python 平方根
There are many ways to find the square root of a number in Python.
有许多方法可以在Python中找到数字的平方根。
1.使用指数运算符计算数字的平方根 (1. Using Exponent Operator for Square Root of a Number)
num = input("Please enter a number:\n")
sqrt = float(num) ** 0.5
print(f'{num} square root is {sqrt}')
Output:
输出:
Please enter a number:
4.344
4.344 square root is 2.0842264752180846
Please enter a number:
10
10 square root is 3.1622776601683795
I am using float() built-in function to convert the user-entered string to a floating-point number.
我正在使用float()内置函数将用户输入的字符串转换为浮点数。
The input() function is used to get the user input from the standard input.
input()函数用于从标准输入获取用户输入。
2.平方根的数学sqrt()函数 (2. Math sqrt() function for square root)
Python math module sqrt() function is the recommended approach to get the square root of a number.
建议使用Python数学模块 sqrt()函数来获取数字的平方根。
import math
num = 10
num_sqrt = math.sqrt(num)
print(f'{num} square root is {num_sqrt}')
Output:
输出:
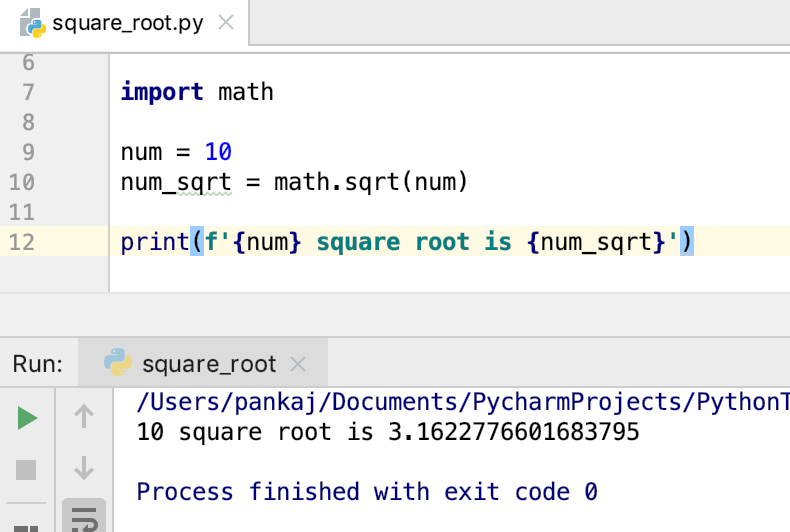
Python Square Root Of Number
Python的数字平方根
3.平方根的数学pow()函数 (3. Math pow() function for square root)
It’s not a recommended approach. But, the square root of a number is the same as the power of 0.5.
这不是推荐的方法。 但是,数字的平方根等于0.5的幂。
>>> import math
>>>
>>> math.pow(10, 0.5)
3.1622776601683795
>>>
4.复数的平方根 (4. Square Root of Complex Number)
We can use cmath module to get the square root of a complex number.
我们可以使用cmath模块获取复数的平方根。
import cmath
c = 1 + 2j
c_sqrt = cmath.sqrt(c)
print(f'{c} square root is {c_sqrt}')
Output:
输出:
(1+2j) square root is (1.272019649514069+0.7861513777574233j)
5.矩阵/多维数组的平方根 (5. Square Root of a Matrix / Multidimensional Array)
We can use NumPy sqrt() function to get the square root of a matrix elements.
我们可以使用NumPy sqrt()函数来获取矩阵元素的平方根。
翻译自: https://www.journaldev.com/32175/python-square-root-number
python 平方根