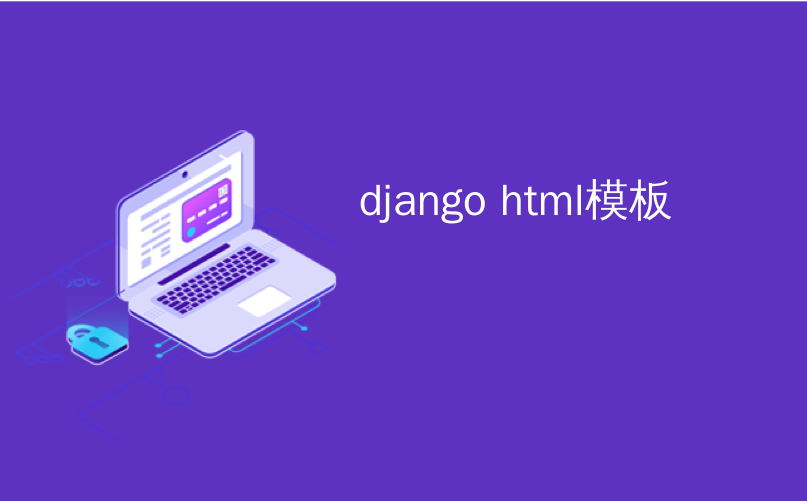
django html模板
In last tutorial we have seen, how we can return a HTML code through a string from views.py. It is not a good idea to pass whole HTML code through a string because if we have to design a complete webpage in HTML then we shouldn’t pass the whole code through the HttpResponse function because it will reduce the readibility of our code. So instead of passing the whole code through the HttpResponse function, we’ll create a separate HTML file.
在上一教程中,我们看到了如何通过views.py中的字符串返回HTML代码。 通过字符串传递整个HTML代码不是一个好主意,因为如果我们必须用HTML设计一个完整的网页,那么我们就不应该通过HttpResponse函数传递整个代码,因为这样会降低代码的可读性。 因此,我们将创建一个单独HTML文件,而不是通过HttpResponse函数传递整个代码。
In this tutorial we’ll see how we can add a seperate Template (HTML) in django project.
在本教程中,我们将看到如何在Django项目中添加单独的模板(HTML)。
A Template is not only a way for you to separate the HTML from these Python (views.py) but it also gives you the option to plugin some Django code inside those templates so those are not just some simple HTML files, that’s why they have the name Templates. We’ll learn a alot of about Templates in upcoming Django articles.
模板不仅是您从这些Python(views.py)分离HTML的一种方式,而且还为您提供了在这些模板中插入一些Django代码的选项,因此这些不仅仅是一些简单HTML文件,这就是为什么它们具有名称模板。 在即将发布的Django文章中,我们将学习有关模板的大量知识。
