonkers to you? Are you a hater? Think this solution is dumb or not for you? To the left.
听起来很傻吗? 你讨厌吗? 认为此解决方案对您而言是愚蠢的吗? 在左边。
For those of you who want to be wacky and amazing, consider if you can do this and the command line:
对于那些想变得古怪而又令人惊奇的人,请考虑是否可以通过命令行执行此操作:
$ cat hello.cs
class Hello {
static int Main(string[] args) {
System.Console.WriteLine("hello world!");
return 0;
}
}
$ mcs -nostdlib -noconfig -r:../../dist/lib/mscorlib.dll hello.cs -out:hello.exe
$ mono-wasm -i hello.exe -o output
$ ls output
hello.exe index.html index.js index.wasm mscorlib.dll
Then you could do this in the browser...look closely on the right side there.
然后,您可以在浏览器中执行此操作...在右侧仔细查看。
You can see the Mono runtime compiled to WASM coming down. Note that Blazor IS NOT compiling your app into WASM. It's sending Mono (compiled as WASM) down to the client, then sending your .NET Standard application DLLs unchanged down to run within with the context of a client side runtime. All using Open Web tools. All Open Source.
您会看到编译为WASM的Mono运行时下降了。 请注意,Blazor不会将您的应用程序编译成WASM。 它向客户端发送Mono(作为WASM编译),然后向下发送未更改的.NET Standard应用程序DLL ,以便在客户端运行时的上下文中运行。 全部使用Open Web工具。 所有开源。
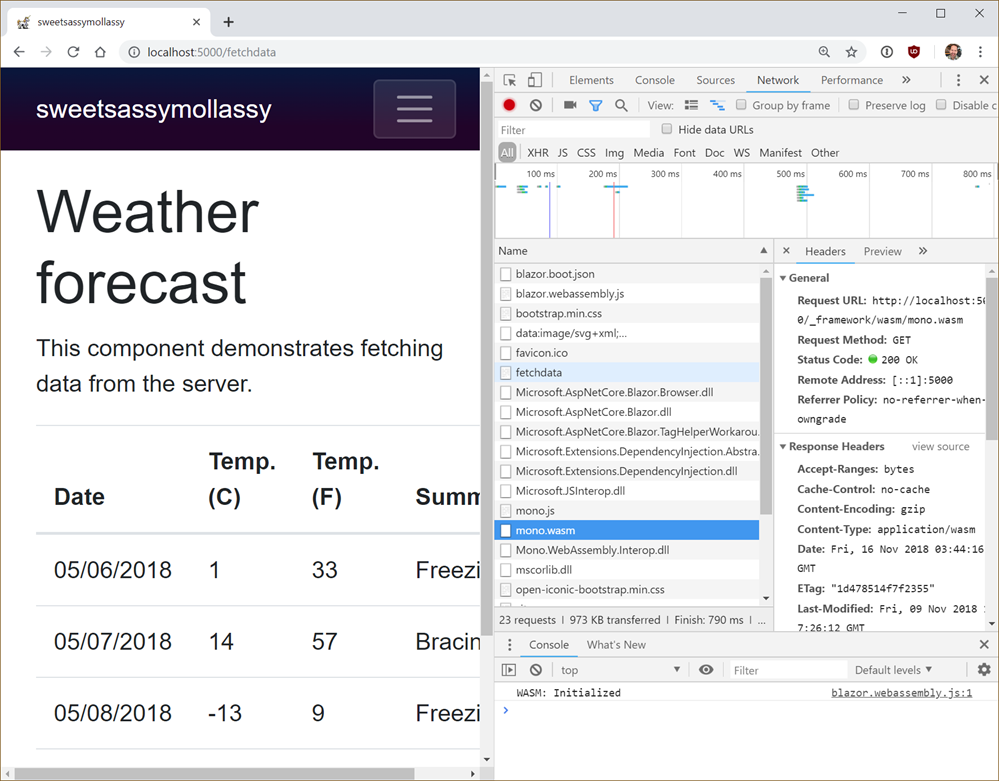
So Blazor allows you to make SPA (Single Page Apps) much like the Angular/Vue/React, etc apps out there today, except you're only writing C# and Razor(HTML).
因此, Blazor允许您像现在的Angular / Vue / React等应用程序一样制作SPA(单页应用程序),只是您只编写C#和Razor(HTML)。
Consider this basic example.
考虑这个基本示例。
@page "/counter"
<h1>Counter</h1>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" onclick="@IncrementCount">Click me</button>
@functions {
int currentCount = 0;
void IncrementCount() {
currentCount++;
}
}
You hit the button, it calls some C# that increments a variable. That variable is referenced higher up and automatically updated. This is trivial example. Check out the source for FlightFinder for a real Blazor application.
