1. Add this dependency to your project:
<dependency> <groupId>net.sf.json-lib</groupId> <artifactId>json-lib</artifactId> <version>2.3</version> <scope>compile</scope> </dependency>
What does this mean? See How to Add a Dependency to a Java Project
2. Put the following JSON sample in your classpath:
{'foo':'bar', 'coolness':2.0, 'altitude':39000, 'pilot':{'firstName':'Buzz', 'lastName':'Aldrin'}, 'mission':'apollo 11'}
3. Load the resource from the classpath and parse this JSON as follows:
package com.discursive.answers; import java.io.InputStream; import net.sf.json.JSONObject; import net.sf.json.JSONSerializer; import org.apache.commons.io.IOUtils; public class JsonParsing { public static void main(String[] args) throws Exception { InputStream is = JsonParsing.class.getResourceAsStream( "sample-json.txt"); String jsonTxt = IOUtils.toString( is ); JSONObject json = (JSONObject) JSONSerializer.toJSON( jsonTxt ); double coolness = json.getDouble( "coolness" ); int altitude = json.getInt( "altitude" ); JSONObject pilot = json.getJSONObject("pilot"); String firstName = pilot.getString("firstName"); String lastName = pilot.getString("lastName"); System.out.println( "Coolness: " + coolness ); System.out.println( "Altitude: " + altitude ); System.out.println( "Pilot: " + lastName ); } }
Note that JSONSerializer returns a JSON object. This is a general object which could be a JSONObject or a JSONArray depending on the JSON you are trying to parse. In this example, since I know that the JSON is a JSONObject, I can cast the result directly to a JSONObject. If you are dealing with JSON that could return a JSONArray, you'll likely want to check the type of the object that is returned by toJSON.
This sample project is available on GitHub here: http://github.com/to...le-json-parsing
For more information about the json-lib project, see JSON-LIB project page
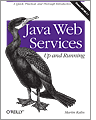
This quick, practical, and thorough introduction to Java web services -- the JAX-WS and JAX-RS APIs -- offers a mix of architectural overview, complete working code examples, and short yet precise instructions for compiling, deploying, and executing a sample application. You'll not only learn how to write web services from scratch, but also how to integrate existing services into your Java applications.

