java中什么是消息摘要
In Java, the Abstract List is the part of the Java Collection Framework. The Abstract list is implemented by the collection interface and the Abstract Collection class. This is used when the list can not be modified. To implement this AbstractList class is used with get() and size() methods.
在Java中,抽象列表是Java集合框架的一部分。 Abstract列表由collection接口和Abstract Collection类实现。 当无法修改列表时使用。 为了实现此AbstractList类,可将其与get()和size()方法一起使用。
下面是类层次结构 (Below is the class Hierarchy)
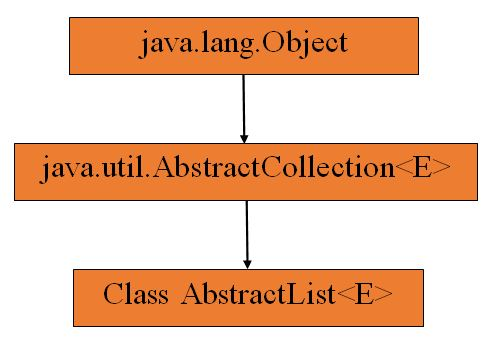
Syntax:
句法:
public abstract class AbstractList<E> extends AbstractCollection<E>implements List<E>
下面是AbstractList类的方法 (Below are the methods of AbstractList Class)
S.no. | Method | Description |
---|---|---|
1 | add(E e) | It is used to add an element to the end of the list. |
2 | add(int index, E element) | It is used to add an element at the specified position of the list. |
3 | add(int index, Collection c) | It is used to add an element in the specified collection in the specified position. |
4 | clear() | It is used to remove all the elements from the list. |
5 | equals(Object o) | It is used to compare an element from another element in the list. |
6 | get(int index) | It is used to get the element from the specified position in the list. |
7 | hashCode() | It is used to get hash code from the list. |
8 | indexOf(Object o) | It is used to get the first element from the list. If the list is empty then it returns -1. |
9 | Iterator() | It returns all the elements from the list. Using Iterator. |
10 | lastIndexOf(Object o) | It is used to get the Last element from the list. If the list is empty then it returns -1. |
11 | listIterator() | It is used to get the Iterated list in a proper sequence. |
12 | remove(int index) | It is used to remove the specified element from the list. |
13 | removeRange(intfromIndex, inttoIndex) | It is used to remove an element from the specified range. |
14 | set(int index, E element) | It is used to replace an element from the specified element. |
15 | subList(intfromIndex, inttoIndex) | It is used to get elements from the specified range. |
序号 | 方法 | 描述 |
---|---|---|
1个 | 加(E e) | 用于将元素添加到列表的末尾。 |
2 | add(int index,E元素) | 它用于在列表的指定位置添加元素。 |
3 | add(int index,Collection c) | 用于在指定位置的指定集合中添加元素。 |
4 | 明确() | 它用于从列表中删除所有元素。 |
5 | 等于(对象o) | 它用于比较列表中另一个元素的元素。 |
6 | get(int索引) | 它用于从列表中的指定位置获取元素。 |
7 | hashCode() | 它用于从列表中获取哈希码。 |
8 | indexOf(Object o) | 它用于从列表中获取第一个元素。 如果列表为空,则返回-1。 |
9 | Iterator() | 它返回列表中的所有元素。 使用迭代器。 |
10 | lastIndexOf(Object o) | 它用于从列表中获取Last元素。 如果列表为空,则返回-1。 |
11 | listIterator() | 它用于以适当的顺序获取迭代列表。 |
12 | remove(int index) | 用于从列表中删除指定的元素。 |
13 | removeRange(intfromIndex,inttoIndex) | 用于从指定范围内删除元素。 |
14 | set(int index,E元素) | 用于替换指定元素中的元素。 |
15 | subList(intfromIndex,inttoIndex) | 它用于获取指定范围内的元素。 |
例: (Example:)
import java.util.*;
public class AbstractListDemo1 {
public static void main(String args[])
{
AbstractList
list = new LinkedList
();
list.add("Dog");
list.add("Cat");
list.add("Bird");