您单击按钮,它调用一些使变量递增的C#。 该变量被向上引用并自动更新。 这是简单的例子。 请查看FlightFinder的源代码,以获取真正的Blazor应用程序。
This is stupid, Scott. How do I debug this mess? I see you're using Chrome but seriously, you're compiling C# and running in the browser with Web Assembly (how prescient) but it's an undebuggable black box of a mess, right?
这太蠢了,斯科特。 我该如何调试这个烂摊子? 我看到您使用的是Chrome,但说真的,您正在编译C#并使用Web Assembly在浏览器中运行(多么有先见之明),但这是一个不能容忍的烂摊子,对吗?
I say nay nay!
我说不!
C:\Users\scott\Desktop\sweetsassymollassy> $Env:ASPNETCORE_ENVIRONMENT = "Development"
C:\Users\scott\Desktop\sweetsassymollassy> dotnet run --configuration Debug
Hosting environment: Development
Content root path: C:\Users\scott\Desktop\sweetsassymollassy
Now listening on: http://localhost:5000
Now listening on: https://localhost:5001
Application started. Press Ctrl+C to shut down.
Then Win+R and run this command (after shutting down all the Chrome instances)
然后Win + R并运行此命令(在关闭所有Chrome实例后)
%programfiles(x86)%\Google\Chrome\Application\chrome.exe --remote-debugging-port=9222 http://localhost:5000
Now with your Blazor app running, hit Shift+ALT+D (or Shift+SILLYMACKEY+D) and behold.
现在,在您的Blazor应用程序运行时,按Shift + ALT + D(或Shift + SILLYMACKEY + D)并观察。
Feel free to click and zoom in. We're at a breakpoint in some C# within a Razor page...in Chrome DevTools.
随时单击并放大。我们在Chrome DevTools中的Razor页面中的某些C#中处于断点。
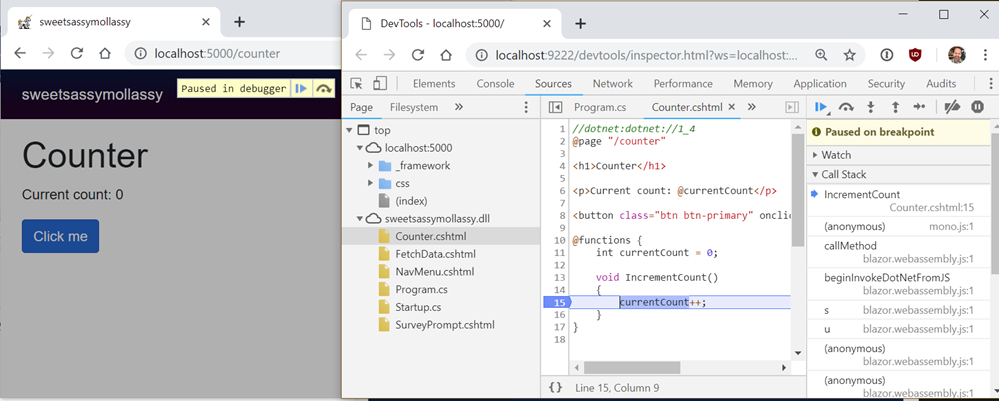
What? How?
什么? 怎么了
Blazor provides a debugging proxy that implements the Chrome DevTools Protocol and augments the protocol with .NET-specific information. When debugging keyboard shortcut is pressed, Blazor points the Chrome DevTools at the proxy. The proxy connects to the browser window you're seeking to debug (hence the need to enable remote debugging).
Blazor提供了一个调试代理,该代理可实现Chrome DevTools协议,并使用.NET特定的信息来扩展该协议。 按下调试键盘快捷键后,Blazor会将Chrome DevTools指向代理。 代理连接到您要调试的浏览器窗口(因此需要启用远程调试)。
It's just getting started. It's limited, but it's awesome. Amazing work being done by lots of teams all coming together into a lovely new choice for the open source web.
它才刚刚开始。 它是有限的,但是很棒。 许多团队共同完成了惊人的工作,成为开源Web的一个不错的新选择。
Sponsor: Preview the latest JetBrains Rider with its Assembly Explorer, Git Submodules, SQL language injections, integrated performance profiler and more advanced Unity support.
赞助商:预览最新的JetBrains Rider,包括其Assembly Explorer,Git子模块,SQL语言注入,集成的性能分析器以及更高级的Unity支持。
mono 编译 c#