为前端创建单独HTML文件(模板) (Create Separate HTML File for Front End (Template))
In our project we need to create a folder that will hold our templates. So go to the top most level of our project where our manage.py file exists, create a folder named as templates. It will be like this:
在我们的项目中,我们需要创建一个文件夹来保存我们的模板。 因此,转到项目的最顶层(存在manage.py文件),创建一个名为template的文件夹。 它将是这样的:
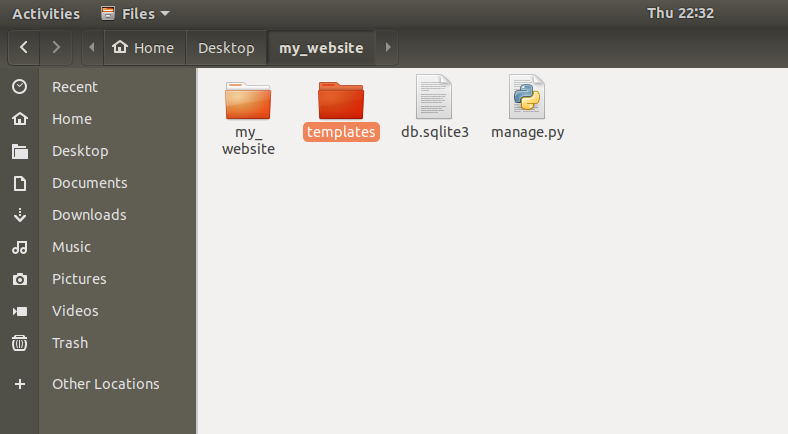
Now inside that templates folder let’s add our first HTML file. Let’s say we are going to make this file as homepage then just call it as home.html
现在,在该模板文件夹中,让我们添加第一个HTML文件。 假设我们要将此文件设为首页,然后将其命名为home.html
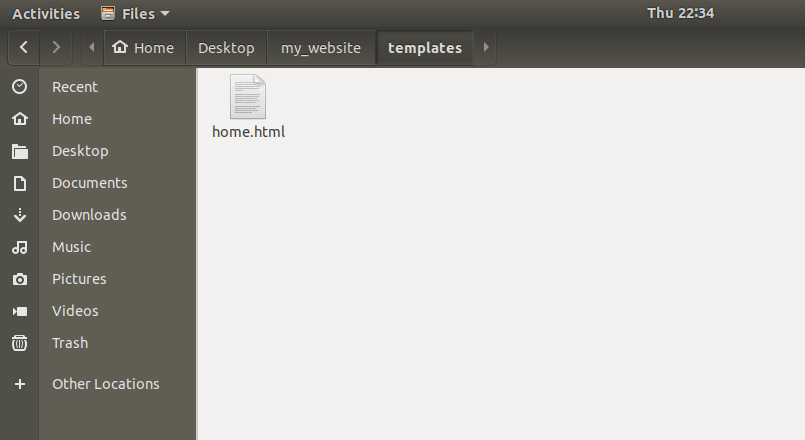
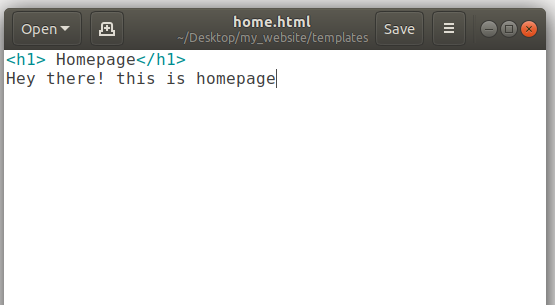
So now our task is that when someone is requesting the homepage then how we can send them to home.html.
因此,现在我们的任务是,当有人请求主页时,我们如何将其发送到home.html。
In order to do this follow the steps given below.
为此,请执行以下步骤。
Step 1: First we’ve to modify settings.py to tell our server that we’ve added a new template. So open settings.py and go to a variable named as TEMPLATES. Inside we have DIRS. Now you can see square brackets infront of DIRS.
第1步:首先,我们必须修改settings.py以告知服务器我们已经添加了新模板。 因此,打开settings.py并转到一个名为TEMPLATES的变量。 里面有DIRS。 现在您可以在DIRS前面看到方括号。
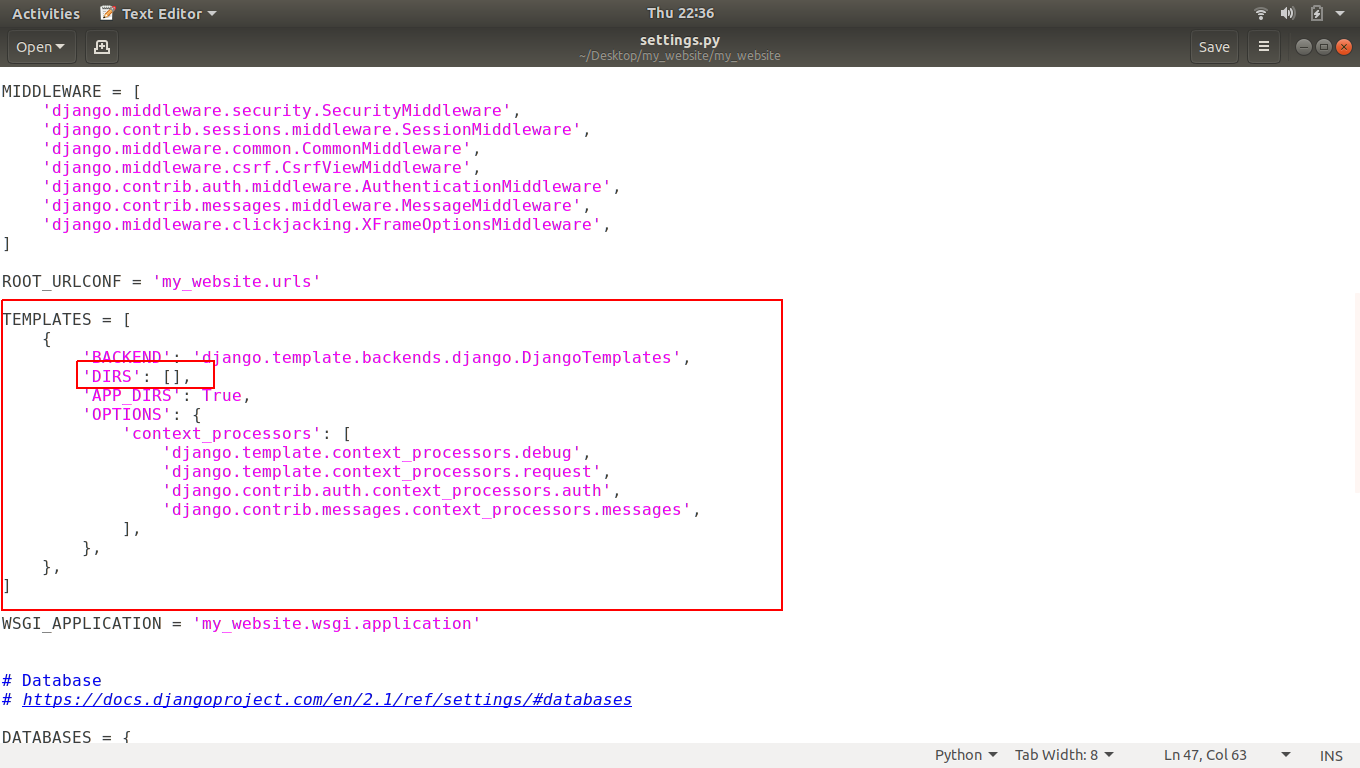