list.add("Tiger");
list.add("Rabit");
System.out.println("***********************************");
System.out.println("Elements in the List 1:" + list);
list.add(3, "Deer");
System.out.println("***********************************************************");
System.out.println("After Adding Deer at position 3");
System.out.println("Elements in the List 1:" + list); //New List
System.out.println("***********************************************************");
AbstractList
list1 = new LinkedList
();
list1.add("Dog");
list1.add("Cat");
list1.add("Bird");
list1.add("Tiger");
list1.add("Rabit");
System.out.println("***********************************************************");
System.out.println("Elements in the List 2 :" + list1); //New List2
System.out.println("***********************************************************");
boolean ab = list.equals(list1);
System.out.println("Are both list equal : "+ ab);
System.out.println("***********************************************************");
String bc = list1.get(3);
System.out.println("Get element of index 3 : "+bc);
System.out.println("***********************************************************");
int cd= list.hashCode();
System.out.println("HashCode : " + cd);
System.out.println("***********************************************************");
int de= list.indexOf("Bird");
System.out.println("index of Bird in List 1: " + de);
System.out.println("***********************************************************");
Iterator ef = list.iterator();
while (ef.hasNext())
{
System.out.println("Element : "+ ef.next());
}
System.out.println("***********************************************************");
intfg= list.lastIndexOf("Rabit");
System.out.println("Last index of Rabit : "+ fg);
System.out.println("***********************************************************");
ListIteratorgh = list.listIterator(3);
System.out.println("The list is as follows:");
while (gh.hasNext()) {
System.out.println(gh.next());
}
System.out.println("***********************************************************");
}
}
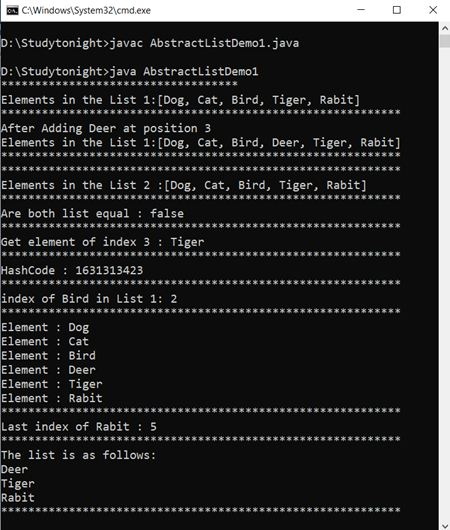
不可变列表 (Immutable List)
In Java, Immutable List is immutable. The Elements of the Immutable list are Fixed. It means the elements of the list are read-only. If we try to add, delete or update any element then it will throw UnsupportedOperationException. It also does not allow null elements. If we try to create a null element then it will throw NullPointerException and if we try to add null element then it will throw UnsupportedOperationException.
在Java中,不可变列表是不可变的。 不可变列表的元素是固定的。 这意味着列表的元素是只读的。 如果我们尝试添加,删除或更新任何元素,则它将引发UnsupportedOperationException。 它还不允许使用null元素。 如果尝试创建null元素,则将抛出NullPointerException;如果尝试添加null元素,则将抛出UnsupportedOperationException。
以下是ImmutableList的优点。 (Following are the Advantages of the ImmutableList.)
1. It is threaded safe.
1.它是线程安全的。
2. The memory is well organized.
2.内存组织良好。
下面是类层次结构 ( Below is the class Hierarchy )
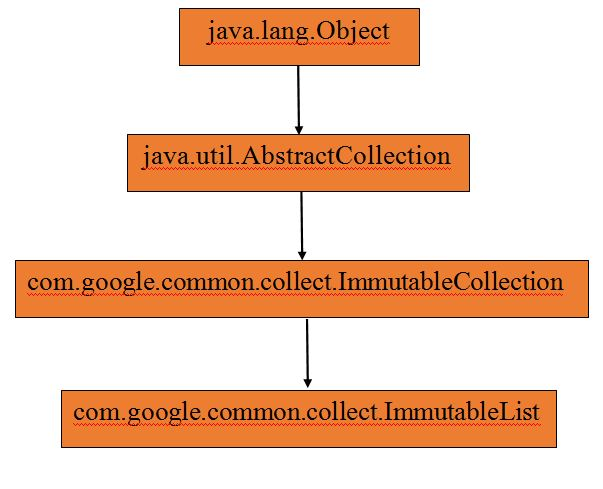
java中什么是消息摘要