关系运算
- 六个关系运算符:> < >= <= ==(相等) !=(不相等)
- 关系运算的结果是一个 布尔值(bool):true,false
- 在字符串中,0 1 2 ... 9 < A B C ... Z < a b c ... z
- 注意!数值 12 和 数值 12.0 不相等
逻辑运算
- 逻辑运算又叫布尔运算,结果是布尔值
- 三个运算符:and,or,not
- 运算符优先级: () 大于 ** 大于 * / % // 大于 > < >= <= == != 大于 and or not 大于 =(赋值运算符)
If 和 Else
- if 是流程控制语句,用来判断
- if 是分支结构,如下图
- if、else 语句格式要求使用 冒号 和 缩进,超级重要!!!
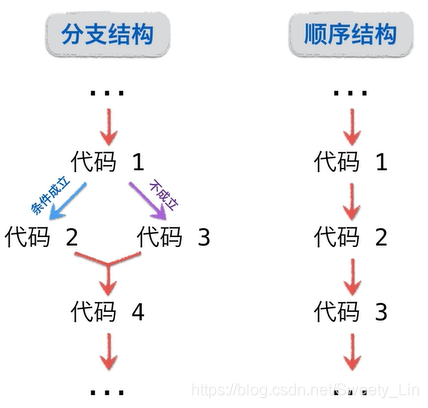
# 1.接收用户输入的数值
number = input('请输入一个整数:')
number = int(number)
# 2.判断这个数是不是偶数
if number % 2 == 0:
print(f"你输入的数是 {number},它是一个偶数")
else:
print(f"你输入的数是 {number},它是一个奇数")
# 3.再判断这个数能否被 3 整除
if number % 2 == 0:
print(f"你输入的数是 {number},它是一个偶数")
if number % 3 == 0:
print('这个数还能被3整除')
else:
print('这个数不能被3整除')
else:
print(f"你输入的数是 {number},它是一个奇数")
Elif 及注意事项
- if 语句中可以添加 elif 处理额外的判断条件
- elif 在 if 语句中不缩进
- if 后的条件中,只有 0、" 、None、空对象为 false,别的均可视为 true
##### 根据用户输入的考试分数确定成绩等级 #####
## 1.提示用户输入自己的考试分数
score = input('Please input your score(0-100):')
## 2.判断考试等级
'''
score level
100 S
90-99 A
80-89 B
70-79 C
60-69 D
0-60 E
'''
if score != '': # 或者 if score:
## 只有 " 、 0 、None、空对象为 false,别的均可视为 true
score = int(score)
if 0 <= score <= 100:
if score == 100:
print(f'Your score is {score},level S.')
elif score >= 90 and score <= 99: # 方法一
print(f'Your score is {score},level A.')
elif 80 <= score <= 89: # 方法二
print(f'Your score is {score},level B.')
elif score >= 70: # 方法三
print(f'Your score is {score},level C.')
elif score >= 60 and score <= 69:
print(f'Your score is {score},level D.')
else:
print(f'Your score is {score},level E.')
else:
print('Wrong Score! Please input an integer between 0 and 100!')
else:
print('No input was detected! Please input your score!')
If 语句练习
例 1. 提示用户输入一个年份,判断这一年是不是闰年
满足下列条件之一就是闰年:
- 能被 400 整除
- 能被 4 整除但不能被 100 整除
##### 提示用户输入一个年份,判断这一年是不是闰年 ######
## 1. 提示用户输入一个年份
year = input('Please input a year: ')
## 2. 判断是不是闰年
if year:
year = int(year)
if year > 0:
if (year % 400 == 0) or (year % 4 == 0 and year % 100 != 0):
print(f'{year} is a leap year.')
else:
print(f'{year} is not a leap year.')
else:
print('Wrong Input! Please input a right year!')
else:
print('No input was detected! Please enter a year!')
例 2. 提示用户输入一个 1 - 99999 之间的整数,分别显示这个数字的 个 十 百 千 万 位上的数字
##### 提示用户输入一个 1 - 99999 之间的整数
##### 分别显示这个数字的 个 十 百 千 万 位上的数字
## 1. 提示用户输入一个数字
number = input('Please input a number(0 - 99999): ')
## 2. 分别显示这个数字的 个 十 百 千 万 位上的数字
if number:
number = int(number)
if 0 <= number <= 99999:
ge = number % 10
shi = number // 10 % 10
bai = number // 100 % 10
qian = number // 1000 % 10
wan = number // 10000
print(f'个位:{ge}\n十位:{shi}\n百位:{bai}\n千位:{qian}\n万位:{wan}')
else:
print('Wrong Input! Please input a number between 0 and 99999!')
else:
print('No input was detected! Please enter a number(0-99999)!')
例 3. 实现一个剪刀、石头、布的猜拳游戏
- 玩家输入 1、2、3来表示剪刀、石头、布
- 程序随机(在 python 中生成随机数)选择剪刀、石头、布与玩家比较
##### 实现一个剪刀、石头、布的猜拳游戏
##### 玩家输入 1、2、3来表示剪刀、石头、布
##### 程序随机(在 python 中生成随机数)选择剪刀、石头、布与玩家比较
import random
## 1. 提示用户出拳
user = input('用户出拳(1-剪刀、2-石头、3-布): ')
## 2. 程序随机出拳
machine = random.randint(1,3)
print(f'机器出拳(1-剪刀、2-石头、3-布): {machine}')
## 3. 判断输赢
if user:
user = int(user)
if user == 1 or user == 2 or user == 3:
if user == machine:
print('平局')
elif (user == 1 and machine == 3) or (user == 2 and machine == 1) or (user == 3 and machine == 2):
print('玩家胜利!')
else:
print('机器胜利!')
else:
print('请按规定出拳!(1-剪刀、2-石头、3-布)')
else:
print('系统没有检测到您出拳,请出拳(1-剪刀、2-石头、3-布)!')