This little brackets is going to be a list of places where it should be looking for the templates. So let’s add the templates directory inside that brackets.
这个小括号将是应该寻找模板的地方的列表。 因此,让我们在括号内添加模板目录。
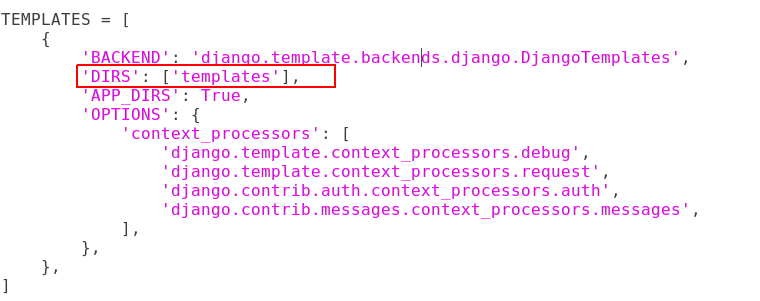
Step 2: As we know when someone is going to request your website, it will check into URLPATTERNS in urls.py file to check is there any path exists for requested URL or not? So we have to make a path for homepage.
第2步:我们知道有人要请求您的网站时,它将在urls.py文件中检入URLPATTERNS ,以检查请求的URL是否存在任何路径? 因此,我们必须为首页做好准备。
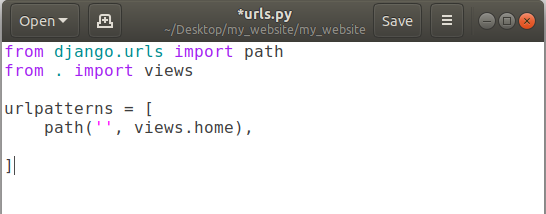
In above screenshot we’ve a set a path for homepage. If anyone requests our website then it will send that request to views.home function.
在上面的屏幕截图中,我们为首页设置了路径。 如果有人请求我们的网站,它将把该请求发送到views.home函数。
Step 3: As above step will send the request to views.home function so we should have a home function inside our views.py. We’ve already created it in our previous tutorial. If you doesn’t have views.py then please create it and add a home function into it like this:
步骤3:如上所述,将请求发送到views.home函数,因此我们应该在views.py内部具有home函数。 我们已经在之前的教程中创建了它。 如果您没有views.py,请创建它并向其中添加一个home函数,如下所示:
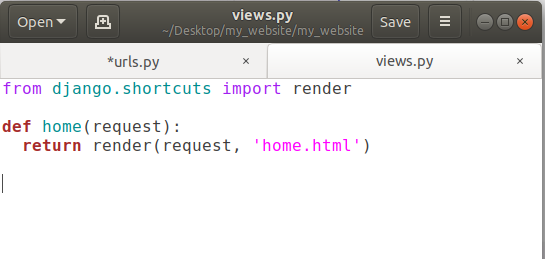
Now we have home function which is returning something.
现在我们有了home函数,该函数正在返回一些东西。
To open that HTML file, we have to use render function and render function takes two parameters, first one is request object and second is the name of the HTML file to open. To use render function we have to import something that’s why we used:
要打开该HTML文件,我们必须使用render函数,并且render函数需要两个参数,第一个是请求对象,第二个是要打开HTML文件的名称。 要使用渲染功能,我们必须导入一些东西,这就是我们使用的原因:
from django.shortcuts import render
从django.shortcuts导入渲染
Now we’re all set to go and run our project. To do that we have to run the server first. So open terminal or command prompt and go to the root of your project directory and run this command.
现在,我们都可以开始并运行我们的项目。 为此,我们必须首先运行服务器。 因此,打开终端或命令提示符,然后转到项目目录的根目录并运行此命令。
python3 manage.py runserver
python3 manage.py运行服务器
(for who have both python 2 and python 3 installed in their system like Ubuntu 18.04)
(对于在他们的系统(例如Ubuntu 18.04 )中同时安装了python 2和python 3的用户)
or
要么
python manage.py runserver
python manage.py运行服务器
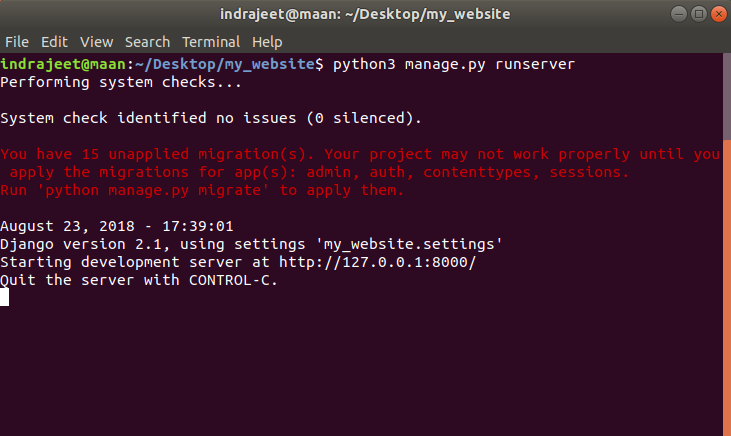
Now open our website using following link in the above image.
现在,使用上图中的以下链接打开我们的网站。
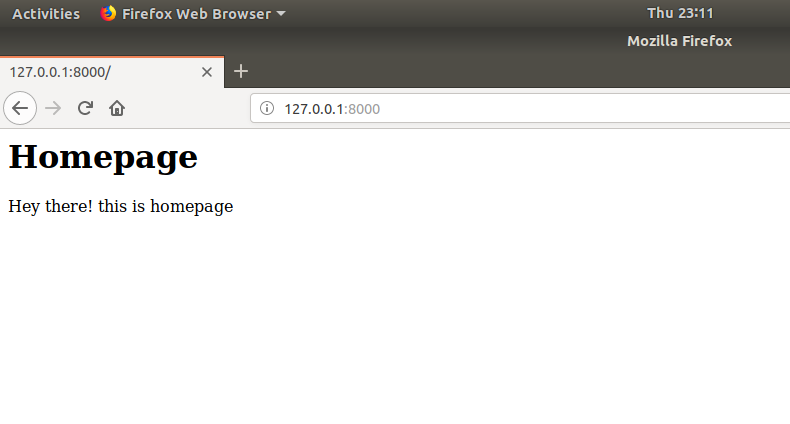
Finally we have our HTML Template opened in browser.
最后,我们在浏览器中打开了HTML模板。
As I mentioned earlier that this HTML file is not any regular HTML file, we can actually run some Python code inside this HTML.
如前所述,该HTML文件不是任何常规HTML文件,我们实际上可以在此HTML中运行一些Python代码。
For example: Let’s say I want to send some special information from our views.py to home.html then we can pass a dictionary through the render function as show in image below.
例如:假设我要从views.py向home.html发送一些特殊信息,然后可以通过render函数传递字典,如下图所示。
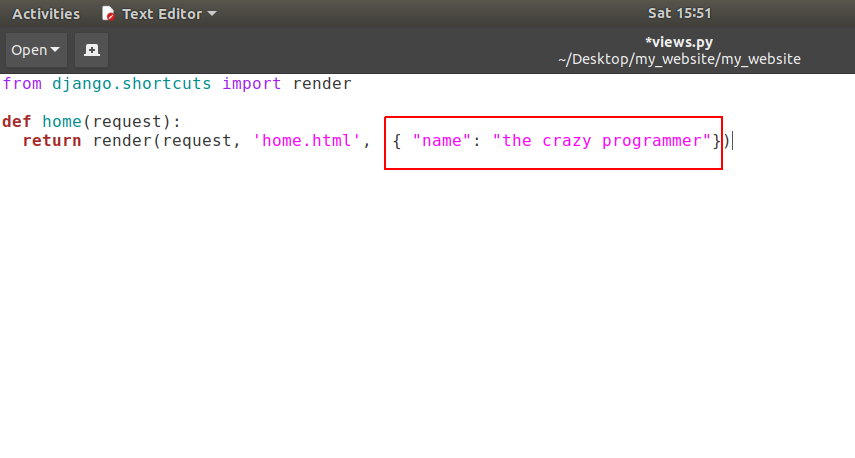
So we’re passing a dictionary here which have a key = name and a value associated with that key is the crazy programmer.
因此,我们在此处传递了一个字典,该字典具有key = name,并且与该key相关联的值是疯狂的程序员。
Now see how we can show this information in our HTML Template.
现在查看如何在HTML模板中显示此信息。
Open your home.html templateand edit it as shown in screenshot below:
打开home.html模板并对其进行编辑,如下面的屏幕截图所示:
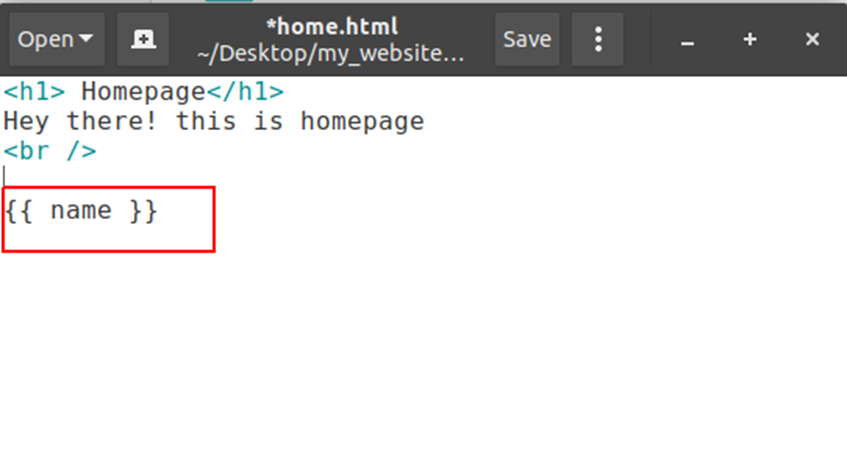
So here we are going to show our value assigned to that key name. If we pass any key inside two curly braces then it will give us the value of that key. So let’s run it in browser.
因此,在这里我们将显示分配给该键名的值。 如果我们在两个花括号内传递任何键,则它将为我们提供该键的值 。 因此,让我们在浏览器中运行它。
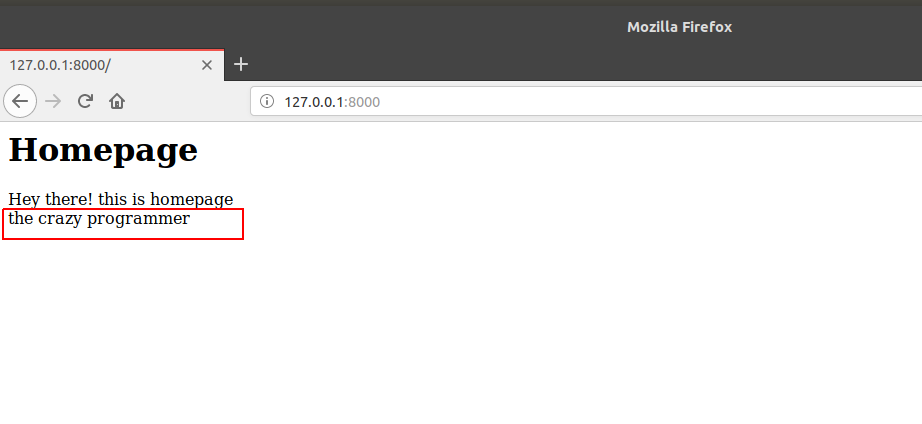
Thats how we can pass some information from python function to the HTML template in django.
那就是我们如何将一些信息从python函数传递到django中HTML模板。
I am sure your queries like:
我相信您的查询如下:
- How to create a custom HTML template page in django? 如何在Django中创建自定义HTML模板页面?
- How to pass information from Python function views to HTML template in django? 如何将信息从Python函数视图传递到Django中HTML模板?
Have been solved.
已经解决了。
If you’ve any query related to this tutorial then please let us know in comment box.
如果您对本教程有任何疑问,请在评论框中告诉我们。
翻译自: https://www.thecrazyprogrammer.com/2018/11/how-to-add-html-template-in-django.html
django html模板